Mastering Classification Models: Advanced Techniques and Best Practices

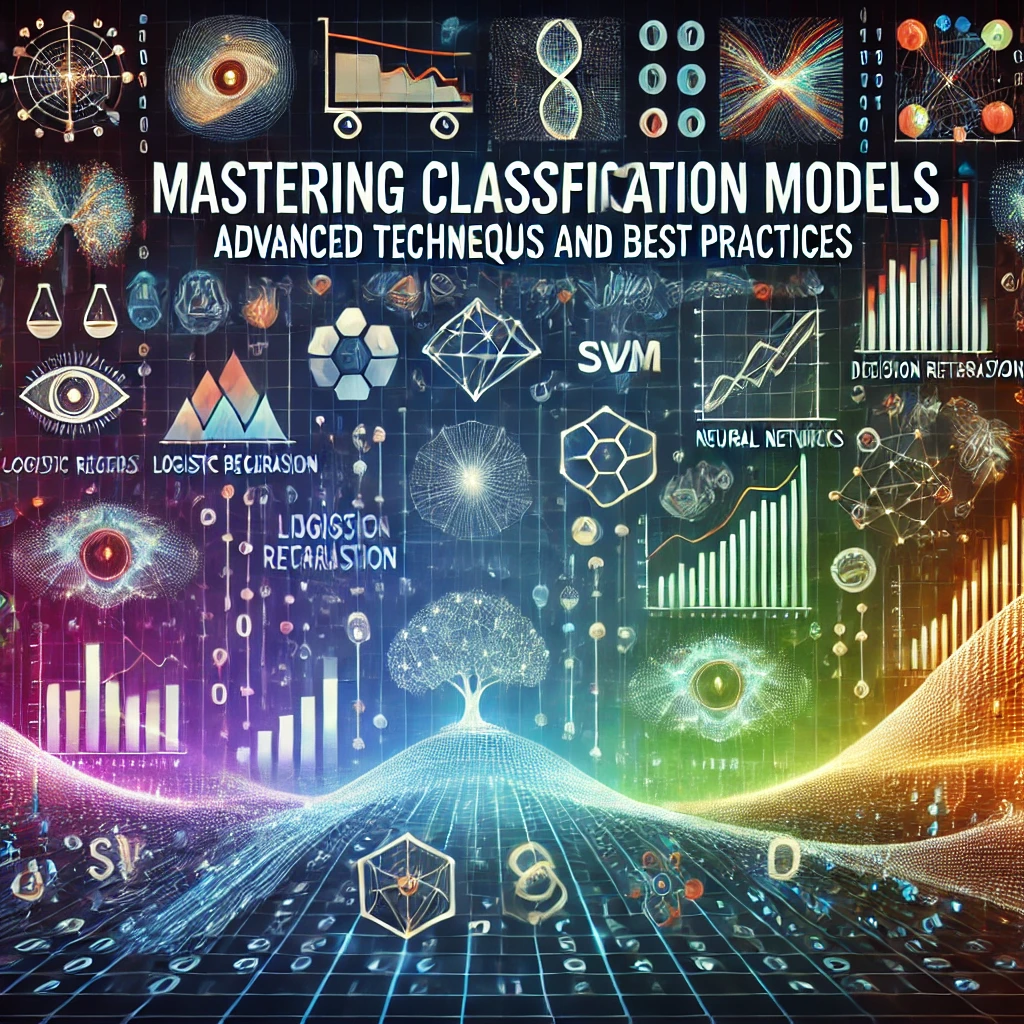
Part-II
Classification models are essential tools in the machine learning arsenal, widely used across various industries such as healthcare, finance, e-commerce, and more. They enable us to make informed decisions by predicting categorical outcomes based on input data. In this comprehensive guide, we'll explore advanced techniques and best practices for mastering classification models. We'll delve into definitions, specifications, industry applications, advantages, limitations, and provide real-world examples with step-by-step solutions and code snippets to help you implement these models effectively.
Logistic Regression
Definition
Logistic regression is a statistical model that uses a logistic function to model a binary dependent variable. It predicts the probability of an outcome that can have two possible values (e.g., yes or no, success or failure).
Specifications
Type: Binary Classification
Output: Probability score between 0 and 1
Common Use-Cases:
Medical diagnosis (disease vs. no disease)
Marketing (click vs. no click)
Industry Applications
Healthcare: Predicting the likelihood of a patient developing a disease.
Finance: Assessing the probability of loan default.
Marketing: Customer segmentation for targeted campaigns.
Advantages
Simple to implement and interpret.
Works well when the relationship between features and the target variable is approximately linear.
Limitations
Assumes a linear relationship between the features and the log-odds of the outcome.
Not suitable for complex relationships.
Example: Predicting Customer Purchase Behavior
Problem
Predict whether a customer will buy a product based on past purchasing behavior.
Solution Methodology
Prepare the Dataset
Collect features such as age, income, browsing time, and previous purchase history.
import pandas as pd from sklearn.model_selection import train_test_split # Load dataset df = pd.read_csv('customer_data.csv') X = df[['age', 'income', 'browsing_time']] y = df['buy_or_not']
Split the Data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Train the Logistic Regression Model
from sklearn.linear_model import LogisticRegression log_reg = LogisticRegression() log_reg.fit(X_train, y_train)
Predict and Evaluate
from sklearn.metrics import accuracy_score y_pred = log_reg.predict(X_test) accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Interpret the Results
The model provides probabilities that help in making business decisions.
probabilities = log_reg.predict_proba(X_test)
K-Nearest Neighbors (KNN)
Definition
K-Nearest Neighbors is a non-parametric algorithm that classifies data points based on the classes of their nearest neighbors in the feature space.
Specifications
Type: Instance-Based Learning, Non-Parametric
Parameters: Number of neighbors
k
, distance metric (e.g., Euclidean)Common Use-Cases:
Recommendation systems
Pattern recognition
Industry Applications
E-commerce: Product recommendations based on customer similarity.
Retail: Customer segmentation based on purchasing behavior.
Advantages
Simple to understand and implement.
Effective for small datasets with fewer features.
Limitations
Computationally intensive for large datasets.
Sensitive to the choice of
k
and distance metric.
Example: Movie Recommendation System
Problem
Recommend movies to users based on their preferences.
Solution Methodology
Prepare the Dataset
Features may include genres like action, comedy, drama, etc.
import pandas as pd from sklearn.model_selection import train_test_split # Load dataset movie_ratings = pd.read_csv('movie_ratings.csv') X = movie_ratings[['action', 'comedy', 'drama', 'romance']] y = movie_ratings['liked'] # 1: Liked, 0: Disliked
Split the Data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Train the KNN Model
from sklearn.neighbors import KNeighborsClassifier knn = KNeighborsClassifier(n_neighbors=5) knn.fit(X_train, y_train)
Predict and Evaluate
y_pred = knn.predict(X_test) from sklearn.metrics import accuracy_score accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Tune the Model
Experiment with different values of
k
to optimize performance.
Support Vector Machine (SVM)
Definition
Support Vector Machine is a supervised learning model that finds the optimal hyperplane which maximizes the margin between different classes.
Specifications
Type: Binary or Multiclass Classification
Parameters: Regularization parameter
C
, kernel type (linear, polynomial, RBF)Common Use-Cases:
Text classification
Image recognition
Industry Applications
Healthcare: Image classification for tumor detection.
Finance: Fraud detection.
Technology: Spam detection in emails.
Advantages
Effective in high-dimensional spaces.
Versatile with different kernel functions.
Limitations
Computationally intensive for large datasets.
Difficult to interpret the results.
Example: Face Recognition
Problem
Classify whether a given image contains a particular person's face.
Solution Methodology
Prepare the Dataset
Convert images into feature vectors.
from sklearn.model_selection import train_test_split # Assume X and y are prepared with image features and labels X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
Train the SVM Model
from sklearn.svm import SVC svm_model = SVC(kernel='rbf', C=1.0) svm_model.fit(X_train, y_train)
Predict and Evaluate
y_pred = svm_model.predict(X_test) from sklearn.metrics import accuracy_score accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Random Forest
Definition
Random Forest is an ensemble method that builds multiple decision trees using random subsets of data and features and aggregates their predictions.
Specifications
Type: Ensemble Learning, Bagging Method
Parameters: Number of trees (
n_estimators
), maximum depth (max_depth
)Common Use-Cases:
Risk assessment
Customer segmentation
Industry Applications
Finance: Predicting loan defaults.
Retail: Targeted marketing strategies.
Advantages
Reduces overfitting compared to individual decision trees.
Handles large datasets well.
Limitations
Less interpretable than individual trees.
Computationally intensive.
Example: Loan Approval Prediction
Problem
Predict whether a loan application should be approved.
Solution Methodology
Prepare the Dataset
Features include credit score, income, employment status, etc.
import pandas as pd from sklearn.model_selection import train_test_split loan_data = pd.read_csv('loan_data.csv') X = loan_data[['credit_score', 'income', 'employment_status']] y = loan_data['loan_approved'] # 1: Approved, 0: Not approved X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
Train the Random Forest Model
from sklearn.ensemble import RandomForestClassifier rf_model = RandomForestClassifier(n_estimators=100, max_depth=None) rf_model.fit(X_train, y_train)
Predict and Evaluate
y_pred = rf_model.predict(X_test) from sklearn.metrics import accuracy_score accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Feature Importance
importances = rf_model.feature_importances_ feature_names = X.columns forest_importances = pd.Series(importances, index=feature_names) print(forest_importances)
Naive Bayes
Definition
Naive Bayes is a probabilistic classifier based on Bayes' theorem with the assumption of feature independence.
Specifications
Type: Probabilistic Classifier
Assumption: Features are independent
Common Use-Cases:
Text classification
Sentiment analysis
Industry Applications
E-commerce: Analyzing customer reviews.
Media: Classifying news articles.
Advantages
Fast and efficient.
Performs well with high-dimensional data.
Limitations
- The independence assumption is often unrealistic.
Example: Sentiment Analysis
Problem
Classify customer reviews as positive or negative.
Solution Methodology
Prepare the Dataset
Collect and preprocess text data.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.feature_extraction.text import CountVectorizer reviews = pd.read_csv('reviews.csv') X = reviews['text'] y = reviews['sentiment'] # 1: Positive, 0: Negative vectorizer = CountVectorizer() X = vectorizer.fit_transform(X)
Split the Data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
Train the Naive Bayes Model
from sklearn.naive_bayes import MultinomialNB nb_model = MultinomialNB() nb_model.fit(X_train, y_train)
Predict and Evaluate
y_pred = nb_model.predict(X_test) from sklearn.metrics import accuracy_score accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy)
Gradient Boosting Machines (GBM) and AdaBoost
Definition
Gradient Boosting builds models sequentially, each correcting the errors of the previous one. AdaBoost adjusts weights on misclassified instances to focus on difficult cases.
Specifications
Type: Ensemble Learning, Boosting Method
Parameters: Learning rate, number of estimators
Common Use-Cases:
Price prediction
Fraud detection
Industry Applications
Real Estate: Predicting house prices.
Finance: Credit card fraud detection.
Advantages
High predictive accuracy.
Effective with complex datasets.
Limitations
Prone to overfitting if not tuned properly.
Computationally intensive.
Example: House Price Prediction
Problem
Predict house prices based on various features.
Solution Methodology
Prepare the Dataset
Features include square footage, number of bedrooms, location score, etc.
import pandas as pd from sklearn.model_selection import train_test_split house_data = pd.read_csv('house_data.csv') X = house_data[['square_feet', 'num_bedrooms', 'location_score']] y = house_data['price'] X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
Train the Gradient Boosting Model
from sklearn.ensemble import GradientBoostingRegressor gb_model = GradientBoostingRegressor(n_estimators=100, learning_rate=0.1) gb_model.fit(X_train, y_train)
Predict and Evaluate
y_pred = gb_model.predict(X_test) from sklearn.metrics import mean_absolute_error mae = mean_absolute_error(y_test, y_pred) print("Mean Absolute Error:", mae)
Neural Networks
Definition
Neural Networks consist of interconnected layers of nodes (neurons) that process data by adjusting weights and biases to minimize prediction errors.
Specifications
Type: Deep Learning, Multiclass Classification
Parameters: Number of layers, neurons per layer, activation functions, learning rate
Common Use-Cases:
Image classification
Natural language processing
Industry Applications
Healthcare: Disease detection from medical images.
Automotive: Autonomous driving systems.
Advantages
Capable of modeling complex patterns.
Versatile across various types of data.
Limitations
Requires large datasets and computational resources.
Often considered a "black box" due to lack of interpretability.
Example: Handwritten Digit Recognition
Problem
Classify handwritten digits from the MNIST dataset.
Solution Methodology
Load and Preprocess the Data
import tensorflow as tf (X_train, y_train), (X_test, y_test) = tf.keras.datasets.mnist.load_data() X_train, X_test = X_train / 255.0, X_test / 255.0
Build the Neural Network Model
from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten model = Sequential([ Flatten(input_shape=(28, 28)), Dense(128, activation='relu'), Dense(10, activation='softmax') ])
Compile and Train the Model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(X_train, y_train, epochs=5)
Evaluate the Model
test_loss, test_acc = model.evaluate(X_test, y_test) print("Test accuracy:", test_acc)
Conclusion
Mastering classification models is vital for tackling complex problems across various industries. By understanding the definitions, specifications, industry applications, and practical implementations of these models, you can select and apply the most appropriate techniques for your specific use cases.
Subscribe to my newsletter
Read articles from Riya Bose directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
