Python for Beginners: Unlock the Power of print(), Variables, and Data Types

Table of contents
- What is the print() Function in Python?
- Example Usage of the print() Function in Python
- Combining Multiple Python print() Statements in One Line
- How to Get User Input in Python
- Using Python’s max() and min() Functions
- Best Practices for Naming Variables in Python
- Understanding Python Data Types
- Understanding Python Complex Data Types
- 🎉 Conclusion: Mastering Python’s Basics
- 🔥FAQs
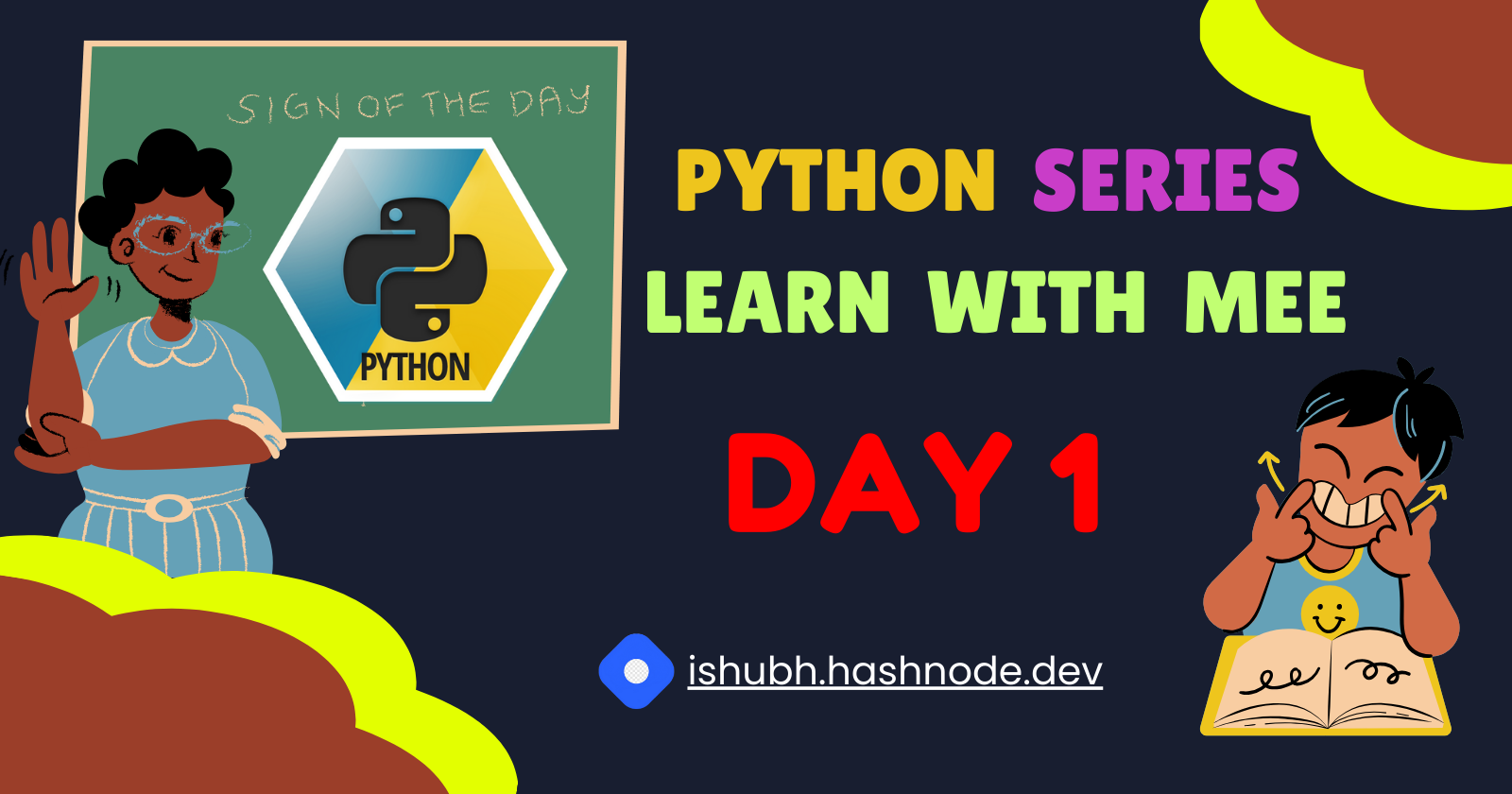
Python is one of the most beginner-friendly programming languages, and the print()
function is often the first function new developers encounter. Understanding how to use it effectively, along with other Python fundamentals like variable naming and data types, can significantly improve your programming skills. In this guide, we’ll break down the print()
function and explore essential Python concepts that every programmer should know. #2Articles1Week, #Hashnode.
What is the print()
Function in Python?
The print()
function outputs data to the console. It accepts several optional parameters that can modify the format of the output, making it a powerful tool for developers.
Key Parameters of the print()
Function
self
: Refers to the instance of the class in object-oriented programming (OOP).*args
: Allows passing multiple arguments to the function.sep
: Determines how the output elements are separated (default is a space).end
: Defines what will be printed at the end of the statement (default is a newline).file
: Redirects output to a file, instead of printing it to the console.
Example Usage of the print()
Function in Python
Here’s how you can use the print()
function in different ways:
print("Hello", "world", 123, True, 3.14)
# Output: Hello world 123 True 3.14
Now, let’s customize the separator:
print("Hello", "world", 123, True, 3.14, sep="-")
# Output: Hello-world-123-True-3.14
This flexibility makes print()
a powerful function for formatting your output.
Combining Multiple Python print()
Statements in One Line
By default, Python prints each statement on a new line. However, using the end
parameter, you can combine multiple print()
statements on the same line.
print("ant", "Parrot", "Rat", "Elephant", end=" ")
print("Apple", "Grapes", "Cherry", "Nuts")
# Output: ant Parrot Rat Elephant Apple Grapes Cherry Nuts
How to Get User Input in Python
Interacting with users is made easy with the input()
function. You can prompt users for input and store their responses in variables.
name = input("Enter your name: ")
empId = input("Enter your employee ID: ")
designation = input("Enter your designation: ")
print("------------ Your Information ------------")
print("Name: " + name, "Employee ID: " + empId, "Designation: " + designation)
Using Python’s max()
and min()
Functions
Python provides built-in functions like max()
and min()
to find the largest and smallest numbers from a list of arguments.
print(max(12, 56, 234, -89, 0, 34, 22, 456, -23, 785, 234))
# Output: 785
print(min(12, 56, 234, -89, 0, 34, 22, 456, -23, 785, 234))
# Output: -89
Best Practices for Naming Variables in Python
When naming variables in Python, follow these rules and best practices:
Start with a letter or underscore: Variable names must begin with a letter (A-Z or a-z) or an underscore (_).
Avoid starting with numbers: Variable names cannot begin with a digit.
Avoid using Python keywords: Keywords like
True
,None
, andstr
are reserved by Python and cannot be used as variable names.
Examples of Valid and Invalid Variable Names
Valid examples:
first_name = "John"
_age = 25
salary = 50000
Invalid examples:
12name = "John" # Invalid: starts with a number
name& = 77 # Invalid: contains a special character
Understanding Python Data Types
Python is a dynamically typed language, meaning you don’t need to specify data types when declaring variables. However, understanding data types is crucial for effective programming.
Here are some common data types in Python:
Integers: Whole numbers, both positive and negative (
1
,-12
,123
).Floating-point numbers: Decimal values (
18.0
,14.5
).Strings: Text values enclosed in quotes (
"Hello World"
,"123"
).Booleans: Represents
True
orFalse
.Complex numbers: Represented by (
5 + 3j
).
You can use the type()
function to determine the data type of any variable:
marks = 86
print(type(marks)) # Output: <class 'int'>
marks = "86"
print(type(marks)) # Output: <class 'str'>
Understanding Python Complex Data Types
Python also supports complex data types like:
Lists
Tuples
Dictionaries
Sets
Classes and Objects
Functions, Modules, and Packages
🎉 Conclusion: Mastering Python’s Basics
Learning how to effectively use the print()
function and other Python basics like variable naming, data types, and user input is essential for any Python developer. Mastering these foundational elements will set you up for success as you dive deeper into programming.
Remember, Python is all about simplicity and readability. Keep practicing, and you’ll become proficient in no time!
🔥FAQs
👉 What is the print()
function in Python?
The print()
function in Python outputs data to the console. It can format the output using optional parameters like sep
and end
.
👉 How do I combine multiple print()
statements in Python?
You can use the end
parameter to prevent print()
from starting a new line after printing. This allows multiple print()
statements to be displayed on the same line.
👉 How do I find the maximum and minimum values in Python?
Python’s built-in max()
and min()
functions can be used to find the largest and smallest values from a list of numbers.
Happy Coding ❤
Subscribe to my newsletter
Read articles from Shubham Sutar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Sutar
Shubham Sutar
"Tech enthusiast and blogger exploring the latest in gadgets, software, and innovation. Passionate about simplifying tech for everyday users and sharing insights on trends that shape the future."