Importance of Linting: Why You Should Be Using a Linter in Your Code

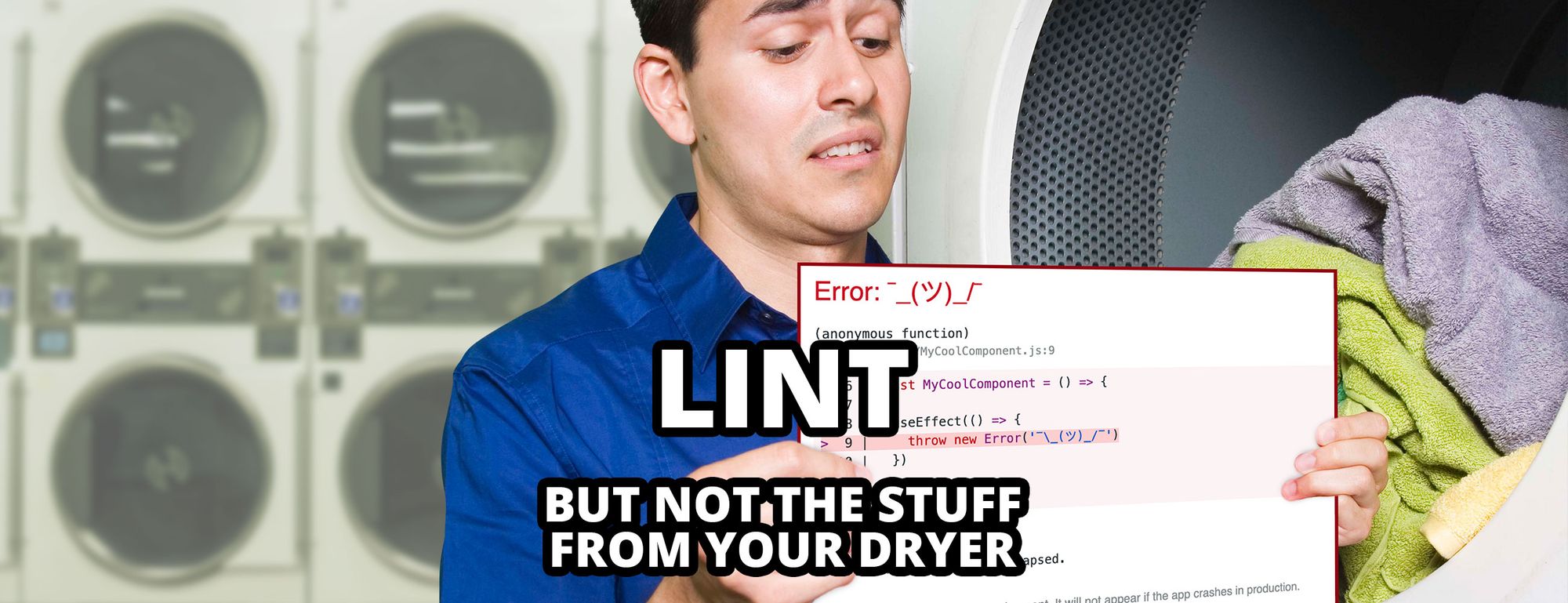
The Importance of Linting: Why You Should Be Using a Linter in Your Code
As developers, we’ve all been there - pouring over lines of code, trying to track down a pesky bug or error. But what if I told you there’s a tool that can help you catch those errors before they even happen? Enter linting, a crucial step in the coding process that can save you time, reduce frustration, and improve the overall quality of your code.
What is Linting?
Linting is the process of analyzing your code for potential errors, warnings, and best practices. A linter is a tool that checks your code against a set of predefined rules, flagging any issues it finds. These rules can range from simple syntax checks to more complex style and security guidelines.
Why is Linting Important?
Linting is important for several reasons:
Catches Errors Early: Linting helps you catch errors and bugs early in the development process, before they become major issues. This saves you time and reduces the likelihood of downstream problems.
Improves Code Quality: Linting enforces coding standards and best practices, ensuring that your code is consistent, readable, and maintainable.
Reduces Security Risks: Linting can help identify potential security vulnerabilities, such as SQL injection or cross-site scripting (XSS) attacks.
Enhances Collaboration: Linting ensures that all team members are following the same coding standards, making it easier to collaborate and review code.
Examples of Linting in Action
Here are some examples of linting in action:
- Syntax Errors:
if (x > 5)
console.log('x is greater than 5')
Linting would flag this code as an error because it’s missing a semicolon at the end of the console.log
statement.
- Undefined Variables:
console.log(x);
Linting would flag this code as an error because x
hasn’t been defined anywhere.
- Unused Variables:
let x = 5;
console.log('Hello, world!');
Linting would flag the x
variable as unused because it’s not being used anywhere in the code.
- Typo Errors:
let myVaraible = 5;
console.log(myVaraible);
Linting would flag the myVaraible
variable as a typo error because it’s likely meant to be myVariable
.
- Code Style:
if (x > 5) {
console.log('x is greater than 5');
}
Linting would flag this code as an error if the project’s code style rules require 2 spaces of indentation instead of 4.
- Security Vulnerabilities:
let userInput = 'alert("XSS")';
eval(userInput);
Linting would flag this code as a security vulnerability because it’s using eval()
with user-input data.
- Best Practices:
let PI = 3.14;
Linting would suggest using const
instead of let
for the PI
variable because it’s a constant value.
How to Get Started with Linting
Getting started with linting is easy. Here are a few steps to follow:
Choose a Linter: There are many linters available, including ESLint, JSLint, and TSLint. Choose one that fits your needs and integrates with your development environment.
Configure Your Linter: Configure your linter to follow your project’s coding standards and best practices.
Run Your Linter: Run your linter on your code to identify any issues or errors.
Fix Issues: Fix any issues or errors identified by your linter.
Conclusion
Linting is an essential step in the coding process that can save you time, reduce frustration, and improve the overall quality of your code. By catching errors early, enforcing coding standards, and identifying potential security vulnerabilities, linting helps ensure that your code is consistent, readable, and maintainable. So why not give linting a try? Your code (and your sanity) will thank you!
Subscribe to my newsletter
Read articles from Vishesh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
