Chapter 7: Patterns (Part 2) - Java With DSA
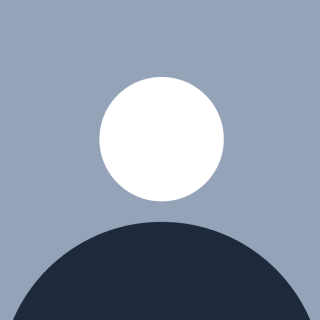
Table of contents
- Introduction
- i) Hollow Rectangle Pattern
- ii) Dry Run: Hollow Rectangle Pattern
- iii) Inverted and Rotated Half Pyramid
- iv) Inverted Half Pyramid with Numbers
- v) Floyd’s Triangle Pattern
- vi) 0-1 Triangle Pattern
- vii) Butterfly Pattern
- viii) Butterfly Pattern Code Explanation
- ix) Solid Rhombus Pattern
- x) Hollow Rhombus Pattern
- xi) Diamond Pattern
- Conclusion
Introduction
In this chapter, we'll delve into more complex pattern problems using nested loops. We'll focus on hollow shapes, inverted patterns, Floyd's triangle, 0-1 patterns, and more. These patterns are essential for improving logical thinking and mastering control over loops in Java.
i) Hollow Rectangle Pattern
A hollow rectangle is similar to a regular rectangle but with hollow interiors. The borders are printed, while the inner part remains empty.
For example, a hollow rectangle of 5 rows and 6 columns:
******
* *
* *
* *
******
Java Code:
public class HollowRectangle {
public static void main(String[] args) {
int rows = 5, cols = 6;
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= cols; j++) {
if (i == 1 || i == rows || j == 1 || j == cols) {
System.out.print("*");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
Explanation:
The outer loop runs for each row, and the inner loop runs for each column.
The condition
if (i == 1 || i == rows || j == 1 || j == cols)
ensures that the stars are printed only on the boundaries.
ii) Dry Run: Hollow Rectangle Pattern
To better understand the above pattern, let’s dry-run the code for a hollow rectangle:
iii) Inverted and Rotated Half Pyramid
An inverted and rotated half pyramid looks like this:
*
**
***
****
*****
Java Code:
public class InvertedRotatedPyramid {
public static void main(String[] args) {
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n - i; j++) {
System.out.print(" ");
}
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
Explanation:
The outer loop controls the rows.
The first inner loop prints spaces, and the second inner loop prints stars, creating the rotated effect.
iv) Inverted Half Pyramid with Numbers
This pattern is an inverted half pyramid but with numbers:
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
Java Code:
public class InvertedHalfPyramidNumbers {
public static void main(String[] args) {
int n = 5;
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= i; j++) {
System.out.print(j + " ");
}
System.out.println();
}
}
}
Explanation:
The outer loop controls the rows, which decrease from
n
to 1.The inner loop prints numbers from 1 to
i
, wherei
decreases in each iteration.
v) Floyd’s Triangle Pattern
Floyd's Triangle is a right-angled triangular array of natural numbers:
1
2 3
4 5 6
7 8 9 10
Java Code:
public class FloydsTriangle {
public static void main(String[] args) {
int n = 5, num = 1;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(num + " ");
num++;
}
System.out.println();
}
}
}
Explanation:
The outer loop controls the rows.
The inner loop prints increasing numbers, starting from 1 and incrementing with each iteration.
vi) 0-1 Triangle Pattern
A 0-1 triangle pattern alternates between 0 and 1:
1
0 1
1 0 1
0 1 0 1
Java Code:
public class ZeroOneTriangle {
public static void main(String[] args) {
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
if ((i + j) % 2 == 0) {
System.out.print("1 ");
} else {
System.out.print("0 ");
}
}
System.out.println();
}
}
}
Explanation:
The outer loop controls the rows.
The inner loop prints 1 or 0 based on whether the sum of the row and column indices is even or odd.
vii) Butterfly Pattern
The butterfly pattern looks like:
* *
** **
*** ***
********
********
*** ***
** **
* *
Java Code:
public class ButterflyPattern {
public static void main(String[] args) {
int n = 4;
// Upper part
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
for (int j = 1; j <= 2 * (n - i); j++) {
System.out.print(" ");
}
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
System.out.println();
}
// Lower part
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
for (int j = 1; j <= 2 * (n - i); j++) {
System.out.print(" ");
}
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
viii) Butterfly Pattern Code Explanation
The first part of the butterfly is printed by the upper loop, where the number of stars increases from 1 to
n
.The lower part is the mirror image of the upper part, where stars decrease from
n
back to 1.
ix) Solid Rhombus Pattern
A solid rhombus is a parallelogram made of stars:
*****
*****
*****
*****
*****
Java Code:
public class SolidRhombus {
public static void main(String[] args) {
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n - i; j++) {
System.out.print(" ");
}
for (int j = 1; j <= n; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
x) Hollow Rhombus Pattern
A hollow rhombus is similar to the solid one but with a hollow interior:
*****
* *
* *
* *
*****
Java Code:
public class HollowRhombus {
public static void main(String[] args) {
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n - i; j++) {
System.out.print(" ");
}
for (int j = 1; j <= n; j++) {
if (i == 1 || i == n || j == 1 || j == n) {
System.out.print("*");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
xi) Diamond Pattern
A diamond pattern looks like this:
*
***
*****
*****
***
*
Java Code:
public class DiamondPattern {
public static void main(String[] args) {
int n = 5;
// Upper half
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n - i; j++) {
System.out.print(" ");
}
for (int j = 1; j <= 2 * i - 1; j++) {
System.out.print("*");
}
System.out.println();
}
// Lower half
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= n - i; j++) {
System.out.print(" ");
}
for (int j = 1; j <= 2 * i - 1; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
Conclusion
This chapter covered various complex patterns using nested loops in Java, ranging from hollow rectangles to intricate diamond shapes. With each pattern, you practiced controlling loops and logical thinking. In the upcoming chapters, we’ll introduce methods and functions, which will allow us to modularize and optimize our code further.
Follow my journey through the Java with DSA series as we explore more exciting concepts!
Related Posts:
You might be interested in exploring more detailed topics in my ongoing series:
- Full Stack Java Development: A comprehensive guide to becoming a full stack Java developer.
Connect with me:
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊