Automation Using Node.js: A Powerful Tool for Efficiency
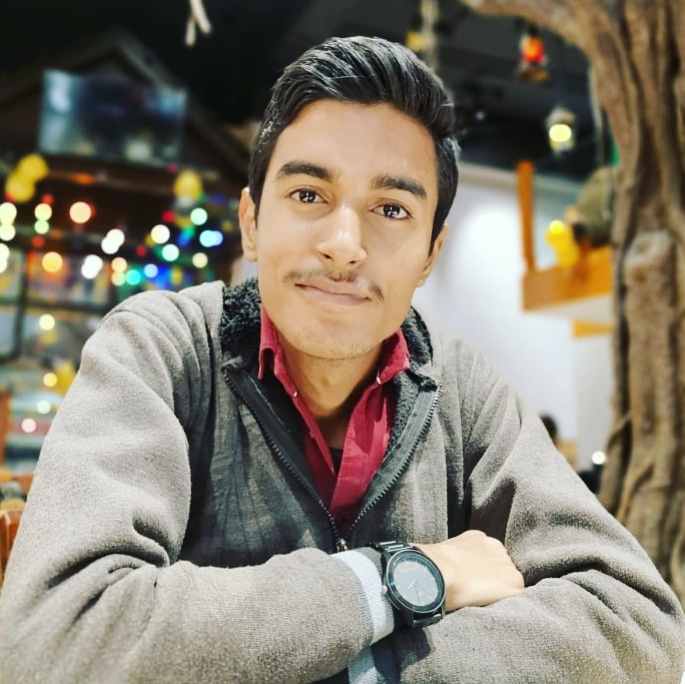
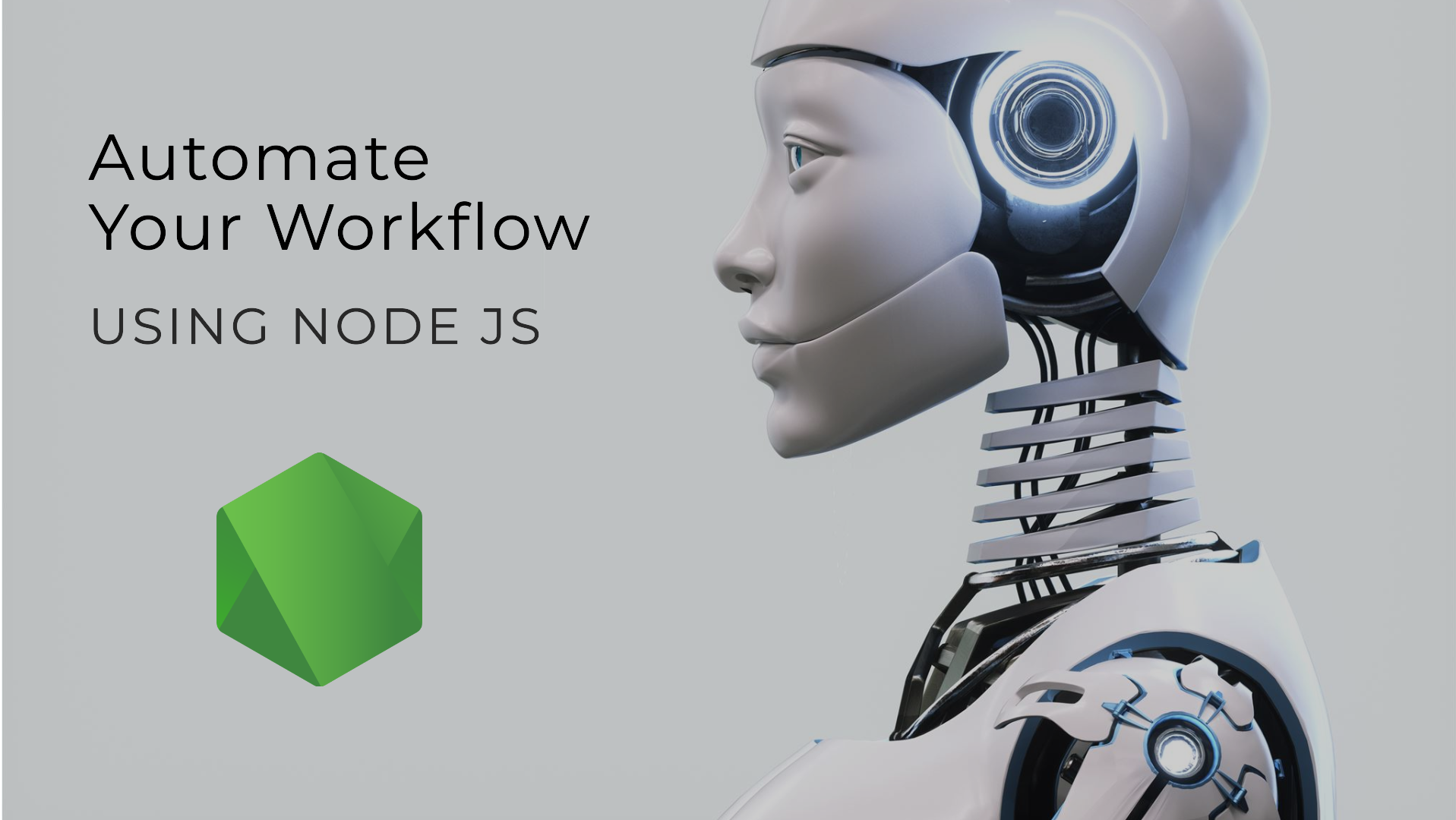
Node.js, a popular JavaScript runtime environment, has gained significant traction for its versatility and efficiency in building scalable web applications. Beyond web development, Node.js can be a powerful tool for automating various tasks, streamlining workflows, and increasing productivity. In this blog post, we'll explore how Node.js can be leveraged for automation and delve into some real-world use cases.
Why Node.js for Automation?
Asynchronous Programming: Node.js's event-driven, non-blocking architecture allows it to handle multiple tasks concurrently without blocking the main thread. This is particularly beneficial for automation scenarios where tasks might involve I/O operations like file manipulation, network requests, or database interactions.
Rich Ecosystem: The vast Node.js ecosystem offers a wide range of modules and libraries that can be easily integrated into your automation projects. These modules provide pre-built functionalities for common tasks, saving you time and effort.
JavaScript Familiarity: If you're already comfortable with JavaScript, learning Node.js for automation will be a smooth transition. The language's syntax and concepts are consistent, making it easier to pick up automation tasks.
Cross-Platform Compatibility: Node.js applications can run on Windows, macOS, and Linux, making them adaptable to various environments.
Common Automation Use Cases
Task Scheduling
Use libraries like node-schedule
or cron
to schedule recurring tasks, such as sending automated emails, running backups, or executing data analysis scripts.
Example:
const schedule = require('node-schedule');
const job = schedule.scheduleJob('0 0 * * *', () => {
console.log('Running scheduled task at midnight.');
});
Web Scraping
Extract data from websites using modules like puppeteer
, cheerio
, or request
. This can be useful for gathering market data, price comparisons, or monitoring website changes.
Example:
const puppeteer = require('puppeteer');
async function scrapeData() {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const data = await page.evaluate(() => {
const elements = document.querySelectorAll('.product-price');
return Array.from(elements).map(element => element.textContent);
});
console.log(data);
await browser.close();
}
scrapeData();
API Interactions
Automate interactions with APIs to fetch data, send requests, or trigger actions. Node.js provides modules like axios
and request
for making HTTP requests.
Example:
const axios = require('axios');
async function fetchData() {
const response = await axios.get('https://api.example.com/data');
const data = response.data;
console.log(data);
}
fetchData();
File and Directory Management
Automate tasks like creating, renaming, copying, and deleting files and directories using modules like fs
(Node.js built-in) or rimraf
.
Example:
const fs = require('fs');
fs.mkdir('new_directory', { recursive: true }, (err) => {
if (err) throw err;
console.log('Directory created successfully!');
});
Deployment and Testing
Automate the deployment of applications to servers or cloud platforms. Node.js can also be used for automating testing processes using frameworks like Jest
or Mocha
.
Example:
const { exec } = require('child_process');
exec('git pull && npm install && npm run build && pm2 restart app', (error, stdout, stderr) => {
if (error) {
console.error(`Error: ${error}`);
} else {
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
}
});
Conclusion
Node.js offers a versatile and efficient platform for automating a wide range of tasks. By leveraging its asynchronous programming model, rich ecosystem, and cross-platform compatibility, you can streamline your workflows, improve productivity, and unlock new possibilities in automation. Whether you're a developer, system administrator, or business owner, exploring Node.js for automation can be a valuable investment.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
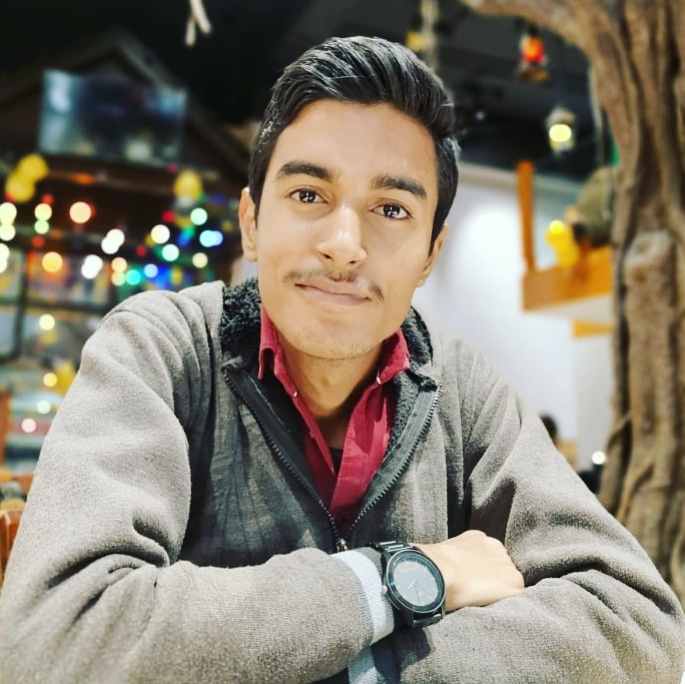
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.