My CS50 Learning Experience ✨
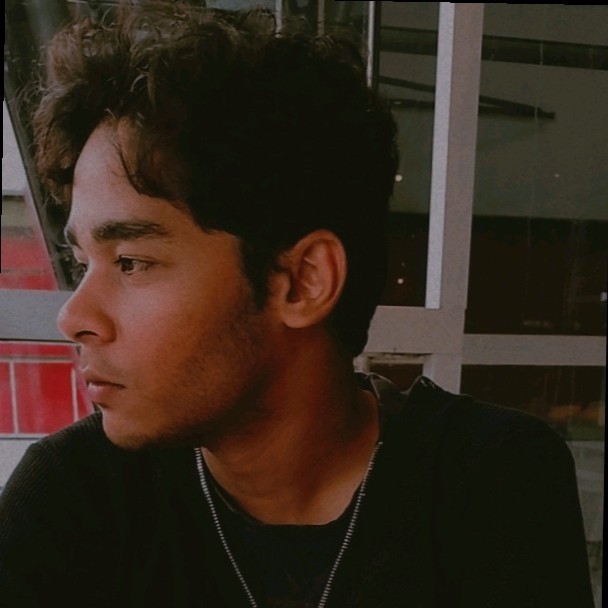
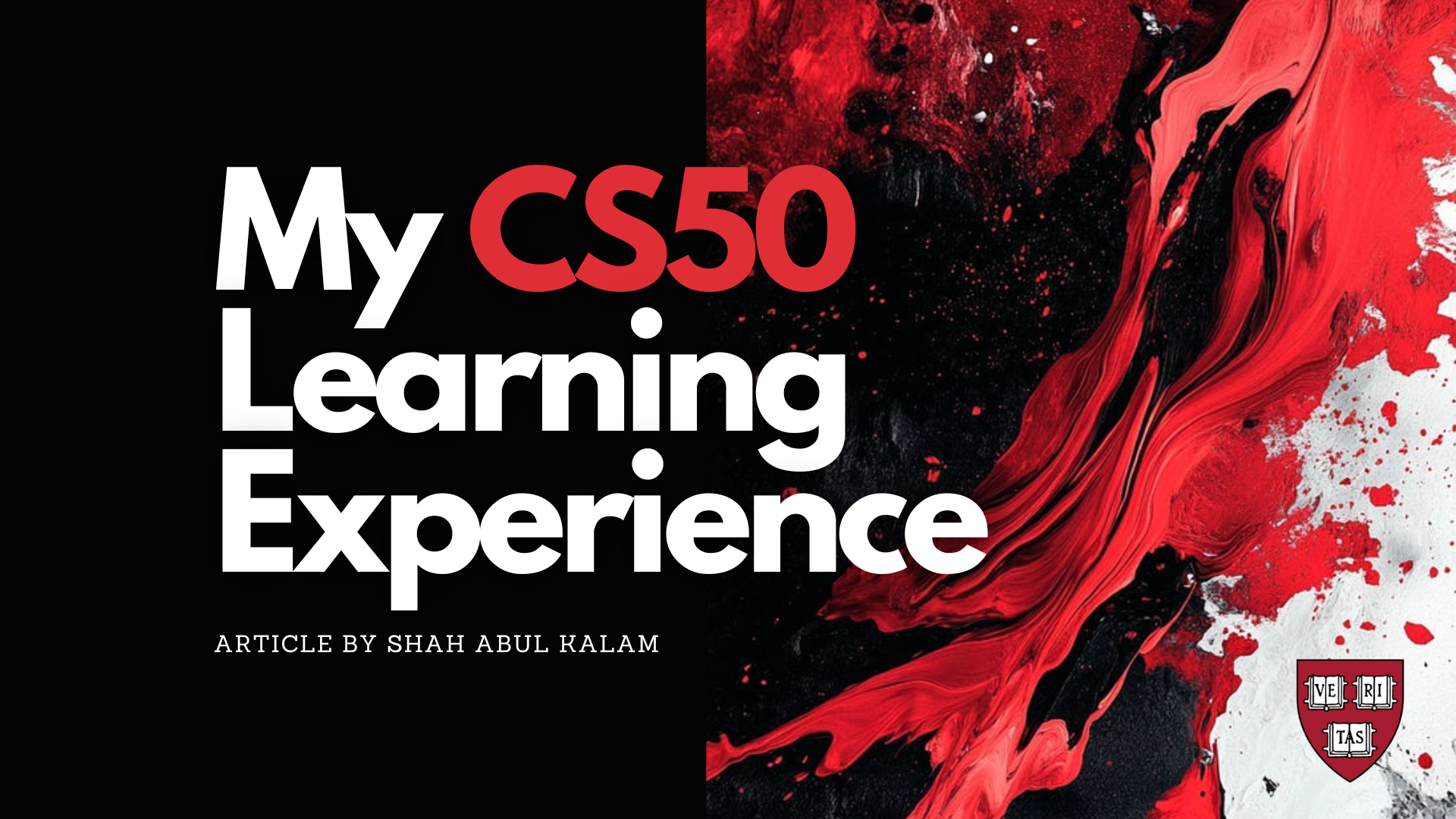
Introduction
I learnt about CS50 through an Instagram reel I saw back in 2022, I was surprised that Harvard University offers free Computer Science courses for the public that explains almost everything you need to know about programming and how the computers work.
I took up the course in 2022, but unfortunately couldn’t complete it because of certain circumstances and lack of time management🥹.
After graduating in 2024, I planned to continue my studies pursuing MTech in Cloud Computing (I find this a fascinating field 😁). My classes were to start by November and I still had few months to spend my time burying myself into learning stuffs that my college failed to do so (I mean, obviously ….my college was shit :p)
So, I started my CS50× 2024 classes and damn was it useful, I am gonna break down the explanation divided by the “Weeks” starting from Week 0 all the way to Week 10.
Week 0 - Scratch😸
This week was foundational yet very informative and the problem set was damn easy.
Professor David J Malan ✨ explained everything about computational thinking, problem solving, how bits and bytes work, ASCII, Unicode and so many interesting stuffs.
Scratch was introduced as a gamified way for programming cool things, the conditionals, loops, user-defined functions and other stuffs were demonstrated.
For the problem set I had to create a scratch file and upload it through google forms. I created a simple fun game where you play as “Astro dog” 🐶 and try to dodge away from the prehistoric - flying pterodactyls 🦖 trying to hunt you down, you can dodge them by using the arrow keys.
Week 1 - C 💻⚙️
This week was my first proper introduction to the C language. Although it's an old programming language, it is very powerful, as it allows you to work at the memory level and manage hardware resources directly.
The jump from Scratch to C was not that hard, the syntax seemed good and understandable. Also learned about Header files and Libraries, in fact CS50 uses their own library cs50.h
as the training wheels for beginners. The get_int
function is used to get the integer input from the user avoiding the scanf
function for now, to teach us the basics.
get_string
is very useful here as in C there is no data type as String, it’s basically char *
(that’s the data type char with pointer)
C was fun here, the problem sets were cool too. The “Mario” is one of favorite homework problem 🍄
Week 2 - Arrays 🧮
David taught us about compiling and debugging (How to use a debugger, setting a breakpoint etc.)
Compiling as 4 major steps:
Preprocessing - the header import files are copied and pasted into your file (such as
#include <cs50.h>
).Compiling - the program is converted into assembly code.
Assembling - the assembly code is converted to machine code (binary).
Linking - Machine code from the included libraries are combined with our code, and the finally an executable file is created.
Also learnt about Arrays & Strings, along with how to define them and manipulate them, how the Array stores data back-to-back in the memory and how every String is an array of characters which always ends with a null character /0
to terminate the string. Command-line Arguments were very useful to learn as they come handy for future problem sets.
A bit of cryptography 🔒 was also taught, I really liked the Caesar Homework problem where we are supposed to encrypt a sentence (plaintext to ciphertext) using a user-defined key (can be any integer)
Week 3 - Algorithms 🔢
This week was fun 😁, I learnt about the time and space complexity thoroughly through few basic searching and sorting algorithms. Also learnt that we are always supposed to take the worse complexity that is Big O.
Apart from learning the concepts about Linear search and Binary Search, practicing to code the Selection Sort and Bubble Sort in C taught me so much more on Problem solving and understanding the art of programming, and to have the cherry on top - Recursion.
Merge Sort is more efficient yet has a higher space complexity than the previous two sorts, It uses recursion for optimized search performance.
Week 4 - Memory 💾
Personally, this was my least favorite week as I found it little boring because of the concept Pointers (cuz it was a lil difficult to understand 🥹)
Although I really liked the hexadecimal pixel explanation part, along with the RGB filtering, the problem set was really creative as we are supposed to convert an image into specific filters like grayscale, reflected, sepia, blur and edges.
Also concepts like comparing strings using strcmp
function, copying of the string address through pointers, Valgrind to check if there are memory-related issues with your programs wherein you utilized malloc. Malloc is used to allocate enough memory for an array.
Week 5 - Data Structures 📚
One of the most useful weeks 🙌🏼 , DSA is a core problem solving skill that most of the programmers are supposed to have.
I knew a little about Stacks, Queues, Linked Lists and Trees before, but got a better and clean explanation of it here along with the new concepts - Hash tables and Tries. Linked Lists in C works with pointers and structs where it uses ->
operator to point to an address and looks inside of a structure. Trees are another data structure that can be used to store data more efficiently such that it can be searched and retrieved.
A hash table 🔑 is a fantastic combination of both arrays and linked lists. When implemented in code, a hash table is an array of pointers to nodes.
Resizing of Arrays in C was fun to learn too because in the memory there’s always a garbage value whenever you try to delete a file or data, it’s not completely deleted, instead it’s stored as a temporary garbage value, which will be later replaced by a newer data.
Week 6 - Python 🐍
Finally moving on to a higher level language, I was so glad that C was over because it was really getting tedious working on a lower level language 😭
Python gives you tons of useful built-in functions and libraries. It saves a lot of time because of it’s famously minimal syntax, but yeah, there is a tradeoff of speed and memory management - Because C allows us to make decisions about memory management, it may run faster than Python – depending on your code. While C only runs your lines of code, Python runs all the code that comes under the hood with it when you call Python’s built-in functions.
We had to revisit the previous problem sets like the filter problem from Week 4, but this time, it was damn easy because of the pre-built modules of the open-source libraries. Example below :
# Blurs an image
from PIL import Image, ImageFilter
# Blur image
before = Image.open("bridge.bmp")
after = before.filter(ImageFilter.BoxBlur(1))
after.save("out.bmp")
Literally 4 lines of code whereas C took more than 15 (⊙ˍ⊙)
As the code is so simplified, I tried working on DSA with it - was a lot helpful and also No Pointers! lesgo🥳
Week 6.5 - Artificial Intelligence 🤖
A quick an special tutorial on how AI has been changing the face of the technology we’ve known, through Image generation, Prompt generation, ChatGPT and other creative things.
Malan explained about Generative AI and how algorithms look for patterns in junk mail, images saved on your phone, and to play games.
Brief introduction to Machine Learning was also given and how the machine learns through reinforcement learning - rewarded for taking good steps and punished for taking the wrong ones. Deep Learning uses neural network whereby problems and solutions are explored, existing training data is given to train the model and based on it, the machine predicts the solutions.
Week 7 - SQL 🗃️
The most fun I had solving the problem set was of this week’s Fiftyville problem 🔥, It uses your querying skills to solve a case where CS50’s duck has been stolen and we are provided with just enough evidence in SQL table format that consists of primary keys, foreign keys and other useful attributes.
I learnt like a heck lot of new stuffs about SQL 💪🏼 and I’m so glad that I swiftly understood this language (I mean obviously it’s fun and easy) I am also thinking of taking up the CS50 SQL course next year to learn more about it, as it would also help me build my foundation on Oracle’s ERP suite (Thinking of combining ERP with my Cloud computing skills in future (*^_^*)).
I also learnt how sqlite3 and python go hand-in-hand through CSV files and how you can manipulate the data through coding it in python and resulting the outputs. CS50 has also created a special library to connect sqlite3 easily instead of taking the longer route to implement from scratch. Below is the example on how to use it.
# The CS50 library I was talking about
from cs50 import SQL
# Open database
db = SQL("sqlite:///favorites.db")
Week 8 - HTML🌐, CSS🎨 & JS💻
One of the easiest week and yet informative, the concepts - TCP/IP protocols, DNS, HTTP, GET and POST request, how to use curl (Client for URL) etc. All these were explained and were clearly visualized how the internet actually works through transmitting data packets and working of addresses.
HTML is a basic structural language to learn if you are just starting out as a web developer. Personally, I was already proficient in it, so I got through the session quickly without much trouble 😉. I also learned regex in detail here, which was a bit confusing.
CSS - Cascading Style Sheets is a markup language that allows us to style our HTML and beautify it, It’s framework bootstrap was introduced although I prefer working with Tailwind CSS (more customizable) and Shadcn.
JavaScript - A strange but useful language for beginners, now used in almost every field. It adds interactivity to the webpage you built with HTML and CSS. Like Python, JavaScript is a high-level language and easier to understand compared to C. Personally, I prefer coding in TypeScript because of its strict typing. 😛
The problem sets were also very easy to solve, as we’re just supposed to create a simple homepage using these 3 technologies.
Week 9 - Flask 🌶️
Up until this point, everything I had been coding was static. To move from static data to dynamic, we need to use frameworks to easily build these dynamic websites. This is where frameworks like Flask, Django, React, and Vue come in.
Flask is a microframework that allows you to host web applications and is based on the Python programming language, while React, Vue, Angular, and others are based on JavaScript.
This wasn’t as fun as the other weeks but still was worth to learn new concepts like the file structure of Flask, usage of Jinja code syntax, Connecting Flask with SQL and finally how to store and use session cookies in Flask.
The problem set “Finance“ was quite challenging as it needed a lot of features and functions to be implemented because it’s a combination of HTML, CSS, JS, Python, SQL, APIs, and so much more.
Week 10 - Cybersecurity 🛡️
Finally, the last week of CS50, got an higher level overview of cybersecurity-related topics like having a strong and unique passwords, OTPs, 2FAs, Password Manager Apps and Hashing.
Hashing is an interesting topic in cybersecurity, the confidential information such as the account credentials and passwords should not be stored in raw text, to solve this hashing algorithms are used (like SHA - 128, SHA - 256, etc.) to store only hashed values of passwords. To have heightened security salting can be used where it becomes unlikely that multiple users may have the same hash value to represent their passwords
And to conclude the course David and the CS50 team prepared a quiz at the end that consisted of all the trivial questions from week 0 till week 10. It was fun, I got almost everything correct! And yeah, no problem set for this week🥳
So that was it, The course ends with the students submitting their final projects through the submit50
command. The project can be anything creative and useful.
I created a banking fintech application called Fintech-Leonidas, that is built using NextJS, TypeScript, Appwrite DB & Auth, Plaid API and Dwolla. Unfortunately, I didn’t use Python or any of it’s framework to build it because of my personal preferences and existing experiences with NextJS.
You can check out the project here! ↗️
Below is my CS50x Certificate I received after submitting the final project 🥹👉🏼👈🏼
If you've read this far about my journey, thank you so much! I wish you all the best for your future! 💖💖 And yes, do take this course if you are just starting in the CS field or need to polish your skills to understand the concepts clearly.
Adios
- Shah 2024🪐
Subscribe to my newsletter
Read articles from Shah Abul Kalam A K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
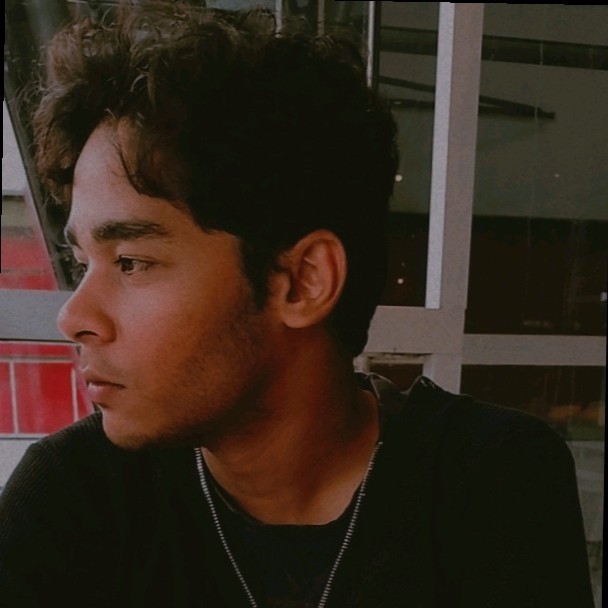
Shah Abul Kalam A K
Shah Abul Kalam A K
Hi, my name is Shah and I'm learning my way through various fascinating technologies.