Software Tips and Tricks
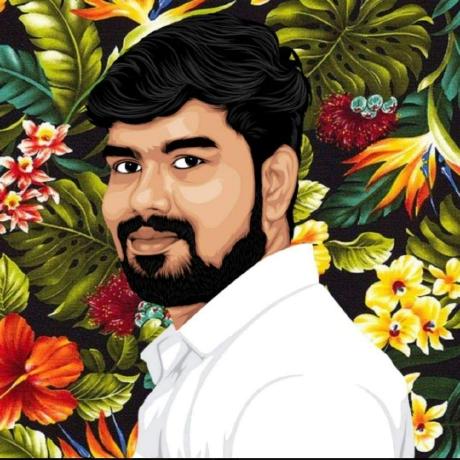
Table of contents
- Senior vs Junior JavaScript Developers : Part - 1
- 1. Variable Declarations: var vs let/const
- 2. String Concatenation vs Template Literals
- 3. Avoiding Repetition: DRY Principle
- 4. Error Handling: Ignored Errors vs Proper Handling
- 5. Code Readability: Long-Winded vs Concise and Clear
- 6. Array Manipulation: Loops vs Built-In Methods
- 7. Conditional Logic: Multiple if Statements vs Ternary Operator
- 8. Object Destructuring: Manual Access vs Destructuring
- 9. Code Optimization: Naive Approach vs Smart Optimization
- Conclusion: Key Takeaways for Junior Developers
Senior vs Junior JavaScript Developers : Part - 1
As developers grow in experience, their approach to coding evolves, often becoming more efficient, elegant, and maintainable. Junior developers might solve problems in a way that works but isn't always optimal, while senior developers typically find shortcuts or best practices that simplify the same tasks. In this post, we’ll highlight some differences between junior and senior JavaScript developers with coding style examples, shortcuts, and tips on improving code quality.
1. Variable Declarations: var
vs let/const
Junior Developer:
var userName = "John Doe";
var age = 25;
Senior Developer:
const userName = "John Doe";
let age = 25;
Explanation: Junior developers may still use var
for declaring variables, which can lead to scope issues. Senior developers prefer let
and const
, which have block scope and prevent unexpected behavior. const
is used for values that won’t change, while let
is for variables that might be reassigned.
2. String Concatenation vs Template Literals
Junior Developer:
const name = "Alice";
const greeting = "Hello, " + name + "!";
console.log(greeting);
Senior Developer:
const name = "Alice";
const greeting = `Hello, ${name}!`;
console.log(greeting);
Explanation: Junior developers might use string concatenation, which can be harder to read and maintain. Senior developers utilize template literals for cleaner, more readable string interpolation.
3. Avoiding Repetition: DRY Principle
Junior Developer:
function getUserInfo(user) {
console.log(user.name);
console.log(user.age);
console.log(user.email);
}
Senior Developer:
function getUserInfo(user) {
for (const key in user) {
console.log(user[key]);
}
}
Explanation: Junior developers often repeat code (violating the "Don't Repeat Yourself" or DRY principle). Senior developers seek to abstract and reuse code to make it more efficient and maintainable.
4. Error Handling: Ignored Errors vs Proper Handling
Junior Developer:
const data = JSON.parse(someInvalidJson); // May throw error, no handling
Senior Developer:
try {
const data = JSON.parse(someInvalidJson);
} catch (error) {
console.error("Failed to parse JSON", error);
}
Explanation: Junior developers may ignore errors or not anticipate edge cases. Senior developers ensure robust error handling, catching exceptions and handling them gracefully.
5. Code Readability: Long-Winded vs Concise and Clear
Junior Developer:
const isValidUser = (user) => {
if (user && user.isActive) {
return true;
} else {
return false;
}
};
Senior Developer:
const isValidUser = (user) => !!user?.isActive;
Explanation: Junior developers may write verbose code to express logic. Senior developers often simplify logic using modern JavaScript features like optional chaining (?.
) and concise return statements.
6. Array Manipulation: Loops vs Built-In Methods
Junior Developer:
const numbers = [1, 2, 3, 4];
const doubled = [];
for (let i = 0; i < numbers.length; i++) {
doubled.push(numbers[i] * 2);
}
Senior Developer:
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(num => num * 2);
Explanation: Junior developers may rely on traditional for
loops for array manipulation. Senior developers leverage built-in array methods like map()
, filter()
, and reduce()
for more declarative and concise code.
7. Conditional Logic: Multiple if
Statements vs Ternary Operator
Junior Developer:
let status;
if (isActive) {
status = "Active";
} else {
status = "Inactive";
}
Senior Developer:
const status = isActive ? "Active" : "Inactive";
Explanation: Junior developers tend to use multiple if
statements, whereas senior developers often utilize the ternary operator for simple conditional assignments.
8. Object Destructuring: Manual Access vs Destructuring
Junior Developer:
const user = { name: "Bob", age: 30, email: "bob@example.com" };
const name = user.name;
const age = user.age;
Senior Developer:
const { name, age } = user;
Explanation: Senior developers take advantage of JavaScript's de-structuring syntax, which allows extracting multiple properties from an object in a concise manner.
9. Code Optimization: Naive Approach vs Smart Optimization
Junior Developer:
function calculateSum(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
Senior Developer:
const calculateSum = arr => arr.reduce((sum, num) => sum + num, 0);
Explanation: Senior developers often find optimized solutions using built-in array methods like reduce()
, making the code shorter and more efficient.
Conclusion: Key Takeaways for Junior Developers
Embrace modern JavaScript features like
let/const
, template literals, de-structuring, and arrow functions.Aim to write concise, readable code by eliminating unnecessary repetition and favoring built-in methods for array manipulation.
Always handle errors properly and ensure your code is resilient to edge cases.
Prioritize code readability and maintainability by following best practices, which will naturally evolve with experience.
By incorporating these tips and adopting a mindset of continuous improvement, junior developers can bridge the gap toward becoming senior developers.
Happy coding!
Subscribe to my newsletter
Read articles from Bharani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
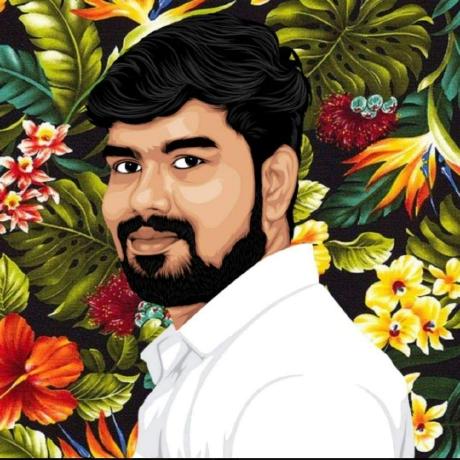