Playwright Selectors and Locators: Everything You Need to Know
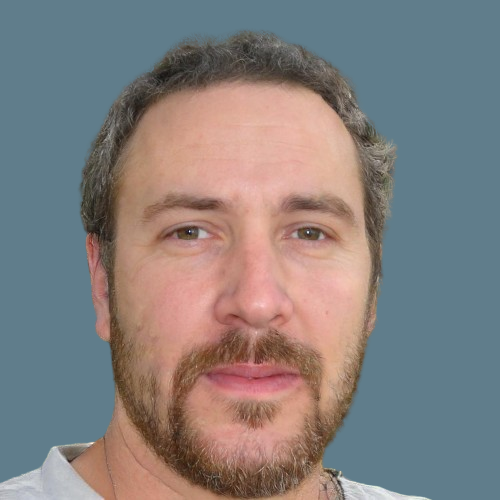
Playwright is a powerful test automation framework, allowing users to control web applications and conduct End to End test automation. In this article, we will try to cover complete functionality and the implementation of playwright selectors and locators.
Selectors act as a crucial component of the playwright framework. It helps automated tests to have interaction with web applications like validating if that element exists on the web page or not, clicking on the available options, providing inputs by typing or reading text. Playwrights come with multiple types of selectors and a good set of methods using which we can perform actions on selected elements.
Few of them are listed below:
CSS Selectors: when users locate Web elements using their attributes, IDs, classes and more, They are widely used while performing test automation.
const element = await page.$('input[type="text"]');
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Find an element using a CSS selector
const element = await page.locator('h1');
// Interact with the element (e.g., click)
await element.click();
await browser.close();
})();
2 . XPath Selectors: While identifying elements which do not have unique attributes or they are nested elements, for these conditions XPath is the most appropriate solution.
const element = await page.$x('//input[@type="text"]');
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Find an element using XPath
const element = await page.locator('//h1');
// Interact with the element (e.g., click)
await element.click();
await browser.close();
})();
3 . Locator API: Locator API is a simplified way of locating web elements using chainable interfaces and performing actions on the selected elements.
const buttonLocator = page.locator('button');
await buttonLocator.click();
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Find an element by its attribute value
const element = await page.locator('[name="search"]');
// Interact with the element (e.g., type text)
await element.type('Playwright');
await browser.close();
})();
4 . Text Selector: When users try to identify elements based on the visible text content of Element on the web page.
const element = await page.locator('text=Click Here');
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Find an element by its text content
const element = await page.locator('text=Welcome');
// Interact with the element (e.g., click)
await element.click();
await browser.close();
})();
5 . Handling Shadow DOM: Playwright has capability to identify and interact with elements which exist in Shadow DOM.
const shadowDomElement = await page.$('shadow-root-element >>> .shadow-element');
6 . Waiting for Elements: Playwrights come with methods using which we can wait for the element to load, be visible and meet any dependent condition before identifying and performing action on it.
await page.waitForSelector('button', { state: 'visible' });
7 . Selecting options in Dropdowns: When a user needs to interact with menu options present in a drawdown, Playwright helps with these methods for performing the actions.
await page.selectOption('select#myDropdown', 'Option 1');
8 . Selectors for Pop Ups and Frames: Playwrights provide options to select elements from pop up windows and iframes. Users can perform actions using these identified web elements.
const popup = await page.waitForPopup('https://example-popup.com');
const popupElement = await popup.$('div.popup-content');
Usage of Playwright Selectors:
Playwright selectors play vital role in performing automation related Task:
We can perform Element Interaction (Selecting, Clicking, Submitting web pages and overing over web elements), Element verification ( Validating whether element is visible, element is present on the web page or not), Data Extraction ( fetching text, files and other data from the UI for further validation) and Waiting for the web elements getting available on the UI.
What is a Playwright Locator?
Playwright locators are a set of methods and APIs that allow you to select and interact with elements available on a web page. They provide a higher-level and more expressive way to locate elements compared to traditional selector types like CSS selectors and XPath.
Types of Playwright Locators:
Playwright comes with multiple types of locator for identifying elements and fields on the web page.
Page.locator(): Users can use this while dealing with multiple elements on the web page, This method creates a locator for finding elements and then we can chain multiple cations on the set of elements.
Locator.locator(): For refining selection further we can chain locator methods with existing locators.
locator.locatorAll(): For locating multiple elements matching a locator we can use this method.
Locator Strategies:
Locator strategies are used for selecting an appropriate locator for performing web automation few of them are listed below:
locator(‘text=Some Text’): locating web elements based on the text visible.
locator(‘aria-label=Label’): locating web elements based on the Aria label.
**locator(‘aria-labelledby=ID’):**locating web elements based on the Aria labelled by ID.
locator(‘css selector’): For targeting elements based on CSS attributes we need to combine CSS selector with the locator.
locator(‘xpath selector’): For locating complex web elements and complex queries by combining Xpath selector with a locator.
Implementation of Locator:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const locator = page.locator('text=Click Here');
await locator.click();
})();
Here the first user is initialising the chrome browser and then accessing a web URL, on this page we are trying to locate the “Click here” button on the UI, using locator by text.
Playwright Locate up-to-date elements
Whenever the locator is used to perform some action; an up-to-date DOM element is located on the page. So in the code below, the highlighted element will be located two times. For the first time it will be located before the hover action occurs, and for the second time it will be located before the click action. This means that the new element corresponding to the locator will be used if the DOM changes in between the calls due to re-render. With this process, you will not be getting stale element exceptions like other automation tools and frameworks.
const locator = page.locator('text=Submit');
await locator.hover();
await locator.click();
End to End implementation of Playwright Selector and locator:
const { chromium } = require('playwright');
const { expect } = require('@playwright/test');
(async () => {
// Launch a browser
const browser = await chromium.launch();
const context = await browser.newContext();
// Create a page
const page = await context.newPage();
await page.goto('https://example.com');
// Use a locator to find an element by CSS selector
const searchInput = page.locator('input[type="text"]');
// Type text into the input field
await searchInput.type('Playwright');
// Use a locator to find an element by text content
const welcomeMessage = page.locator('text=Welcome');
// Assert that the element with the text content exists
expect(await welcomeMessage.isVisible()).toBeTruthy();
// Click on the element
await welcomeMessage.click();
// Use a locator to find an element by XPath
const heading = page.locator('//h1');
// Assert that the element with the XPath selector exists
expect(await heading.isVisible()).toBeTruthy();
// Capture a screenshot
await page.screenshot({ path: 'example.png' });
// Close the browser
await browser.close();
})();
In this example:
1. We launch a Chromium browser (Chrome Browser) and create a new context and page.
2. We use locators (Selector) to find elements on the page using CSS selectors, text content, and XPath.
3. We are doing actions like typing into an input field, clicking on an element, and taking a screenshot.
4. We are using assertions from @playwright/test
(assuming you have it installed) to check if elements are visible.
5. At the end, we close the browser.
Conclusion:
In summary, Playwright’s selector and locator capabilities provide a powerful and flexible way to interact with web elements during automation and testing. They are designed to work with accuracy across different browsers and can adapt to various scenarios, making Playwright a valuable choice for web automation projects. However, it’s very important to select the most appropriate selector strategy based on your specific use case and application requirements for performing test automation with minimum failures.
Source: This article was originally published at https://testgrid.io/blog/playwright-selectors-and-locators/
Subscribe to my newsletter
Read articles from Jace Reed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
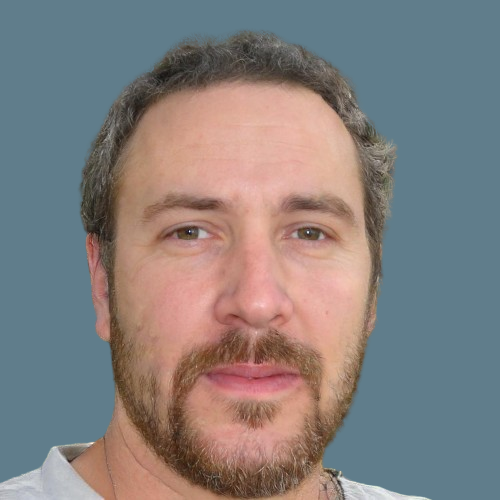
Jace Reed
Jace Reed
Senior Software Tester with 7+ years' experience. Expert in automation, API, and Agile. Boosted test coverage by 30%. Focused on delivering top-tier software.