Learn Sets, Frozensets in Python: Day 6 Guide

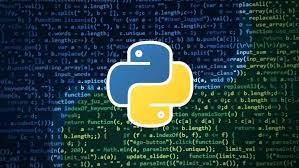
Introduction:
Welcome to Day 6 of my Python journey!
Today, I ventured into the realm of sets, frozensets .
These concepts are essential for efficient data manipulation and storage.
class set :
BASIC :
. set is a predefined class in python.
. to get the mannuel of set class, >> help ( set )
. how to create the object of set class,
obj = set ()
print ( obj , type ( obj ))
obj = set ( { 10, 20, 30 } )
print ( obj , type ( obj ) )
obj = { 10, 20, 30, 40 }
print ( obj , type ( obj ))
. set object is represented by {}
. set does not store the data as per the insertion order.
. set is an unordred data structure.
. set does not support duplicate element.
. set is a collection of similar or dissimilar types of elements.
. it is a iterable object .
. set object is mutable object.
. we can modify a set object in future as per the demand of situation.
. set object supports item assignment and item deletion.
INDEXING
. subscript operator is not supported with set object.
object [ index ]
. indexing mechanism is not supported with set object.
operation with set object :
UNION
INTERSECTION
DIFFERENCE
SYMETRIC_DIFFERENCE
OPERATORS :
arithmatic
- : difference
bitwise
& : intersection
| : union
^ : symmetric_difference
membership
in not in
is is not
deletion
not supported
slicing not supported bcz set does support indexing mechanism
FUNCTIONS :
len ()
sum ()
max ()
min ()
sorted () no sorted because index is not aval for set object
METHOD :
UNION
union ()
update ()
INTERSECTION
intersection ()
intersection_update ()
DIFFERENCE
difference ()
difference_update ()
SYMMETIRC_DIFFERENCE
symmetric_difference ()
symmetric_difference_update ()
INSERTION
add ()
DELETION
pop () : remove arbitrary element
remove () : remove a specific element, but on failuer raise KeyError exception
discard () : remove a specific element, on faileruer return None
clear () : delete all elements
IS METHOD
issuperset ()
issubset ()
etc
bytes Data Type:
bytes data type represents a group of byte numbers just like an array.
x = [10,20,30,40]
b = bytes(x)
Conclusion 1:
The only allowed values for byte data type are 0 to 256. By mistake if we are trying to provide any other values then we will get value error.
Conclusion 2:
Once we creates bytes data type value, we cannot change its values,otherwise we will get TypeError.
it is a immutable object
x=[10,20,30,40]
b=bytes(x)
#b[0]=100 #TypeError: 'bytes' object does not support item assignment
bytearray Data type:
bytearray is exactly same as bytes data type except that its elements can be modified.
it is a mutable object
example :
x=[10,20,30,40]
b = bytearray(x)
print (b) x[1] = 200
print (b)
Frozenset :
Frozenset is an immutable (unchangeable) version of a set in Python.
It provides a way to store unique elements without duplicates, and its immutability makes it useful for various applications.
Key Features:
Immutable: Cannot be modified after creation.
Unordered: Elements stored without any particular order.
Unique elements: No duplicates allowed.
Hashable: Can be used as dictionary keys.
Creating a Frozenset:
From a list
my_list = [1, 2, 2, 3, 4]
my_frozenset = frozenset(my_list)
From a set
my_set = {1, 2, 2, 3, 4}
my_frozenset = frozenset(my_set)
Directly
my_frozenset = frozenset([1, 2, 3, 4])
Frozenset Operations:
Union: my_frozenset | other_frozenset
Intersection: my_frozenset & other_frozenset
Difference: my_frozenset - other_frozenset
Symmetric Difference: my_frozenset ^ other_frozenset
Subset: my_frozenset.issubset(other_frozenset)
Superset: my_frozenset.issuperset(other_frozenset)
Use Cases:
Dictionary keys
Set operations
Data deduplication
Thread-safe data structures
Mathematical operations (e.g., finding prime numbers)
Graph algorithms
Benefits:
Thread-safe
Memory-efficient
Improved code readability
Faster execution (due to immutability)
Common Methods:
add(): Not supported (immutable)
remove(): Not supported (immutable)
union(): Returns a new frozenset with combined elements
intersection(): Returns a new frozenset with common elements
difference(): Returns a new frozenset with unique elements
issubset(): Checks if all elements are present in another frozenset
issuperset(): Checks if all elements of another frozenset are present
Example Code:
fs1 = frozenset([1, 2, 3])
fs2 = frozenset([3, 4, 5])
print(fs1 | fs2) # Union print(fs1 & fs2) # Intersection
my_dict = {fs1: "value"}
print(my_dict[fs1]) # Dictionary key
Best Practices:
Use frozenset when immutability is required.
Avoid modifying frozenset instances.
Use set when mutability is necessary.
By mastering Frozenset, you'll write more efficient, readable, and thread-safe code in Python!
numbers = frozenset({1, 2, 3, 4, 5})
print(numbers)
# Output: frozenset({1, 2, 3, 4, 5})
challenges:
Understanding set, frozenset operations.
Handling coding errors.
Resources:
Official Python Documentation: Sets, Frozensets
W3Schools' Python Tutorial: Sets, Frozensets
Scaler's Python Course: File Input/Output
Goals for Tomorrow:
- Explore String.
Conclusion:
Day 6 was a success!
Sets, frozensets are now under my belt.
What are your favorite Python libraries for data manipulation? Share in the comments below.
Connect with me:
LinkedIn : [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
GitHub: [ https://github.com/p-archana1 ]
Join the conversation:
Share your own learning experiences or ask questions in the comments.
Next Post: Day 7: we'll learn String.
Happy learning!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.