Kubernetes OneShot

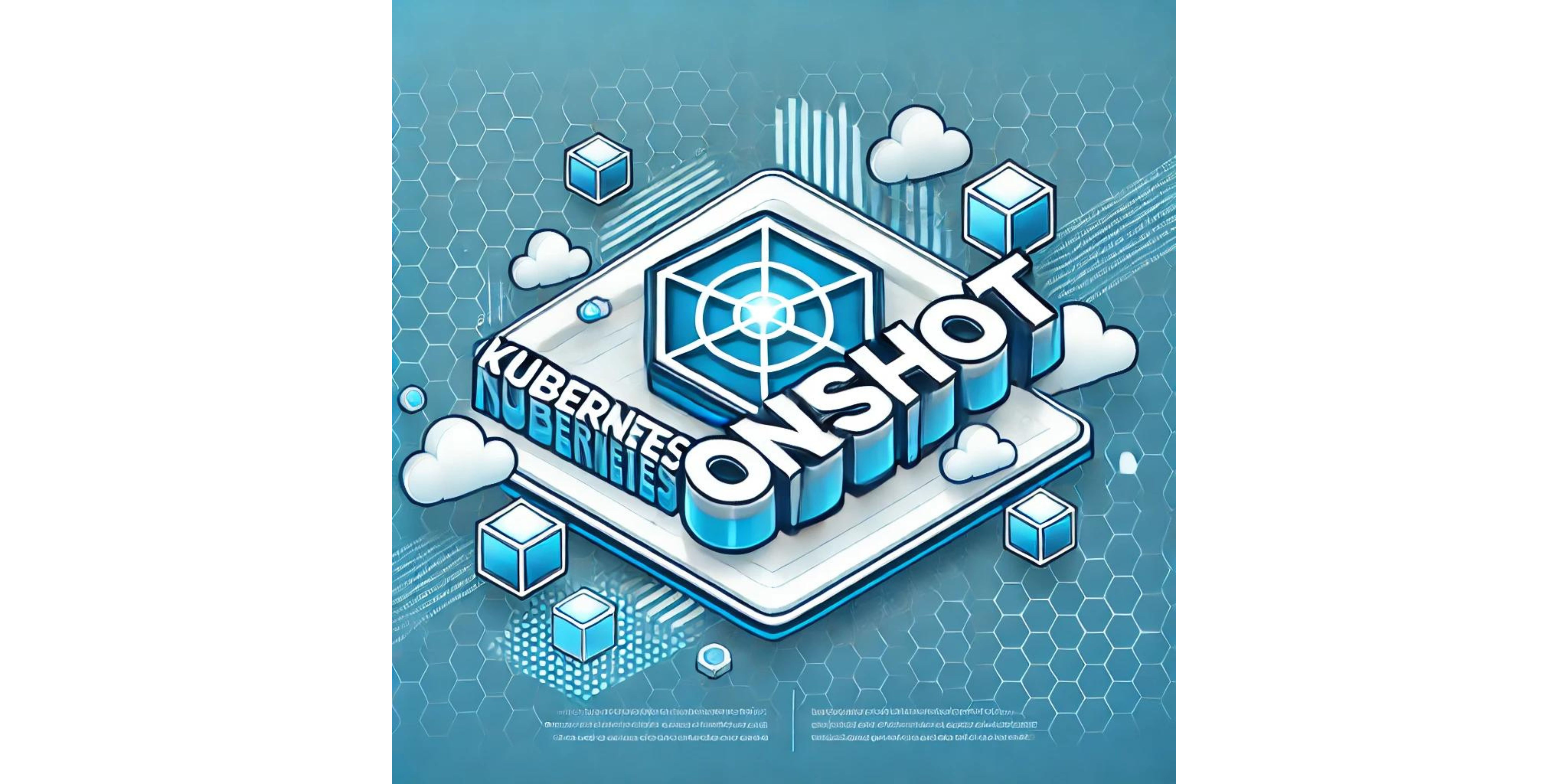
Creating a complete Kubernetes one-shot is a challenge due to the sheer breadth of topics within Kubernetes. However, I will give you an extensive guide that covers almost every major concept and component in Kubernetes, organized by topics, providing an overview, essential commands, and key resources to master Kubernetes.
1. Kubernetes Architecture
Kubernetes follows a master-worker architecture, where the master controls the cluster, and the worker nodes run the containerized applications.
1.1 Key Components:
Control Plane: Manages the cluster.
API Server: The front-end for the Kubernetes control plane.
etcd: A key-value store used for configuration data and service discovery.
Scheduler: Assigns pods to nodes.
Controller Manager: Runs various controllers (e.g., Replication Controller, Node Controller).
Worker Nodes: Where the actual application workloads run.
Kubelet: Communicates with the API server, manages the lifecycle of containers.
Kube-Proxy: Manages networking for services.
Container Runtime: Runs the containers (e.g., Docker, containerd, CRI-O).
2. Basic Kubernetes Concepts
2.1 Installation
Windows
Install Docker Desktop (includes Kubernetes support)
Download Docker Desktop: https://www.docker.com/products/docker-desktop
Enable Kubernetes in Docker Desktop settings.
Install
kubectl
:choco install kubernetes-cli
Ubuntu
Disable Swap:
sudo swapoff -a sudo sed -i '/ swap / s/^\(.*\)$/#\1/g' /etc/fstab
Install Docker:
sudo apt install -y docker.io sudo systemctl enable docker sudo systemctl start docker
Install Kubernetes (
kubeadm
,kubectl
, andkubelet
):
sudo apt update
sudo apt install -y apt-transport-https curl
curl -s https://packages.cloud.google.com/apt/doc/apt-key.gpg | sudo apt-key add -
sudo apt-add-repository "deb http://apt.kubernetes.io/ kubernetes-xenial main"
sudo apt update
sudo apt install -y kubelet kubeadm kubectl
sudo apt-mark hold kubelet kubeadm kubectl
macOS
Install Docker Desktop (includes Kubernetes):
Download Docker Desktop: https://www.docker.com/products/docker-desktop
Enable Kubernetes in Docker Desktop settings.
Install
kubectl
using Homebrew:
brew install kubectl
2.2 Pods
The smallest deployable unit in Kubernetes. A pod can contain one or more containers.
Single-container Pod: The most common type.
Multi-container Pod: Useful when containers share resources like storage.
Commands | Functionality |
kubectl get pods | List all pods |
kubectl describe pod [pod-name] | Describe a pod |
kubectl delete pod [pod-name] | Delete a pod |
kubectl exec -it [pod-name] -- /bin/sh | Access a pod shell |
2.3 Replica Sets
- Ensures a specified number of pod replicas are running at all times.
Commands | Functionality |
kubectl get rs | List Replica Sets |
kubectl scale --replicas=[n] rs/[rs-name] | Scale Replica Set |
2.4 Deployments
- Provides declarative updates for Pods and Replica Sets. Used for rolling updates and rollbacks.
Commands | Functionality |
kubectl create deployment [name] --image=[image] | Create a deployment from an image |
kubectl get deployments | List Deployments |
kubectl rollout undo deployment [name] | Rollback to the previous version |
kubectl delete deployment [name] | Delete Deployment |
3. Services and Networking
3.1 Service Types
Cluster IP: Default, exposes the service only inside the cluster.
Node Port: Exposes the service on a static port on each node.
Load Balancer: Exposes the service externally using a cloud provider’s load balancer.
External Name: Maps a service to an external DNS name.
Commands | Functionality |
kubectl expose pod [pod-name] --type=NodePort --port=8080 | Expose a port |
kubectl get services | List all services |
3.2 Ingress
- Manages external access to services, typically via HTTP/HTTPS, and provides load balancing, SSL termination, and name-based virtual hosting.
Example Ingress:
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: example-ingress
spec:
rules:
- host: example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: example-service
port:
number: 80
Commands | Functionality |
kubectl get ingress | List Ingress resources |
kubectl describe ingress [ingress-name] | Describe Ingress |
3.3 Network Policies
- Define how pods communicate with each other and other network endpoints. They are used to enforce network security rules.
Example:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-specific-traffic
spec:
podSelector:
matchLabels:
role: db
policyTypes:
- Ingress
ingress:
- from:
- podSelector:
matchLabels:
role: frontend
4. Storage
4.1 Volumes
- Allow data to persist even after a pod is deleted.
Common Volume Types:
empty Dir: Temporary storage that exists as long as the pod is running.
host Path: Maps a directory from the host to the pod.
Persistent Volume (PV): Represents physical storage.
Persistent Volume Claim (PVC): Requests storage from a PV.
Commands | Functionality |
kubectl get pv | List Persistent Volumes |
kubectl get pvc | List Persistent Volume Claims |
4.2 Storage Classes
- Dynamically provisions storage when a PVC is created.
Example Storage Class:
apiVersion: storage.k8s.io/v1
kind: StorageClass
metadata:
name: fast
provisioner: kubernetes.io/gce-pd
parameters:
type: pd-ssd
5. Config Maps and Secrets
5.1 Config Maps
- Store configuration data in key-value pairs that can be consumed by pods.
Commands | Functionality |
kubectl create configmap [name] --from-literal=[key=value] | Create a Config Map |
kubectl get configmaps | List Config Maps |
kubectl describe configmap [name] | Describe Config Map |
5.2 Secrets
- Store sensitive information such as passwords, OAuth tokens, and SSH keys.
Commands | Functionality |
kubectl create secret generic [name] --from-literal=[key=value] | Create a new Secret |
kubectl get secrets | List secrets |
kubectl describe secret [name] | Describe a secret |
6. Controllers
6.1 Daemon Sets
- Ensures that a copy of a pod runs on all or some nodes in the cluster.
Commands | Functionality |
kubectl create -f [daemonset.yaml] | Create a Daemon Set |
kubectl get ds | List Daemon Sets |
6.2 Stateful Sets
- Manages stateful applications, where each pod has a unique, stable identity and storage.
Commands | Functionality |
kubectl create -f [statefulset.yaml] | Create a Stateful Set |
kubectl get statefulsets | List Stateful Sets |
6.3 Jobs and Cron Jobs
Job: Ensures a task runs to completion.
Cron Job: Schedules jobs to run at specific times.
Example Cron Job:
apiVersion: batch/v1
kind: CronJob
metadata:
name: example-cronjob
spec:
schedule: "*/1 * * * *"
jobTemplate:
spec:
template:
spec:
containers:
- name: hello
image: busybox
args:
- /bin/sh
- -c
- date; echo Hello from the Kubernetes cluster
restartPolicy: OnFailure
7. Security
7.1 Role-Based Access Control (RBAC)
- Controls access to the Kubernetes API.
Example Role and Role Binding:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "list"]
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: User
name: "jane"
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
7.2 Pod Security Policies (PSP)
- Controls security settings for pods, such as running as root, using host namespaces, or allowing privileged containers.
8. Helm
- Helm is a package manager for Kubernetes. It packages multiple Kubernetes manifests into charts, making them reusable.
Commands | Functionality |
helm repo add [repo-name] [repo-url] | Add a repo using helm |
helm install [release-name] [chart-name] | install a helm chart to your cluster |
helm list | List all releases |
helm upgrade [release-name] [chart-name] | Upgrade a release |
helm delete [release-name] | Delete a release |
9. Monitoring and Logging
9.1 Metrics Server
- Provides resource usage data for pods and nodes. It's required for Horizontal Pod Autoscaling (HPA).
Commands | Functionality |
kubectl top nodes | View resource usage for nodes |
kubectl top pods | View resource usage for pods |
9.2 Prometheus and Grafana
- Prometheus is widely used for monitoring Kubernetes clusters. Grafana is used to visualize metrics.
9.3 Logs
- Kubernetes logs can be accessed using the
kubectl logs
command.
Commands | Functionality |
kubectl logs [pod-name] | View logs for a pod |
kubectl logs -f [pod-name] | Follow logs for a pod |
10. High Availability
10.1 Horizontal Pod Autoscaling (HPA)
- Automatically adjusts the number of pods based on resource usage (e.g., CPU).
Commands:
kubectl autoscale deployment [name] --cpu-percent=50 --min=1 --max=10
10.2 Cluster Auto scaler
- Automatically scales worker nodes based on pending pods.
This one-shot guide covers the most important components of Kubernetes, from core architecture and concepts to advanced topics like autoscaling, storage, networking, and monitoring. Each section can be explored in much more depth, but this guide should give you a solid foundation. Let me know if you need further elaboration on any topic!
Subscribe to my newsletter
Read articles from Tushar Pant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tushar Pant
Tushar Pant
Cloud and DevOps Engineer with hands-on expertise in AWS, CI/CD pipelines, Docker, Kubernetes, and Monitoring tools. Adept at building and automating scalable, fault-tolerant cloud infrastructures, and consistently improving system performance, security, and reliability in dynamic environments.