Introduction Web Fuzzing
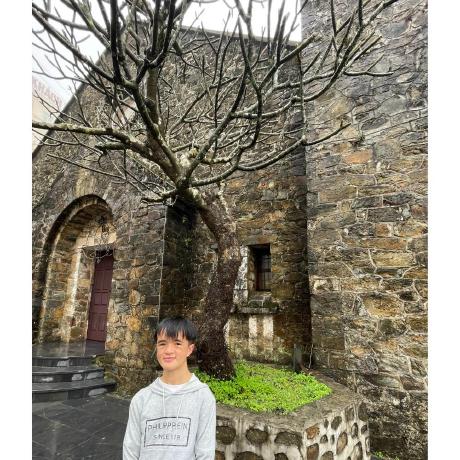
Web fuzzing is a critical technique in web application security to identity vulnerabilities by testing various inputs. It involves automated testing of web applications by providing unexpected of random data to detect potential flaws that attackers could exploit.
Why Fuzz Web Application?
Web applications have become the backbone of modern businesses and communication, handling vast amounts of sensitive data and enabling critical online interactions. However, their complexity and interconnectedness also make them prime targets for cyberattacks. Manual testing, while essential, can only go so far in identifying vulnerabilities. Here’s where web fuzzing shines:
Uncovering Hidden Vulnerabilities
: Fuzzing can uncover vulnerabilities that traditional security testing methods might miss. By bombarding a web application with unexpected and invalid inputs, fuzzing can trigger unexpected behaviors that reveal hidden flaws in the code.Automating Security Testing
: Fuzzing automates generating and sending test inputs, saving valuable time and resources. This allows security teams to focus on analyzing results and addressing the vulnerabilities found.Simulating Real-World Attacks
: Fuzzers can mimic attacker’ techniques, helping you identify weaknesses before malicious actors exploit them. This proactive approach can significantly reduce the risk of a successful attack.Strengthening Input Validation
: Fuzzing helps identify weaknesses in input validation mechanisms, which are crucial for preventing common vulnerabilities likeSQL injection
andcross-site scripting (XSS)
.Improving Code Quality
: Fuzzing improves overall code quality by uncovering bugs and errors. Developers can use the feedback from fuzzing to write more robust and secure code.Continuous Security
: Fuzzing can be integrated into thesoftware development lifecycle (SDLC)
as part ofcontinuous integration and continuous deployment (CI/CD)
pipelines, ensuring that security testing is performed regularly and vulnerabilities are caught early in the development process.
In a nutshell, web fuzzing is an indispensable tool in the arsenal of any security professional. By proactively identifying and addressing vulnerabilities through fuzzing, you can significantly enhance the security of your web applications and protect them from potential threats.
Essential Concepts
Before we dive into the practical aspects of web fuzzing, it's important to understand some key concepts:
Concept | Description |
Wordlist | A dictionary or list of words, phrases, file names, directory names, or parameter values used as input during fuzzing. |
Payload | The actual data sent to the web application during fuzzing. Can be a simple string, numerical value, or complex data structure. |
Response Analysis | Examining the web application's responses (e.g., response codes, error messages) to the fuzzer's payloads to identify anomalies that might indicate vulnerabilities. |
Fuzzer | A software tool that automates generating and sending payloads to a web application and analyzing the responses. |
False Positive | A result that is incorrectly identified as a vulnerability by the fuzzer. |
False Negative | A vulnerability that exists in the web application but is not detected by the fuzzer. |
Fuzzing Scope | The specific parts of the web application that you are targeting with your fuzzing efforts. |
Tooling
Installing Go, Python and PIPX
You will require Go and Python installed for these tools. Install them as follows if you don't have them installed already.
pipx
is a command-line tool designed to simplify the installation and management of Python applications. It streamlines the process by creating isolated virtual environments for each application, ensuring that dependencies don't conflict. This means you can install and run multiple Python applications without worrying about compatibility issues. pipx
also makes it easy to upgrade or uninstall applications, keeping your system organized and clutter-free.
If you are using a Debian-based system (like Ubuntu), you can install Go, Python, and PIPX using the APT package manager.
Open a terminal and update your package lists to ensure you have the latest information on the newest versions of packages and their dependencies.
khoafrancisco@htb[/htb]$ sudo apt update
Use the following command to install Go:
khoafrancisco@htb[/htb]$ sudo apt install -y golang
Use the following command to install Python:
khoafrancisco@htb[/htb]$ sudo apt install -y python3 python3-pip
Use the following command to install and configure pipx:
khoafrancisco@htb[/htb]$ sudo apt install pipx khoafrancisco@htb[/htb]$ pipx ensurepath khoafrancisco@htb[/htb]$ sudo pipx ensurepath --global
To ensure that Go and Python are installed correctly, you can check their versions:
khoafrancisco@htb[/htb]$ go version khoafrancisco@htb[/htb]$ python3 --version
If the installations were successful, you should see the version information for both Go and Python.
FFUF
FFUF
(Fuzz Faster U Fool
) is a fast web fuzzer written in Go. It excels at quickly enumerating directories, files, and parameters within web applications. Its flexibility, speed, and ease of use make it a favorite among security professionals and enthusiasts.
You can install FFUF
using the following command:
Tooling
khoafrancisco@htb[/htb]$ go install github.com/ffuf/ffuf/v2@latest
Use Cases
Use Case | Description |
Directory and File Enumeration | Quickly identify hidden directories and files on a web server. |
Parameter Discovery | Find and test parameters within web applications. |
Brute-Force Attack | Perform brute-force attacks to discover login credentials or other sensitive information. |
Gobuster
Gobuster
is another popular web directory and file fuzzer. It's known for its speed and simplicity, making it a great choice for beginners and experienced users alike.
You can install GoBuster
using the following command:
Tooling
khoafrancisco@htb[/htb]$ go install github.com/OJ/gobuster/v3@latest
Use Cases
Use Case | Description |
Content Discovery | Quickly scan and find hidden web content such as directories, files, and virtual hosts. |
DNS Subdomain Enumeration | Identify subdomains of a target domain. |
WordPress Content Detection | Use specific wordlists to find WordPress-related content. |
FeroxBuster
FeroxBuster
is a fast, recursive content discovery tool written in Rust. It's designed for brute-force discovery of unlinked content in web applications, making it particularly useful for identifying hidden directories and files. It's more of a "forced browsing" tool than a fuzzer like ffuf
.
To install FeroxBuster
, you can use the following command:
Tooling
khoafrancisco@htb[/htb]$ curl -sL https://raw.githubusercontent.com/epi052/feroxbuster/main/install-nix.sh | sudo bash -s $HOME/.local/bin
Use Cases
Use Case | Description |
Recursive Scanning | Perform recursive scans to discover nested directories and files. |
Unlinked Content Discovery | Identify content that is not linked within the web application. |
High-Performance Scans | Benefit from Rust's performance to conduct high-speed content discovery. |
wfuzz/wenum
wenum
is a actively maintained fork of wfuzz
, a highly versatile and powerful command-line fuzzing tool known for its flexibility and customization options. It's particularly well-suited for parameter fuzzing, allowing you to test a wide range of input values against web applications and uncover potential vulnerabilities in how they process those parameters.
If you are using a penetration testing Linux distribution like PwnBox or Kali, wfuzz
may already be pre-installed, allowing you to use it right away if desired. However, there are currently complications when installing wfuzz
, so you can substitute it with wenum
instead. The commands are interchangeable, and they follow the same syntax, so you can simply replace wenum
commands with wfuzz
if necessary.
The following commands will use pipx
, a tool for installing and managing Python applications in isolated environments, to install wenum
. This ensures a clean and consistent environment for wenum
, preventing any possible package conflicts:
Tooling
khoafrancisco@htb[/htb]$ pipx install git+https://github.com/WebFuzzForge/wenum
khoafrancisco@htb[/htb]$ pipx runpip wenum install setuptools
Use Cases
Use Case | Description |
Directory and File Enumeration | Quickly identify hidden directories and files on a web server. |
Parameter Discovery | Find and test parameters within web applications. |
Brute-Force Attack | Perform brute-force attacks to discover login credentials or other sensitive information. |
Subscribe to my newsletter
Read articles from Khoa Nguyen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
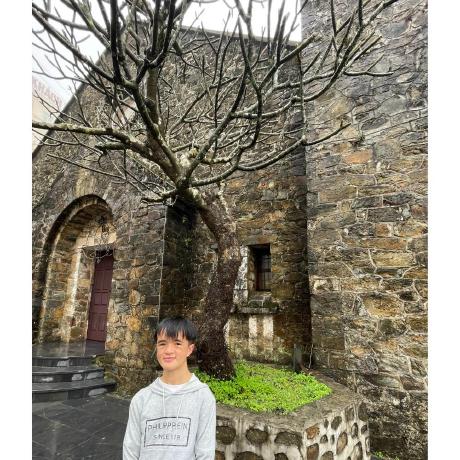
Khoa Nguyen
Khoa Nguyen
Mình là người mới bắt đầu tìm hiểu công nghệ đặc biệt về ngành an toàn thông tin. Mình có viết lại các bài blog này để ghi nhớ thêm cũng như nắm bắt các kiến thức. Rất vui vì được chia sẻ với mọi người!