Docker Project on LocalHost for DevOps Engineers
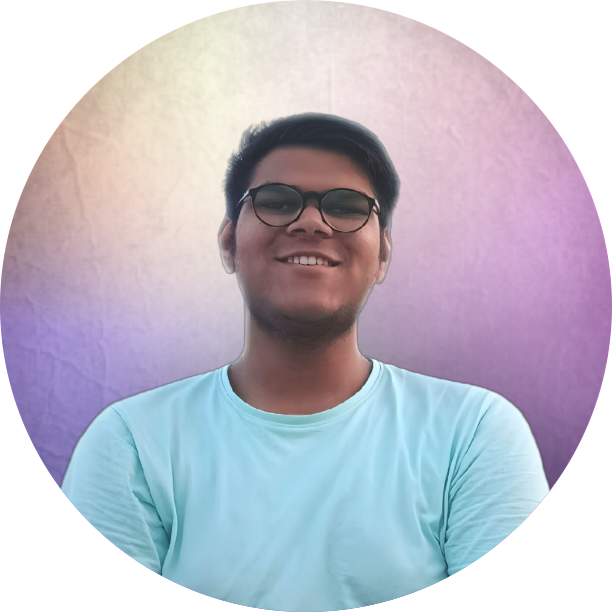
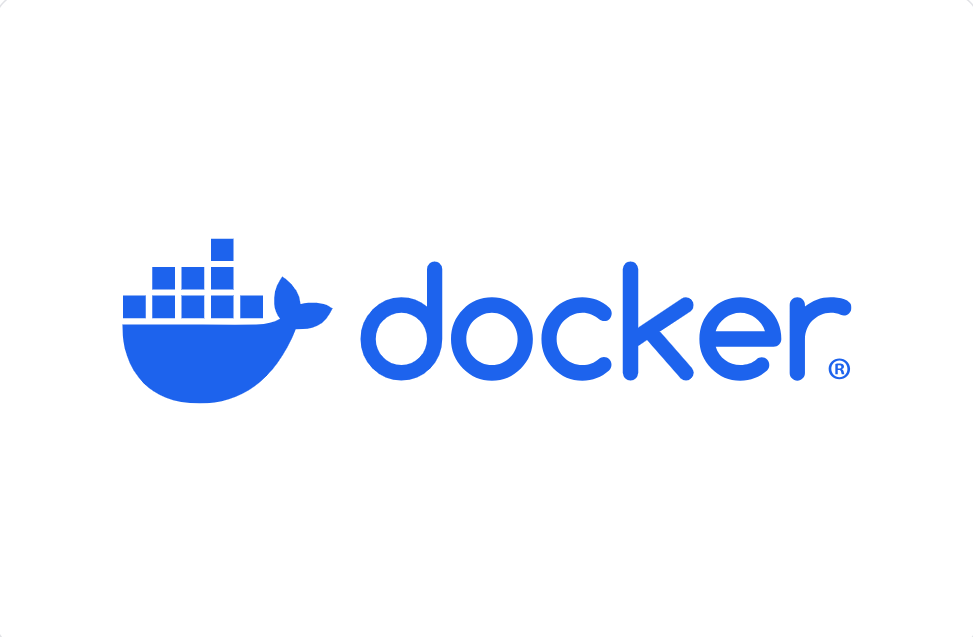
In this blog, we will show you how to create a basic Docker project using localhost.
π What is a Docker File?
A Docker file is a simple text file that contains a series of instructions (steps) on how to build a Docker image. Think of it as a recipe for creating your application environment. It specifies everything from the base image to use, to what files to include, and how to configure your application. When you run the docker build
command, Docker reads the Dockerfile and executes the instructions step-by-step to create a Docker image.
π οΈ Why Use Docker files?
Consistency: Ensures your environment is the same across all stages of development, from testing to production.
Portability: Makes it easy to share your application setup with others or deploy it on different machines.
Automation: Automates the image creation process, reducing human error and saving time.
π Structure of a Docker file
A Docker file consists of a sequence of instructions. Each instruction creates a layer in the Docker image. Hereβs a breakdown of some common instructions:
FROM
: Specifies the base image to start with. Every Docker file starts with this instruction.FROM ubuntu:20.04
This line tells Docker to use the official Ubuntu 20.04 image as the base for your image.
LABEL
: Adds metadata to your image, such as the authorβs name or a description.LABEL maintainer="yourname@example.com"
RUN
: Executes commands inside the container during the image build process. Commonly used to install software packages.RUN apt-get update && apt-get install -y python3
COPY
andADD
: Copies files from your host machine to the Docker image.COPY
is straightforward, whileADD
has additional features like extracting archives.COPY . /app
WORKDIR
: Sets the working directory inside the container. All subsequent instructions will be executed in this directory.WORKDIR /app
EXPOSE
: Informs Docker that the container will listen on the specified network ports at runtime.EXPOSE 8080
CMD
: Specifies the command to run when the container starts. UnlikeRUN
, which is executed during the build,CMD
is executed when the container runs.CMD ["python3", "app.py"]
ENTRYPOINT
: Similar toCMD
, but withENTRYPOINT
, the command and parameters are not overridden if Docker is run with command-line arguments. It is typically used to define a default application to run.ENTRYPOINT ["python3", "app.py"]
ENV
: Sets environment variables in the container.ENV APP_ENV=production
Example of a Sample Docker File
# Step 1: Use an official Python runtime as a base image
FROM python:3.8-slim-buster
# Step 2: Set the working directory in the container
WORKDIR /app
# Step 3: Copy the current directory contents into the container at /app
COPY . /app
# Step 4: Run pip to install dependencies
# (if you had a requirements.txt, you'd use that here)
RUN pip install --no-cache-dir -r requirements.txt
# Step 5: Make port 80 available to the world outside this container
EXPOSE 80
# Step 6: Define environment variable
ENV NAME World
# Step 7: Run app.py when the container launches
CMD ["python", "app.py"]
ποΈ Task: To create a Docker file for a simple web application and successfully run it
Step 1: Create Your Application First, create a simple Python script. For example, app.py
:
print("Hello, DevOps Enthusiast!")
Step 2: Create a Docker file In the same directory as your Python script, create a file named Dockerfile
(no file extension).
Step 3: Add Instructions to Your Dockerfile
# Step 1: Use an official Python runtime as a base image
FROM python:3.8-slim-buster
# Step 2: Set the working directory in the container
WORKDIR /app
# Step 3: Copy the current directory contents into the container at /app
COPY . /app
# Step 4: Run pip to install dependencies
# (if you had a requirements.txt, you'd use that here)
RUN pip install --no-cache-dir -r requirements.txt
# Step 5: Make port 80 available to the world outside this container
EXPOSE 80
# Step 6: Define environment variable
ENV NAME World
# Step 7: Run app.py when the container launches
CMD ["python", "app.py"]
Step 4: Build the Docker Image Open your terminal, navigate to the directory containing your Docker file, and run the following command:
docker build -t hello-enthusiasts .
This command tells Docker to build an image with the tag hello-enthusiasts
using the instructions in your Docker file.
Step 5: Run Your Docker Container After building the image, run it with:
docker run -p 4000:80 hello-enthusiasts
This will start a container from the hello-enthusiasts
image and map port 80 in the container to port 4000 on your host machine. Navigate to http://localhost:4000
in your browser, and you should see the output from app.py
.
π Tips for Writing Docker files
Keep It Simple: Avoid adding unnecessary layers to keep your image lightweight.
Use Multi-Stage Builds: For complex applications, consider using multi-stage builds to reduce the final image size.
Leverage Official Images: Always start with an official base image whenever possible to ensure security and stability.
Optimize
RUN
Instructions: Combine multipleRUN
commands into one to reduce the number of layers and minimize image size.
π Conclusion
Docker files are essential for defining, automating, and sharing application environments. In this guide, we covered Docker installation and key commands for managing containers and images. As I continue my AWS and DevOps journey, Iβm excited to share more insights. Feel free to connect with me on LinkedIn at Deepesh Gupta for feedback or collaboration!
Subscribe to my newsletter
Read articles from Deepesh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
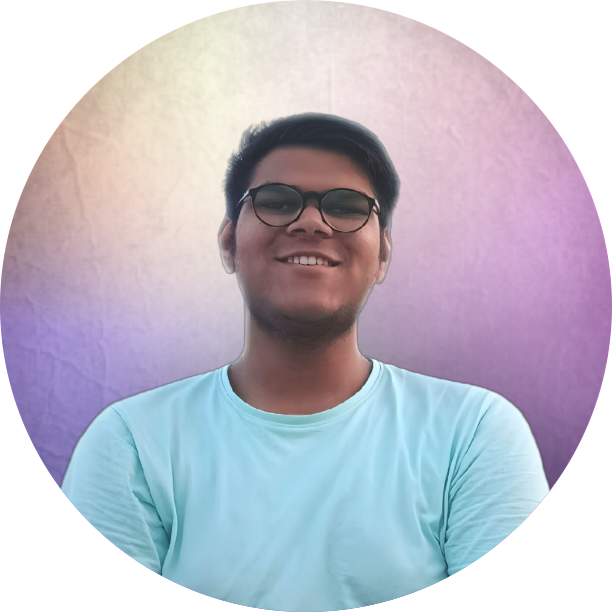
Deepesh Gupta
Deepesh Gupta
DevOps & Cloud Enthusiast | Open Source Contributor | Driving Technological Excellence in DevOps and Cloud Solutions