HTML and CSS bringing it all together!
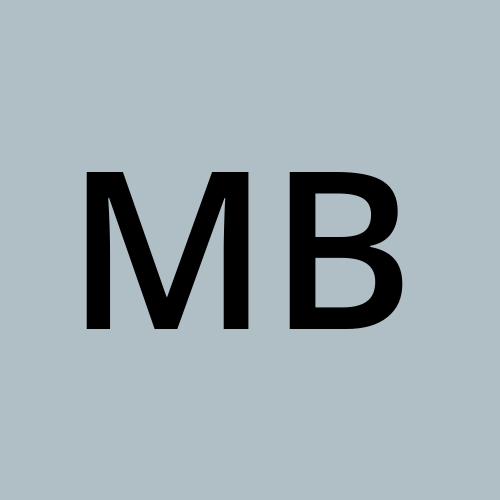
Table of contents
- Intro
- How to Style HTML with CSS
- MORE CSS!
- 1. CSS Box Model
- Visualizing the Box Model
- Example with CSS
- Why Is the Box Model Important?
- 2. Display Property
- Main Display Property Values:
- Why is the Display Property Important?
- Example with CSS
- 3. Positioning
- Main Positioning Values:
- Why is Positioning Important?
- Example with CSS
- Breakdown of the Example:
- 4. Flexbox (Flexible Box Layout)
- How Flexbox Works
- Key Flexbox Properties
- Why is Flexbox Important?
- Example with Flexbox
- Breakdown of the Example:
- 5. CSS Grid Layout
- How Grid Layout Works
- Key Grid Properties
- Why is Grid Layout Important?
- Example with CSS Grid
- Breakdown of the Example:
- 6. Media Queries (Responsive Design)
- How Media Queries Work
- Basic Structure of a Media Query
- Common Use Cases
- Example of Media Queries
- Breakdown of the Example:
- Why are Media Queries Important?
- 7. Z-index
- How Z-Index Works
- Key Points About Z-Index
- Example of Z-Index
- Breakdown of the Example:
- Why is Z-Index Important?
- 8. CSS Transitions
- How CSS Transitions Work
- Basic Structure of a CSS Transition
- Example of CSS Transitions
- Breakdown of the Example:
- Why are CSS Transitions Important?
- 9. Pseudo-Classes and Pseudo-Elements
- Pseudo-Classes
- Pseudo-Elements
- Example of Pseudo-Classes and Pseudo-Elements
- Breakdown of the Example:
- Why are Pseudo-Classes and Pseudo-Elements Important?
- 10. CSS Variables (Custom Properties)
- How CSS Variables Work
- Example of CSS Variables
- Breakdown of the Example:
- Why are CSS Variables Important?
- In Conclusion
- Thoughts and Feelings
- Story Time
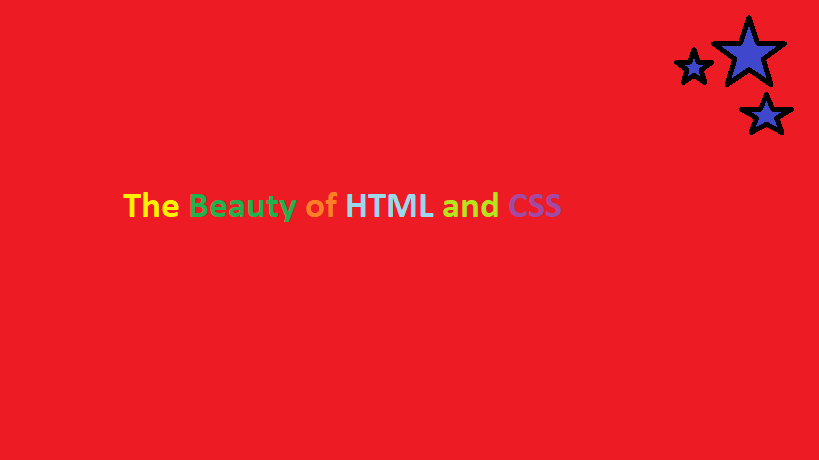
Intro
This is my CSS Part 2. In the previous article, I covered the basics of CSS, including what CSS is, the syntax, and some common properties. Now that we have an understanding of HTML and CSS, I will show you how to use them together. I'll explain how to style HTML with CSS, and and introduce more advanced CSS concepts. So, without further delay, let's start designing today.
How to Style HTML with CSS
Three Ways to Insert CSS
There are three main ways to apply CSS to HTML: External CSS, Internal CSS, and Inline CSS. External CSS involves linking a separate .css
file to your HTML, which is ideal for larger projects as it keeps the structure and style separate, making the code easier to maintain and reuse across multiple pages. Internal CSS is placed within the <style>
tag in the <head>
section of an HTML file, allowing you to define styles for a single page, which is useful for small projects or when you need unique styles for just one page. Inline CSS applies styles directly to individual elements using the style
attribute within the HTML tag, offering quick and specific style adjustments but leading to cluttered code and poor scalability. External CSS is generally preferred for maintainability, while internal and inline CSS are better for small, quick, or one-off styling needs. Here are examples of each CSS method—External, Internal, and Inline CSS—demonstrating how they apply styles in different ways.
External CSS
Internal CSS
Inline CSS
External CSS
With an external style sheet, you can change the look of an entire website by modifying just one file. This is a great way to optimize your code by keeping it as minimal as possible. It also helps with debugging by isolating code and styles to fix problems more easily, and it makes updating big webpage with new styles and fonts simpler. Example of how to code it using what was in the previous lessons. <link rel="stylesheet" href="mystyle.css">
So, as we can see, the <link>
tag has the rel
and href
attributes. The rel
attribute specifies the relationship between the current document and the linked document. The href
attribute specifies the URL that the hyperlink points to. An external style sheet can be written in any text editor (like VS Code) and must be saved with a .css extension. For example, you would save your file as (BBQWEBPAGE.CSS). The external .css file should not contain any HTML tags.
To sum it up, external CSS refers to a method of applying styles to your HTML document by linking to a separate .css
file. This approach keeps the structure (HTML) and presentation (CSS) separate, making the code cleaner and easier to maintain.
How to Connect HTML and CSS Files
Place HTML and CSS Files Next to Each Other: When your HTML and CSS files are in the same directory (folder), you can link the CSS file to the HTML file like this:
HTML (index.html):
htmlCopy code<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>External CSS Example</title> <!-- Link to the external CSS file --> <link rel="stylesheet" href="style.css"> </head> <body> <h1>Hello, World!</h1> <p>This is an example of linking an external CSS file.</p> </body> </html>
CSS (style.css):
cssCopy codeh1 { color: blue; font-family: Arial, sans-serif; } p { color: gray; font-size: 18px; }
Place HTML and CSS Files in Different Folders: If your CSS file is in a different folder, say in a folder called
css
, you would need to adjust the file path in thehref
attribute:HTML (index.html):
htmlCopy code<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>External CSS Example</title> <!-- Link to the external CSS file located in the css folder --> <link rel="stylesheet" href="css/style.css"> </head> <body> <h1>Hello, World!</h1> <p>This is an example of linking an external CSS file from a folder.</p> </body> </html>
In this case, you would have a folder structure like(Visual Studio CODE):
bashCopy code/project
├── index.html
└── /css
└── style.css
This separation of concerns (HTML for structure, CSS for styling) is a recommended practice in web development.
Internal CSS
Internal CSS involves embedding CSS styles directly within the <head>
section of an HTML file. This approach allows you to keep the CSS and HTML in a single file, which can be useful for small projects or when specific styles need to be applied to just one page.
How to Use Internal CSS
Instead of linking to an external CSS file, you include your CSS within a <style>
tag inside the <head>
section of your HTML document.
Here’s an example:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Internal CSS Example</title>
<!-- Internal CSS -->
<style>
h1 {
color: blue;
font-family: Arial, sans-serif;
}
p {
color: gray;
font-size: 18px;
}
</style>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is an example of using internal CSS.</p>
</body>
</html>
Key Points about Internal CSS:
Advantages:
Great for small projects or single-page applications.
Easy to modify CSS directly within the HTML file.
Disadvantages:
Not as scalable for larger projects.
Repeated styles across multiple pages can be hard to maintain.
For larger projects, it's better to use external CSS, as it promotes separation of concerns and makes maintenance easier. However, internal CSS can be handy for quick prototypes or when CSS needs to be applied to a single page.
Inline CSS
Inline CSS refers to applying styles directly to individual HTML elements using the style
attribute. This method allows you to style a specific element without affecting other elements on the page.
How to Use Inline CSS
Unlike external and internal CSS, with inline CSS, the styles are written directly within the opening tag of an HTML element using the style
attribute.
Here’s an example:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Inline CSS Example</title>
</head>
<body>
<h1 style="color: blue; font-family: Arial, sans-serif;">Hello, World!</h1>
<p style="color: gray; font-size: 18px;">This is an example of using inline CSS.</p>
</body>
</html>
Key Points about Inline CSS:
Advantages:
Useful for applying quick, one-time styles to individual elements.
Does not require any additional files or
<style>
sections.
Disadvantages:
Can make the HTML code messy and harder to read/maintain.
Repeated styles must be duplicated across multiple elements, leading to redundancy.
Not recommended for large projects where styles are reused often.
Inline CSS is typically used for small changes or when you need to apply specific styles directly to an element, but it is not recommended for larger projects as it goes against the principle of separation of concerns between HTML and CSS.
MORE CSS!
FreeCodeCamp covered a lot in their Responsive Web Design courses. Now, I will go over 10 more advanced CSS concepts that FCC taught me, such as the CSS box model, display property, positioning, flexbox, and more.
1. CSS Box Model
The CSS Box Model is a core concept that defines how elements are structured on a page. It consists of content, padding, border, and margin. Understanding this helps you control the size and spacing of elements. Imagine every element on a web page (like a paragraph or a button) is a box. The CSS Box Model is like the set of rules that controls how these boxes are drawn and how they interact with each other. Think of it like a picture frame: you have the picture (content), space around the picture (padding), the frame itself (border), and the space between frames (margin).
Example:
cssCopy code.box { width: 200px; padding: 20px; border: 5px solid black; margin: 10px; }
This creates a box that has a width of 200 pixels for the content, with 20 pixels of padding, a 5-pixel border, and 10 pixels of margin separating it from other elements. Here’s how the Box Model breaks down:
Content: This is the main part of the box, where the actual stuff (like text, images, etc.) goes. For example, if you have a paragraph, the text inside it is the content.
Padding: The padding is the space inside the box, between the content and the border. It pushes the content away from the edges of the box. For example, if you want your text to not touch the border of its box, you add padding. The more padding you add, the more space there is between the content and the border.
Border: This is like the picture frame around the content and padding. The border is the line that wraps around the box. You can change its thickness, style (solid, dashed, etc.), and color. You can also remove it if you don’t want the frame to show.
Margin: The margin is the space outside of the border. It separates the box from other elements on the page. Think of it like the space between two framed pictures on a wall. The bigger the margin, the more space you create between the boxes.
Visualizing the Box Model
Here’s how the CSS Box Model would look for an element:
luaCopy code+-------------------------------+ <- Margin
| +-------------------------+ | <- Border
| | +-----------------+ | | <- Padding
| | | Content | | |
| | +-----------------+ | |
| +-------------------------+ |
+-------------------------------+
Example with CSS
Let’s say you have a box (a <div>
) and you want to apply the box model to it:
cssCopy codediv {
width: 200px; /* Content width */
padding: 20px; /* Space inside the box */
border: 5px solid black; /* Border around the box */
margin: 10px; /* Space outside the box */
}
Here’s what happens:
The content of the box has a width of 200 pixels.
The padding adds 20 pixels of space inside the box between the content and the border.
The border is 5 pixels thick and solid black.
The margin adds 10 pixels of space around the outside of the box, separating it from other boxes on the page.
Why Is the Box Model Important?
Spacing: It controls how much space an element takes up and how it sits next to other elements.
Layout: It helps you organize and design the layout of a webpage.
Readability: It ensures that the content isn’t squished against other elements, making it easier to read.
Understanding the Box Model helps you design web pages that are clean, organized, and visually appealing, making it one of the most important concepts in web design.
2. Display Property
The display
property defines how elements are displayed on the page. Imagine you have different blocks of content, like images, paragraphs, or buttons, and you need to decide how they should be arranged on the page. The CSS Display Property is like the rulebook that tells each element how to behave on the page—whether it should take up the full width, sit next to other elements, or be hidden completely. Common values include:
Main Display Property Values:
Block: Think of this like a big block or a paragraph. When an element has
display: block
, it takes up the entire width of the page or its container, and starts on a new line. Even if it doesn’t need all the space, it will grab the full width.- Example:
<div>
,<p>
, and<h1>
are block elements by default.
- Example:
What it looks like:
cssCopy codediv {
display: block;
background-color: lightblue;
}
In this case, a <div>
with display: block
will stretch across the entire width, and the next element will appear on a new line below it.
Inline: When an element has
display: inline
, it only takes up as much space as the content inside it, and it can sit next to other elements on the same line. This is like when you’re typing in a text message, and all the words stay on the same line until the space runs out.- Example:
<span>
,<a>
, and<strong>
are inline elements by default.
- Example:
What it looks like:
cssCopy codespan {
display: inline;
background-color: lightgreen;
}
With display: inline
, the element doesn’t force a new line and will only take up as much space as it needs, allowing other elements to sit next to it.
Inline-Block: This is like a mix between
block
andinline
. The element will take up only the space it needs (likeinline
), but you can also add padding, margins, and borders (likeblock
), and it won’t force other elements to a new line.- Example:
cssCopy codediv {
display: inline-block;
width: 100px;
height: 100px;
background-color: lightcoral;
}
In this case, a div
with display: inline-block
will only be as wide as you specify (100px), but it can sit next to other elements without forcing them onto a new line.
None: This value completely hides the element from the page. It’s like turning off the visibility of the element—it's not displayed and takes up no space.
- Example:
cssCopy code.hidden {
display: none;
}
With display: none
, the element is removed from the page layout and doesn’t affect the positioning of other elements.
Why is the Display Property Important?
The Display Property controls the basic structure and layout of your webpage:
Block elements help create sections and divide content into separate chunks.
Inline elements allow text and small elements to flow naturally in a line without breaking the layout.
Inline-block elements give more control over sizing while still allowing elements to sit side by side.
None is used to hide content when it’s not needed, which is useful for interactive content like menus or popups.
Example with CSS
Here’s a simple example showing how each value of display
works:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Display Property Example</title>
<style>
.block {
display: block;
background-color: lightblue;
width: 100px;
margin-bottom: 10px;
}
.inline {
display: inline;
background-color: lightgreen;
padding: 5px;
}
.inline-block {
display: inline-block;
width: 100px;
height: 50px;
background-color: lightcoral;
margin: 5px;
}
</style>
</head>
<body>
<div class="block">Block</div>
<span class="inline">Inline</span>
<span class="inline">Inline</span>
<div class="inline-block">Inline-Block</div>
<div class="inline-block">Inline-Block</div>
</body>
</html>
The
.block
element will take up the full width of the page and push any other elements to the next line.The
.inline
elements will appear side by side on the same line.The
.inline-block
elements will be sized like blocks but will also sit next to each other on the same line, respecting their individual widths.
Understanding the Display Property is crucial for controlling how elements are arranged on your webpage, making it one of the fundamental building blocks of web design.
3. Positioning
CSS provides different ways to position elements, including static
, relative
, absolute
, and fixed
. When you're building a webpage, you often want to decide exactly where each piece of content (like images, text, or buttons) should appear on the page. The CSS Positioning property gives you the power to do just that! It tells the browser how to place an element in relation to its normal position or its surrounding elements.
Main Positioning Values:
Static: This is the default positioning for all elements. When an element is positioned statically, it appears in the normal flow of the document, meaning it will be placed based on the order it appears in the HTML. If you don’t use any positioning properties (like
top
,bottom
,left
, orright
), it will be static.- Example:
cssCopy codediv {
position: static;
}
With static positioning, the element will follow the natural flow of the document.
Relative: When you set an element to
position: relative
, it stays in the normal flow of the document, but you can move it relative to its original position using thetop
,bottom
,left
, orright
properties. The space it originally occupied remains in place, so other elements won’t shift around to fill the gap.- Example:
cssCopy codediv {
position: relative;
top: 20px; /* Moves the element down 20 pixels from its original position */
left: 10px; /* Moves the element right 10 pixels from its original position */
}
Absolute: An element with
position: absolute
is removed from the normal document flow and positioned relative to the nearest positioned ancestor (an ancestor with a position ofrelative
,absolute
, orfixed
). If there’s no such ancestor, it will be positioned relative to the viewport (the browser window). Because it's out of the normal flow, other elements will behave as if it doesn't exist.- Example:
cssCopy code.absolute-box {
position: absolute;
top: 50px; /* 50 pixels from the top of the nearest positioned ancestor or viewport */
left: 100px; /* 100 pixels from the left */
}
Fixed: When an element is set to
position: fixed
, it is also removed from the normal flow, but instead of moving with its parent or the page, it stays in a fixed position relative to the viewport. This means that even if you scroll down the page, the fixed element will stay in the same spot on the screen.- Example:
cssCopy code.fixed-box {
position: fixed;
bottom: 10px; /* 10 pixels from the bottom of the viewport */
right: 20px; /* 20 pixels from the right of the viewport */
}
Sticky: This is a combination of relative and fixed positioning. An element with
position: sticky
behaves like a relative element until it reaches a specified scroll position, then it becomes fixed. It's great for keeping headings visible while scrolling.- Example:
cssCopy code.sticky-box {
position: sticky;
top: 0; /* It will stick to the top of its container when you scroll down */
}
Why is Positioning Important?
Understanding CSS positioning allows you to:
Control the layout of your webpage precisely.
Overlap elements or keep certain elements visible while scrolling (like navigation bars or buttons).
Create complex designs that can adapt to different screen sizes and orientations.
Example with CSS
Here’s an example that shows how different positioning works together:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Positioning Example</title>
<style>
.container {
position: relative; /* This will be the reference for absolute positioning */
width: 400px;
height: 400px;
border: 2px solid black;
margin: 20px;
}
.relative {
position: relative;
top: 10px; /* Moves down 10 pixels */
left: 10px; /* Moves right 10 pixels */
background-color: lightblue;
width: 100px;
height: 100px;
}
.absolute {
position: absolute; /* Positioned relative to the nearest positioned ancestor */
top: 20px; /* 20 pixels from the top of the container */
left: 20px; /* 20 pixels from the left of the container */
background-color: lightgreen;
width: 100px;
height: 100px;
}
.fixed {
position: fixed; /* Stays fixed in the viewport */
bottom: 10px; /* 10 pixels from the bottom */
right: 10px; /* 10 pixels from the right */
background-color: lightcoral;
width: 100px;
height: 100px;
}
</style>
</head>
<body>
<div class="container">
<div class="relative">Relative</div>
<div class="absolute">Absolute</div>
</div>
<div class="fixed">Fixed</div>
</body>
</html>
Breakdown of the Example:
Container: A box with
position: relative
. This is the reference point for the absolutely positioned element.Relative Element: Moves 10 pixels down and to the right from its original spot inside the container.
Absolute Element: Moves 20 pixels down and to the right, positioned relative to the container.
Fixed Element: Always stays 10 pixels from the bottom and right of the viewport, no matter how much you scroll.
By mastering CSS positioning, you can create dynamic, visually engaging web pages that look great and function well!
4. Flexbox (Flexible Box Layout)
Flexbox is a layout model that helps in creating flexible and responsive layouts by distributing space within a container.When you want to create a layout for your webpage that adjusts nicely no matter how big or small the screen is, you can use Flexbox! The Flexible Box Layout (Flexbox) is a powerful CSS layout model that makes it easy to design responsive layouts. It helps you arrange elements in rows or columns and gives you control over how they should grow, shrink, or space out.
How Flexbox Works
To use Flexbox, you need to define a parent container as a flex container, and then all the child elements inside it become flex items. Here’s how it works:
Flex Container: You declare a container as a flex container by setting its
display
property toflex
.- Example:
cssCopy code.container {
display: flex; /* This makes the container a flex container */
}
- Flex Items: The direct children of the flex container become flex items. You can control their size, alignment, and order easily.
Key Flexbox Properties
Here are some important properties you can use with Flexbox:
flex-direction: This property defines the direction of the flex items inside the container. You can set it to:
row
(default): Items are placed in a row, from left to right.column
: Items are placed in a column, from top to bottom.row-reverse
: Items are placed in a row, but in reverse order.column-reverse
: Items are placed in a column, but in reverse order.
Example:
cssCopy code.container {
display: flex;
flex-direction: row; /* Items will be arranged in a row */
}
justify-content: This property controls the alignment of the flex items along the main axis (the direction defined by
flex-direction
). Common values include:flex-start
: Items are packed toward the start of the flex container.flex-end
: Items are packed toward the end.center
: Items are centered in the container.space-between
: Items are evenly distributed, with the first item at the start and the last item at the end.space-around
: Items are evenly distributed with space around them.
Example:
cssCopy code.container {
display: flex;
justify-content: center; /* Centers the items in the flex container */
}
align-items: This property controls the alignment of the flex items along the cross axis (perpendicular to the main axis). Common values include:
flex-start
: Items are aligned to the start of the container.flex-end
: Items are aligned to the end.center
: Items are centered in the container.baseline
: Items are aligned along their baseline.stretch
: Items stretch to fill the container (default).
Example:
cssCopy code.container {
display: flex;
align-items: stretch; /* All items stretch to fill the container height */
}
flex-wrap: This property defines whether the flex items should wrap onto a new line if there isn't enough space. You can set it to:
nowrap
(default): Items will not wrap and will stay in one line.wrap
: Items will wrap onto the next line if there isn't enough space.wrap-reverse
: Items will wrap onto the next line in reverse order.
Example:
cssCopy code.container {
display: flex;
flex-wrap: wrap; /* Items will wrap onto the next line if they overflow */
}
flex: This property is a shorthand for defining how a flex item can grow or shrink to fill the available space. It takes three values:
flex-grow
,flex-shrink
, andflex-basis
. You can use it to set how much space an item should take relative to other items.flex-grow
: Defines how much space the item should take when there's extra space.flex-shrink
: Defines how much the item should shrink when there’s not enough space.flex-basis
: Defines the default size of the item before the remaining space is distributed.
Example:
cssCopy code.item {
flex: 1; /* This means the item can grow to fill the available space equally with other items */
}
Why is Flexbox Important?
Using Flexbox helps you create layouts that:
Adapt: Flexbox makes it easier to create responsive designs that work on different screen sizes and orientations.
Align: It provides a simple way to align items horizontally and vertically without complex calculations.
Space: You can easily distribute space between items, making your layout look balanced and professional.
Example with Flexbox
Here’s a simple example demonstrating how Flexbox works:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexbox Example</title>
<style>
.container {
display: flex; /* Make the container a flex container */
flex-direction: row; /* Arrange items in a row */
justify-content: space-around; /* Distribute space around items */
align-items: center; /* Center items vertically */
height: 100px; /* Set a height for the container */
background-color: lightgray; /* Background color for visibility */
}
.item {
flex: 1; /* Allow items to grow equally */
margin: 5px; /* Add space around items */
background-color: lightblue; /* Background color for items */
text-align: center; /* Center text in items */
padding: 20px; /* Padding inside items */
}
</style>
</head>
<body>
<div class="container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
</div>
</body>
</html>
Breakdown of the Example:
Container: The
.container
is defined as a flex container with items arranged in a row, spaced evenly around each item, and centered vertically.Items: Each
.item
can grow equally to fill the space. The background color and padding make them stand out.
By mastering Flexbox, you can create modern, responsive web layouts that look great on any device! It’s an essential tool for web designers and developers.
5. CSS Grid Layout
CSS Grid is a powerful layout system for creating 2D layouts (both rows and columns) easily.When you want to create complex web layouts that involve both rows and columns, CSS Grid Layout is your best friend! The Grid Layout system allows you to design your webpage in a structured way by dividing it into rows and columns, making it super easy to control the placement of elements on the page.
How Grid Layout Works
To use Grid Layout, you need to define a parent container as a grid container, and then all the child elements inside it become grid items. Here’s how it works:
Grid Container: You declare a container as a grid container by setting its
display
property togrid
.- Example:
cssCopy code.container {
display: grid; /* This makes the container a grid container */
}
- Grid Items: The direct children of the grid container become grid items. You can control their placement within the grid using grid lines and areas.
Key Grid Properties
Here are some important properties you can use with Grid Layout:
grid-template-columns: This property defines the number and size of columns in the grid. You can specify the sizes in pixels, percentages, or use the
fr
(fraction) unit, which allows for flexible sizing.- Example:
cssCopy code.container {
display: grid;
grid-template-columns: 1fr 2fr; /* Creates two columns, the second is twice as wide as the first */
}
grid-template-rows: This property defines the number and size of rows in the grid, similar to
grid-template-columns
.- Example:
cssCopy code.container {
display: grid;
grid-template-rows: 100px 200px; /* Creates two rows, the second is 200 pixels tall */
}
grid-gap (or gap): This property controls the space between grid items, both row-wise and column-wise. You can set a single value for both or separate values for rows and columns.
- Example:
cssCopy code.container {
display: grid;
gap: 10px; /* 10 pixels of space between items */
}
grid-area: This property allows you to assign a specific grid item to a specific area in the grid by naming the areas. You can also use it in conjunction with
grid-template-areas
.- Example:
cssCopy code.item {
grid-area: header; /* Places the item in the area named "header" */
}
grid-template-areas: This property lets you define a visual layout by naming areas in the grid. This is a powerful way to create complex layouts with a simple syntax.
- Example:
cssCopy code.container {
display: grid;
grid-template-areas:
"header header"
"sidebar content";
}
Why is Grid Layout Important?
Using Grid Layout helps you:
Structure: Easily create a structured layout that organizes content in both rows and columns.
Flexibility: Adapt layouts to different screen sizes with responsive design, ensuring that elements look good on any device.
Simplicity: Simplify the process of aligning and positioning elements without the need for complex positioning techniques.
Example with CSS Grid
Here’s a simple example demonstrating how CSS Grid Layout works:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Grid Layout Example</title>
<style>
.container {
display: grid; /* Make the container a grid container */
grid-template-columns: 1fr 3fr; /* Two columns: first takes 1 part, second takes 3 parts */
grid-template-rows: 100px 1fr; /* Two rows: first is 100 pixels, second takes remaining space */
gap: 10px; /* Space between items */
height: 100vh; /* Full height of the viewport */
}
.header {
grid-column: 1 / -1; /* Span across both columns */
background-color: lightblue;
}
.sidebar {
background-color: lightcoral;
}
.content {
background-color: lightgreen;
}
.footer {
grid-column: 1 / -1; /* Span across both columns */
background-color: lightyellow;
}
</style>
</head>
<body>
<div class="container">
<div class="header">Header</div>
<div class="sidebar">Sidebar</div>
<div class="content">Content</div>
<div class="footer">Footer</div>
</div>
</body>
</html>
Breakdown of the Example:
Container: The
.container
is defined as a grid with two columns and two rows. Thegap
property creates space between the grid items.Items:
The header spans across both columns using
grid-column: 1 / -1
.The sidebar and content sit side by side in the second row.
The footer also spans both columns at the bottom.
By mastering CSS Grid Layout, you can create sophisticated and responsive web designs that adapt to various screen sizes and content types, making it a fundamental tool for modern web development!
6. Media Queries (Responsive Design)
Media queries allow your website to be responsive, adapting its layout to different screen sizes and devices. When you create a webpage, you want it to look good on all types of devices—like phones, tablets, and computers. Media Queries are a powerful feature in CSS that allow you to apply different styles to your webpage based on the device's characteristics, such as its screen size, resolution, or orientation. This helps you create responsive designs that adapt to various screen conditions.
How Media Queries Work
Media queries consist of a media type (like screen
for computer and mobile displays) and one or more expressions that check for specific conditions. When these conditions are met, the styles inside the media query are applied.
Basic Structure of a Media Query
Here’s the basic syntax for a media query:
cssCopy code@media media-type and (condition) {
/* CSS rules go here */
}
media-type: Defines the type of device (e.g.,
screen
,print
).condition: Specifies the criteria, like maximum or minimum width or height.
Common Use Cases
Changing Layouts: Adjusting the layout for smaller screens (e.g., stacking columns into rows).
Font Size Adjustments: Making text larger or smaller based on screen size.
Hiding or Showing Elements: Displaying different navigation options for mobile versus desktop.
Example of Media Queries
Here’s an example that demonstrates how to use media queries to change styles based on the screen width:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Media Queries Example</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: lightgray;
margin: 0;
padding: 20px;
}
h1 {
color: darkblue;
}
/* Default styles */
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
}
.box {
width: 30%;
padding: 20px;
background-color: lightblue;
border: 1px solid blue;
box-shadow: 2px 2px 10px rgba(0, 0, 0, 0.1);
}
/* Media query for devices with max width of 768px */
@media screen and (max-width: 768px) {
.container {
flex-direction: column; /* Stack boxes vertically */
}
.box {
width: 100%; /* Make boxes full width */
margin-bottom: 10px; /* Space between boxes */
}
}
/* Media query for devices with max width of 480px */
@media screen and (max-width: 480px) {
h1 {
font-size: 24px; /* Smaller font size */
}
.box {
padding: 10px; /* Reduce padding in boxes */
}
}
</style>
</head>
<body>
<h1>Responsive Media Queries Example</h1>
<div class="container">
<div class="box">Box 1</div>
<div class="box">Box 2</div>
<div class="box">Box 3</div>
</div>
</body>
</html>
Breakdown of the Example:
Default Styles: The default styles create a flexible layout with three boxes displayed in a row.
Media Query for Screens Up to 768px:
Changes the layout to a column format when the screen width is 768 pixels or less.
Makes each box full width and adds space between them.
Media Query for Screens Up to 480px:
Reduces the font size of the heading for smaller devices.
Decreases the padding inside the boxes to make them fit better.
Why are Media Queries Important?
Using media queries helps you:
Create Responsive Designs: Ensure your webpage looks great on all devices, enhancing the user experience.
Control Layout: Adjust layouts dynamically based on available screen space, making it easier to read and navigate.
Optimize Performance: Improve load times by providing device-specific styles, reducing unnecessary CSS for smaller screens.
By mastering media queries, you can build modern, adaptable websites that provide a seamless experience, regardless of the device being used!
7. Z-index
The z-index
property controls the stack order of overlapping elements. Elements with a higher z-index
appear on top of those with lower values. When you create a webpage, you often have multiple elements overlapping each other, like images, text boxes, and buttons. The z-index property in CSS allows you to control the stacking order of these overlapping elements. Think of it like layers in a stack of paper—some pieces might be on top, while others are underneath.
How Z-Index Works
The z-index property only works on positioned elements, which means the elements must have a position
value set to relative
, absolute
, fixed
, or sticky
. The default stacking order of elements is determined by their order in the HTML document. The element that comes later in the document is drawn on top of earlier elements.
Key Points About Z-Index
Default Behavior: By default, elements stack according to their order in the HTML. Elements defined later will cover elements defined earlier.
Positioned Elements: Only elements with a position property of
relative
,absolute
,fixed
, orsticky
can have a z-index.Integer Values: The z-index can take any integer value:
A higher value means the element will be on top of those with lower values.
Negative values can also be used to place elements behind others.
Example of Z-Index
Here’s a simple example demonstrating how to use z-index to control overlapping elements:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Z-Index Example</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 20px;
position: relative; /* Make body a positioned element */
}
.box {
width: 200px;
height: 200px;
position: absolute; /* Use absolute positioning */
}
.box1 {
background-color: lightcoral;
left: 50px;
top: 50px;
z-index: 1; /* Lower z-index */
}
.box2 {
background-color: lightblue;
left: 100px; /* Overlap with box1 */
top: 100px; /* Overlap with box1 */
z-index: 2; /* Higher z-index, so it appears on top */
}
.box3 {
background-color: lightgreen;
left: 150px;
top: 150px;
z-index: 0; /* Lowest z-index, so it appears underneath */
}
</style>
</head>
<body>
<div class="box box1">Box 1</div>
<div class="box box2">Box 2</div>
<div class="box box3">Box 3</div>
</body>
</html>
Breakdown of the Example:
Box Definitions:
Box 1 has a lower z-index (1) and is positioned first, so it appears underneath.
Box 2 has a higher z-index (2) and overlaps Box 1, so it appears on top.
Box 3 has the lowest z-index (0) and is positioned below both Box 1 and Box 2, making it the furthest back.
Why is Z-Index Important?
Using z-index helps you:
Control Overlapping Elements: Determine which elements should appear in front or behind others, giving you precise control over your layout.
Create Layered Designs: Build more visually engaging designs where elements overlap creatively.
Manage Interactivity: Ensure that interactive elements (like buttons or modals) are accessible by keeping them on top of other elements.
By mastering the z-index property, you can effectively manage the stacking order of elements on your webpage, making your designs look organized and professional!
8. CSS Transitions
Transitions allow smooth changes between property values when an element’s state changes (e.g., hover). CSS transitions allow you to create smooth changes between different styles of an element over a specified duration. Instead of an element changing instantly from one style to another, transitions let it gradually change, adding a sense of motion and polish to your web designs. This can make your website feel more dynamic and engaging for users.
How CSS Transitions Work
To create a transition, you need to define the properties you want to animate, the duration of the animation, and optionally, the timing function (which controls how the transition progresses). You can apply transitions to various CSS properties like color
, background-color
, width
, height
, and more.
Basic Structure of a CSS Transition
Here’s the basic syntax to set up a transition:
cssCopy code.element {
transition: property duration timing-function delay;
}
property: The CSS property you want to animate (e.g.,
background-color
,width
).duration: How long the transition should take (e.g.,
0.5s
for half a second).timing-function: Optional. This controls the speed of the transition (e.g.,
ease
,linear
,ease-in
,ease-out
).delay: Optional. The amount of time to wait before starting the transition.
Example of CSS Transitions
Here’s a simple example demonstrating how to use CSS transitions to animate a button when hovered over:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Transitions Example</title>
<style>
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f4f4f4; /* Light gray background */
}
.button {
padding: 15px 30px;
font-size: 16px;
color: white;
background-color: #007BFF; /* Blue background */
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease, transform 0.3s ease; /* Transition for color and scale */
}
.button:hover {
background-color: #0056b3; /* Darker blue */
transform: scale(1.1); /* Scale up the button */
}
</style>
</head>
<body>
<button class="button">Hover Me!</button>
</body>
</html>
Breakdown of the Example:
Button Styles: The button is styled with padding, color, and a border-radius.
Transition Property: The
transition
property is set to animate thebackground-color
andtransform
properties over 0.3 seconds with an ease timing function.Hover Effect: When the button is hovered over, the background color changes to a darker blue, and the button scales up slightly (10% larger).
Why are CSS Transitions Important?
Using CSS transitions helps you:
Enhance User Experience: Smooth transitions make interactions feel more natural and engaging, improving the overall user experience.
Highlight Changes: Draw attention to elements that change, such as buttons or links, making it clear to users that something is interactive.
Create Visual Interest: Add aesthetic appeal to your website by using transitions to create dynamic effects without needing complex animations or JavaScript.
By mastering CSS transitions, you can elevate your web designs with polished effects, making your sites more enjoyable and visually appealing for users!
9. Pseudo-Classes and Pseudo-Elements
Pseudo-classes are used to define the state of an element (like :hover
, :active
), while pseudo-elements create elements that don’t exist in the HTML, such as ::before
and ::after
. In CSS, pseudo-classes and pseudo-elements are powerful tools that allow you to apply styles to elements based on their state or structure without adding extra classes or elements to your HTML. They help enhance the functionality and appearance of your webpage by providing a way to target specific parts of an element or its states.
Pseudo-Classes
Pseudo-classes are used to define a special state of an element. They enable you to style elements when a user interacts with them, such as when they hover over a link or focus on an input field.
Common Pseudo-Classes
:hover
: Applies styles when the user hovers over an element.- Example:
cssCopy codea:hover {
color: red; /* Changes link color to red on hover */
}
:focus
: Applies styles when an element, like an input field, is focused (clicked or tabbed into).- Example:
cssCopy codeinput:focus {
border: 2px solid blue; /* Highlights input border when focused */
}
:nth-child(n)
: Applies styles to elements based on their position in a parent element (e.g., every second child).- Example:
cssCopy codeli:nth-child(2) {
background-color: lightgray; /* Styles the second list item */
}
Pseudo-Elements
Pseudo-elements are used to style specific parts of an element, such as the first line of a paragraph or a specific part of a text. They let you add styles to elements without modifying the HTML structure.
Common Pseudo-Elements
::before
: Inserts content before the content of an element.- Example:
cssCopy codeh1::before {
content: "Note: "; /* Adds "Note: " before every h1 */
color: orange; /* Styles it with an orange color */
}
::after
: Inserts content after the content of an element.- Example:
cssCopy codep::after {
content: " (end)"; /* Adds "(end)" after every paragraph */
font-style: italic; /* Styles it in italics */
}
::first-line
: Styles the first line of a block of text.- Example:
cssCopy codep::first-line {
font-weight: bold; /* Makes the first line of the paragraph bold */
}
::first-letter
: Styles the first letter of a block of text.- Example:
cssCopy codep::first-letter {
font-size: 2em; /* Increases the size of the first letter */
color: blue; /* Changes its color to blue */
}
Example of Pseudo-Classes and Pseudo-Elements
Here’s a simple example to show how both pseudo-classes and pseudo-elements work together:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Pseudo-Classes and Pseudo-Elements Example</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
a {
color: blue;
text-decoration: none;
}
a:hover {
color: red; /* Changes link color on hover */
}
p::first-line {
font-weight: bold; /* Makes the first line of the paragraph bold */
}
p::first-letter {
font-size: 2em; /* Increases the size of the first letter */
color: green; /* Changes its color to green */
}
</style>
</head>
<body>
<h1>Learning CSS Pseudo-Classes and Pseudo-Elements</h1>
<p>This is a simple paragraph to demonstrate the use of pseudo-elements. <a href="#">Hover over this link</a> to see the effect.</p>
</body>
</html>
Breakdown of the Example:
Link Styling: The link changes color when hovered over, using the
:hover
pseudo-class.First Line and First Letter: The first line of the paragraph is bold, and the first letter is larger and green, demonstrating how pseudo-elements can style specific parts of the content.
Why are Pseudo-Classes and Pseudo-Elements Important?
Using pseudo-classes and pseudo-elements helps you:
Enhance Interactivity: Create responsive designs that react to user actions, improving engagement.
Add Style Without Extra Markup: Style specific parts of elements or states without modifying your HTML, keeping it clean and semantic.
Create Unique Designs: Apply unique styles to different states or parts of elements, making your webpage more visually appealing.
By mastering pseudo-classes and pseudo-elements, you can create more interactive and well-styled web pages, enriching the user experience without cluttering your HTML structure!
10. CSS Variables (Custom Properties)
CSS variables allow you to store values that can be reused throughout your styles, making it easier to manage and update your CSS. CSS Variables, also known as custom properties, are a powerful feature in CSS that allow you to store values that can be reused throughout your stylesheets. This makes it easier to maintain and update your styles, as you can change a single variable and have that change apply wherever the variable is used.
How CSS Variables Work
CSS variables are defined using a specific syntax and are scoped to the element they are defined on. You typically define a variable within a CSS selector and access it using the var()
function.
Defining CSS Variables
To define a CSS variable, you use the following syntax:
cssCopy code:root {
--variable-name: value;
}
:root
: This selector targets the root element of the document (usually the<html>
element), making the variable available globally.--variable-name
: The name of the variable, which must start with two dashes (--
).value
: The value you want to assign to the variable (e.g., colors, sizes, etc.).
Using CSS Variables
To use the defined variable, you use the var()
function:
cssCopy codeelement {
property: var(--variable-name);
}
Example of CSS Variables
Here’s a simple example demonstrating how to use CSS variables to manage colors and spacing in a webpage:
htmlCopy code<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Variables Example</title>
<style>
:root {
--primary-color: #3498db; /* A shade of blue */
--secondary-color: #2ecc71; /* A shade of green */
--text-color: #333; /* Dark gray */
--padding: 20px; /* Standard padding */
}
body {
font-family: Arial, sans-serif;
color: var(--text-color); /* Use the text color variable */
margin: 0;
padding: var(--padding); /* Use the padding variable */
background-color: #f4f4f4; /* Light gray background */
}
h1 {
color: var(--primary-color); /* Use the primary color variable */
}
button {
background-color: var(--secondary-color); /* Use the secondary color */
color: white;
padding: var(--padding); /* Use the padding variable */
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease; /* Smooth transition for hover */
}
button:hover {
background-color: #27ae60; /* Darker green on hover */
}
</style>
</head>
<body>
<h1>Welcome to CSS Variables!</h1>
<button>Click Me!</button>
</body>
</html>
Breakdown of the Example:
Variable Definitions: The variables are defined in the
:root
selector, making them globally accessible. This includes colors and padding values.Using Variables: Throughout the styles, the
var()
function is used to apply the variables to different properties, such as color and padding.Hover Effect: The button changes color when hovered over, using a hardcoded color value.
Why are CSS Variables Important?
Using CSS variables helps you:
Enhance Maintainability: Make it easier to update your styles by changing the value of a variable in one place, rather than hunting down every instance.
Promote Consistency: Ensure consistent styling across your website by reusing the same variables for colors, sizes, and other properties.
Facilitate Theming: Create themes for your website easily by adjusting variable values to change the overall look without rewriting styles.
By mastering CSS variables, you can make your stylesheets more organized and flexible, allowing for easier updates and a more dynamic web design!
In Conclusion
In conclusion, I discussed the three ways to insert CSS: External, Internal, and Inline, along with their pros and cons. Mastering CSS concepts like the box model, display property, positioning, flexbox, grid, media queries, and z-index, as well as learning about pseudo-classes, pseudo-elements, transitions, and CSS variables, elevates you from simply styling web pages to controlling the very essence of the web's appearance. Think of CSS as the wardrobe department of the internet—you get to decide if your site wears a sleek suit, comfy pajamas, or (let's be honest) that one "coming soon" page you keep forgetting to style.
With CSS, it's not just about making things look good—it's about creating a seamless, responsive experience for users. And once you start using CSS variables, maintaining your code feels less like wrangling a wild horse and more like riding a well-trained stallion. After all, why hunt down and change every instance of a color when you can let a single variable handle it? (And let’s be real, it's the closest we’ll get to casting spells in web development.)
So, as you dive deeper into CSS, remember: your site is your stage, and you’re the designer and director. Just make sure it doesn't crash on opening night—unless you're aiming for a performance art piece about failed web development.
Thoughts and Feelings
I’m so happy, plain and simple. I’ve said it once, and I’ll say it a thousand times: I love coding, programming, and learning. That’s why I know I’m doing what’s right for me. I can look in the mirror and see an amazing programmer. I’m waiting for imposter syndrome to kick in, as I’ve heard it does for many, but right now, I’m filled with confidence. One reason is my belief in myself, and the other is FreeCodeCamp and their teaching methods. I’m picking up the information well; I know this because I practice on leetcode.com, use apps like Mimo when I’m AFK (Away From Keyboard), and generate pop quiz questions for myself with ChatGPT. I aim for 20 questions a day to retain and comprehend all the information I’ve learned so far. This helps verify that I’m learning, which can be challenging on this long, never-ending yellow brick road that is the self-taught programmer’s path.
Story Time
I went to a high school game this week and watched my girlfriend's nephew play soccer. It was a lot of fun. For privacy reasons, I won't give any details about them except to say, "Go Eagles!" I do want to mention how talented he is. Although the team lost 0-1, they played hard and had fun. They went on to win the following game 2-0, and my nephew scored one of the goals. He's very talented and driven.
After the game, we caught up since it had been two months since I started my self-taught journey. I found out he's done some coding and is also interested in it. We talked about it, and I told him about my blog, FreeCodeCamp, and the meetups I've been attending. He could hear my excitement, and I'd love to help him or even do a project together. I will aim to support him in any way I can, even if it's just a hobby for him.
Moral of the story: talk about your passions, get out and into others' lives, take time to have fun, and share interests. Thank you for reading. This has been a very long one. I hope you enjoyed it, learned something, and until next time, this has been another installment of MIKESELFTAUGHTBOLG.
Subscribe to my newsletter
Read articles from Michael Baisden directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
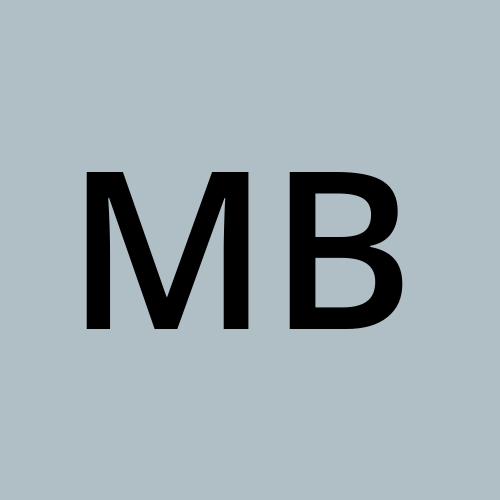
Michael Baisden
Michael Baisden
I am a developer from Columbus, Ohio, born and raised. I've always been into coding and working with computers. At the end of July 2024, I decided to teach myself how to program and write code. I love video games and music. I also love food—cooking, eating, and sharing. I am a God-loving man who keeps an open mind and loves to learn. I have a loving, caring girlfriend named Kerry and a cute dachshund named Kobe. When I'm not working or coding, I'm usually with them.