How to Send Emails in a Laravel Project Using Gmail SMTP?
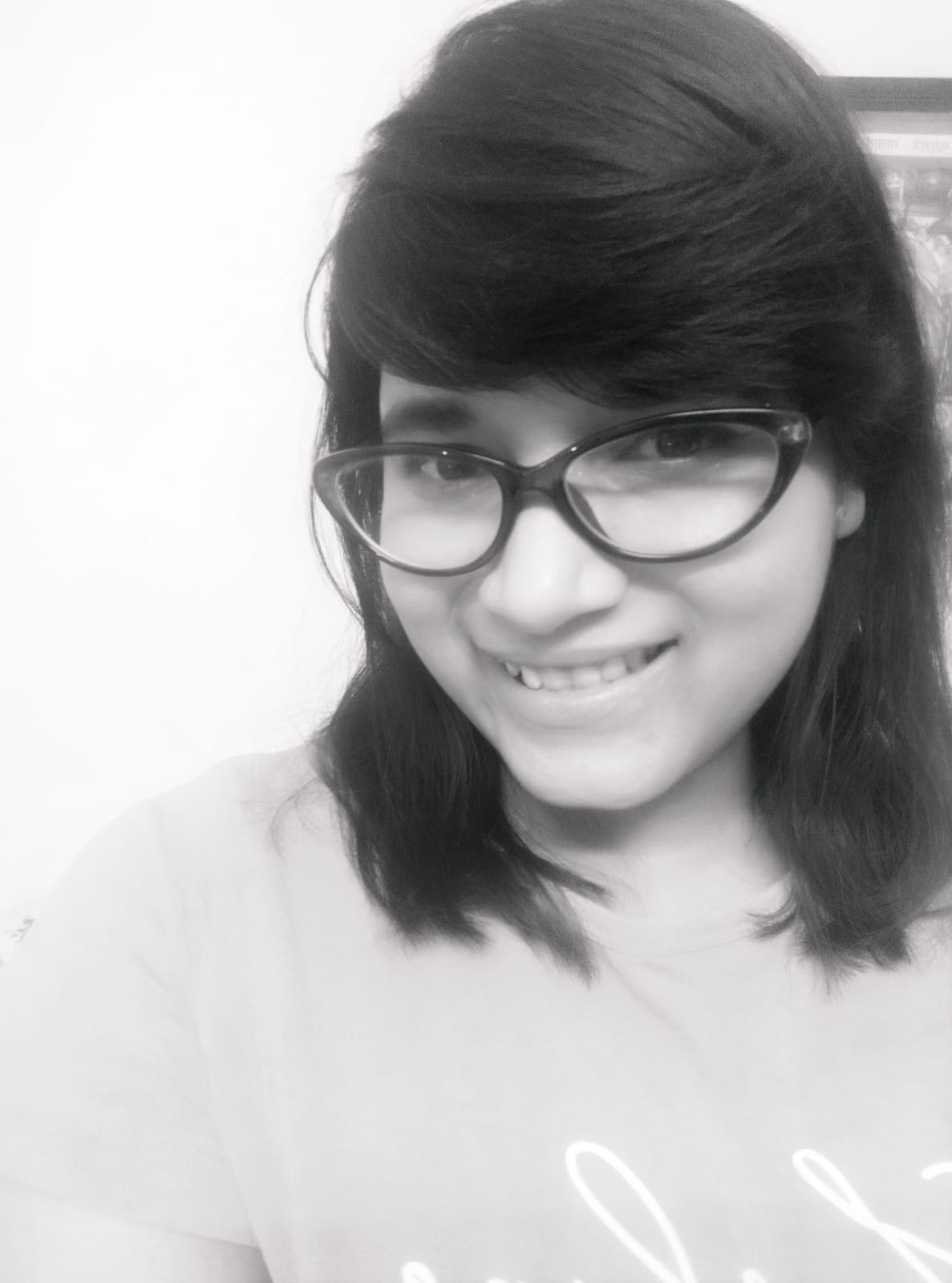
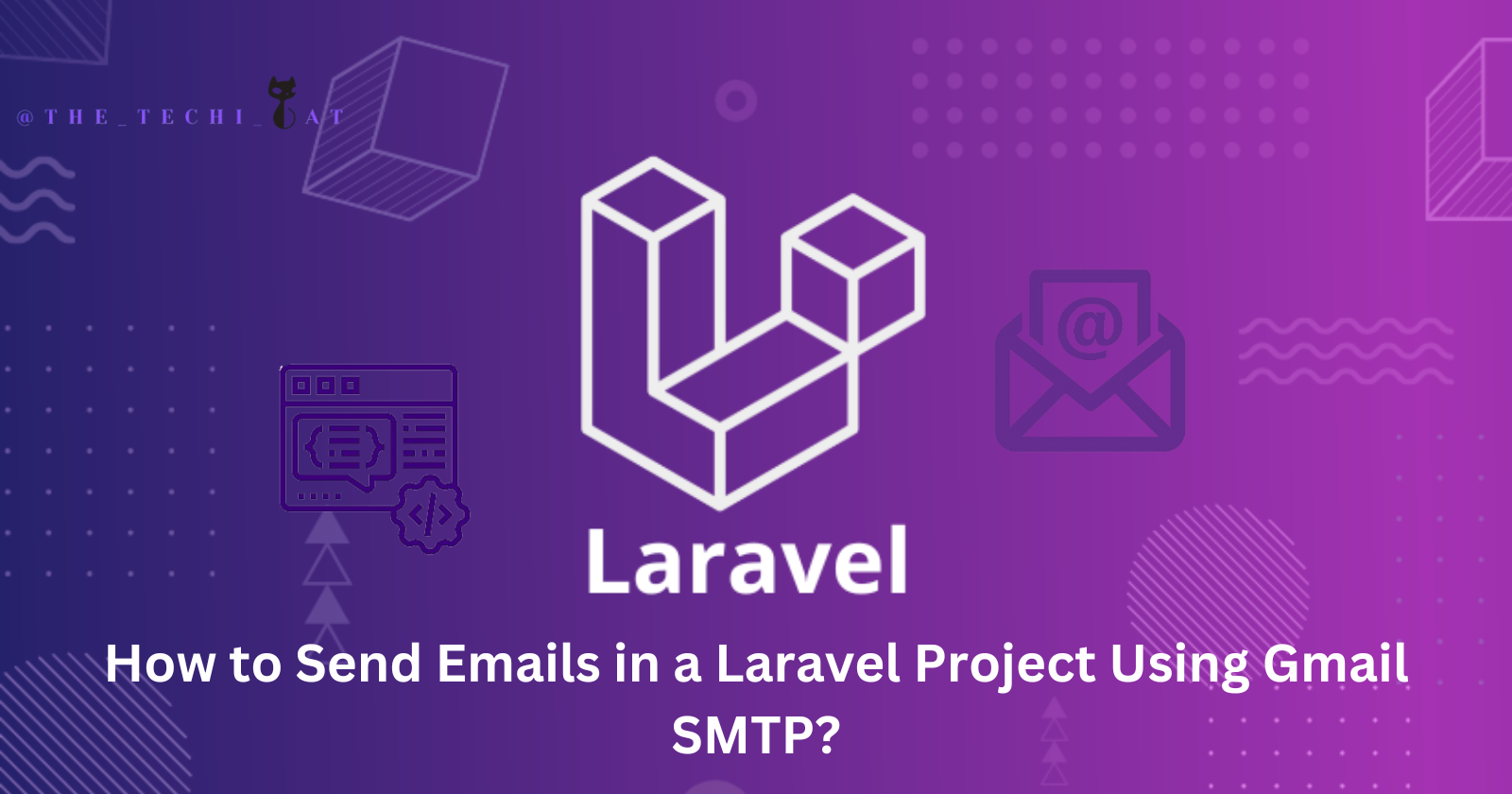
In many web applications, sending emails is a fundamental feature. Laravel provides an elegant and robust solution for handling emails, including sending emails via external SMTP services like Gmail. Integrating Gmail’s SMTP server lets you send secure emails through a trusted provider.
This comprehensive guide will walk you through the step-by-step process of configuring Gmail SMTP in a Laravel project.
Prerequisites
Before proceeding, ensure that you have the following:
Laravel: A working Laravel application.
Gmail Account: A valid Gmail account for SMTP configuration.
Composer: Ensure that Composer is installed to manage your dependencies.
Basic Understanding of Laravel: Familiarity with Laravel routes, controllers, and environment variables.
Step 1: Install and Configure Laravel Mailer
Laravel uses a mail configuration file to define various mail drivers. To start sending emails, ensure that you have the correct Laravel setup for mail services.
1.1 Installing Laravel
First, if you haven't installed Laravel, use Composer to set up a new project:
composer create-project --prefer-dist laravel/laravel laravel-gmail-smtp
If you already have a Laravel project, ensure that it’s updated to the latest version for optimal compatibility.
1.2 Set Up .env
for Gmail SMTP
The email configuration is primarily managed through the .env
file. Update your .env
file to include the Gmail SMTP settings:
MAIL_MAILER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=your-gmail-username@gmail.com
MAIL_PASSWORD=your-app-password
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=your-gmail-username@gmail.com
MAIL_FROM_NAME="${APP_NAME}"
Explanation of Fields:
MAIL_MAILER: Set to
smtp
for Gmail.MAIL_HOST: Gmail’s SMTP server is
smtp.gmail.com
.MAIL_PORT: Gmail uses port
587
for TLS encryption.MAIL_USERNAME: Your Gmail email address.
MAIL_PASSWORD: Generate an App Password from your Google Account (explained below).
MAIL_ENCRYPTION: Set to
tls
for secure email transmission.MAIL_FROM_ADDRESS: The email address that the email will appear to come from.
MAIL_FROM_NAME: Name to be used as the sender.
1.3 Create an App Password
If you have 2-Step Verification enabled for your Gmail account, you will need to create an App Password to use in your Laravel configuration.
To generate an App Password:
Log in to your Google account and go to Google Account Security.
Under "Signing in to Google," enable 2-Step Verification if it's not enabled.
Once 2-step Verification is set, find the App Passwords section and generate a password for your Laravel application.
Use this password in the .env
file under MAIL_PASSWORD
.
Step 2: Test Gmail SMTP Integration
Now that the configuration is set, you can test if everything is working as expected by sending a test email.
2.1 Create a Mail Controller
In your terminal, run the following command to create a Mail Controller:
php artisan make:controller MailController
This command creates a new controller file. Navigate to app/Http/Controllers/MailController.php
and add the following code:
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Mail;
class MailController extends Controller
{
public function sendEmail()
{
$details = [
'title' => 'Test Email from Laravel',
'body' => 'This is a test email sent using Gmail SMTP in Laravel.'
];
Mail::to('recipient@example.com')->send(new \App\Mail\TestMail($details));
return 'Email Sent Successfully!';
}
}
2.2 Create a Mailable Class
Next, generate a Mailable class by running:
php artisan make:mail TestMail
This will create a TestMail.php
file in the app/Mail
directory. Open this file and update it as follows:
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class TestMail extends Mailable
{
use Queueable, SerializesModels;
public $details;
public function __construct($details)
{
$this->details = $details;
}
public function build()
{
return $this->subject('Mail from Laravel')
->view('emails.testMail');
}
}
2.3 Create a Blade View for the Email
Now, create a simple Blade view to format the email content. In the resources/views/emails
directory, create a file named testMail.blade.php
:
<!DOCTYPE html>
<html>
<head>
<title>Test Email</title>
</head>
<body>
<h1>{{ $details['title'] }}</h1>
<p>{{ $details['body'] }}</p>
</body>
</html>
2.4 Set Up Routes
To send the email, create a route in the routes/web.php
file:
use App\Http\Controllers\MailController;
Route::get('send-email', [MailController::class, 'sendEmail']);
Step 3: Sending the Email
To trigger the email, simply access the route http://your-domain/send-email
. If everything is set up correctly, you should see the message Email Sent Successfully!
and an email should arrive in the specified recipient’s inbox.
Step 4: Handling Errors and Debugging
4.1 View Laravel Logs
If you encounter any errors while sending the email, check the Laravel logs located at storage/logs/laravel.log
. Look for any issues related to email configuration, such as incorrect credentials, SSL problems, or connectivity issues.
4.2 Enable Verbose Error Logging
You can also configure Laravel to show detailed SMTP errors by updating the config/mail.php
file and setting the log_channel
:
'log_channel' => env('MAIL_LOG_CHANNEL', 'stack'),
This ensures that all email-related logs are properly stored.
Step 5: Security Best Practices for Gmail SMTP in Laravel
Using Gmail’s SMTP for sending emails in Laravel is convenient, but it’s important to ensure security. Here are a few tips:
Use App Passwords: Do not use your main Gmail password in the
.env
file. Always generate and use App Passwords.Secure
.env
file: Make sure your.env
file is not exposed publicly. Double-check that it is included in your.gitignore
file.Limit API Access: If possible, limit the IPs and applications that can access your Gmail SMTP to mitigate unauthorized access.
Conclusion
By following the steps outlined in this guide, you can easily set up Gmail’s SMTP service in your Laravel project for sending emails. This provides a reliable, secure, and scalable way to integrate email functionality into your web applications.
Subscribe to my newsletter
Read articles from Suravi Farmania directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
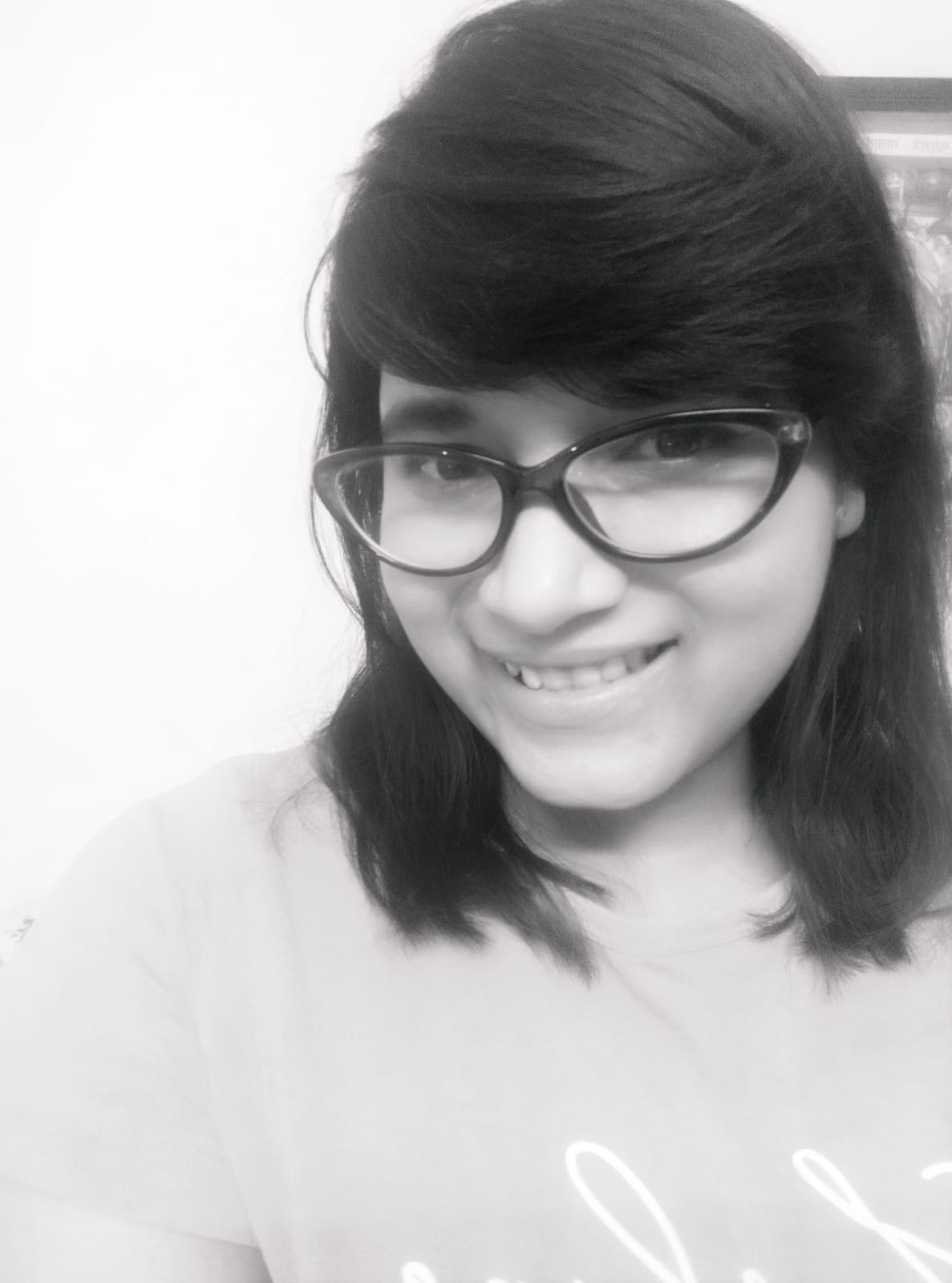
Suravi Farmania
Suravi Farmania
As a software engineer mostly focusing on the backend, I use this platform to share what I’ve learned, explore new topics, and document my journey in tech.