[Detailed Guide] How to Combine Word Documents in Python Quickly

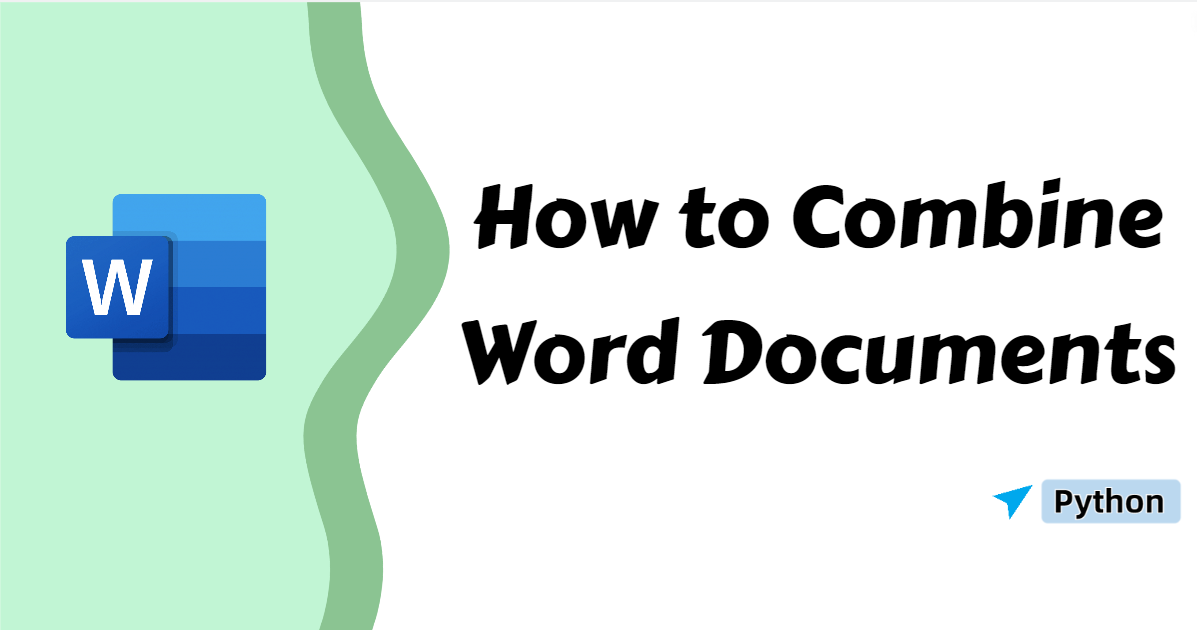
Merging Word documents is a common task both in work and life. While you can manually copy and paste contents from one document to another, this approach quickly becomes impractical when handling multiple files, such as collecting reports. It can be time-consuming and may lead to formatting issues.
But don’t worry, this blog will explore how to combine Word documents in Python, offering a solution that simplifies the process. By automating the task, you can save time and merge Word files seamlessly.
Prepare for Tasks
To combine multiple Word documents in Python, we will use Spire.Doc for Python. This Python component enables developers to merge multiple MS Word files without hassle. Furthermore, this Word control is compatible with Microsoft Word documents in a variety of formats, from 97-03 to 2007-2019.
You can install Spire.Doc for Python from PyPI using the following pip command:
pip install Spire.Doc
If you already have Spire.PDF for Python installed and would like to upgrade to the latest version, use the following pip command:
pip install --upgrade Spire.Doc
How to Combine Word Documents by Inserting Files
Now that you have fully prepared, let’s get down to the point. Spire.Doc for Python offers the method, Document.InsertTextFromFile(), to help you merge Word documents right from files. Read the detailed steps below and learn how it works.
Steps to combine Word documents by inserting files:
Import essential modules.
Create an object of the Document class and use the Document.LoadFromFile() method to open a Word document from the disk.
Merge Word documents by calling the Document.InsertTextFromFile() method.
Save the document as a new file with the Document.SaveToFile() method.
Release the resource.
Below is the code example of combining Word documents directly by inserting files:
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load a Word document from the disk
doc.LoadFromFile("/sample.docx")
# Insert the content from another Word document to the current one
doc.InsertTextFromFile("/sample2.docx", FileFormat.Auto)
# Save the updated document
doc.SaveToFile("/InsertDocuments.docx", FileFormat.Docx2016)
# Release resources
doc.Close()
How to Combine Word Documents by Cloning Contents
Merging Word documents can be finished by cloning contents from one document to another one. This solution is simply a copy-and-paste task, but when performing this task with Python, it's more detailed. You first need to identify a section, then iterate through all the objects contained in the section, and finally clone them into the target document.
Steps to combine Word documents by Cloning:
Import needed modules.
Create instances of the Document class.
Read Word files using the Document.LoadFromFile() method.
Get a section of the target document with the Document.Sections.get_item() method.
Iterate through the sections and their child objects in the source document, retrieving each one using the Body.ChildObjects.get_Item() method.
Add these objects to the target document by calling the Body.ChildObjects.Add() method.
Save the updated document to the disk with the Document.SaveToFile() method.
Release the resource.
Here is the code example of combing Word documents by cloning contents:
from spire.doc import *
from spire.doc.common import *
# Create two objects of the Document class and load two Word documents
doc1 = Document()
doc1.LoadFromFile("/sample.docx")
doc2 = Document()
doc2.LoadFromFile("/sample2.docx")
# Get the last section of the first document
lastSection = doc1.Sections.get_Item(doc1.Sections.Count - 1)
# Loop through sections in the second document
for i in range(doc2.Sections.Count):
section = doc2.Sections.get_Item(i)
# Loop through the child objects in the sections
for j in range(section.Body.ChildObjects.Count):
obj = section.Body.ChildObjects.get_Item(j)
# Add the child objects from the second document to the last section of the first document
lastSection.Body.ChildObjects.Add(obj.Clone())
# Save the resulting document as a new Word document
doc1.SaveToFile("/MergeByCloning.docx")
# Release resources
doc1.Close()
doc2.Close()
Body.ChildObjects
), so headers and footers from the second document will not be included.The Conclusion
This article explains how to combine Word documents using Python, including inserting files and cloning contents. Moreover, there are step-by-step instructions and code examples for your reference. After checking this page, you can handle Word document combinations with no effort.
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
