How to Use Terraform: The Complete Guide

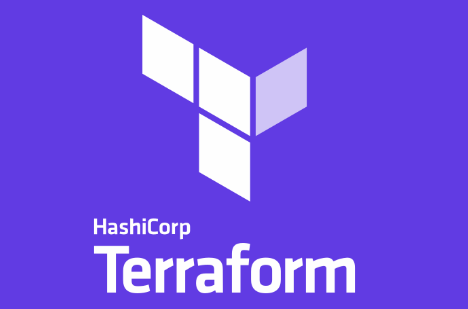
Introduction
In today’s world of cloud computing, managing infrastructure manually is both time-consuming and prone to errors. Enter Terraform – an open-source infrastructure-as-code (IaC) tool developed by HashiCorp that allows you to define, provision, and manage your cloud infrastructure with code. Whether you're deploying resources to AWS, Azure, Google Cloud, or other providers, Terraform makes it easier to build, scale, and maintain your infrastructure consistently.
This blog post is a deep dive into how to use Terraform to automate your infrastructure deployments. If you're a software engineer, DevOps enthusiast, or cloud architect, this post will walk you through a step-by-step guide to getting started with Terraform. By the end, you'll understand how to use Terraform to define infrastructure as code and deploy it across various cloud platforms.
What We’ll Cover:
Installing Terraform
Setting up Your First Terraform Configuration
Terraform Providers and Modules
Provisioning Resources with Terraform
State Management in Terraform
Using Terraform in CI/CD Pipelines
Let’s dive in!
Step 1: Installing Terraform
Before writing any code, you need to install Terraform on your machine. Terraform supports various platforms, and installation is a breeze.
1.1 Downloading Terraform
You can download Terraform by visiting Terraform's official website. Choose your operating system (e.g., Windows, macOS, Linux) and download the appropriate binary.
For Linux users, you can run:
bashCopy codecurl -O https://releases.hashicorp.com/terraform/1.0.11/terraform_1.0.11_linux_amd64.zip
unzip terraform_1.0.11_linux_amd64.zip
sudo mv terraform /usr/local/bin/
terraform --version
1.2 Verifying the Installation
After installing Terraform, verify the installation by running:
bashCopy codeterraform --version
This command will display the version number of Terraform installed on your machine.
Suggested Visual: A simple flowchart showing the steps to download, unzip, and verify Terraform installation.
Step 2: Setting up Your First Terraform Configuration
Now that Terraform is installed, it's time to write your first configuration. Terraform configurations are written in HCL (HashiCorp Configuration Language), which is easy to understand.
2.1 Creating a Directory for Your Project
Create a new directory for your Terraform configuration. This is where all your Terraform files will be stored.
bashCopy codemkdir terraform-demo
cd terraform-demo
2.2 Writing a Simple Configuration File
Let’s start by writing a configuration file that provisions an AWS EC2 instance. Create a file called main.tf
in your project directory.
hclCopy codeprovider "aws" {
region = "us-east-1"
}
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "Terraform-Example"
}
}
In this configuration:
We define AWS as the provider.
The aws_instance resource creates an EC2 instance.
The
ami
andinstance_type
specify the Amazon Machine Image (AMI) and instance type.The
tags
block allows us to tag the instance for easy identification.
2.3 Initializing Terraform
Before running the configuration, initialize Terraform by running:
bashCopy codeterraform init
This command downloads the necessary provider plugins (in this case, the AWS provider) and sets up your working directory for running Terraform commands.
Suggested Visual: A tree diagram showing the project structure with main.tf
file and the initialization process, from local project to cloud provider connection.
Step 3: Terraform Providers and Modules
Terraform providers and modules are core components of how Terraform interacts with various cloud platforms and services.
3.1 Understanding Providers
Providers are responsible for defining resources and interacting with APIs. Each cloud provider (e.g., AWS, Azure, Google Cloud) has its own Terraform provider, which you can configure.
In our previous example, we used the AWS provider. Let’s break down how you can configure different providers, like Azure and GCP, in your Terraform configurations.
For Azure:
hclCopy codeprovider "azurerm" {
features = {}
}
For Google Cloud:
hclCopy codeprovider "google" {
project = "my-project-id"
region = "us-central1"
}
3.2 Using Modules
Modules are reusable pieces of Terraform configuration. They allow you to create abstractions that can be shared across different projects, improving consistency and reducing code duplication.
For instance, you can define a module that provisions a VPC in AWS. In your main configuration, you simply call the module instead of repeating the code.
hclCopy codemodule "vpc" {
source = "./modules/vpc"
cidr = "10.0.0.0/16"
}
Suggested Visual: A conceptual diagram showing how providers connect Terraform to different cloud platforms and how modules can be reused across configurations.
Step 4: Provisioning Resources with Terraform
Now, it’s time to provision your resources. After writing and initializing your configuration, you can use the terraform apply
command to create infrastructure in your cloud environment.
4.1 Running Terraform Plan
Before applying your configuration, you can use the terraform plan
command to see what actions Terraform will perform.
bashCopy codeterraform plan
This command shows a detailed output of the resources that will be created, modified, or destroyed. It’s a good way to review your changes before making them.
4.2 Applying the Configuration
Once you're satisfied with the plan, you can apply the configuration:
bashCopy codeterraform apply
Terraform will prompt you to confirm the action before proceeding. Once you confirm, it will provision the resources defined in your configuration.
4.3 Verifying Resource Creation
After the apply process completes, you can verify the created resources by logging into your cloud provider’s console. For example, if you’ve provisioned an AWS EC2 instance, you should see it in the EC2 Dashboard in the AWS Management Console.
Suggested Visual: A screenshot of the terraform apply
command showing the process of resource creation and a screenshot of the AWS console with the provisioned EC2 instance.
Step 5: State Management in Terraform
Terraform uses state files to keep track of the infrastructure it manages. State files contain the information about your resources and help Terraform determine what changes to make.
5.1 Understanding the Terraform State
By default, Terraform stores its state locally in a file called terraform.tfstate
. However, for collaboration, you might want to store the state remotely (e.g., in S3 for AWS).
hclCopy codeterraform {
backend "s3" {
bucket = "my-terraform-state"
key = "terraform.tfstate"
region = "us-east-1"
}
}
5.2 Locking the State
When storing state remotely, Terraform supports state locking to prevent multiple users from running terraform apply
simultaneously. This ensures that no conflicting changes are made.
Suggested Visual: A flowchart illustrating how Terraform tracks state locally and in remote backends (like S3). Include how state locking prevents conflicts in a shared environment.
Step 6: Using Terraform in CI/CD Pipelines
Once you’ve mastered the basics of Terraform, it’s time to integrate it into your CI/CD pipeline. Terraform can be used in automated workflows to deploy infrastructure alongside your code.
6.1 Setting up Terraform in GitLab CI/CD
Let’s configure a basic pipeline to run Terraform commands in GitLab CI. Create a .gitlab-ci.yml
file in your repository:
yamlCopy codestages:
- apply
apply_infrastructure:
stage: apply
script:
- terraform init
- terraform apply -auto-approve
only:
- master
This pipeline runs the terraform apply
command automatically whenever changes are pushed to the master
branch.
6.2 Using Terraform with GitHub Actions
For GitHub Actions, create a .github/workflows/terraform.yml
file:
yamlCopy codename: 'Terraform'
on:
push:
branches:
- main
jobs:
terraform:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Terraform
uses: hashicorp/setup-terraform@v1
- name: Initialize Terraform
run: terraform init
- name: Apply Terraform
run: terraform apply -auto-approve
Suggested Visual: A diagram or flowchart showing the integration of Terraform into a CI/CD pipeline, illustrating how the Terraform workflow is triggered by code changes.
Conclusion
Terraform is a powerful and flexible tool that allows you to define and manage your infrastructure as code. By following this guide, you've learned how to install Terraform, write basic configuration files, provision resources, manage state, and integrate Terraform into CI/CD pipelines.
With Terraform, infrastructure management becomes more efficient, scalable, and consistent across cloud environments. If you haven’t yet explored Terraform’s full potential, now is the time to start!
Have you used Terraform in your projects? What are some challenges you've faced while managing infrastructure as code? Feel free to drop a comment below – I'd love to hear about your experiences!
Meta Description: Learn how to use Terraform to provision and manage cloud infrastructure as code. This guide covers installation, providers, modules, and integration into CI/CD pipelines.
Subscribe to my newsletter
Read articles from Deepak parashar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
