Mastering HTTP Node Proxy: Guide for Developers and Businesses

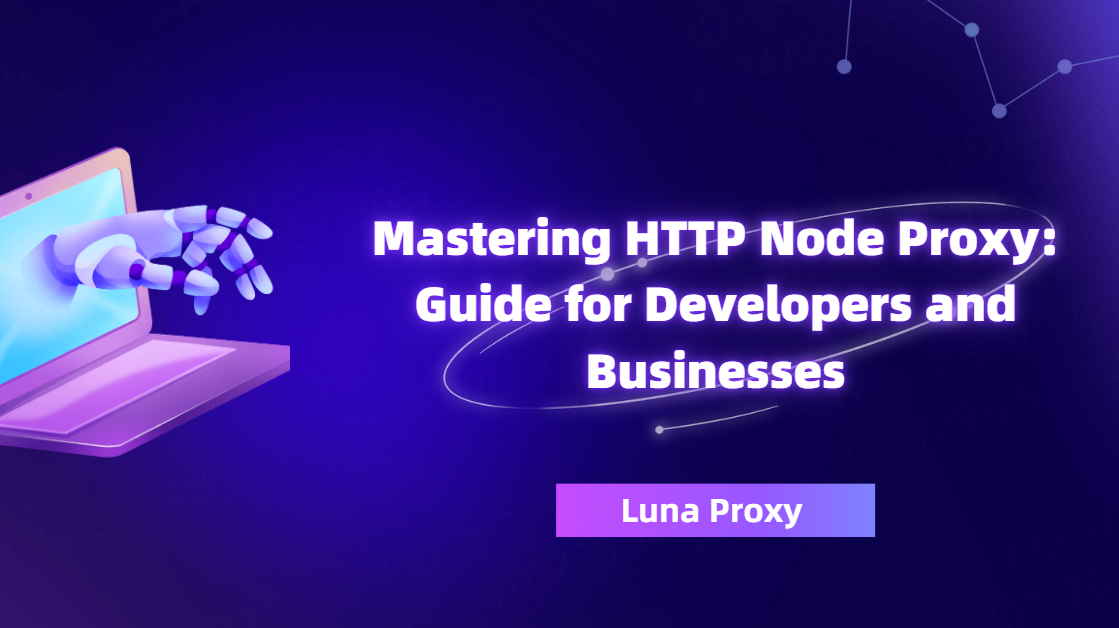
For developers, businesses, and anyone dealing with web services, proxies play a crucial role in achieving these goals. Among the most versatile and widely used technologies is the HTTP node proxy. This guide will walk you through what an HTTP node proxy is, how it works, and why it's essential for optimizing online operations. We’ll also explore how to implement and best utilize these proxies to maximize your efficiency and security.
What Is an HTTP Node Proxy?
An HTTP node proxy is a server that acts as an intermediary between a client making a request and the server receiving it. In practical terms, when you send an HTTP request (like visiting a website or accessing an API), the proxy intercepts the request, processes it, and then forwards it to the destination server on your behalf. When the server responds, the proxy retrieves the data and sends it back to the client.
Why Use an HTTP Node Proxy?
HTTP node proxies offer several advantages, making them a valuable tool for developers and businesses alike. Here are some key reasons why you might want to consider using one:
Anonymity and Privacy: By using a proxy, the server you are communicating with doesn't see your actual IP address but instead sees the proxy’s IP address.
Load Distribution and Scalability: When dealing with large-scale applications or high-traffic environments, managing incoming and outgoing requests efficiently is crucial.
By using an HTTP node proxy, you can distribute the load across multiple servers, enhancing the scalability and reliability of your system. This makes proxies essential in content delivery networks (CDNs) and large-scale web scraping projects.
Enhanced Security: Proxies can act as a first line of defense against cyber threats. By acting as an intermediary, HTTP node proxies can filter malicious traffic, block harmful requests, and prevent direct access to sensitive data.
Setting Up an HTTP Node Proxy: A Step-by-Step Guide
Now that we understand the benefits of using an HTTP node proxy, let’s walk through how to set one up using Node.js. Below is a basic guide for implementing an HTTP proxy server using the http-proxy
library, a widely-used tool for creating proxy servers in Node.js.
Step 1: Install Node.js and the HTTP-Proxy Library
First, ensure that Node.js is installed on your system. You can download it from the official Node.js website.
Once installed, create a new directory for your project, navigate into it, and install the http-proxy
package:
mkdir http-node-proxy
cd http-node-proxy
npm init -y
npm install http-proxy --save
Step 2: Create the Proxy Server
After the package is installed, create a file (e.g., proxy.js
) and write the following code to set up a basic HTTP proxy server:
const http = require('http');
const httpProxy = require('http-proxy');
// Create a proxy server
const proxy = httpProxy.createProxyServer({});
// Create an HTTP server that listens for incoming requests
const server = http.createServer((req, res) => {
console.log('Request received at: ' + req.url);
// Proxy the request to the target server
proxy.web(req, res, { target: 'http://example.com' });
});
// Listen on a specific port
server.listen(8000, () => {
console.log('Proxy server is running on http://localhost:8000');
});
In this example, the HTTP server listens on port 8000 and proxies any incoming requests to http://example.com
. You can replace this target URL with any server you'd like to proxy requests to.
Step 3: Run the Proxy Server
Run the proxy server using Node.js:
node proxy.js
Now, any requests made to http://localhost:8000
will be proxied to the target server (in this case, http://example.com
). You can test it by opening a browser and navigating to http://localhost:8000
.
Step 4: Customizing the Proxy
You can further customize the proxy by adding features like error handling, logging, and rate limiting. For example, you can add error handling to ensure the proxy doesn't crash when the target server is unreachable:
proxy.on('error', (err, req, res) => {
console.log('Proxy error:', err);
res.writeHead(500, { 'Content-Type': 'text/plain' });
res.end('Proxy server encountered an error.');
});
Best Use Cases for an HTTP Node Proxy
HTTP node proxies offer a wide array of applications for both businesses and developers. Here are some of the most effective use cases:
Web Scraping: By distributing requests across multiple IP addresses, an HTTP node proxy helps web scrapers avoid detection and rate limiting. Whether gathering competitive intelligence, monitoring price fluctuations, or aggregating user reviews, proxies are an essential tool for large-scale data collection.
API Management: For businesses relying on third-party APIs, HTTP node proxies can manage and distribute API requests more efficiently. By routing requests through a proxy, you can balance the load, prevent IP bans, and ensure high availability of your services.
Ad Verification: Digital marketers can use HTTP node proxies to verify that their online ads are being displayed correctly across various locations and platforms. By rotating proxies, businesses can monitor their ad campaigns and detect any potential fraud.
Conclusion
An HTTP node proxy is an invaluable tool for businesses and developers who need to manage large volumes of web traffic, protect their privacy, and optimize their operations. Whether you're using it for web scraping, API management, or simply to boost your network's security, the flexibility and power of HTTP node proxies make them an essential asset in today's digital environment.
By understanding how to set up and customize an HTTP node proxy, you can take full advantage of its features, helping your business stay competitive, secure, and efficient in the online world.
Subscribe to my newsletter
Read articles from Marx Lee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
