How to Automate MySQL Database Backups on Any Operating System
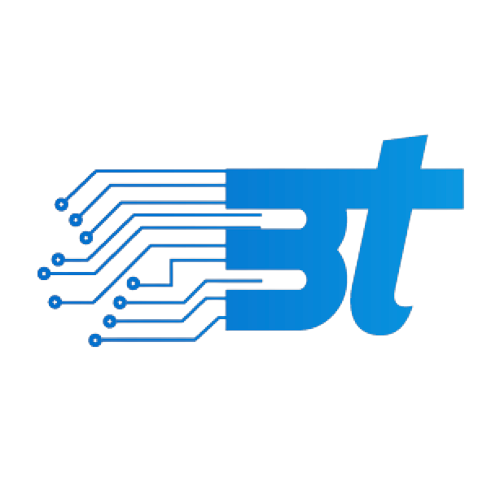
Table of contents
- Understanding MySQL Backups
- Why Automate Backups?
- Prerequisites for Backing Up MySQL Databases
- Creating a Backup Script
- Excluding Default Databases
- Structuring Backups with Timestamps
- Automating Backups with Cron Jobs
- Testing Your Backup Process
- Common Issues and Troubleshooting
- Best Practices for MySQL Backups
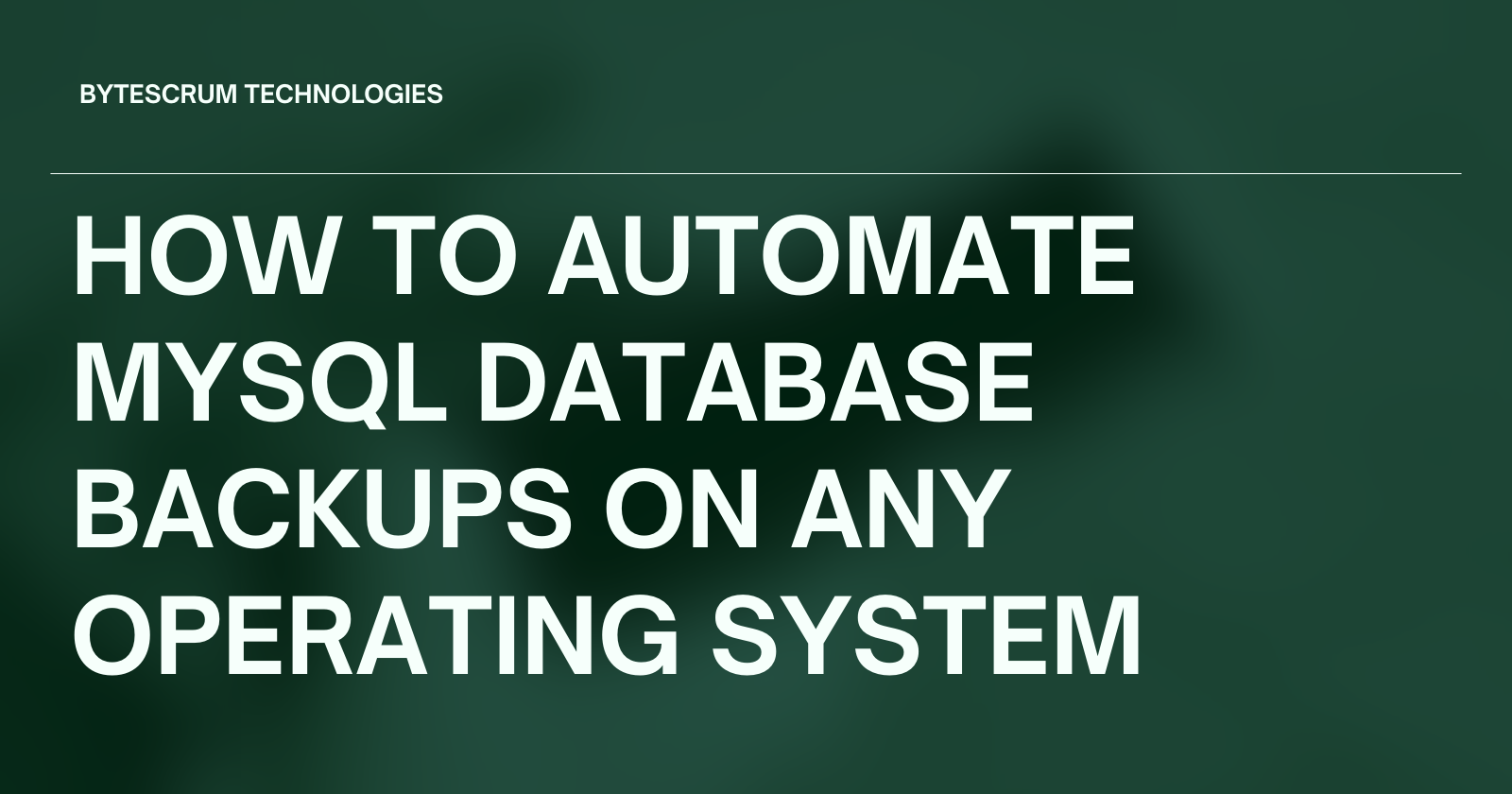
In today’s data-driven world, maintaining the integrity and availability of your data is crucial. For businesses and developers using MySQL, having a reliable backup strategy is paramount to safeguard against potential data loss due to corruption, hardware failure, or accidental deletion. This extensive guide will walk you through the process of automating MySQL database backups across various operating systems, including Windows, macOS, and Linux.
Understanding MySQL Backups
MySQL backups are crucial for ensuring data security. They allow you to restore your database to a previous state in case of data loss or corruption. There are two primary types of MySQL backups:
Logical Backups: Created using the
mysqldump
command, these backups consist of SQL statements that can recreate your database schema and data.Physical Backups: These involve copying MySQL’s data files directly from the server’s data directory, which requires more in-depth knowledge of the database structure.
For this guide, we will focus on logical backups using mysqldump
, as it is more accessible for most users and provides a robust method for backing up and restoring databases.
Why Automate Backups?
Automating your MySQL database backups offers numerous advantages:
Consistency: Automated backups ensure that your data is backed up regularly without human intervention.
Reduced Human Error: Manual backups can lead to mistakes; automation minimizes this risk.
Scheduled Backups: You can set backups to occur during off-peak hours, minimizing the impact on performance.
Peace of Mind: Knowing that your data is backed up regularly allows you to focus on other critical tasks.
Prerequisites for Backing Up MySQL Databases
Before diving into the backup process, make sure you have the following prerequisites in place:
MySQL Server: Ensure that MySQL Server is installed and running on your machine.
MySQL Client Tools: Install the MySQL client tools to access the
mysqldump
command.User Permissions: You must have a MySQL user account with sufficient privileges to perform backups, typically requiring
SELECT
access on the databases you want to back up.
Installing MySQL
On Windows:
Download the MySQL installer from the official MySQL website.
Run the installer and follow the instructions, choosing a setup type (Developer Default is recommended).
Set a root password and remember it for future use.
On macOS:
Using Homebrew, install MySQL:
brew install mysql
Start the MySQL service:
brew services start mysql
On Linux:
For Ubuntu-based systems:
sudo apt update
sudo apt install mysql-server
For CentOS:
sudo yum install mysql-server
Creating a Backup Script
To streamline the backup process, we will create a script that can be executed on any operating system. This script will dynamically locate all databases, exclude default system databases, and create structured backups with timestamps.
Dynamic Database Backup
Create a script named backup_databases.sh
(for Linux/macOS) or backup_databases.bat
(for Windows).
For Linux/macOS:
#!/bin/bash
# Configuration
USER="your_user" # MySQL username
PASSWORD="your_password" # MySQL password
OUTPUT_DIR="/path/to/your/backup/directory" # Backup directory
DATE=$(date +"%Y%m%d%H%M%S") # Current date and time
# Create output directory if it does not exist
mkdir -p "$OUTPUT_DIR"
# Get the list of databases, excluding default ones
DATABASES=$(mysql -u "$USER" -p"$PASSWORD" -e "SHOW DATABASES;" | grep -Ev "(information_schema|performance_schema|mysql|sys)")
# Backup each database
for DB in $DATABASES; do
OUTPUT_FILE="$OUTPUT_DIR/${DB}_backup_$DATE.sql"
echo "Backing up database: $DB to $OUTPUT_FILE"
mysqldump -u "$USER" -p"$PASSWORD" "$DB" > "$OUTPUT_FILE"
# Check if the backup was successful
if [ $? -eq 0 ]; then
echo "Backup of $DB completed successfully."
else
echo "Error backing up database $DB."
fi
done
echo "All backups completed."
For Windows (backup_databases.bat):
@echo off
:: Configuration
set USER=your_user :: MySQL username
set PASSWORD=your_password :: MySQL password
set OUTPUT_DIR=C:\path\to\your\backup\directory :: Backup directory
set DATE=%date:~-4,4%%date:~-10,2%%date:~-7,2%_%time:~0,2%%time:~3,2%%time:~6,2%
:: Create output directory if it does not exist
if not exist "%OUTPUT_DIR%" (
mkdir "%OUTPUT_DIR%"
)
:: Get the list of databases, excluding default ones
for /f "skip=1" %%d in ('mysql -u %USER% -p%PASSWORD% -e "SHOW DATABASES;"') do (
if /i "%%d" neq "information_schema" (
if /i "%%d" neq "performance_schema" (
if /i "%%d" neq "mysql" (
if /i "%%d" neq "sys" (
set DB=%%d
set OUTPUT_FILE=%OUTPUT_DIR%\%DB%_backup_%DATE%.sql
echo Backing up database: %%d to %OUTPUT_FILE%
mysqldump -u %USER% -p%PASSWORD% %%d > %OUTPUT_FILE%
if errorlevel 1 (
echo Error backing up database %%d.
) else (
echo Backup of %%d completed successfully.
)
)))))
)
echo All backups completed.
pause
Excluding Default Databases
In the scripts provided, we used grep -Ev
for Linux/macOS and conditional checks for Windows to filter out default databases (information_schema
, performance_schema
, mysql
, and sys
). This ensures that unnecessary backups of system databases are not created, saving time and storage space.
Structuring Backups with Timestamps
Both scripts append a timestamp to the name of each backup file. This structure helps maintain organization and allows for easy identification of different backup versions.
Automating Backups with Cron Jobs
To automate the backup process, we can use cron
on Linux/macOS or Task Scheduler on Windows.
Linux and macOS
Open the crontab for editing:
crontab -e
Add a new line to schedule the backup script to run daily at 2 AM:
0 2 * * * /path/to/your/backup_databases.sh >> /path/to/your/backup.log 2>&1
0 2 * * *
specifies that the script should run at 2:00 AM every day.>> /path/to/your/backup.log 2>&1
appends both output and errors to a log file for monitoring.
Save and exit the editor. You can verify the scheduled jobs with:
crontab -l
Windows Task Scheduler
Open Task Scheduler from the Start menu.
Select “Create Basic Task.”
Name your task (e.g., “MySQL Backup”).
Choose “Daily” for the task trigger and set the desired time (e.g., 2:00 AM).
Choose “Start a Program” for the action.
Browse and select your
backup_databases.bat
script.Finish and save the task.
Testing Your Backup Process
Before relying on automated backups, it’s essential to test your script manually to ensure it works as intended.
Run the script manually (both on Windows and Linux/macOS) to see if it executes without errors.
Check the output directory to verify that backup files are created.
Test restoring a backup by using the
mysql
command to import the backup file:mysql -u your_user -p your_database < /path/to/your/backup/file.sql
Common Issues and Troubleshooting
Access Denied Errors
You may encounter an error like:
ERROR 1045 (28000): Access denied for user 'username'@'localhost' (using password: YES)
This error indicates that the MySQL user credentials provided in the script are incorrect. Ensure that:
The username and password are correct.
The user has the necessary permissions to access the databases.
To resolve this, you can set up a login path using mysql_config_editor
:
mysql_config_editor set --login-path=local --host=localhost --user=matchcare --password
Permission Denied Errors
If you receive a “permission denied” error, ensure that the backup script has executable permissions:
chmod +x /path/to/your/backup_databases.sh
For Windows, ensure that the user account running the task has permission to access the script and backup directory.
MySQL Connection Errors
Make sure that:
MySQL server is running.
You can manually connect to MySQL using the provided credentials:
mysql -u your_user -p
Best Practices for MySQL Backups
Regular Testing: Regularly test your backup and restore processes to ensure they function correctly.
Storage Management: Monitor your backup directory for disk space. Implement a retention policy to delete old backups.
Offsite Backups: Consider storing backups offsite or in the cloud for additional redundancy.
Security: Ensure that backup files are stored securely, especially if they contain sensitive data.
Documentation: Document your backup strategy and procedures to ensure clarity among team members.
Conclusion
Implementing these practices not only enhances your data management strategy but also provides peace of mind, knowing that you can quickly recover your data in the event of an emergency. Regularly review and refine your backup processes to adapt to your changing needs.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
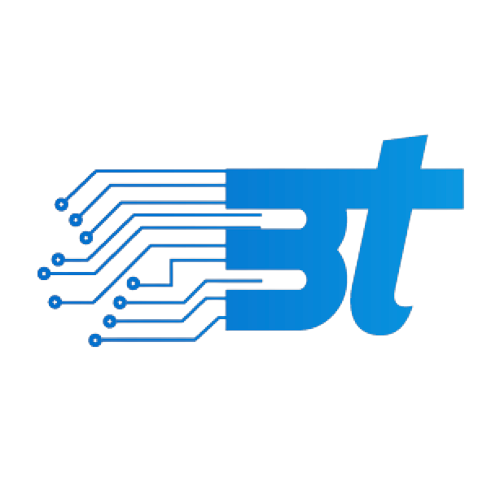
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.