Introduction to Python Flask Framework
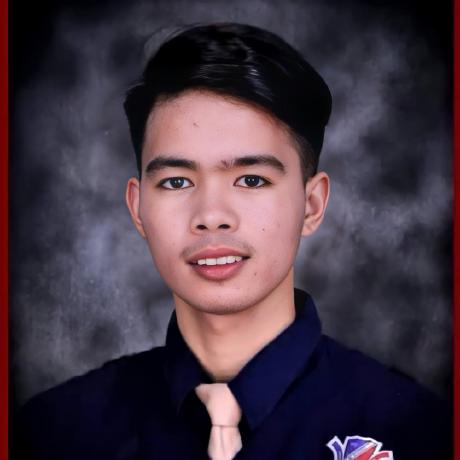
Flask is a lightweight, micro web framework written in Python. It’s designed to be simple and easy to use, making it perfect for building small to medium web applications. Unlike larger frameworks like Django, Flask doesn’t come with many built-in features but gives developers the flexibility to add only what they need.
Key Features of Flask:
Lightweight: Flask is minimalistic, allowing you to create simple web applications quickly.
Modular: You can add only the components you need, making it flexible and customizable.
Built-in Development Server: Flask includes a server for testing and development.
Great for Beginners: Flask is easy to learn and a great starting point for learning web development.
2. Installing Flask
Before using Flask, you need to install it. You can install Flask using the Python package manager pip.
In your terminal or command prompt, type:
pip install Flask
Once Flask is installed, you are ready to create your first Flask application.
3. Creating Your First Flask Application
Let’s start by building a simple Flask application that displays a "Hello, World!" message when you visit a webpage.
Basic Flask Code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return "Hello, World!"
if __name__ == '__main__':
app.run()
Code Explanation:
from flask import Flask
: Imports the Flask class from the Flask library.app = Flask(__name__)
: Creates an instance of the Flask class. This object will be the web application.@app.route('/')
: Defines a route (or URL). The"/"
route is the homepage of the web app.def hello_world()
: This function runs when someone visits the route"/"
. It returns the text "Hello, World!" to the browser.app.run()
: Starts the Flask development server.
Running the Application:
Save the file as
app.py
.Run the file using the command:
python app.py
In your browser, go to
http://127.0.0.1:5000/
. You should see "Hello, World!" displayed.
4. Flask Routing
Flask uses routing to map URLs to Python functions. You can define multiple routes, each associated with a different function.
Example of Multiple Routes:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to the Home Page!"
@app.route('/about')
def about():
return "This is the About Page."
if __name__ == '__main__':
app.run()
Code Explanation:
@app.route('/')
: The homepage route.@app.route('/about')
: The/about
route will show a message when you visithttp://127.0.0.1:5000/about
.
You can create routes for different parts of the website, like /contact
, /profile
, etc.
5. Dynamic URL Routing
Flask allows you to create dynamic routes, where part of the URL can change. This is useful for things like user profiles or blog posts, where you might want to show different data based on the URL.
Example of Dynamic Routes:
from flask import Flask
app = Flask(__name__)
@app.route('/user/<name>')
def user(name):
return f"Hello, {name}!"
if __name__ == '__main__':
app.run()
Code Explanation:
@app.route('/user/<name>')
: The<name>
in the route is a placeholder for a dynamic value.return f"Hello, {name}!"
: Thef"{}"
syntax is used to insert the value ofname
into the string.
When you visit http://127.0.0.1:5000/user/Alice
, it will display "Hello, Alice!". You can replace "Alice" with any name in the URL.
6. Handling HTTP Methods
Flask supports different HTTP methods, such as GET and POST. By default, routes use the GET
method, which is used to retrieve data from the server. The POST
method is often used to send data to the server, such as form submissions.
GET and POST Example:
from flask import Flask, request
app = Flask(__name__)
@app.route('/greet', methods=['GET', 'POST'])
def greet():
if request.method == 'POST':
name = request.form['name']
return f"Hello, {name}!"
return '''
<form method="post">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
'''
if __name__ == '__main__':
app.run()
Code Explanation:
methods=['GET', 'POST']
: Specifies that the/greet
route can handle both GET and POST requests.request.method
: Checks if the request is aPOST
orGET
.request.form['name']
: Retrieves the value of thename
field from the form.
How It Works:
When you visit
/greet
in your browser, you will see a form asking for your name.After entering your name and submitting the form, the server will respond with a personalized greeting using the POST method.
7. Templates in Flask
In most web applications, you will want to separate the HTML code from your Python code. Flask allows you to use templates to do this. Templates are HTML files that can be dynamically generated with data from your Flask app.
Using Templates Example:
Create a folder called
templates
in your project directory.Inside the
templates
folder, create a file calledhello.html
with the following content:<!DOCTYPE html> <html> <body> <h1>Hello, {{ name }}!</h1> </body> </html>
Modify your Flask app to use this template:
from flask import Flask, render_template app = Flask(__name__) @app.route('/user/<name>') def user(name): return render_template('hello.html', name=name) if __name__ == '__main__': app.run()
Code Explanation:
render_template('hello.html', name=name)
: Renders thehello.html
template and passes thename
variable to the template.{{ name }}
: This is Jinja2 template syntax, which allows you to insert dynamic content into your HTML file.
Now, when you visit http://127.0.0.1:5000/user/Alice
, the browser will display an HTML page with "Hello, Alice!".
8. Flask Forms and User Input
Flask allows handling forms easily using Flask-WTF, a form-handling extension. For now, let's keep it simple by handling forms without external libraries.
In the previous example, we already demonstrated a basic form. Let’s expand on that by processing form input to create a personalized user experience.
Example of Handling Forms:
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route('/submit', methods=['GET', 'POST'])
def submit():
if request.method == 'POST':
name = request.form['name']
return render_template('hello.html', name=name)
return '''
<form method="post">
Name: <input type="text" name="name">
<input type="submit" value="Submit">
</form>
'''
if __name__ == '__main__':
app.run()
Here, the user submits a name through a form, and the server processes it to render the template with a personalized greeting.
Flask is a simple and flexible framework that allows you to build anything from simple web pages to complex applications. Its modular nature allows you to start with small, simple projects and expand as you learn more.
References:
Grinberg, Miguel. “Flask Web Development.” Flask Documentation, https://flask.palletsprojects.com/
"Flask: A Simple Framework for Building Complex Web Applications." RealPython, https://realpython.com/flask-by-example/
Subscribe to my newsletter
Read articles from Thirdy Gayares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
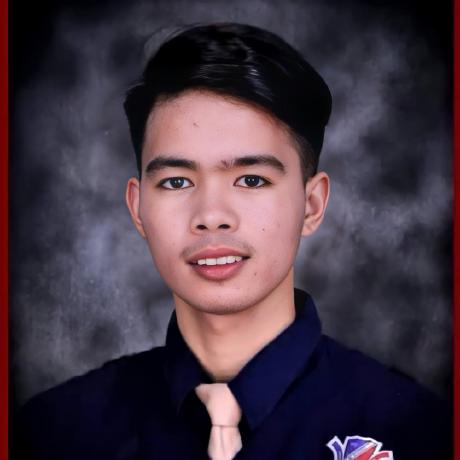
Thirdy Gayares
Thirdy Gayares
I am a dedicated and skilled Software Engineer specializing in mobile app development, backend systems, and creating secure APIs. With extensive experience in both SQL and NoSQL databases, I have a proven track record of delivering robust and scalable solutions. Key Expertise: Mobile App Development: I make high-quality apps for Android and iOS, ensuring they are easy to use and work well. Backend Development: Skilled in designing and implementing backend systems using various frameworks and languages to support web and mobile applications. Secure API Creation: Expertise in creating secure APIs, ensuring data integrity and protection across platforms. Database Management: Experienced with SQL databases such as MySQL, and NoSQL databases like Firebase, managing data effectively and efficiently. Technical Skills: Programming Languages: Java, Dart, Python, JavaScript, Kotlin, PHP Frameworks: Angular, CodeIgniter, Flutter, Flask, Django Database Systems: MySQL, Firebase Cloud Platforms: AWS, Google Cloud Console I love learning new things and taking on new challenges. I am always eager to work on projects that make a difference.