Database Management & Optimization in PHP and Laravel

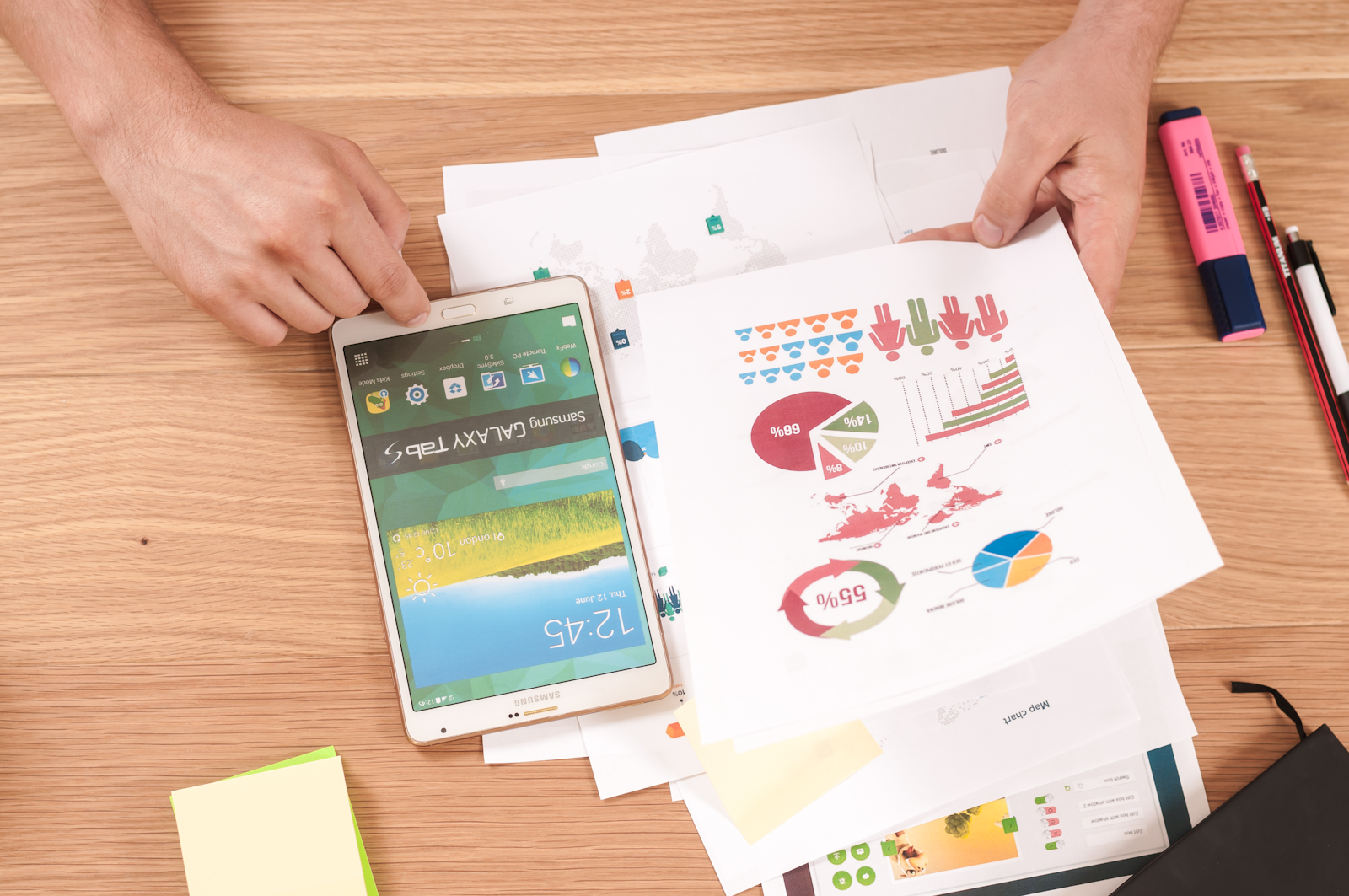
Managing and optimizing databases is crucial in web development, especially when working with large datasets and high-traffic applications. This involves a range of skills from writing efficient SQL queries to using ORM (Object-Relational Mapping) tools effectively and optimizing database transactions.
Advanced SQL
Master Complex Queries
Scenario: Suppose you have an e-commerce site and want to generate a report of all customers who have made more than 5 purchases in the last year.
SQL Example:
SELECT customers.name, COUNT(orders.id) AS total_orders
FROM customers
JOIN orders ON customers.id = orders.customer_id
WHERE orders.created_at >= NOW() - INTERVAL 1 YEAR
GROUP BY customers.name
HAVING total_orders > 5
ORDER BY total_orders DESC;
Indexing
Scenario: Your application is slow when filtering products by category or price range.
Solution: Create an index on the
category_id
andprice
columns in theproducts
table.SQL Example:
CREATE INDEX idx_category_price ON products(category_id, price);
Views
Scenario: You need to frequently fetch reports of monthly sales by product. Instead of repeating complex queries, you can create a view.
SQL Example:
CREATE VIEW monthly_sales AS
SELECT product_id, SUM(total_amount) AS total_sales, MONTH(created_at) AS sales_month
FROM orders
GROUP BY product_id, sales_month;
Stored Procedures
Scenario: You have a complex task like recalculating the discount for all products every night, which should be automated.
SQL Example:
DELIMITER //
CREATE PROCEDURE recalculate_discounts()
BEGIN
UPDATE products SET discount = price * 0.1 WHERE stock > 100;
END//
DELIMITER ;
Database Optimization Techniques
Scenario: Your e-commerce application is slow due to large datasets.
Optimization Techniques:
Optimize Queries: Use
EXPLAIN
to analyze slow queries and add missing indexes.Use Joins Efficiently: Prefer
INNER JOIN
when you know that both tables will have matching data.Pagination: Use database-level pagination to avoid fetching unnecessary data, especially in large datasets.
SQL Example (with EXPLAIN):
EXPLAIN SELECT * FROM orders WHERE customer_id = 1;
ORM Usage & Optimization
Advanced Use of ORM (Eloquent in Laravel)
Scenario: You're working on a social media platform, and you need to retrieve a user's posts, comments, and likes in a single query using relationships.
Laravel Eloquent Example:
$user = User::with(['posts', 'comments', 'likes'])->find($userId);
Query Optimization Techniques
Scenario: Fetching a user's posts with comments leads to multiple queries (N+1 problem).
Solution: Use eager loading to load related models in a single query.
Example:
// Before: N+1 problem
$posts = Post::all();
foreach ($posts as $post) {
echo $post->comments->count(); // Executes a new query for each post
}
// After: Eager Loading
$posts = Post::with('comments')->get();
foreach ($posts as $post) {
echo $post->comments->count(); // Only two queries
}
Optimizing Database Transactions
Scenario: You need to perform multiple database operations that should either all succeed or all fail. Example: deducting inventory and placing an order.
Laravel Example (Using Transactions):
DB::transaction(function () use ($orderData) {
$order = Order::create($orderData);
foreach ($order->items as $item) {
Product::where('id', $item->product_id)
->decrement('stock', $item->quantity);
}
});
Handling Large Datasets Efficiently
Scenario: You need to process millions of records from the
orders
table for a report.Solution: Use chunking to avoid memory overload.
Laravel Example:
Order::chunk(1000, function ($orders) {
foreach ($orders as $order) {
// Process each order
}
});
Database Design
Advanced Database Design & Normalization
Scenario: You are designing a database for a multi-tenant application (where multiple companies can use the same database) and need to ensure data integrity and avoid duplication.
Normalization: Split data into related tables to reduce redundancy.
- Example: Instead of storing
customer_address
in theorders
table, create a separateaddresses
table and relate it tocustomers
.
- Example: Instead of storing
CREATE TABLE addresses (
id INT AUTO_INCREMENT PRIMARY KEY,
customer_id INT,
address VARCHAR(255),
city VARCHAR(50),
FOREIGN KEY (customer_id) REFERENCES customers(id)
);
Handling Complex Relationships
Scenario: A project management application where tasks can have multiple tags and users can be assigned to multiple tasks (many-to-many relationships).
Database Design:
Tables:
tasks (id, name)
tags (id, name)
task_tag (task_id, tag_id)
user_task (user_id, task_id)
CREATE TABLE task_tag ( task_id INT, tag_id INT, PRIMARY KEY (task_id, tag_id), FOREIGN KEY (task_id) REFERENCES tasks(id), FOREIGN KEY (tag_id) REFERENCES tags(id) ); CREATE TABLE user_task ( user_id INT, task_id INT, PRIMARY KEY (user_id, task_id), FOREIGN KEY (user_id) REFERENCES users(id), FOREIGN KEY (task_id) REFERENCES tasks(id) );
In Laravel: This many-to-many relationship can be defined using Eloquent.
class Task extends Model { public function tags() { return $this->belongsToMany(Tag::class); } }
Example with Laravel Eloquent:
$tasks = Task::with('tags')->get(); foreach ($tasks as $task) { echo $task->name . ' has tags: '; foreach ($task->tags as $tag) { echo $tag->name . ', '; } }
Summary
Database Management & Optimization in PHP/Laravel requires mastering both SQL and ORM techniques to write efficient queries, manage large datasets, and design scalable databases.
Real-life scenarios include handling large e-commerce datasets, optimizing complex queries in social media platforms, and designing multi-tenant applications with normalized databases.
Subscribe to my newsletter
Read articles from Dale Lanto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dale Lanto
Dale Lanto
A passionate Full Stack and Backend Web Developer with 7+ years of experience, specializing in PHP, Laravel, and a range of modern web technologies. I enjoy solving complex problems, optimizing systems, and delivering efficient, maintainable code. Check out some of my work at dalelanto.netlify.app or explore my GitHub at github.com/dalelantowork.