Building a Java File Explorer: A Personal Project Journey

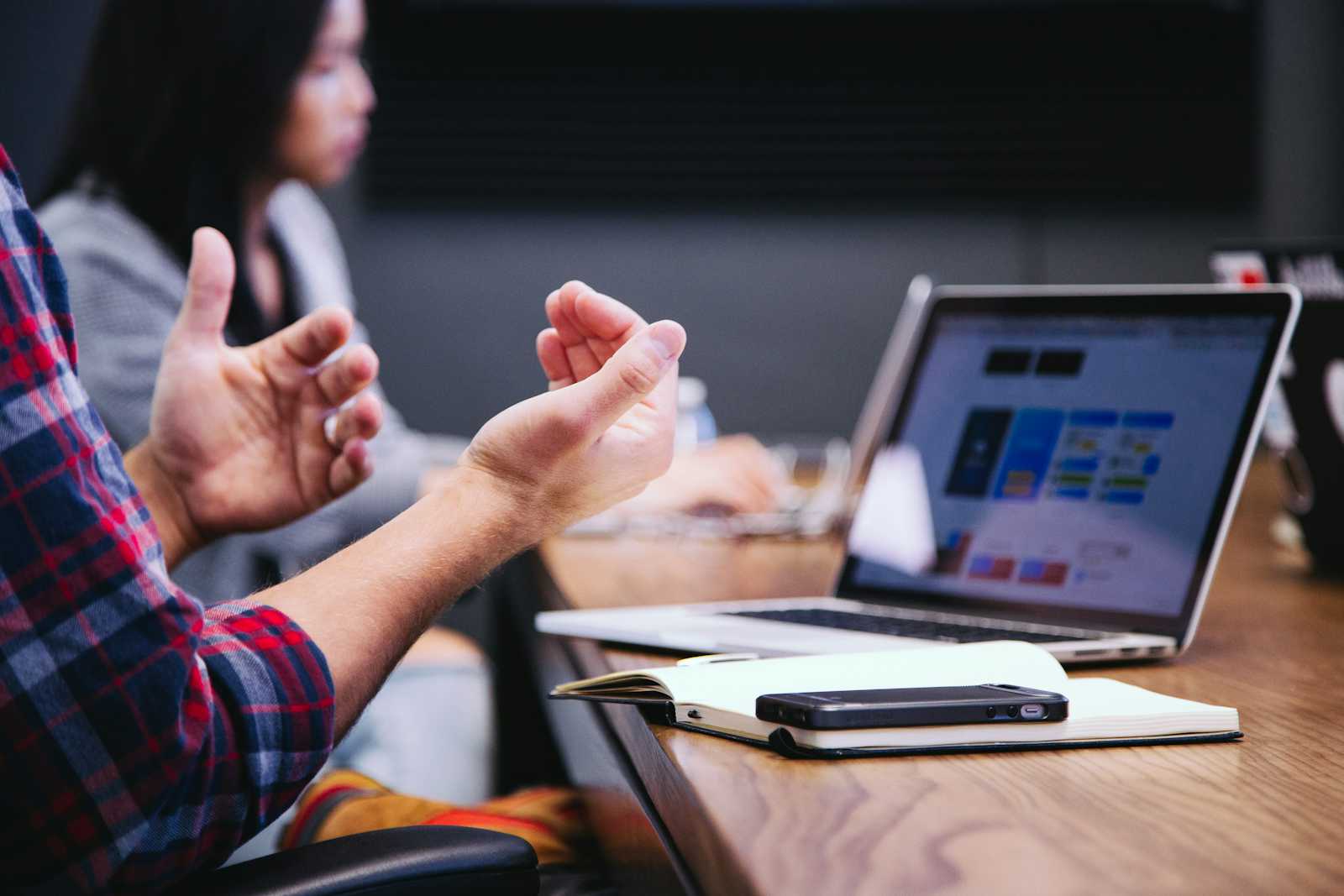
Introduction
In this project, I developed a fully functional file explorer application using Java, simulating the core features found in traditional file management systems like Windows Explorer. This article details the design, development process, and challenges faced while creating this application.
Project Overview
The file explorer application features a user-friendly graphical interface that allows users to navigate their file systems easily. Key functionalities implemented include:
Navigation: Users can view and navigate through directories.
File Operations:
Basic file operations such as open, rename, copy, move, delete.
An "Open With" feature to launch files with associated applications.
Context Menu: Right-click context menus provide quick access to file operations.
Status Bar: Displays the current path and file information, enhancing user experience.
Technical Implementation
The application was built using Java Swing for the GUI, with a structured folder organization that includes:
UI Components: The main UI and context menu classes handle the graphical elements.
File Operations: Dedicated classes for handling various file operations ensure modularity and ease of maintenance.
Data Structures: Utilized collections like ArrayList and DefaultListModel to manage and display file information.
Code Snippet Example
// code snippet for file operation
public void deleteFile(File file) {
if (file.delete()) {
System.out.println("File deleted successfully");
} else {
System.out.println("Failed to delete the file");
}
}
// Method to open a file using the Desktop class
public void openFile(File file) {
if (file.exists()) {
try {
Desktop.getDesktop().open(file);
System.out.println("File opened successfully: " + file.getName());
} catch (IOException e) {
System.out.println("Error opening the file: " + e.getMessage());
}
} else {
System.out.println("File does not exist: " + file.getName());
}
}
Enhancements
To improve the overall look and feel, I applied a modern UI design:
Icons: Implemented file and folder icons using FileSystemView to make the UI more intuitive.
Look and Feel: Set the application to use Nimbus for a polished appearance.
Toolbars and Buttons: Added a toolbar for common actions and improved layout for better usability.
Real-World Applications
A file explorer application has several practical applications, including:
Personal File Management: Helps users manage and organize their personal files effectively.
Software Development Tools: Assists developers in navigating project directories, managing source files, and version control.
Educational Tools: Can be used in educational environments to teach students about file systems and basic programming concepts.
Data Analysis Applications: Facilitates easy access to data files and logs for analysis in data science projects.
Challenges and Solutions
Throughout the development process, I encountered several challenges:
File Path Handling: Ensuring the application correctly navigates and displays files across various operating systems.
Performance: Loading directories with a large number of files required optimization.
Solutions Implemented
To overcome these issues, I utilized effective exception handling, implemented multi-threading for file loading, and conducted thorough testing to ensure reliability across different scenarios.
Conclusion
This file explorer project not only enhanced my programming skills but also deepened my understanding of GUI development in Java. I’ve shared the complete code on GitHub for anyone interested in exploring or contributing to the project.
Subscribe to my newsletter
Read articles from Pranay Bhatkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranay Bhatkar
Pranay Bhatkar
I’m a programmer passionate about software development and technology. I love sharing my projects and insights on coding, and I’m always eager to connect with fellow tech enthusiasts. Currently available for freelance work. Let’s collaborate!