Programming Paradigms 101
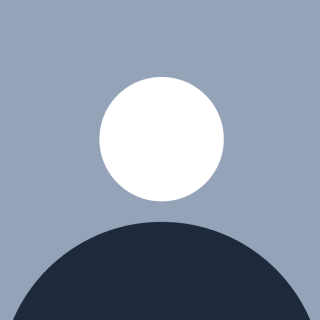
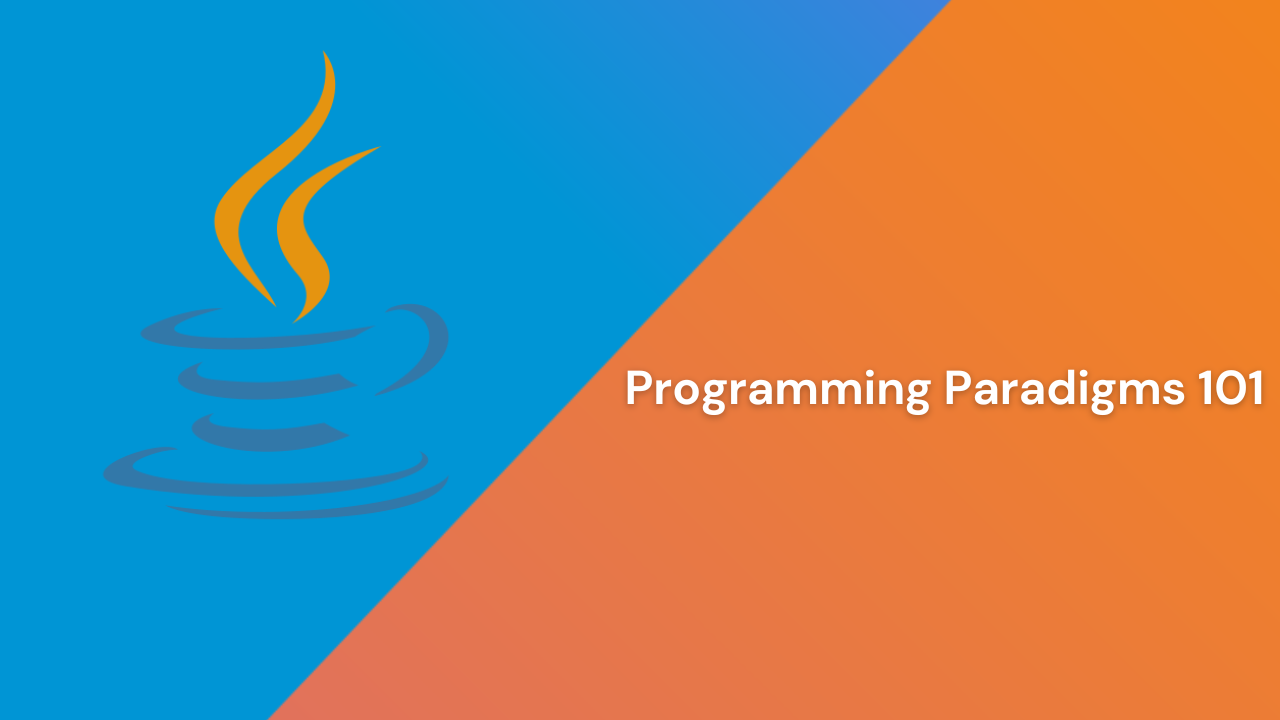
In the world of software development, programming paradigms are essential as they provide a framework for developers to solve problems and structure their code. Each paradigm has its own set of principles and concepts that guide the way programs are written and maintained. we will explore some of the most common programming paradigms and their characteristics.
1. Procedural Programming
Procedural programming is one of the oldest and most straightforward paradigms. It is based on the concept of procedure calls, where a program is composed of a sequence of instructions that are executed in order.
Key Concepts:
Procedures (or functions):
- Procedures, also known as functions or subroutines, are blocks of code designed to perform a specific task. They can be called multiple times within a program, promoting code reuse and reducing redundancy. Each procedure can take inputs, process them, and return outputs.
Sequential execution:
In procedural programming, the flow of the program is determined by the sequence of instructions. The program starts executing from the beginning and follows the instructions one by one until it reaches the end. This linear flow makes it easier to understand and debug the code.
Modularity:
Modularity refers to the division of a program into smaller, self-contained units or modules. Each module represents a specific functionality and can be developed, tested, and maintained independently. This approach enhances code organization, readability, and maintainability.
Languages:
C is a powerful and widely-used procedural programming language. It provides low-level access to memory and system resources, making it suitable for system programming, embedded systems, and performance-critical applications.
Pascal is a high-level procedural programming language designed for teaching programming concepts and structured programming. It emphasizes readability and ease of use, making it a popular choice for educational purposes.
Fortran (Formula Translation) is one of the oldest high-level programming languages, primarily used for scientific and engineering applications. It excels in numerical computation and array manipulation, making it ideal for complex mathematical calculations and simulations.
2. Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a programming paradigm that uses "objects" to design applications and computer programs. It leverages several key concepts to promote code reusability, scalability, and efficiency. Here is a detailed explanation of the key concepts and languages associated with OOP:
Key Concepts:
Classes and Objects:
Classes: A class is a blueprint for creating objects. It defines a datatype by bundling data and methods that work on the data into one single unit. For example, a class
Car
might include attributes likecolor
,model
, andyear
, and methods likedrive()
andbrake()
.Objects: An object is an instance of a class. When a class is defined, no memory is allocated until an object of that class is created. For example,
myCar
could be an object of the classCar
, with specific values forcolor
,model
, andyear
.
Encapsulation:
Encapsulation is the concept of wrapping data (variables) and code (methods) together as a single unit. It restricts direct access to some of an object's components, which can prevent the accidental modification of data. This is typically achieved using access modifiers like
private
,protected
, andpublic
.For example, in a
Car
class, thespeed
attribute might be private, and access to it is controlled through public methods likegetSpeed()
andsetSpeed()
.
Inheritance:
Inheritance is a mechanism where a new class inherits properties and behavior (methods) from an existing class. The existing class is called the "base" or "parent" class, and the new class is called the "derived" or "child" class.
For example, if you have a
Vehicle
class, aCar
class can inherit from it, gaining all its attributes and methods, while also adding new features or modifying existing ones.
Polymorphism:
Polymorphism allows methods to do different things based on the object it is acting upon, even though they share the same name. It can be achieved through method overriding (inherited methods are redefined in the child class) and method overloading (same method name with different parameters).
For example, a method
draw()
might behave differently in classesCircle
,Square
, andTriangle
, even though the method name is the same.
Languages:
Java is a widely-used OOP language known for its portability across platforms, thanks to the Java Virtual Machine (JVM). It emphasizes simplicity, object-oriented principles, and robustness. Java is used in web applications, enterprise software, and Android app development.
C++ is an extension of the C programming language with OOP features. It provides low-level memory manipulation capabilities along with high-level abstractions. C++ is used in system/software development, game development, and real-time simulation.
Python is a high-level, interpreted language known for its readability and simplicity. It supports multiple programming paradigms, including OOP. Python is widely used in web development, data science, artificial intelligence, and scripting.
3. Functional Programming
Functional programming treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the use of functions and immutability.
Key Concepts:
Pure Functions:
- Pure functions are functions where the output value is determined only by its input values, without observable side effects. This means that given the same input, a pure function will always return the same output. Pure functions do not modify any state or data outside their scope, making them predictable and easier to test.
Immutability:
- Immutability refers to the concept that data cannot be changed once it is created. Instead of modifying existing data, new data structures are created. This approach helps in avoiding side effects and makes the code more predictable and easier to reason about. In functional programming, variables are often immutable, meaning their values cannot be altered after they are set.
First-Class Functions:
- First-class functions are functions that are treated as first-class citizens. This means they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables. This property allows for higher-order functions and functional composition, enabling more abstract and flexible code.
Higher-Order Functions:
- Higher-order functions are functions that can take other functions as arguments and/or return functions as their result. This allows for powerful abstractions and code reuse. Examples of higher-order functions include
map
,filter
, andreduce
, which are commonly used to process collections of data.
- Higher-order functions are functions that can take other functions as arguments and/or return functions as their result. This allows for powerful abstractions and code reuse. Examples of higher-order functions include
Languages:
Haskell is a purely functional programming language known for its strong static typing, lazy evaluation, and emphasis on immutability. It is often used in academia and industry for research, teaching, and developing complex software systems.
Lisp (LISt Processing) is one of the oldest programming languages and has a strong emphasis on symbolic computation and recursion. It supports functional programming paradigms and is known for its powerful macro system, which allows for code transformation and metaprogramming.
Scala is a hybrid functional and object-oriented programming language that runs on the Java Virtual Machine (JVM). It combines the best features of both paradigms, offering a concise syntax, powerful type system, and support for immutability and higher-order functions. Scala is widely used in data processing, distributed computing, and web development.
4. Logic Programming
Logic programming is based on formal logic, specifically predicate logic, and is used to express facts and rules about a problem domain. It is a declarative programming paradigm, meaning that it focuses on what the program should accomplish rather than how to accomplish it. Here is a detailed explanation of the key concepts and languages associated with logic programming:
Key Concepts:
Facts:
Facts are basic assertions about some world or domain. They are used to represent known information. In logic programming, facts are typically written as simple statements or predicates.
For example, in a family domain, a fact might be
parent(john, mary).
which asserts that John is a parent of Mary.
Rules:
Rules are logical statements that define relationships between facts. They are used to infer new information from known facts. A rule consists of a head and a body, where the head is a conclusion that can be drawn if the body (a series of conditions) is true.
For example, a rule might be
grandparent(X, Z) :- parent(X, Y), parent(Y, Z).
which states that X is a grandparent of Z if X is a parent of Y and Y is a parent of Z.
Queries:
Queries are questions asked about the information stored in the form of facts and rules. They are used to retrieve information or check the truth of certain statements.
For example, a query might be
?- parent(john, mary).
which asks whether John is a parent of Mary. The system will respond withtrue
orfalse
based on the facts and rules defined.
Languages:
Prolog (Programming in Logic) is the most well-known logic programming language. It is used in artificial intelligence, natural language processing, and computational linguistics. Prolog programs consist of a series of facts, rules, and queries.
Example:
parent(john, mary). parent(mary, alice). grandparent(X, Z) :- parent(X, Y), parent(Y, Z).
Datalog is a subset of Prolog, designed specifically for database queries. It is used in deductive databases and data integration systems. Datalog does not support complex terms or functions, making it simpler and more efficient for certain applications.
Example:
parent(john, mary). parent(mary, alice). grandparent(X, Z) :- parent(X, Y), parent(Y, Z).
5. Event-Driven Programming
Event-driven programming is a paradigm in which the flow of the program is determined by events such as user actions, sensor outputs, or message passing. This approach is particularly useful for applications that require a high level of interactivity or need to respond to asynchronous events.
Key Concepts:
Event Handlers: Event handlers are functions or methods that are triggered in response to specific events. These events can be user actions like clicks, key presses, or mouse movements, as well as system-generated events like timers or network responses. Event handlers are registered with specific events, and when the event occurs, the corresponding handler is executed.
Example: In JavaScript, you might attach an event handler to a button click like this:
document.getElementById('myButton').addEventListener('click', function() { alert('Button was clicked!'); });
Event Loops: An event loop is a programming construct that waits for and dispatches events or messages in a program. It continuously checks for new events and processes them as they arrive. The event loop is essential for managing asynchronous operations and ensuring that the program remains responsive.
Example: In JavaScript, the event loop is a fundamental part of the runtime environment, handling events like I/O operations, timers, and user interactions.
Callbacks: Callbacks are functions that are passed as arguments to other functions and are executed after some operation has been completed. They are commonly used in event-driven programming to handle asynchronous events. When an event occurs, the callback function is invoked to handle the event.
Example: In JavaScript, you might use a callback to handle the response from an asynchronous HTTP request:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { console.log(data); });
Languages:
JavaScript is one of the most popular languages for event-driven programming, especially in web development. It is designed to handle events like user interactions, network requests, and timers efficiently. JavaScript's event-driven nature makes it ideal for creating dynamic and interactive web applications.
C# supports event-driven programming through its event and delegate mechanisms. It is commonly used in Windows applications, game development with Unity, and web applications with ASP.NET. C# provides robust support for handling events, making it a powerful language for building responsive applications.
Visual Basic (VB) is another language that supports event-driven programming, particularly in the context of Windows applications. VB makes it easy to create event-driven applications with its straightforward syntax and integrated development environment (IDE). It is often used for developing desktop applications with graphical user interfaces (GUIs).
6. Concurrent Programming
Concurrent programming is concerned with the execution of multiple sequences of operations simultaneously. It is essential for developing applications that perform multiple tasks at the same time.
Key Concepts:
Threads:
Threads are the smallest unit of processing that can be scheduled by an operating system. They allow multiple sequences of instructions to run concurrently within the same program. Each thread operates independently but shares the same memory space, which allows for efficient communication and data sharing.
Threads can be created and managed using various programming constructs and libraries. For example, in Java, the
Thread
class andRunnable
interface are used to create and manage threads. In Go, goroutines are lightweight threads managed by the Go runtime.Proper management of threads is crucial to avoid issues like race conditions, where multiple threads access shared resources simultaneously, leading to unpredictable results.
Synchronization:
Synchronization is the coordination of concurrent threads to ensure that they do not interfere with each other while accessing shared resources. It is essential to prevent race conditions and ensure data consistency.
Common synchronization mechanisms include locks, semaphores, and monitors. These constructs allow threads to acquire exclusive access to shared resources, ensuring that only one thread can modify the resource at a time.
For example, in Java, the
synchronized
keyword can be used to lock methods or code blocks, ensuring that only one thread can execute the synchronized code at a time. In Go, channels can be used to synchronize goroutines by passing messages between them.
Parallelism:
Parallelism involves dividing a task into smaller sub-tasks that can be executed simultaneously on multiple processors or cores. It aims to improve the performance and efficiency of applications by leveraging the capabilities of modern multi-core processors.
Parallelism can be achieved through various techniques, such as data parallelism, where the same operation is performed on different pieces of data simultaneously, and task parallelism, where different tasks are executed concurrently.
In languages like Java, the
ForkJoinPool
class provides a framework for parallel execution of tasks. In Go, thesync
package offers primitives for managing parallel execution.
Languages:
Java provides robust support for concurrent programming through its
java.util.concurrent
package, which includes classes likeThread
,ExecutorService
, andForkJoinPool
. Java's concurrency model is based on threads, and it offers various synchronization mechanisms like locks and semaphores.- Java's concurrency utilities make it easier to manage threads, handle synchronization, and implement parallelism, making it a popular choice for developing multi-threaded applications.
Go, also known as Golang, is designed with concurrency in mind. It provides lightweight threads called goroutines, which are managed by the Go runtime. Goroutines are more efficient than traditional threads, allowing for the creation of thousands of concurrent tasks with minimal overhead.
- Go also offers channels for communication and synchronization between goroutines. Channels provide a safe way to pass data between goroutines, ensuring that data is shared without race conditions.
Erlang is a functional programming language designed for building concurrent and distributed systems. It uses lightweight processes, which are similar to threads but managed by the Erlang runtime system. These processes are isolated and communicate through message passing, making them highly efficient and fault-tolerant.
- Erlang's concurrency model is based on the Actor model, where each process is an actor that can send and receive messages. This model simplifies the development of concurrent applications and ensures that processes do not interfere with each other.
Conclusion
Choosing the best programming paradigm depends on the problem you are trying to solve. Each paradigm has its strengths and weaknesses, and understanding them can help you select the most appropriate one for your project. In the software industry, developers often need to be proficient in multiple paradigms to tackle various challenges effectively.
Procedural Programming: Uses procedures, sequential execution, and modularity. Languages: C, Pascal, Fortran.
Object-Oriented Programming (OOP): Utilizes classes, objects, encapsulation, inheritance, and polymorphism. Languages: Java, C++, Python.
Functional Programming: Emphasizes pure functions, immutability, first-class functions, and higher-order functions. Languages: Haskell, Lisp, Scala.
Logic Programming: Based on facts, rules, and queries. Languages: Prolog, Datalog.
Event-Driven Programming: Driven by events, event handlers, event loops, and callbacks. Languages: JavaScript, C#, Visual Basic.
Concurrent Programming: Focuses on threads, synchronization, and parallelism. Languages: Java, Go, Erlang.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer