Python Strings Explained: Day 7 of Your Coding Journey

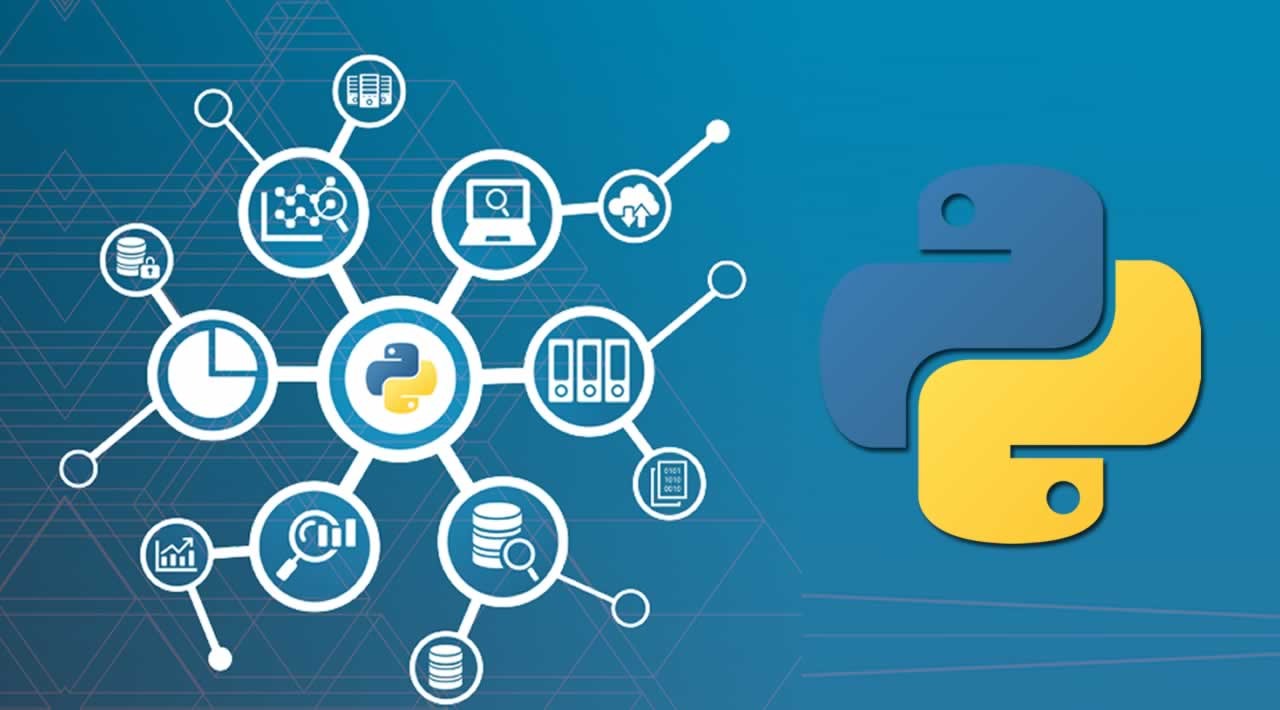
Introduction:
Welcome to Day 7 of my Python journey!
Today, I ventured into the realm of String. These concepts are essential.
String Data Types :
(str → string)
. str is a predefined class in python.
. to get the manual of str class,
>>> help ( str )
. how to create object of str class,
obj = str ()
print ( obj , type ( obj ))
obj = str ( "india" )
print ( obj , type ( obj ))
obj = "ithon"
print ( obj , type ( obj ))
obj = 'a'
print ( obj , type ( obj ))
obj = 'abc'
print ( obj , type ( obj ))
obj = '''this is an example of multiline string'''
print ( obj , type ( obj ) )
obj = """welcome to the python class """
print ( obj , type ( obj ))
. Any sequence of characters within either single quotes or double quotes is considered as a String.
. python does not support char data type.
. in python char is also represented as str object.
. tripple quote is used for multiline string.
. anonymus string object is used for comment line also.
. the object having no name is known as anonymus object.
. to get the length of a string object we can apply len () function.
. str object does not support item assignemnt or item deletion.
. we can not modify string object in future.
. string object is immutable object in python.
INDEXING :
. to target a specific char in a string object we can apply index mechanism.
. by using index we can perfrom read operation with string object
. by using index we can not perfrom write / update / delete operation with string object.
. to provide index with string object we have to use subscript operator
strObj [ index ]
. +ve index range 0 to len - 1
. -ve index supported by str object.
. -ve index range -len to -1
OPERATORS
all the operator available in python is not going to supported with str object
. arithmatic
+ concatination
* repetation
. relational
relational operator in a string object works according to ASCI value.
. membership
in
not in
return bool type object ( True / False )
is
is not
s1 = "Hello Sir"
s2 = "Hello Sir"
print ("sir" in s1 )
print ("H" in s1 )
print ("h" in s1 )
print (" " in s1 )
print (s1 is s2)
s3 = "Hello sir"
print (s1 is s3 )
Example:
arithmatic
obj1 = 'hello '
obj2 = 'sir'
obj = obj1 + obj2
print ( obj )
#obj = obj1 + 10 #TypeError
obj = obj1 + str ( 10 )
print ( obj )
obj = 'hi ' * 3
print ( obj )
relational operator
print ( 'a' < 'b' )
slicing
strObj = 'india'
print ( strObj [ 1 : 4 ] )
print ( strObj [ 1 : 4 : 2 ])
print ( strObj [ : : -1 ])
FUNCTIONS
len ()
max ()
min ()
sum () : can not work with string because string + int is not permitted in python
ord ( ) to get the asci value of a specific char
chr ( ) to get the char from asci value.
str = "Python"
print ("max element =", max(str))
print ("min element =", min(str))
print ("length of list =", len(str))
#print ("sum =", sum (str)) #Error
s= "A"
print ("ASCII value of A=", ord (s))
s1= 90
print ("char of 90=", chr (s1))
Methods :
for change case :
. upper ()
. lower ()
. title ()
. swapcase()
. capitalize()
. split ()
. splitlines ()
. zfill()
.join ()
s = "A$ababc$abcdabcde"
print (s.split ("$"))
print (s.split ("$", 2))
print (s. partition("$",) ) #from left side it will partition
print (s. rpartition("$",) )# from right side it will partition
s1 = '''Heloo c
hello C++
Hello Java
hello Py'''
print (s1)
print (s1.split ("\n"))
print (s1.splitlines ()) #split line wise
s2 = "1"
print (s2)
print (s2.zfill (5)) #zerofill
s3 = "abc"
print (",". join (s3))
print ("==". join (s3))
String Methods :
capitalize() Converts the first character to upper case
casefold() Converts string into lower case
center() Returns a centered string
count() Returns the number of times a specified value occurs in a string
encode() Returns an encoded version of the string
endswith() Returns true if the string ends with the specified value
expandtabs() Sets the tab size of the string
find() Searches the string for a specified value and returns the position of where it was found
format() Formats specified values in a string
format_map() Formats specified values in a string
index() Searches the string for a specified value and returns the position of where it was found
isalnum() Returns True if all characters in the string are alphanumeric
isalpha() Returns True if all characters in the string are in the alphabet
isascii() Returns True if all characters in the string are ascii characters
isdecimal() Returns True if all characters in the string are decimals
isdigit() Returns True if all characters in the string are digits
isidentifier() Returns True if the string is an identifier
islower() Returns True if all characters in the string are lower case
isnumeric() Returns True if all characters in the string are numeric
isprintable() Returns True if all characters in the string are printable
isspace() Returns True if all characters in the string are whitespaces
istitle() Returns True if the string follows the rules of a title
isupper() Returns True if all characters in the string are upper case
join() Converts the elements of an iterable into a string
ljust() Returns a left justified version of the string
lower() Converts a string into lower case
lstrip() Returns a left trim version of the string
maketrans() Returns a translation table to be used in translations
partition() Returns a tuple where the string is parted into three parts
removeprefix() Return a str with the given prefix string removed if present.
removesuffix() Return a str with the given suffix string removed if present.
replace() Returns a string where a specified value is replaced with a specified value
rfind() Searches the string for a specified value and returns the last position of where it was found
rindex() Searches the string for a specified value and returns the last position of where it was found
rjust() Returns a right justified version of the string
rpartition() Returns a tuple where the string is parted into three parts
rsplit() Splits the string at the specified separator, and returns a list
rstrip() Returns a right trim version of the string
split() Splits the string at the specified separator, and returns a list
splitlines() Splits the string at line breaks and returns a list
startswith() Returns true if the string starts with the specified value
strip() Returns a trimmed version of the string
swapcase() Swaps cases, lower case becomes upper case and vice versa
title() Converts the first character of each word to upper case
translate() Returns a translated string
upper() Converts a string into upper case
zfill() Fills the string with a pspecified number of 0 values at the beginning
str = "Python"
print (str)
s1 = str .center (13, "*")
print (s1)
s2= str.rjust (9, "*")
print (s2)
s3= str .ljust (9, "*")
print (s3)
s1= s1.strip ("*")
print (s1)
s2= s2.lstrip ("*")
print (s2)
s3= s3.rstrip ("*")
print (s3)
expandtabs ()
str = "hello sir my name is raj & my dept is ME"
print (str)
print (str.expandtabs (1) )
print (str.expandtabs (0) )
print (str.expandtabs (2) )
count ()
find ()
replace ()
index ()
obj = "litiitniit"
#ind 0123456789
print (obj) #litiitniit
print (obj.count ("i")) #from 0 to len -1 index
print (obj.count ("i", 2 )) # 2 means start index to len -1
print (obj.count ("i", 2 , 4)) # 2 means start index 4 means stop index - 1
print (obj.count ("i", 2 , 5)) # 2 means start index 4 means stop index - 1
print ("---------find method ----------")
print ( obj.find ("i"))
print ( obj.find ("i", 0))
print ( obj.find ("i", 1))
print ( obj.find ("i", 7))
print ( obj.find ("i", 8))
print ( obj.find ("i", 9)) #-1
print ("-----index method -----")
print (obj.index ("i"))
print (obj.index ("i", 0))
print (obj.index ("i", 1))
print (obj.index ("i", 8))
#print (obj.index ("i", 9)) #Error
print (obj)
print (obj.replace ("i", "I") )
print (obj.replace ("i", "I", 2 ) )
print (obj.replace ("i", "I", 4 ) )
format ()
format_map ()
str = "hello sir my name is {name} , my age is {no} & dept is {dept}"
print (str)
print (str.format (name = "raj", no = 23, dept = "cse") )
maketrans ()
translate ()
str= "Hello Python"
table = str.maketrans ("P", "J")
#print (table )
print (str.translate (table) )
Challenges:
Understanding String operations.
Handling coding errors.
Resources:
Official Python Documentation: String
Scaler' Python Tutorial: string
Goals for Tomorrow:
Explore operator in python.
Learn about operator , types.
Conclusion:
Day 7 was a success! String is now under my belt.
What are your favorite Python libraries for data manipulation? Share in the comments below.
Connect with me:
LinkedIn: [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
GitHub: [ https://github.com/p-archana1 ]
Join the conversation:
Share your own learning experiences or ask questions in the comments.
Next Post:
Day 8: Operators and types.
Happy reading!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.