Mastering JavaScript Closures: A Comprehensive Guide
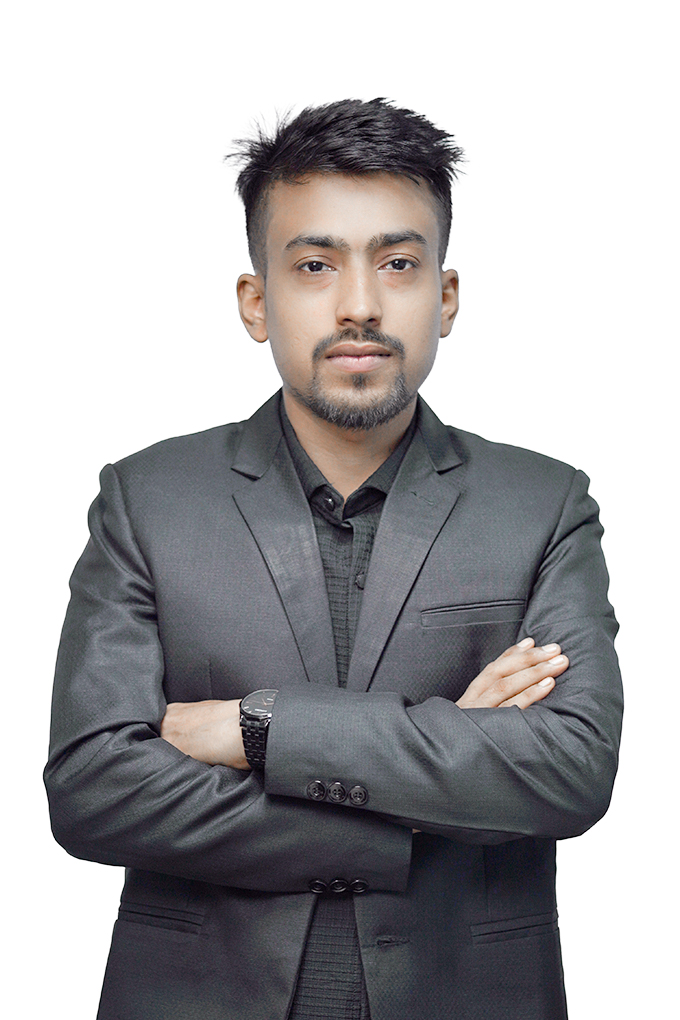
Introduction:
Closures are a fundamental concept in JavaScript that allows functions to access variables from their outer scope, even after the outer function has returned. This ability gives JavaScript its unique approach to state management and functional programming.
What is a closure?
A closure is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives a function access to its outer scope. In JavaScript, closures are created every time a function is created, at the function creation time.
Examples:
function outer() {
let count = 0;
return function increment() {
count++;
console.log(count);
};
}
const counter = outer();
counter(); // Output: 1
counter(); // Output: 2
In this example, increment
forms a closure with the count
variable, allowing it to retain and modify the count
value across multiple calls.
Use Cases for Closures:
Data privacy: Using closures, you can create private variables.
Callbacks & asynchronous programming: Closures are key when working with callbacks (e.g., in-event listeners or timers).
Example: Private Variables:
function createCounter() {
let count = 0;
return {
increment: () => count++,
getValue: () => count
};
}
const myCounter = createCounter();
myCounter.increment();
console.log(myCounter.getValue()); // Output: 1
Closures are critical in JavaScript, especially in scenarios where state management, data privacy, and functional programming are needed.
Subscribe to my newsletter
Read articles from Borhan Uddin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
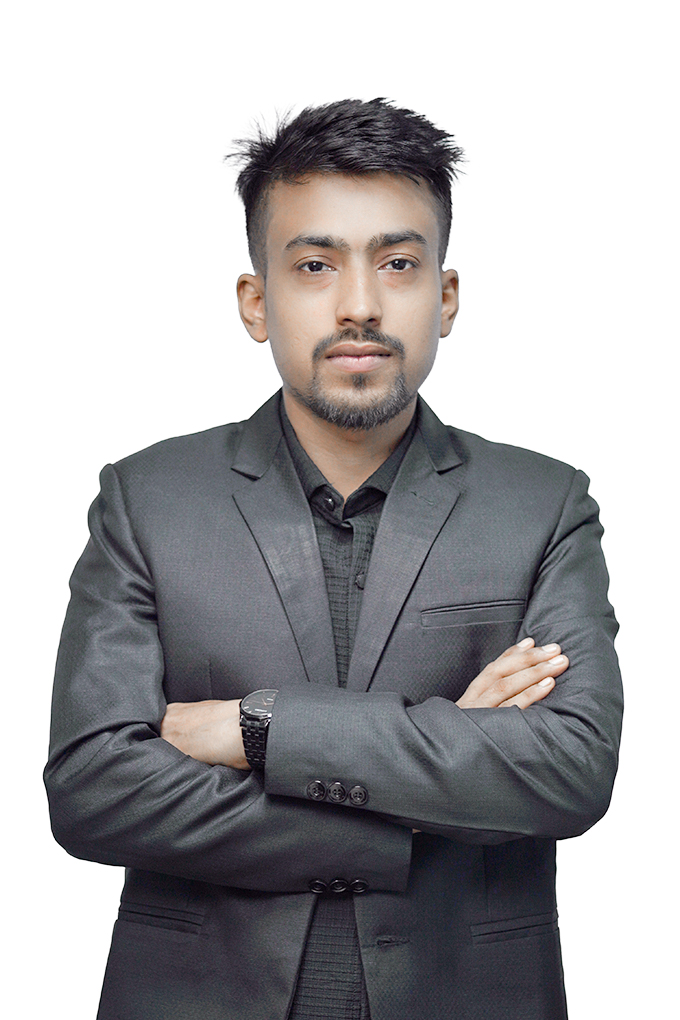
Borhan Uddin
Borhan Uddin
Experienced Full Stack Developer with 3 years of experience. Adept at creating efficient and user-friendly web applications. Strong problem-solving and teamwork skills. Committed to delivering high-quality results and continuously improving development practices.