Async/Await vs Promises: How to Handle Asynchronous JavaScript
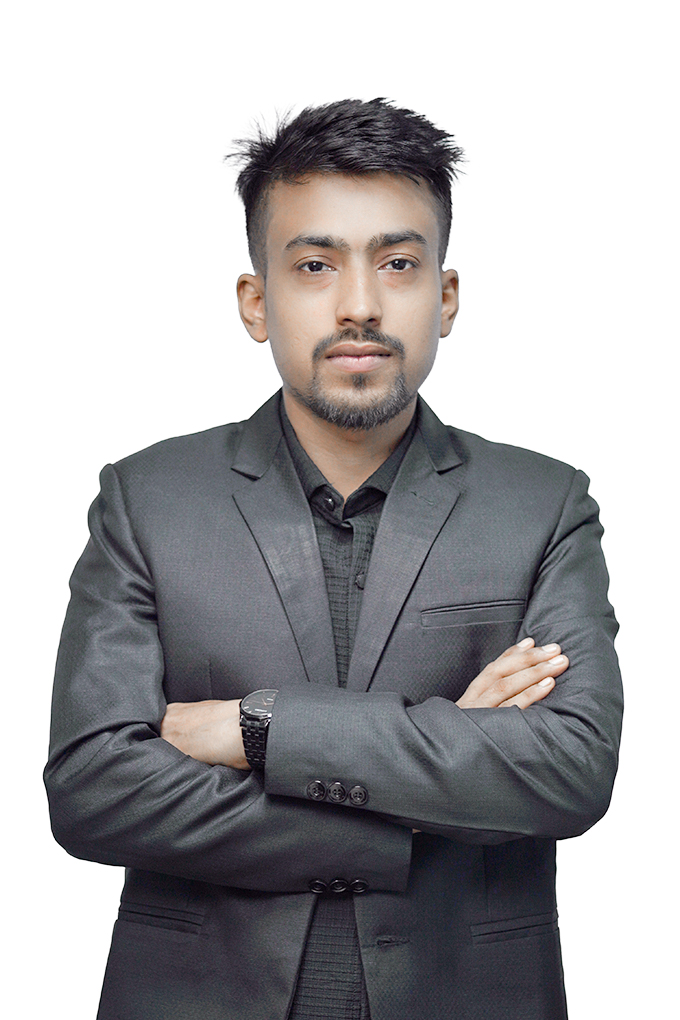
Introduction:
JavaScript is asynchronous, which means certain actions (like fetching data) don’t block the rest of your code. Promises and async/await help manage these non-blocking actions.
Working with Promises:
A promise is an object that represents the eventual completion (or failure) of an asynchronous operation.
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => resolve("Data fetched!"), 2000);
});
};
fetchData().then(data => {
console.log(data); // Output: "Data fetched!" after 2 seconds
});
Using Async/Await:
Async/await simplifies the syntax of promises by making asynchronous code look like synchronous code.
async function getData() {
const data = await fetchData();
console.log(data); // Output: "Data fetched!"
}
getData();
Error Handling:
Promises handle errors using .catch()
, while async/await uses try...catch
.
// Using Promises
fetchData()
.then(data => console.log(data))
.catch(err => console.error(err));
// Using Async/Await
async function getData() {
try {
const data = await fetchData();
console.log(data);
} catch (err) {
console.error(err);
}
}
When to Use Which:
Async/await is generally easier to read and write but works on top of promises. Promises are more flexible and are required in scenarios like parallel execution (Promise.all
).
Subscribe to my newsletter
Read articles from Borhan Uddin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
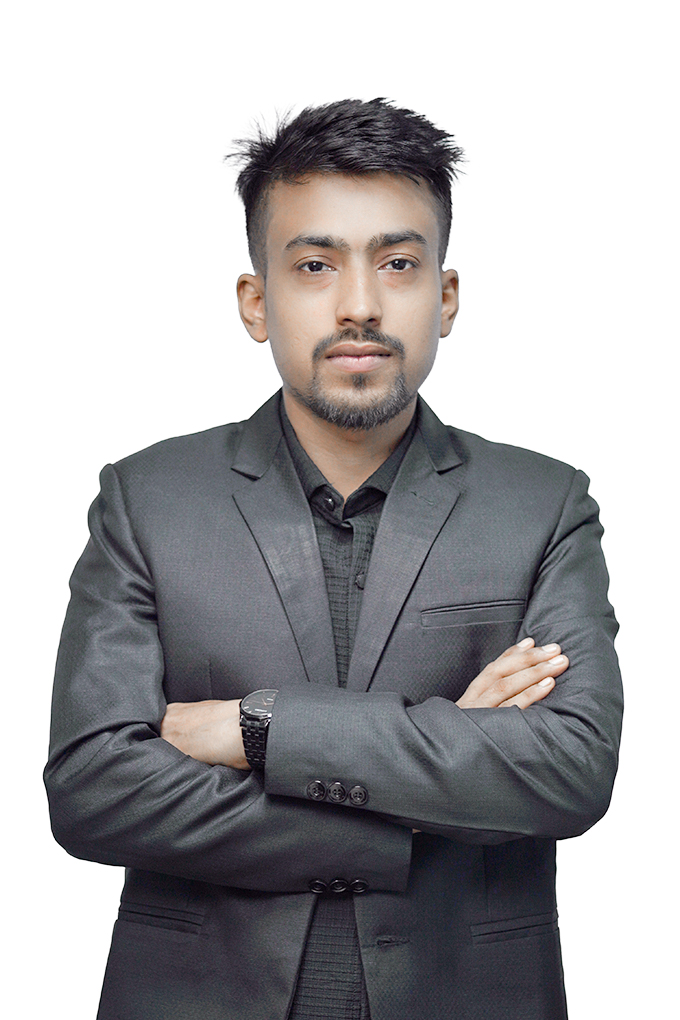
Borhan Uddin
Borhan Uddin
Experienced Full Stack Developer with 3 years of experience. Adept at creating efficient and user-friendly web applications. Strong problem-solving and teamwork skills. Committed to delivering high-quality results and continuously improving development practices.