Understanding Rust's Ownership, Borrowing, and References
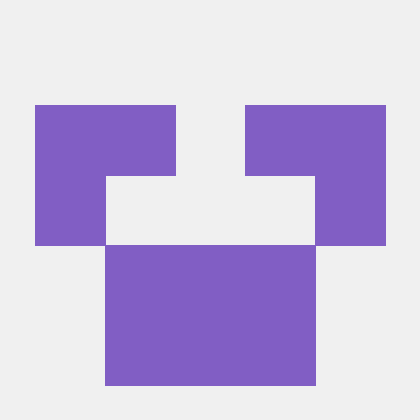
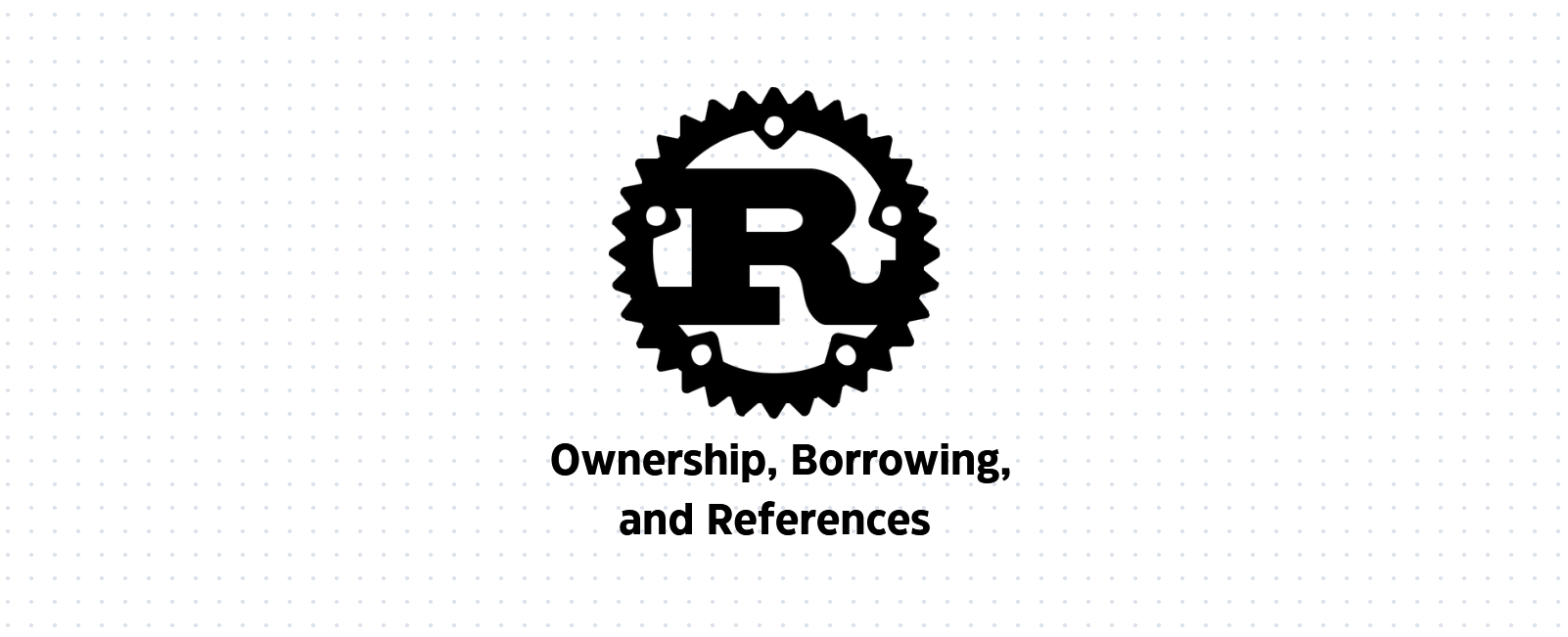
Rust has gained significant popularity in recent years, largely due to its unique approach to memory management and concurrency. At the heart of Rust's safety guarantees lies its ownership system, along with the concepts of borrowing and references. In this comprehensive guide, we'll explore these fundamental concepts in depth, providing you with a solid understanding of how Rust manages memory and ensures safety without sacrificing performance.
1. The Foundations of Rust's Memory Management
Before we dive into the specifics of Rust's ownership system, it's crucial to understand why Rust takes this unique approach to memory management.
The Memory Management Spectrum
Manual Memory Management: Languages like C and C++ give programmers full control over memory allocation and deallocation. This approach offers maximum flexibility and performance but puts the burden of correct memory management on the programmer.
Garbage Collection: Languages like Java, Python, and JavaScript use garbage collectors to automatically free memory that's no longer in use. This approach is convenient for developers but can lead to unpredictable performance pauses and higher memory usage.
Ownership System: Rust introduces a novel approach that combines the performance of manual memory management with the safety of garbage collection, all enforced at compile-time.
2. Ownership: The Core Concept
Ownership is Rust's most unique feature and the cornerstone of its memory safety guarantees. Let's explore this concept in depth.
What is Ownership?
In Rust, every value has an "owner" - a variable that is responsible for that value. The owner is responsible for cleaning up the memory associated with the value when it's no longer needed.
The Rules of Ownership
Rust's ownership system follows three fundamental rules:
Each value in Rust has a variable that's called its owner.
There can only be one owner at a time.
When the owner goes out of scope, the value will be dropped.
Let's examine each of these rules in detail with examples.
Rule 1: Each value has an owner
fn main() {
let s = String::from("hello"); // s is the owner of this String
// s is valid here
// do stuff with s
} // s goes out of scope here, and the String is automatically freed
In this example, s
is the owner of the String
value. It's responsible for that value throughout its lifetime.
Rule 2: One owner at a time
fn main() {
let s1 = String::from("hello");
let s2 = s1; // s1's ownership is moved to s2
// println!("{}", s1); // This would cause a compile-time error
println!("{}", s2); // This is fine
}
Here, the ownership of the String
is moved from s1
to s2
. After this move, s1
is no longer valid, and Rust won't let us use it. This prevents double free errors and use-after-free bugs.
Rule 3: Value is dropped when owner goes out of scope
fn main() {
{
let s = String::from("hello"); // s is valid from this point forward
// do stuff with s
} // this scope is now over, and s is no longer valid
}
When s
goes out of scope, Rust automatically calls the drop
function and cleans up the memory for the String
.
Move Semantics
Rust uses move semantics by default for types that don't implement the
Copy
trait. When a value is assigned to another variable or passed as an argument to a function, ownership is moved.fn main() { let s1 = String::from("hello"); let s2 = s1; // Move occurs here // println!("{}", s1); // Error: value borrowed here after move println!("{}", s2); // This is OK }
This behavior prevents multiple parts of your code from trying to free the same memory, which could lead to corruption.
Clone for Deep Copy
If you want to create a deep copy of a value instead of moving it, you can use the
clone
method:fn main() { let s1 = String::from("hello"); let s2 = s1.clone(); println!("s1 = {}, s2 = {}", s1, s2); // Both are valid }
However,
clone
can be expensive for large data structures, so it's used sparingly in Rust code.
3. Borrowing and References: Flexible Use Without Ownership
While ownership is powerful, it can be inconvenient if we want to use a value in multiple places without transferring ownership. This is where borrowing comes in.
What is Borrowing?
Borrowing allows you to refer to some value without taking ownership of it. In Rust, borrowing is done through references, denoted by the
&
symbol.fn main() { let s1 = String::from("hello"); let len = calculate_length(&s1); // We borrow s1 here println!("The length of '{}' is {}.", s1, len); } fn calculate_length(s: &String) -> u32 { s.len() }
In this example,
&s1
creates a reference tos1
without taking ownership. Thecalculate_length
function borrows theString
, uses it, and then the borrowed reference goes out of scope.Mutable and Immutable References
Rust allows two types of references:
Immutable references (
&T
)Mutable references (
&mut T
)
Immutable References
Immutable references allow you to read the data but not modify it:
fn main() { let s = String::from("hello"); let r1 = &s; // Immutable reference let r2 = &s; // Another immutable reference println!("{} and {}", r1, r2); }
You can have multiple immutable references to the same data at the same time.
Mutable References
Mutable references allow you to modify the data:
fn main() { let mut s = String::from("hello"); let r = &mut s; // Mutable reference r.push_str(", world"); println!("{}", r); }
However, you can only have one mutable reference to a particular piece of data in a particular scope.
The Rules of References
Rust enforces some important rules with references:
At any given time, you can have either one mutable reference or any number of immutable references.
References must always be valid.
These rules prevent data races at compile-time. A data race occurs when:
Two or more pointers access the same data at the same time.
At least one of the pointers is being used to write to the data.
There's no mechanism being used to synchronize access to the data.
Rust prevents data races by making them impossible to compile!
fn main() {
let mut s = String::from("hello");
let r1 = &s; // no problem
let r2 = &s; // no problem
println!("{} and {}", r1, r2);
// r1 and r2 are no longer used after this point
let r3 = &mut s; // no problem
println!("{}", r3);
}
This code compiles because the last usage of the immutable references r1
and r2
occurs before the mutable reference r3
is introduced.
The Slice Type
Slices are a kind of reference that allow you to refer to a contiguous sequence of elements in a collection rather than the whole collection. Let's look at string slices as an example:
fn main() { let s = String::from("hello world"); let hello = &s[0..5]; let world = &s[6..11]; println!("{} {}", hello, world); }
Slices are particularly useful for operations that need to work with a portion of a string or array without taking ownership.
4. Lifetimes: Ensuring Reference Validity
Lifetimes are a crucial concept in Rust that ensures references are valid for as long as we need them to be. Every reference in Rust has a lifetime, which is the scope for which that reference is valid. Understanding lifetimes is key to writing safe and efficient Rust code.
Implicit Lifetimes
In most cases, lifetimes are implicit and inferred by the Rust compiler. This means you don't need to explicitly annotate them in your code. The compiler uses a set of rules to determine how long each reference should live based on how it's used in your program.
Let's look at some examples to understand how implicit lifetimes work:
Example 1: Simple Reference
fn main() {
let x = 5; // ----------+-- 'b
// |
let r = &x; // --+-- 'a |
// | |
println!("r: {}", r); // | |
// --+ |
} // ----------+
In this example:
The variable
x
has a lifetime that we can call'b
. It starts whenx
is created and ends at the end of themain
function.The reference
r
has a lifetime that we can call'a
. It starts whenr
is created and ends after theprintln!
statement.
The Rust compiler can see that the lifetime 'a
of the reference r
is completely contained within the lifetime 'b
of x
. This means r
will always refer to valid data, so this code is safe.
Example 2: References in Functions
fn longest(x: &str, y: &str) -> &str {
if x.len() > y.len() {
x
} else {
y
}
}
fn main() {
let string1 = String::from("short");
let string2 = String::from("longer");
let result = longest(string1.as_str(), string2.as_str());
println!("The longest string is {}", result);
}
In this example, the longest
function takes two string slices and returns a reference to the longer one. The Rust compiler infers that the lifetime of the returned reference must be the same as the shorter of the two input lifetimes. This ensures that the returned reference is always valid.
Why Lifetimes Matter
Lifetimes are crucial for preventing dangling references. A dangling reference is a reference that points to memory that may have been deallocated or repurposed. Here's an example of code that Rust prevents:
fn main() { let r; { let x = 5; r = &x; } // x is dropped here println!("r: {}", r); // Error: `x` does not live long enough }
In this case,
r
would be a dangling reference because it refers tox
, which was dropped when its scope ended. Rust's lifetime system catches this at compile-time and prevents the program from compiling.Lifetime Inference in Practice
In practice, you often don't need to think about lifetimes explicitly. The Rust compiler is quite sophisticated and can infer lifetimes in many situations. However, understanding the concept of lifetimes is crucial for those cases where the compiler needs more information or when you're dealing with more complex scenarios involving references. By ensuring that all references are valid for their entire lifetime, Rust prevents a whole class of bugs related to memory safety, without sacrificing performance. This is one of the key features that makes Rust both safe and efficient.
5. Comparing Rust's Approach with C++ and JavaScript
To fully appreciate Rust's memory management approach, let's compare it with two other popular languages: C++ and JavaScript.
C++: Manual Memory Management
C++ gives programmers full control over memory management. In C++, you need to manually manage memory allocation (new
) and deallocation (delete
). This approach offers maximum flexibility and performance but can lead to issues like:
Memory leaks (forgetting to free memory)
Double free errors (freeing memory twice)
Use-after-free bugs (using memory after it's been freed)
JavaScript: Garbage Collection
JavaScript uses a garbage collector to automatically manage memory. Garbage collection frees developers from manual memory management but can lead to:
Unpredictable performance pauses when the garbage collector runs
Higher memory usage, as objects aren't immediately freed when they're no longer needed
Rust: The Best of Both Worlds
Rust's ownership system combines the performance of manual memory management with the safety of garbage collection.
Rust's approach offers several advantages:
Memory is freed immediately when it's no longer needed, just like in manual memory management.
The compiler ensures memory safety at compile-time, preventing common bugs found in C++.
There's no need for a garbage collector, avoiding unpredictable runtime pauses.
The ownership rules are enforced at compile-time, so there's no runtime cost for these checks.
Conclusion
Rust's ownership system, along with borrowing, references, and lifetimes, provides a unique and powerful approach to memory management. While these concepts might seem complex at first, they enable Rust to guarantee memory safety without sacrificing performance.
As you continue your journey with Rust, you'll find that these rules become second nature, leading to safer and more efficient code. The ownership system not only prevents common programming errors but also encourages you to think carefully about the structure and data flow in your programs.
Remember, Rust's ownership system is not just about memory management it's a fundamental design philosophy that influences how you architect your entire program. By embracing these concepts, you're not just writing safer code; you're adopting a new way of thinking about software design that can improve your skills across all programming languages.
Happy coding in Rust, and may your programs be forever free of segmentation faults and data races!
Subscribe to my newsletter
Read articles from Siddhesh Parate directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
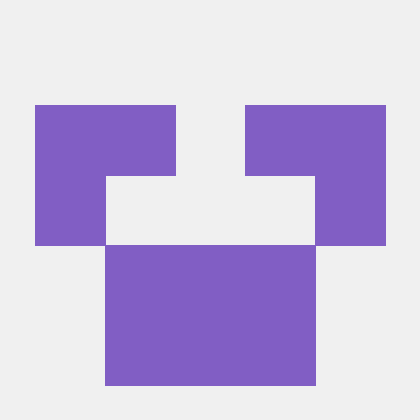