Are You Handling Type Conversion Properly in JavaScript?

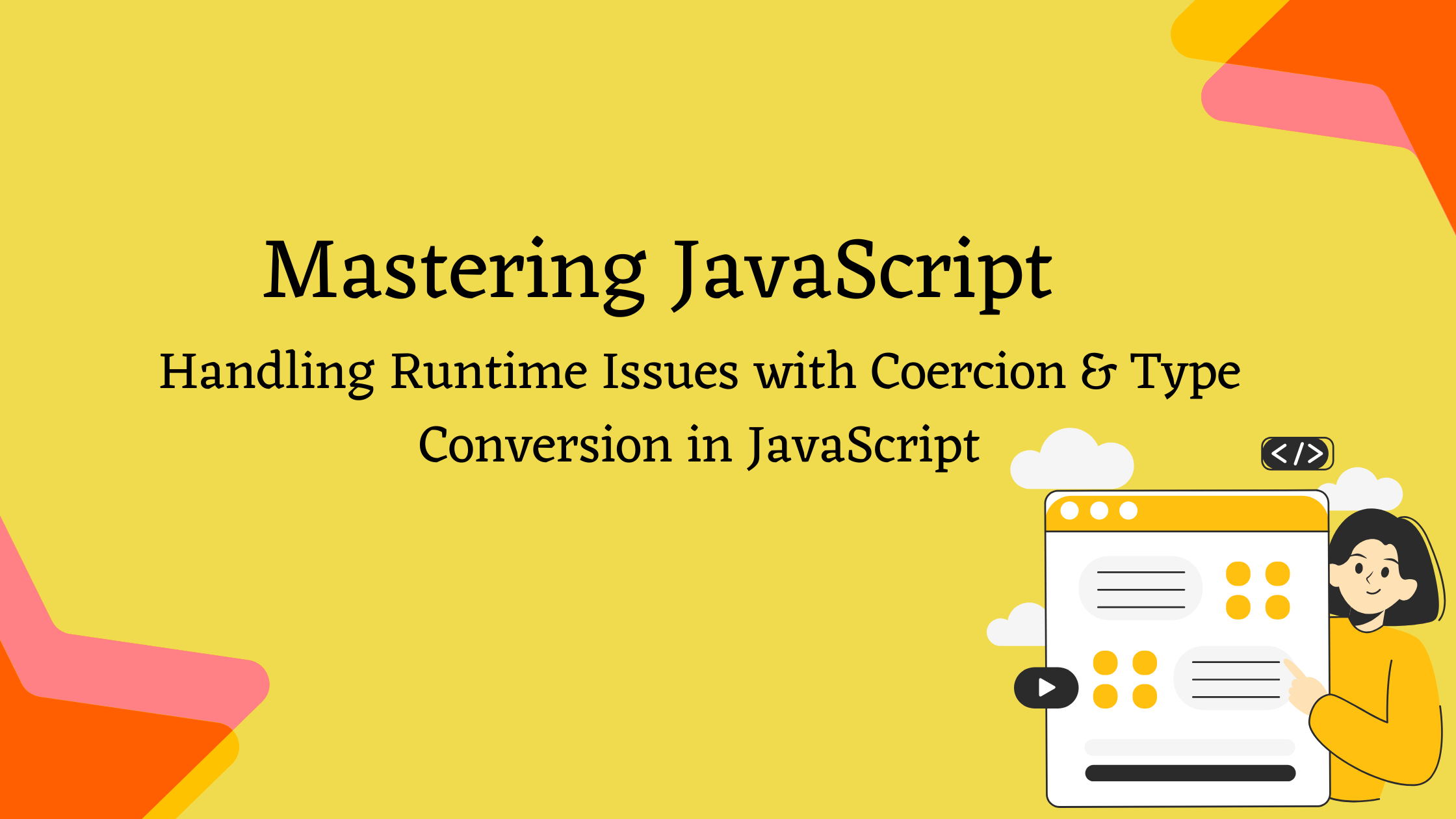
In JavaScript, one common source of bugs arises from type coercion—the automatic conversion of data types that the language performs behind the scenes. When JavaScript tries to "help" by implicitly converting values into types it thinks are needed, it can lead to unpredictable behavior and runtime issues, especially when working with different types like strings, numbers, or booleans.
If you're facing strange results in your JavaScript code and looking for a solution, this article will help you understand coercion, type conversion, and how to avoid the issues they cause.
Understanding the Problem
JavaScript variables can hold any type of value at any time, which makes it flexible but also prone to errors during runtime. The most frequent issues occur when you’re trying to perform an operation on variables that seem right but are of unexpected types.
For example:
let result = "5" + 2;
console.log(result); // "52"
Here, instead of getting the numeric sum 7
, you end up with "52"
. This happens because the +
operator coerces the number 2
into a string "2"
and concatenates it with the string "5"
. This automatic coercion can be dangerous if you're unaware of it, as it might produce unexpected results.
To solve these problems, we first need to understand two key concepts: Type Conversion and Type Coercion.
Type Conversion (Explicit Conversion)
Type conversion is a process where you, the developer, explicitly change the type of a variable. This is the safest way to handle mixed data types since you have control over the conversion.
Example:
let numStr = "123";
let num = Number(numStr); // Explicit conversion from string to number
console.log(typeof num); // "number"
console.log(num); // 123
By explicitly converting the string "123"
to the number 123
using Number()
, you avoid any unexpected behavior during operations involving this value.
Here are the most common explicit type conversions in JavaScript:
Number()
– Converts a value to a number.String()
– Converts a value to a string.Boolean()
– Converts a value to a boolean (true
orfalse
).
Type Coercion (Implicit Conversion)
Type coercion happens automatically when JavaScript tries to perform an operation with mismatched types. It tries to convert one or both values to a common type, but this can lead to runtime issues if not handled carefully.
Here’s an example of type coercion in action:
let value = "10" * 2;
console.log(value); // 20 (string "10" is coerced to number 10)
In this case, JavaScript converts the string "10"
to a number automatically. But consider this example:
let value = "Hello" * 2;
console.log(value); // NaN
Here, "Hello"
cannot be converted to a valid number, and the result is NaN
(Not a Number). This is where runtime issues start to creep in.
Common Runtime Problems due to Coercion
1. Unintended String Concatenation
One of the most common bugs occurs when numbers are accidentally treated as strings due to the +
operator:
let a = "10";
let b = 5;
let result = a + b;
console.log(result); // "105" (string concatenation)
Solution: Explicitly convert variables to the desired type before performing operations.
let a = "10";
let b = 5;
let result = Number(a) + b;
console.log(result); // 15 (correct result)
2. NaN Errors
If you try to perform mathematical operations on non-numeric values, you'll likely end up with NaN
. This can happen unexpectedly if you assume a value is a number when it’s not.
let x = "abc" * 10;
console.log(x); // NaN
Solution: Always check the type of variables or ensure they are numbers before performing arithmetic operations.
let x = "abc";
if (!isNaN(x)) {
x = Number(x) * 10;
} else {
console.log("Invalid input");
}
3. Boolean Coercion in Conditions
In conditions, JavaScript automatically coerces values into booleans. Some values are considered "falsy" and others "truthy". Here’s an example of a falsy value causing unexpected behavior:
let num = 0;
if (num) {
console.log("This will not execute"); // Because 0 is falsy
} else {
console.log("0 is falsy");
}
Solution: Use Boolean()
for explicit coercion when needed, and always ensure you're handling truthy/falsy values properly.
let num = 0;
if (Boolean(num)) {
console.log("This will execute if num is truthy");
} else {
console.log("This will execute if num is falsy");
}
How to Avoid Coercion Issues
To avoid problems caused by type coercion, follow these best practices:
Use Strict Equality (
===
): The loose equality operator (==
) triggers coercion, while strict equality (===
) does not. Use===
to avoid unintended type coercion during comparisons.console.log(1 == "1"); // true (due to coercion) console.log(1 === "1"); // false (no coercion)
Always Explicitly Convert Types: If you know what type a variable should be, explicitly convert it before performing operations or comparisons. This gives you control over the type and avoids surprises.
let str = "123"; let num = Number(str); console.log(num); // 123
Check for
NaN
: When working with numbers, always check if the result of a calculation isNaN
. UseisNaN()
to validate the result of arithmetic operations before proceeding.let result = "abc" * 10; if (isNaN(result)) { console.log("Invalid operation"); }
Handle Falsy Values Explicitly: In logical operations, handle falsy values explicitly to avoid unexpected behavior.
let value = 0; if (Boolean(value)) { console.log("This is truthy"); } else { console.log("This is falsy"); }
Conclusion
Type coercion and conversion in JavaScript are at the heart of many runtime issues, particularly when dealing with mixed data types like strings, numbers, and booleans. By understanding how JavaScript automatically converts values—and using explicit type conversions when needed—you can avoid many common pitfalls.
Remember to:
Use strict equality (
===
) instead of loose equality (==
).Explicitly convert values before performing operations.
Always check for
NaN
when performing arithmetic.Be aware of truthy and falsy values in conditions.
With these strategies, you’ll reduce runtime errors and write more reliable, predictable code. Happy debugging!
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
I’m a problem solver at heart, driven by the idea of building solutions that genuinely make a difference in people’s everyday lives. I’m always curious, always learning, and always looking for ways to improve the world around me through thoughtful, impactful work. Beyond building, I love giving back to the community — whether it’s by sharing what I’ve learned through blogs, tutorials, or helpful insights. My goal is simple: to make technology a little more accessible and useful for everyone. Let’s learn, build, and grow together.