Cracking the Code: Mastering Dimensionality Reduction Techniques in Machine Learning

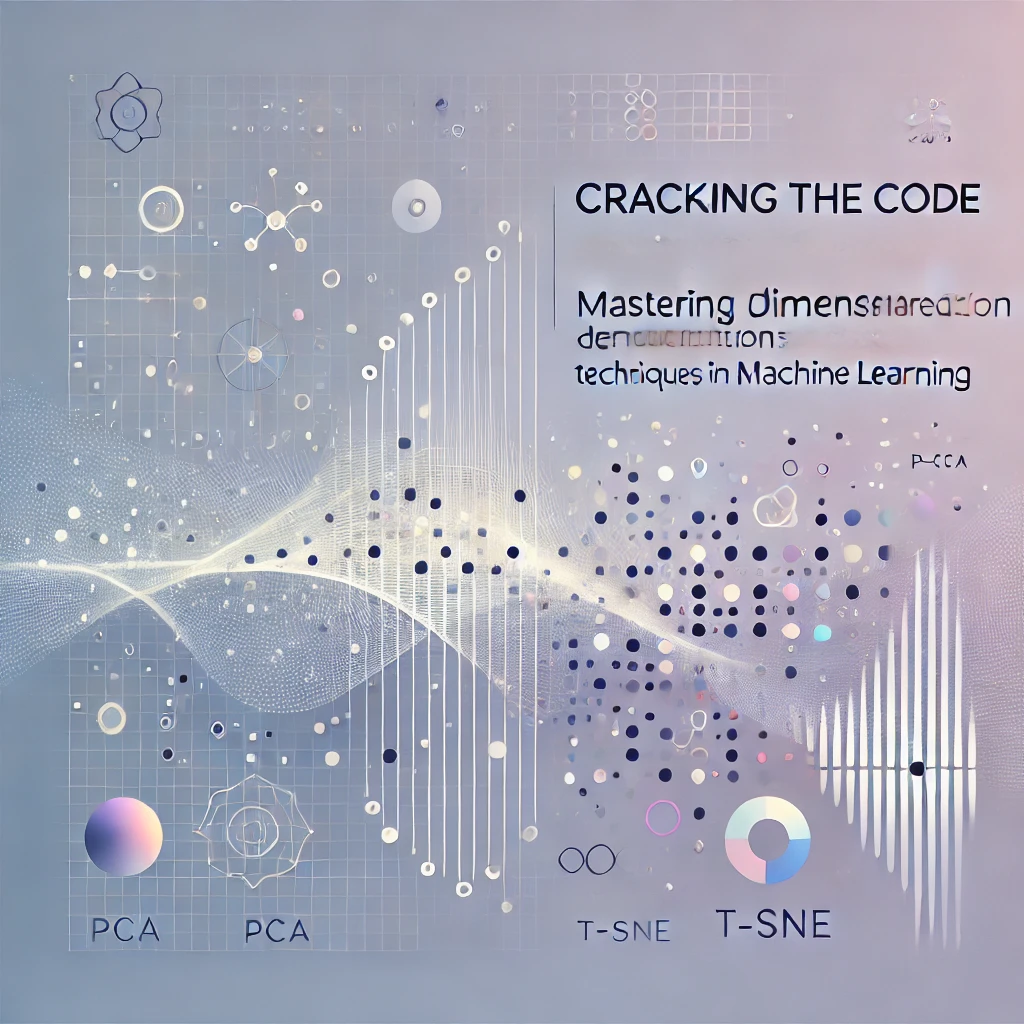
Introduction
In machine learning, we often work with datasets containing a large number of features or variables. While having more data might seem beneficial, high-dimensional datasets can lead to overfitting, increased computational costs, and reduced model performance. This problem is known as the curse of dimensionality. Dimensionality reduction helps solve this by transforming high-dimensional data into a lower-dimensional space without losing significant information.
In this blog, we’ll explore common dimensionality reduction techniques, how they work, and how you can implement them with Python.
1. Principal Component Analysis (PCA)
Definition
PCA is a linear technique that reduces dimensionality by projecting data onto the directions of maximum variance. It transforms the original variables into new ones called principal components (PCs), which are ordered by the amount of variance they explain.
How PCA Works:
Compute the covariance matrix of the data.
Compute the eigenvectors and eigenvalues of the covariance matrix.
Sort the eigenvectors by their corresponding eigenvalues in descending order.
Select the top
k
eigenvectors to form the principal components.Project the original data onto the new
k
-dimensional space.
Real-world Example:
PCA is used in image compression, where high-dimensional pixel data can be reduced to capture the most important features.
Code Snippet: Implementing PCA in Python
from sklearn.decomposition import PCA
import numpy as np
import matplotlib.pyplot as plt
# Generate sample data
np.random.seed(42)
X = np.random.rand(100, 5)
# Apply PCA to reduce to 2 dimensions
pca = PCA(n_components=2)
X_reduced = pca.fit_transform(X)
# Plot the reduced data
plt.scatter(X_reduced[:, 0], X_reduced[:, 1], c='blue')
plt.title('PCA: 2D Projection of Data')
plt.xlabel('Principal Component 1')
plt.ylabel('Principal Component 2')
plt.show()
# Explained variance ratio
print("Explained variance ratio:", pca.explained_variance_ratio_)
Visual Aid:
- A scatter plot showing the projection of high-dimensional data onto two principal components.
Advantages:
Reduces dimensionality while preserving as much variance as possible.
Useful for visualizing high-dimensional data.
Limitations:
Assumes linear relationships between features.
Sensitive to outliers.
2. Linear Discriminant Analysis (LDA)
Definition
LDA is both a dimensionality reduction and classification technique. It aims to maximize the separation between multiple classes by finding the feature space that maximizes class separability.
How LDA Works:
Compute the within-class and between-class scatter matrices.
Solve for the eigenvectors and eigenvalues of the scatter matrices.
Select the eigenvectors that correspond to the largest eigenvalues to form the new subspace.
Real-world Example:
LDA is used in face recognition systems, where it helps reduce the dimensionality of pixel data while maintaining class separability (different faces).
Code Snippet: Implementing LDA in Python
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis as LDA
from sklearn.datasets import load_iris
import matplotlib.pyplot as plt
# Load Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Apply LDA
lda = LDA(n_components=2)
X_reduced = lda.fit_transform(X, y)
# Plot the reduced data
plt.scatter(X_reduced[:, 0], X_reduced[:, 1], c=y, cmap='rainbow', edgecolor='k')
plt.title('LDA: 2D Projection of Data')
plt.xlabel('LDA Component 1')
plt.ylabel('LDA Component 2')
plt.show()
Visual Aid:
- A scatter plot showing the reduced data with clear separation between different classes.
Advantages:
Improves class separability, making it easier for classifiers to perform well.
Reduces dimensionality while preserving class information.
Limitations:
Works best when the classes are linearly separable.
Requires labeled data, making it unsuitable for unsupervised learning.
3. t-SNE (t-Distributed Stochastic Neighbor Embedding)
Definition
t-SNE is a nonlinear dimensionality reduction technique that maps high-dimensional data to a lower-dimensional space, often used for visualizing complex datasets in 2D or 3D. It is particularly effective in revealing cluster structures in data.
How t-SNE Works:
Computes the pairwise similarities between data points in high dimensions.
Projects these similarities to a lower-dimensional space while preserving local structure.
Real-world Example:
t-SNE is used in bioinformatics for visualizing high-dimensional gene expression data to reveal patterns or clusters.
Code Snippet: Implementing t-SNE in Python
from sklearn.manifold import TSNE
import matplotlib.pyplot as plt
# Load Iris dataset
X, y = iris.data, iris.target
# Apply t-SNE
tsne = TSNE(n_components=2, perplexity=30, random_state=42)
X_reduced = tsne.fit_transform(X)
# Plot the reduced data
plt.scatter(X_reduced[:, 0], X_reduced[:, 1], c=y, cmap='rainbow', edgecolor='k')
plt.title('t-SNE: 2D Projection of Data')
plt.show()
Visual Aid:
- A scatter plot that shows how t-SNE clusters similar data points together in a 2D space.
Advantages:
Captures nonlinear relationships between features.
Great for visualizing clusters in high-dimensional data.
Limitations:
Computationally expensive for large datasets.
The result may vary significantly with different parameter settings (e.g., perplexity).
4. Autoencoders (for Dimensionality Reduction)
Definition
Autoencoders are a type of neural network that can be used for dimensionality reduction. They consist of two parts: an encoder that compresses the data into a lower-dimensional space, and a decoder that attempts to reconstruct the original data.
How Autoencoders Work:
The encoder learns a lower-dimensional representation (latent space) of the data.
The decoder reconstructs the original data from this latent representation.
The network is trained to minimize reconstruction error.
Real-world Example:
Autoencoders are used for image compression and feature extraction in deep learning applications.
Code Snippet: Implementing an Autoencoder in Python (with TensorFlow)
import tensorflow as tf
from tensorflow.keras import layers
# Define Autoencoder
input_dim = 784 # Assuming we are using flattened 28x28 images
encoding_dim = 32
input_img = tf.keras.Input(shape=(input_dim,))
encoded = layers.Dense(encoding_dim, activation='relu')(input_img)
decoded = layers.Dense(input_dim, activation='sigmoid')(encoded)
autoencoder = tf.keras.Model(input_img, decoded)
# Compile and train the autoencoder
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# Example training data: MNIST images (flattened)
(X_train, _), (X_test, _) = tf.keras.datasets.mnist.load_data()
X_train = X_train.reshape(-1, 784) / 255.0
X_test = X_test.reshape(-1, 784) / 255.0
autoencoder.fit(X_train, X_train, epochs=50, batch_size=256, shuffle=True, validation_data=(X_test, X_test))
# Extract compressed representation
encoder = tf.keras.Model(input_img, encoded)
encoded_imgs = encoder.predict(X_test)
print("Encoded representations shape:", encoded_imgs.shape)
Visual Aid:
- You could show the difference between the original images and the reconstructed images using an autoencoder.
Advantages:
Captures complex, nonlinear relationships in data.
Can be used for unsupervised learning and anomaly detection.
Limitations:
Requires a large amount of data and computing power.
Can be challenging to interpret.
Conclusion
Dimensionality reduction techniques play a critical role in making machine learning models more efficient and interpretable by removing redundancy and noise in high-dimensional datasets. Techniques like PCA and LDA are great for linear relationships, while t-SNE and autoencoders are powerful for handling nonlinear data. Selecting the right technique depends on the dataset and the goals of your analysis.
Subscribe to my newsletter
Read articles from Riya Bose directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
