Understand Binary Search!
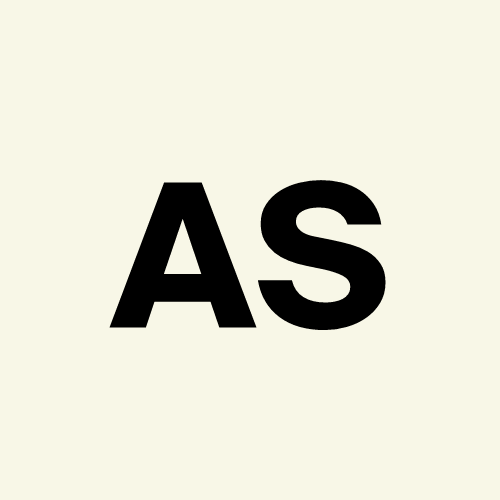
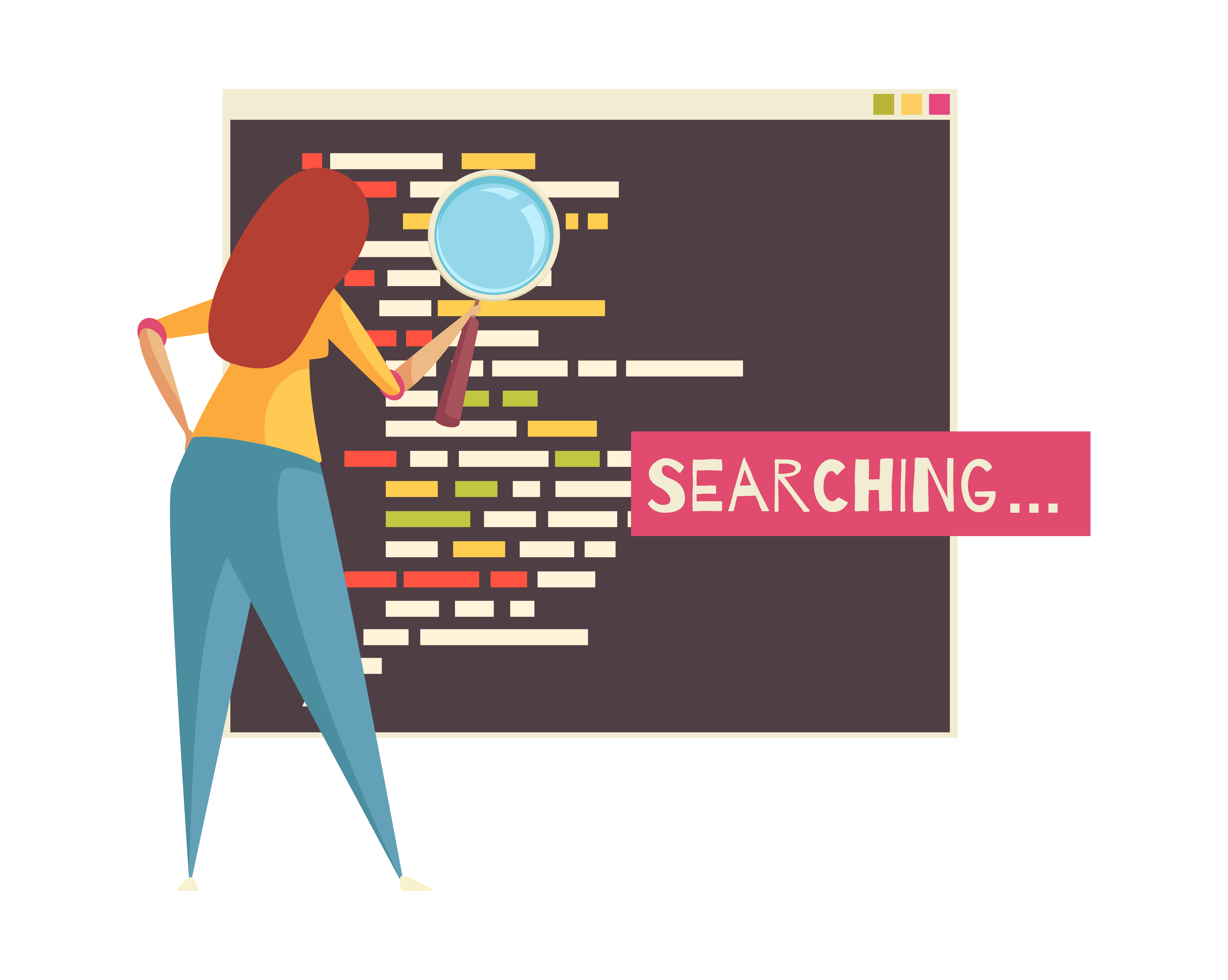
Introduction
In the realm of computer science and algorithms, searching for an element in a sorted dataset is a common task. While a linear search method is straightforward, it can be inefficient for large datasets. Enter binary search—an elegant and efficient algorithm that drastically reduces the number of comparisons needed to find an item. In this blog, we'll explore how binary search works, its implementation, and its applications.
What is Binary Search?
Binary search is an algorithm that finds the position of a target value within a sorted array. It does this by repeatedly dividing the search interval in half. If the target value is less than the item in the middle of the interval, the search continues in the lower half. Otherwise, it continues in the upper half. This process continues until the target value is found or the interval is empty.
How Binary Search Works
Initialization: Start with two pointers—
low
at the beginning of the array andhigh
at the end.Iteration:
Calculate the middle index:
mid = (low + high) / 2
.Compare the middle element with the target value:
If it matches, return the index.
If the target is less, narrow the search to the lower half:
high = mid - 1
.If the target is greater, narrow the search to the upper half:
low = mid + 1
.
Termination: Repeat until
low
is greater thanhigh
. If the target isn't found, return an indication that it's not in the array.
#include<iostream>
using namespace std;
int binarySearch(int arr[], int size, int n){
int high = 0;
int low = size -1;
while(high <=low ){
int mid = high - (high -low )/2;
if(n==arr[mid]) return mid;
if(n>arr[mid]){
high = mid +1;
}
if(n<arr[mid]) {
low = mid -1;
}
}
return -1;
}
int main(){
int even[6] = {2, 4, 6, 8, 10};
int odd[5] = {10, 11, 19, 21, 22};
// int ans = binarySearch(even, 6, 4);
int ans = binarySearch(odd, 6, 21);
cout<<"The answer is: "<<ans<<endl;
return 0;
}
Time Complexity
Binary search operates in O(logn)O(\log n)O(logn) time complexity, making it significantly faster than linear search's O(n)O(n)O(n) when dealing with large datasets. The logarithmic nature of binary search is what makes it so powerful—each iteration cuts the search space in half.
Advantages of Binary Search
Efficiency: Ideal for large datasets due to its logarithmic time complexity.
Simplicity: Conceptually easy to understand and implement.
Versatility: Can be applied to various data structures, such as arrays and trees.
Limitations
Requires Sorted Data: The input must be sorted for binary search to work.
Static Data: Not ideal for datasets that change frequently, as maintaining order can be costly.
Applications
Database Indexing: Quickly locate records in a sorted database.
Searching in Libraries: Fast retrieval of books or documents.
Games and Graphics: Finding positions in sorted lists of objects.
Conclusion
Binary search is a fundamental algorithm that showcases the power of divide-and-conquer strategies. By understanding its mechanism, you can enhance your programming skills and tackle more complex problems efficiently. Whether you’re working with large datasets or looking to optimize your code, binary search is an essential tool in any developer's toolkit.
Subscribe to my newsletter
Read articles from Ajay Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
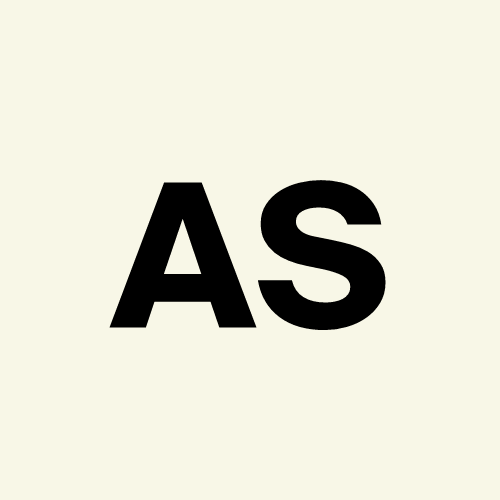