Angular Directives

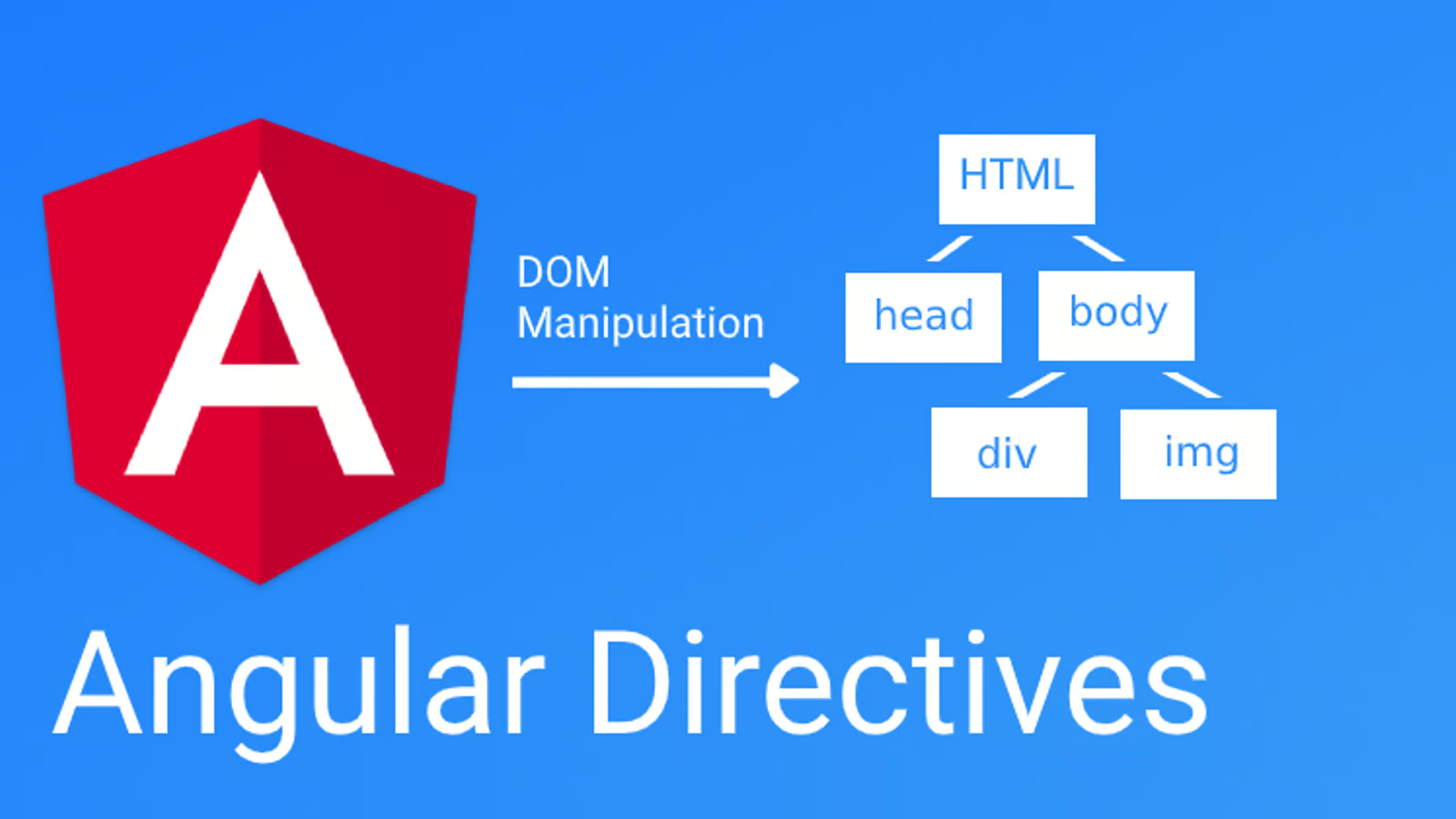
In this article we will tackle what Angular Directives, their types, use cases and examples.
What is Angular Directives?
Directives are classes that add additional behavior to elements in your Angular Applications.
Built-in Angular Directives is use to manage forms, lists, styles, and what users see.
There are three types of Angular Directives this are Components, Attribute and Structural Directives.
What is Components Directive?
- A component is completely a form of Component Directive, and it is a simple directive with its templates. These are like custom HTML elements that encapsulate their behavior and appearance, making them reusable components.
What is Attribute Directive?
- Attribute directives change the appearance or behavior of an element, component, or another directive. Essentially, it is a class annotated with the
Directive
decorator where you specify what change you want to occur and what CSS event (if any) you want to trigger that change.ngClass
,ngStyle
, andngModel
are examples of attribute directives built-in to the Angular framework.
What is Structural Directive?
- A directive that is placed on a template element and is providing possibilities to structure the DOM with it. The most well known structural directives provided by Angular are ngIf and ngFor. We can recognize a structural directive by the * in front of its selector name. This is syntactic sugar provided by Angular in order to make them more convenient to use.
Use Cases of Components Directive
Given that components are used to break down an application into smaller parts, they are widely used by developers. They can build applications via a complete component-based model.
In Angular, the Component is the one that provides data to the view. It is used to create a new View(Shadow DOM) with attached behavior.
Use Cases of Attribute Directive
You would use an attribute directive anytime you want logic or events to change the appearance or behavior of the view. Dropdowns, accordions, and tabs are just a few common use cases for custom attribute directives.
These directives are commonly used to dynamically modify the style, directive class, or behaviour of an element based on certain conditions. For example, the
ngClass
directive allows you to add or remove CSS classes based on the evaluation of an expression. Similarly, thengStyle
directive lets you set inline styles for a host element based on the evaluation of an expression.
Use Cases of Structural Directive
Show or hide content based on a boolean condition,Display lists or repeated elements dynamically based on an array or iterable and Embed or reuse templates dynamically.
Unlike attribute directives, which only change the appearance or behavior of an element, structural directives actually add, remove, or manipulate elements in the DOM. The ability to manipulate the DOM is powerful because it lets you create highly dynamic layouts.
In Angular templates, structural directives are typically recognized by a leading asterisk (*) syntax, such as
*ngIf
or*ngFor
. The asterisk is a syntactic sugar that makes it easier to write and read structural directives within templates.
Code Snippets
Component Directive Code Snippet Example
import {Component} from '@angular/core' @Component({ selector: 'app-hello', template: `<h1>Hello, World!</h1>}) export class AppComponent{}
- Here, "AppComponent" is a component directive that displays "Hello, World!" on the UI. Component directives have their own lifecycle hooks and are fundamental to Angular’s architecture. This code imports the Component class from the Angular core library and creates an Angular component called AppComponent. The @Component decorator specifies details about the component, such as its HTML template and styles.
Attributive Directive Code Snippet Example
For NgClass:
@Component({ selector: 'app-root', template: ` <div [ngClass]="{'text-success': isSuccess,'text-danger': !isSuccess}"> Text </div> ` }) export class AppComponent { isSuccess: boolean = true; }
- In this example, the text-success class is applied when isSuccess is true, and the text-danger class is applied when isSuccess is false. The isSuccess property should be defined in your component class.
For NgStyle:
@Component({
selector: 'app-root',
template: `
<div
[ngStyle]="{ 'background-color': isSuccess ? 'yellow' : 'green',
'font-size': isSuccess ? '24px' : '20px' }">
This div's background color and font size change based
on the value of isSuccess.
</div>
`
})
export class AppComponent {
isSuccess: boolean = true;
}
- In this example, the ngStyle directive applies a background color of yellow and a font size of 24px if isSuccess is true, and green and a font size of 24px if isSuccess is false. The isSuccess property should be defined in your component class.
Structural Directive Code Snippet Example
For NgIf:
@Component({ selector: 'app-root', template: ` <div *ngIf="isSuccess;"> Hello! </div> ` }) export class AppComponent { isSuccess: boolean = true; }
- In this case, since isSuccess is set to true, the "Hello!" message will be displayed. If you change isSuccess to false nothing will be display.
For NgSwitch:
@Component({
selector: 'app-root',
template: `
<div [ngSwitch]="color">
<div *ngSwitchCase="'red'">Red color is selected</div>
<div *ngSwitchCase="'blue'">Blue color is selected</div>
<div *ngSwitchCase="'green'">Green color is selected</div>
<div *ngSwitchDefault>No color is selected</div>
</div>
`
})
export class AppComponent {
color: string = 'green';
}
- In this example, since the color is set to 'green', the message "Green color is selected" will be displayed. If you change the color to 'red' or 'blue', the corresponding message will be displayed instead. If the color is set to a value that is not 'red', 'blue', or 'green', the message "No color is selected" will be displayed as the default case.
For NgFor:
@Component({
selector: 'app-root',
template: `
<div>
<ng-container *ngFor="let user of users">
<p>
{{ user.name }} - {{ user.email }}
</p>
</ng-container>
</div>
`
})
export class AppComponent {
users = [
{ "id": 1, "name": "Leanne Graham", "email": "leanne@april.biz" },
{ "id": 2, "name": "Ervin Howell", "email": "ervin@melissa.tv" },
{ "id": 3, "name": "Clementine Bauch", "email": "clementine@yesenia.net"},
];
}
- In this example, we use a users array with objets inside and this arrays with objects use to make a paragraph in the template component it makes the user name and user email.
References
https://angular.dev/guide/directives
https://www.angularminds.com/blog/directives-in-angular
https://medium.com/angular-in-depth/having-fun-with-structural-directives-in-angular-69b4d229ad93
https://brandonclapp.com/angular-structural-directives
https://www.scholarhat.com/tutorial/angular/angular-directives-example
Subscribe to my newsletter
Read articles from Cañete,Brandon L. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
