Avoiding Default Exports: A Guide for JavaScript Developers

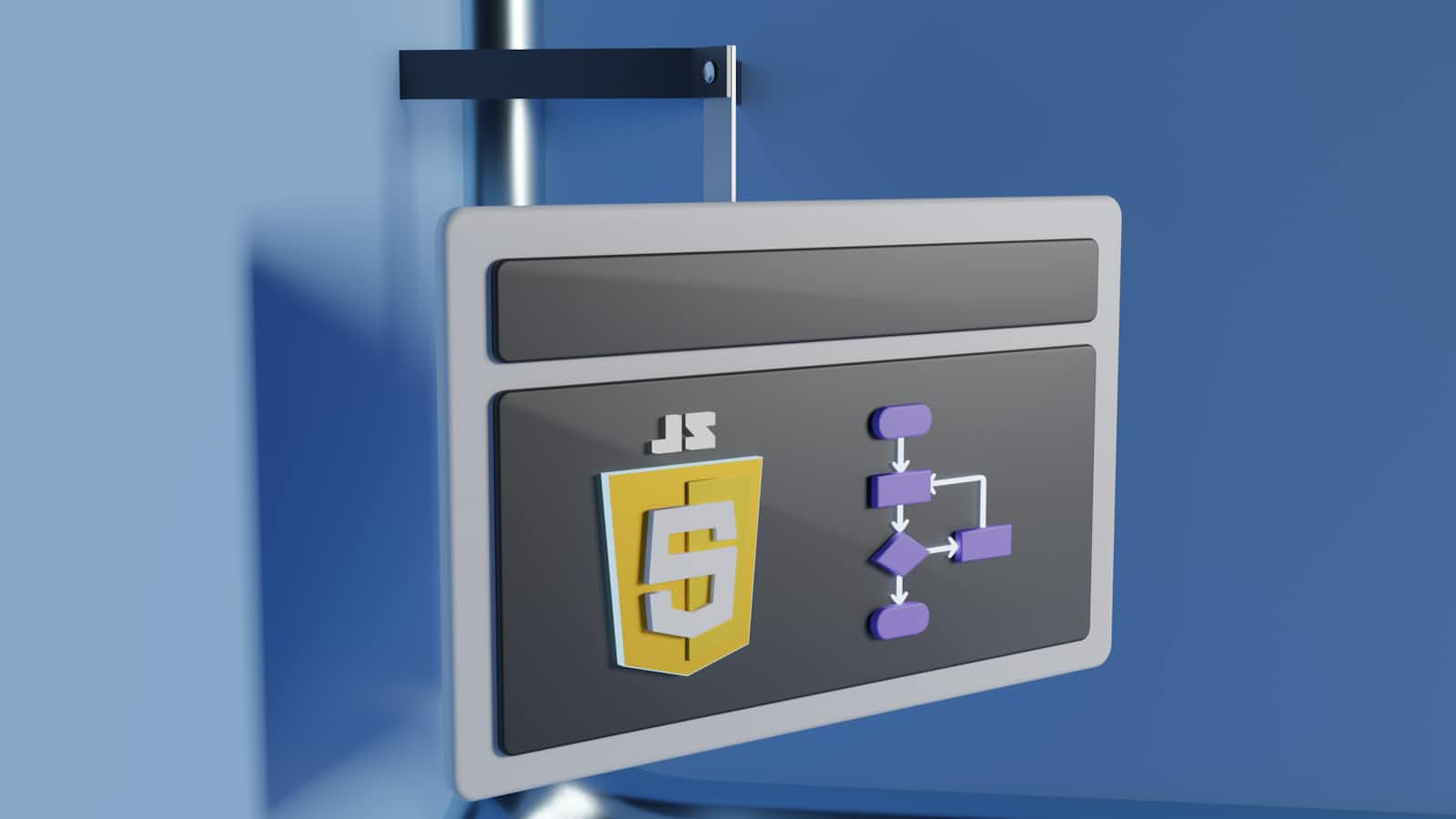
What are Default Exports in JavaScript Modules?
Default exports allow you to export a single value from a module that can be imported with any name.
This value is the default export for the module and can be any type, such as a function, object
, or primitive value. Here’s an example of a module that exports a default function
Let’s imagine that we have a math.js
file with the following operation.
// math.js
export default function subtract(a, b) {
return a - b;
}
In this example, the subtract function is the default export for the math module. It can be imported with any name in another file, like this 👇
// app.js
import add from './math.js'
const result = add(2, 2);
console.log(result) // return 0
Notice that we imported the subtract function as addition. This is possible because default exports can be imported with any name. This can lead to confusion and make code harder to maintain as a codebase grows.
Alternatives to Default Exports is Named Exports
Named exports are a good alternative to default exports. With named exports, you can export multiple values from a module and give each one a clear and consistent name. This makes it easier for other developers to understand and use your code.
Here’s an example of a module that exports two named functions:
// math.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
In this example, we exported two named functions, add and subtract. These functions can be imported in another file like this:
// app.js
import { add, subtract } from './math';
const sum = add(2, 2);
console.log(sum); // returns 4
const difference = subtract(2, 2);
console.log(dfference); // returns 0
Notice that we imported the functions using their names, which makes the code easier to understand and maintain.
Best Practices
There are some other best practices you can follow to improve the maintainability of your code:
Use consistent naming conventions for your exports. This will make it easier for other developers to understand and use your code.
Avoid exporting too many values from a single module. This can make your code harder to understand and maintain.
Subscribe to my newsletter
Read articles from Shivam Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivam Sharma
Shivam Sharma
I’m an open source enthusiast and I am passionate Web Development, DevOps & I enjoy learning new things.