Exploring the Internals of C# Reflection and Metadata
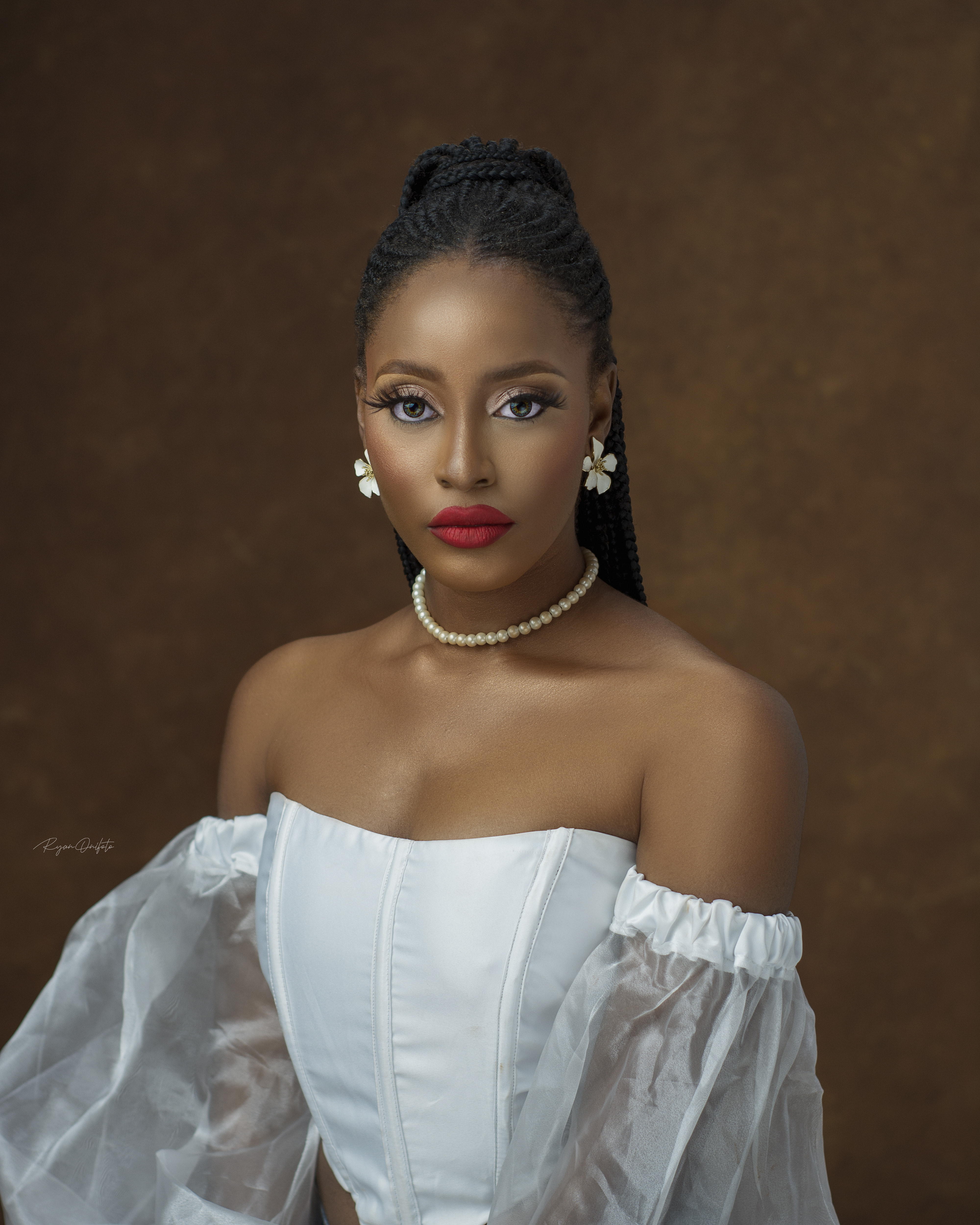
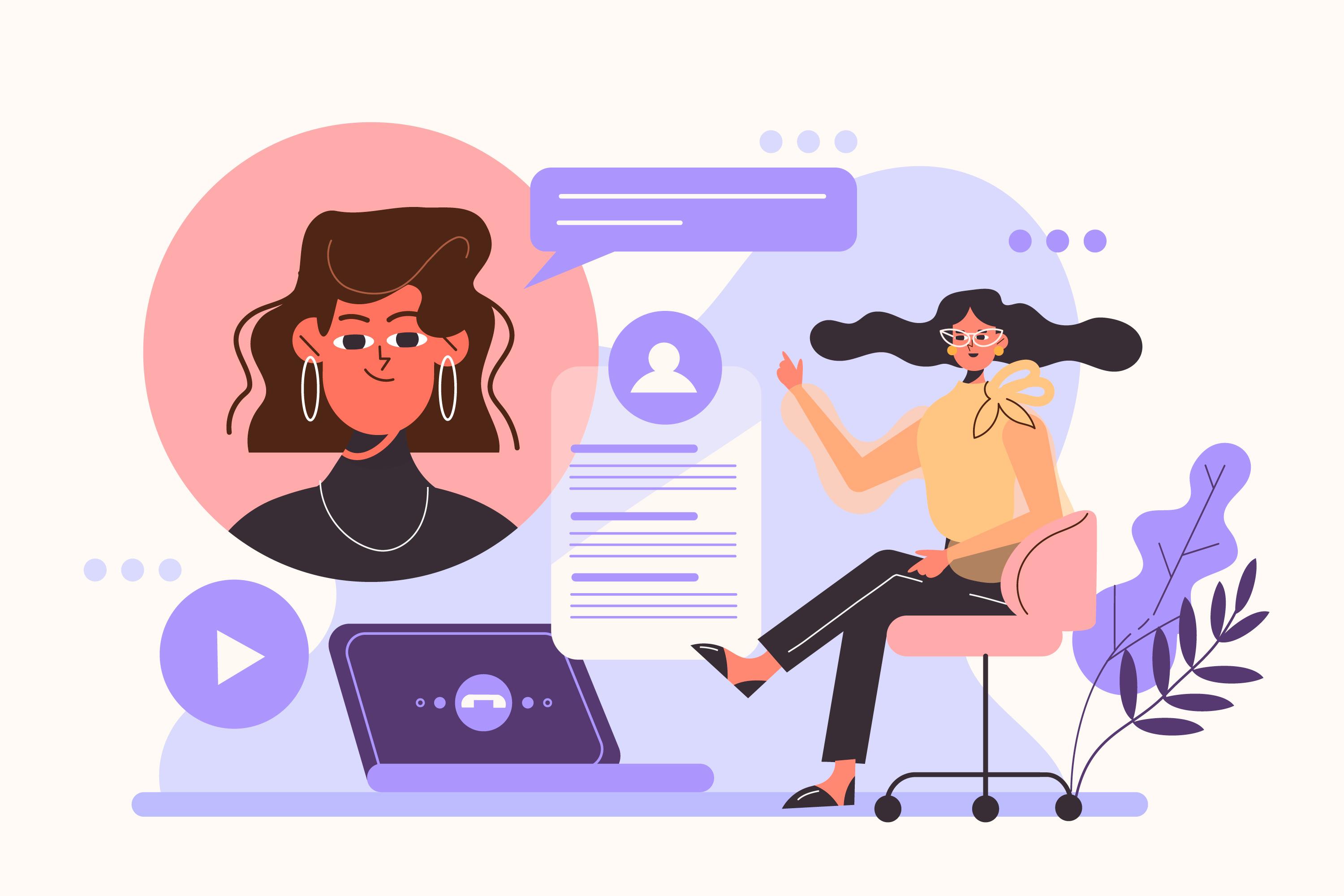
Hello, and Welcome again to another installment in the Mastering C# series. Today, I will walk you through a step-by-step guide to understanding C# Reflection and Metadata.
C# Reflection allows you to inspect and interact with code at runtime. Simply put, it lets you look inside your code while it’s running—so you can see information about types (classes, methods, properties, etc.) and even modify or call them dynamically.
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Understanding of C#
Familiarity with core C# concepts like classes, objects, methods, properties, and access modifiers is essential for understanding how Reflection interacts with these elements.Object-Oriented Programming (OOP) Principles
Knowledge of OOP concepts such as inheritance, polymorphism, encapsulation, and abstraction will help you grasp how Reflection manipulates types at runtime.Familiarity with .NET Runtime
A basic understanding of how the .NET runtime works, including assemblies, the Common Language Runtime (CLR), and the purpose of metadata.Working Knowledge of Visual Studio (or any C# IDE)
Experience with Visual Studio or any other C# IDE, along with compiling and running C# projects, will be necessary to follow along with the examples in the guide.Comfort with Debugging Tools
Being familiar with using debugging tools in Visual Studio (or any C# IDE) will help in exploring the results of reflection and inspecting runtime behavior.
Table of Contents
Introduction to C# Reflection
How Reflection Works Under the Hood
Practical Uses of Reflection
Performance Implications
Best Practices for Working with Reflection
Conclusion and Additional Resources
Introduction to C# Reflection
C# Reflection allows you to inspect and interact with code at runtime. Simply put, it lets you look inside your code while it’s running—so you can see information about types (classes, methods, properties, etc.) and even modify or call them dynamically.
What is Reflection?
Reflection is a way to "reflect" on the code. With Reflection, you can:
Inspect the structure of types (like classes and methods).
Access metadata (information about your code).
Invoke methods or create instances of classes dynamically.
Imagine you have a toolbox. Normally, you use tools from the box without checking them. With Reflection, you open the box and inspect every tool, checking what it can do.
Basic Concepts of Metadata in C#
Metadata is data about the structure of your code. In C#, metadata includes things like:
Class names
Method signatures
Property types
Attributes applied to code elements
Reflection allows you to read this metadata at runtime.
Simple Example of Reflection in C#
Let’s start with a basic example: inspecting a class using Reflection.
using System;
using System.Reflection;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void SayHello()
{
Console.WriteLine($"Hello, my name is {Name}");
}
}
class Program
{
static void Main()
{
// Create an instance of the Person class
Person person = new Person { Name = "Alice", Age = 25 };
// Use Reflection to get type information about the Person class
Type personType = person.GetType();
// Display the class name
Console.WriteLine("Class: " + personType.Name);
// Display properties of the class
Console.WriteLine("Properties:");
foreach (PropertyInfo prop in personType.GetProperties())
{
Console.WriteLine(prop.Name + " (" + prop.PropertyType.Name + ")");
}
// Display methods of the class
Console.WriteLine("Methods:");
foreach (MethodInfo method in personType.GetMethods())
{
Console.WriteLine(method.Name);
}
}
}
What’s Happening Here?
We created a class
Person
with two properties (Name
andAge
) and a methodSayHello()
.Using
person.GetType()
, we used Reflection to get the type information of thePerson
class at runtime.We displayed the class name, properties, and methods.
Output:
Class: Person
Properties:
Name (String)
Age (Int32)
Methods:
get_Name
set_Name
get_Age
set_Age
SayHello
This simple example shows how Reflection can be used to inspect the structure of a class at runtime.
Why Use Reflection?
Reflection is useful when:
You don’t know the type at compile time (e.g., dynamic plugins or libraries).
You need to inspect or manipulate objects at runtime (e.g., for testing frameworks or object mappers).
Note: Performance Impact
Reflection is powerful, but it can slow down your program if overused because it involves inspecting the runtime structure of objects. Always use it carefully in performance-critical applications.
How Reflection Works Under the Hood
Reflection in C# is a powerful feature that allows you to inspect and interact with the metadata of types (classes, methods, properties, etc.) at runtime. It's like looking inside the structure of your code while it's running, enabling you to create instances of types, invoke methods, and access fields dynamically without knowing them beforehand.
Understanding How C# Interacts with Metadata
When you compile a C# program, it gets converted into Intermediate Language (IL) code. Along with the code, metadata (data about the types, methods, and properties) is stored inside the assembly (DLL or EXE). Reflection allows you to access and manipulate this metadata during runtime.
Here's a simple analogy: Imagine you have a box (your assembly), and the box contains different objects (your classes, methods, and properties). With Reflection, you're able to open the box and see what's inside, and even interact with those objects.
Key Components Involved in Reflection
Type
TheType
class is the core part of Reflection. It provides information about any type (class, interface, enum, etc.) in C#.Assembly
TheAssembly
class represents the compiled code (EXE or DLL). It holds all the types and metadata related to the program.MethodInfo, PropertyInfo, FieldInfo
These classes represent methods, properties, and fields of a type, allowing you to get information or interact with them.
Example 1: Getting Type Information Using Reflection
Here’s a basic example where we use Reflection to inspect the metadata of a class at runtime.
using System;
using System.Reflection;
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void Greet()
{
Console.WriteLine("Hello! My name is " + Name);
}
}
class Program
{
static void Main(string[] args)
{
// Get the Type of the class 'Person'
Type personType = typeof(Person);
// Print the type name
Console.WriteLine("Class Name: " + personType.Name);
// Get and print properties of the class
Console.WriteLine("Properties:");
foreach (PropertyInfo prop in personType.GetProperties())
{
Console.WriteLine(prop.Name + " - " + prop.PropertyType);
}
// Get and print methods of the class
Console.WriteLine("\nMethods:");
foreach (MethodInfo method in personType.GetMethods())
{
Console.WriteLine(method.Name);
}
}
}
Explanation:
We use
typeof(Person)
to get theType
of thePerson
class.GetProperties()
fetches all properties of thePerson
class.GetMethods()
fetches all methods, includingGreet
and other default methods.
Output:
Class Name: Person
Properties:
Name - System.String
Age - System.Int32
Methods:
get_Name
set_Name
get_Age
set_Age
Greet
ToString
Equals
GetHashCode
GetType
Example 2: Invoking Methods Dynamically Using Reflection
Reflection allows you to dynamically invoke methods without directly referencing them in your code. Here’s how:
class Program
{
static void Main(string[] args)
{
// Create an instance of the Person class
Person person = new Person();
person.Name = "Alice";
person.Age = 25;
// Get the Type
Type personType = typeof(Person);
// Get the Greet method info
MethodInfo greetMethod = personType.GetMethod("Greet");
// Invoke the Greet method on the person instance
greetMethod.Invoke(person, null);
}
}
Explanation:
GetMethod("Greet")
retrieves the method metadata for theGreet
method.Invoke(person, null)
calls theGreet
method on theperson
object.
Output:
Hello! My name is Alice
Key Takeaways:
Reflection is used to inspect types, properties, methods, and fields at runtime.
Type, MethodInfo, and PropertyInfo are key components for interacting with metadata.
You can dynamically create instances, invoke methods, and modify properties using Reflection, making your code more flexible.
Reflection is powerful but should be used carefully as it can affect performance and make your code harder to debug.
Practical Uses of Reflection
Dynamically Inspecting and Modifying Types at Runtime
Reflection allows you to inspect the details of types (like classes, methods, properties, etc.) at runtime. This is useful when you don't know the structure of the object beforehand.
Example: Inspecting a class structure at runtime
using System;
using System.Reflection;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void Greet() => Console.WriteLine($"Hello, my name is {Name}");
}
class Program
{
static void Main()
{
Type personType = typeof(Person); // Get the 'Person' type
Console.WriteLine("Properties of Person class:");
// Inspect the properties of the class
foreach (PropertyInfo prop in personType.GetProperties())
{
Console.WriteLine(prop.Name);
}
}
}
Output:
Properties of Person class:
Name
Age
This example shows how Reflection can be used to inspect the properties of a class at runtime.
Creating Objects Dynamically Using Reflection
You can use Reflection to create objects dynamically without knowing their type at compile time. This is helpful when you need to create instances of classes based on user input or external data.
Example: Creating an instance of a class dynamically
using System;
using System.Reflection;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void Greet() => Console.WriteLine($"Hello, my name is {Name}");
}
class Program
{
static void Main()
{
Type personType = typeof(Person);
// Dynamically create an instance of the 'Person' class
object personInstance = Activator.CreateInstance(personType);
// Set property values using reflection
personType.GetProperty("Name").SetValue(personInstance, "Alice");
personType.GetProperty("Age").SetValue(personInstance, 30);
// Call a method on the object
personType.GetMethod("Greet").Invoke(personInstance, null);
}
}
Output:
Hello, my name is Alice
In this example, we dynamically create an instance of the Person
class, set its properties, and call its Greet
method using Reflection.
Accessing Methods, Properties, and Fields Using Reflection
Reflection allows you to access (and even modify) methods, properties, and fields of objects dynamically.
Example: Accessing and modifying fields using Reflection
using System;
using System.Reflection;
public class Person
{
public string Name;
private int age;
public Person(string name, int age)
{
this.Name = name;
this.age = age;
}
public void DisplayInfo()
{
Console.WriteLine($"Name: {Name}, Age: {age}");
}
}
class Program
{
static void Main()
{
Person person = new Person("Bob", 25);
Type personType = person.GetType();
// Access and modify the private 'age' field
FieldInfo ageField = personType.GetField("age", BindingFlags.NonPublic | BindingFlags.Instance);
ageField.SetValue(person, 30);
// Call the method to display the modified info
person.DisplayInfo();
}
}
Output:
Name: Bob, Age: 30
In this example, we use Reflection to access and modify the private field age
, even though it's not directly accessible.
Reflection is a powerful feature in C# that allows you to dynamically inspect, create, and modify types at runtime. It can be used in scenarios like plugin systems, dynamically loading assemblies, or even building your own development tools.
Performance Implications of Reflection
Reflection is powerful, but it can slow down your application if not used wisely. Here's why:
How Reflection Affects Performance:
Reflection allows your code to inspect and modify types (classes, methods, properties) at runtime, but this dynamic behavior comes at a cost. Reflection is slower than normal method calls because the system has to do extra work to find and execute methods or access properties.Example:
using System; using System.Reflection; class Program { static void Main() { // Regular method call MyClass myClass = new MyClass(); myClass.SayHello(); // Method call using reflection Type type = typeof(MyClass); MethodInfo method = type.GetMethod("SayHello"); method.Invoke(myClass, null); // Slower due to reflection } } class MyClass { public void SayHello() { Console.WriteLine("Hello, world!"); } }
What's happening?
The regular method call is direct and fast.
The reflection-based method call (
Invoke
) is slower because the runtime has to look up the method by name, verify it, and then call it.
Best Practices to Minimize Performance Overhead:
Avoid Reflection in Performance-Critical Code:
If you need to call methods or access properties frequently (e.g., inside loops or real-time systems), avoid using reflection as it can degrade performance.// Avoid reflection in a loop or critical performance sections for (int i = 0; i < 1000000; i++) { method.Invoke(myClass, null); // Bad idea }
Cache Reflection Results:
You can reduce the performance hit by caching the results of reflection lookups. Instead of repeatedly looking up the same method or property, store it in a variable and reuse it.// Cache the method info MethodInfo cachedMethod = typeof(MyClass).GetMethod("SayHello"); // Reuse cached method for (int i = 0; i < 1000000; i++) { cachedMethod.Invoke(myClass, null); // Faster due to caching }
Use Reflection Only When Necessary:
Use reflection only for scenarios where dynamic behavior is necessary, like when you're working with plugins, frameworks, or libraries that need to load types at runtime. For most cases, stick to regular method calls.
Conclusion:
Reflection is a useful tool but can slow down your application if used improperly. The key is to limit its use, cache the results when possible, and avoid using it in performance-critical areas. This approach allows you to benefit from Reflection's power while keeping your application running efficiently.
Best Practices for Working with Reflection
When to Use Reflection
Reflection is a powerful feature in C# that allows you to inspect and interact with types (classes, interfaces, etc.) at runtime. Here are some scenarios where Reflection is beneficial:
Dynamic Type Inspection: When you need to examine an object's properties or methods dynamically without knowing its type at compile time.
Creating Instances of Types: If you need to create instances of types based on their names (e.g., plugins or factories).
Accessing Attributes: When you want to retrieve custom attributes associated with types, methods, or properties.
Code Sample: Inspecting an Object
using System;
using System.Reflection;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public void Greet()
{
Console.WriteLine($"Hello, my name is {Name}.");
}
}
public class Program
{
public static void Main()
{
Person person = new Person { Name = "Alice", Age = 30 };
// Get the type of the person object
Type type = person.GetType();
// Display properties
Console.WriteLine("Properties:");
foreach (PropertyInfo prop in type.GetProperties())
{
Console.WriteLine($"{prop.Name}: {prop.GetValue(person)}");
}
// Invoke a method
MethodInfo greetMethod = type.GetMethod("Greet");
greetMethod.Invoke(person, null);
}
}
Alternatives to Reflection When Possible
While Reflection is powerful, it can be slower and more complex than traditional approaches. Here are some alternatives:
Generics: Use generics for type-safe code that avoids runtime type inspection.
Interfaces: Use interfaces to define contracts for your classes without needing Reflection to inspect types.
Attributes: Instead of reflecting on attributes, consider using dependency injection or configuration files where applicable.
Code Sample: Using Generics
using System;
public class GenericPrinter<T>
{
public void Print(T item)
{
Console.WriteLine(item);
}
}
public class Program
{
public static void Main()
{
GenericPrinter<string> stringPrinter = new GenericPrinter<string>();
stringPrinter.Print("Hello, World!");
GenericPrinter<int> intPrinter = new GenericPrinter<int>();
intPrinter.Print(42);
}
}
Summary
While Reflection can be a powerful tool in your C# toolbox, it's important to use it wisely. Understanding when to use Reflection and knowing the alternatives can help you write more efficient and maintainable code. Stick to simpler approaches when they fit your needs to avoid the performance overhead of Reflection.
Conclusion
In this guide, we explored the fascinating world of C# Reflection and Metadata. We learned how Reflection allows us to inspect and interact with types and objects at runtime, giving us powerful capabilities such as dynamically creating instances, invoking methods, and accessing properties.
Reflection is a valuable tool, but it's essential to use it judiciously, as it can impact performance. By understanding the underlying principles, we can make informed decisions about when and how to use Reflection in our applications.
Additional Resources
To further your understanding of Reflection and enhance your C# skills, check out these resources:
Official Microsoft Documentation
The official documentation is a great place to learn more about Reflection and its capabilities. Visit: C# Reflection DocumentationTutorials and Articles
Look for tutorials on popular coding websites like CodeProject or Medium for practical examples and deeper dives into Reflection.Video Tutorials
Platforms like YouTube offer video tutorials that walk you through the basics of Reflection and its applications.Books on C#
Consider books like "C# in Depth" by Jon Skeet or "Pro C# 9 with .NET 5" by Andrew Troelsen for more extensive coverage of C# features, including Reflection.
By leveraging the concepts of Reflection, you can create more dynamic and flexible applications. With the additional resources provided, you can continue your journey in mastering C# and its powerful features. I hope you found this guide helpful and learned something new. Stay tuned for the next article in the Mastering C# series: Advanced Memory Management Techniques in C#
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
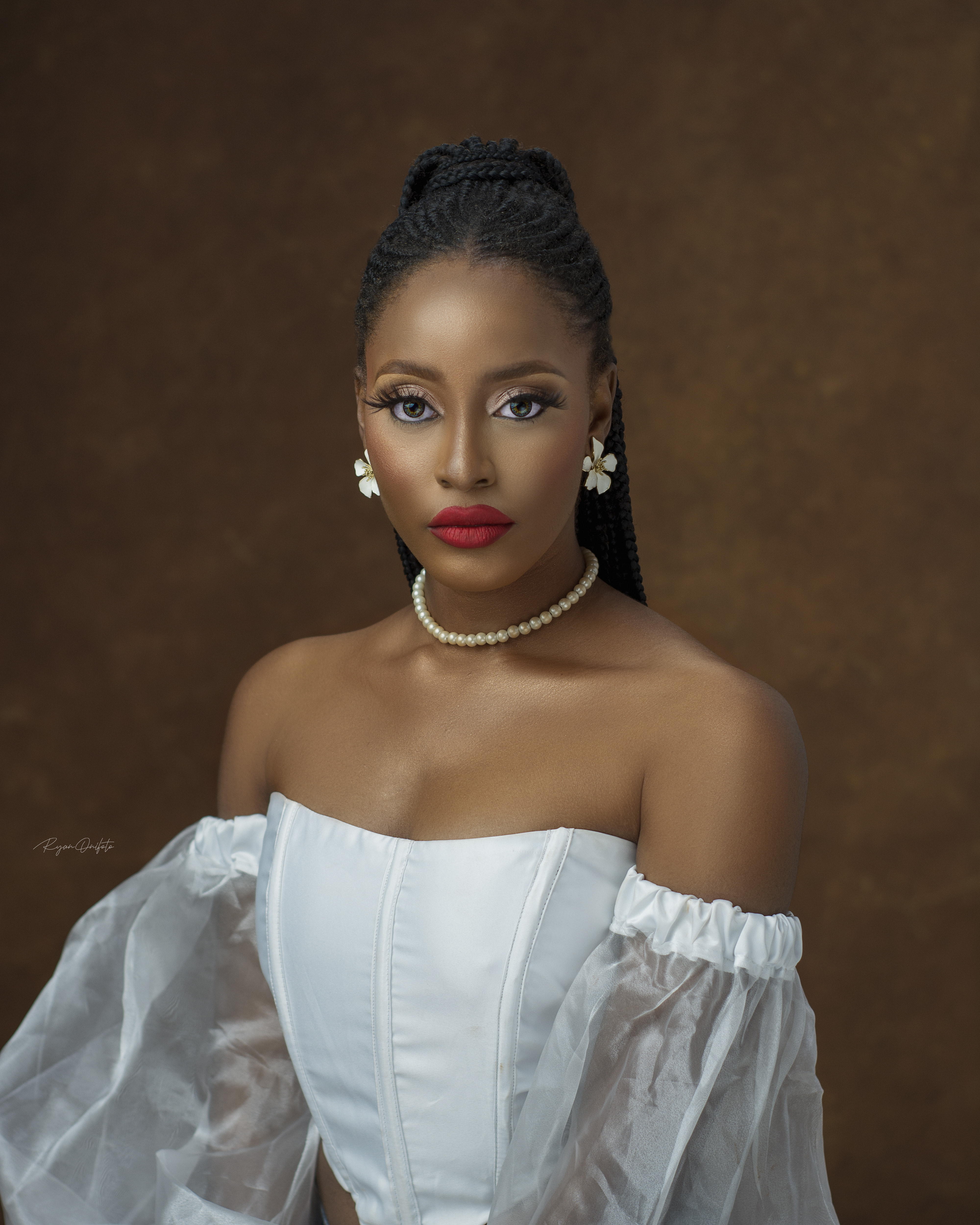