[Detialed Guide] How to Password Protect a Word Document in Python without Hassle

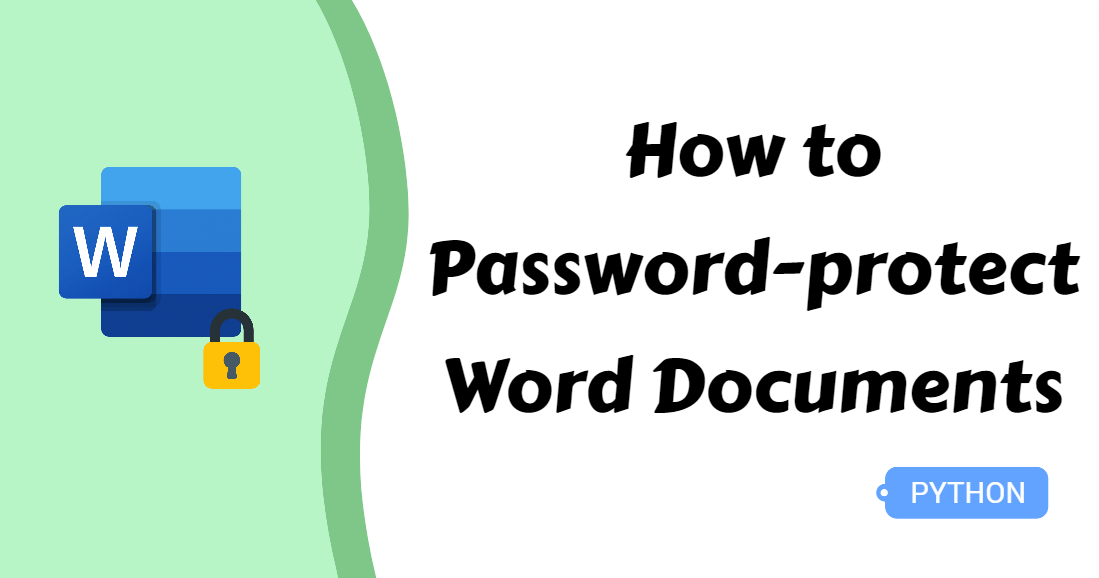
Although modern electronic documents emphasize that they are easy to access and edit, document security should not be ignored, especially when dealing with Word documents containing sensitive or confidential information. When sending Word files over the internet, there are many points where data can be leaked unless you encrypt the document beforehand.
This article will demonstrate how to password protect a Word document in Python, helping you easily add a password, modify the password, and eventually improve work efficiency.
Prepare for the Task
To protect a Word document with a password, this blog recommends Spire.Doc for Python. It is a professional component that allows you to create, edit, convert MS Word documents, etc. This tool helps you to finish multiple tasks without installing Microsoft Office.
You can install Spire.Doc for Python from PyPI using the following pip command:
pip install Spire.Doc
How to Set Password Protection for Word Documents
Assuming you are fully ready, let’s get down to the business, how to password-protect a Word document. Securing sensitive data in a Word document is vital in business and other fields, for example, client data, finance budget, and so on. This section will introduce how to make a Word document password-protected in Python, providing specific steps and a code example.
Steps to protect a Word document with a password:
Import needed modules.
Create an object of the Document class.
Load the Word document to be encrypted from the disk using the Document.LoadFromFile() method.
Set the password for the document by calling the Document.Encrypt() method.
Save the protected file as a new Word document with the Document.SaveToFile() method and release the resource.
Below is the code example for protecting a Word document with a password:
from spire.doc import *
from spire.doc.common import *
# Create an instance of the Document class
doc = Document()
# Load a Word document from the disk
doc.LoadFromFile("/sample.docx")
# Protect the Word document with a password
doc.Encrypt("password")
# Save protected document as a Word file
doc.SaveToFile("/EncryptedDocument.docx")
# Release resources
doc.Close()
How to Password Protect a Word Document: Changing Password
There may be times when you wish to change the password. It can be done with Python as well. The step-by-step instructions are just as follows:.
Steps to change the password of a Word document:
Import essential modules.
Create an instance of the Document class.
Specify the file path of the source Word document using the Document.LoadFromFile() method.
Change the password for the document with the Document.Encrypt() method.
Calling the Document.SaveToFile() method to save the updated document and release resources.
Below is an example of changing the password of a Word document:
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load an encrypted Word document
doc.LoadFromFile("/EncryptedDocument.docx", FileFormat.Docx, "password")
# Change the password of the Word document
doc.Encrypt("password1")
# Save the document as a new file
doc.SaveToFile("/ChangeDocument.docx")
# Release the resource
doc.Close()
How to Remove Password from Word Documents without Effort
If the password of a Word document is no longer needed for some reasons, such as the content is now available for public viewing, the confidentiality period has expired, or you may simply forget the password, unlocking it can save you the hassle of repeatedly entering credentials.
In this section, you will learn how to remove a password from a Word document in Python, making these documents accessible without restrictions.
Steps to remove a Word document password:
Import needed modules.
Instantiate a Document class.
Load a Word document that is password-protected using the Document.LoadFromFile() method.
Remove the password from the Word document with the Document.RemoveEncryption() method.
Save the decrypted document to the disk using the Document.SaveToFile() method
Release the resource.
Here is a code example of removing the password from a Word document:
from spire.doc import *
from spire.doc.common import *
# Instantiate a Document class
doc = Document()
# Load a password protected Word document
doc.LoadFromFile("output/EncryptedDocument.docx", FileFormat.Auto, "password")
# Remove the password from the document
doc.RemoveEncryption()
# Write the document to the disk
doc.SaveToFile("output/RemovePassword.docx", FileFormat.Docx)
# Release resources
doc.Close()
The Bottom Line
This blog illustrates how to password-protect a Word document using Python. And, to make the process as a whole, the article explores how to change a password or remove a password from a Word document as well. We hope you can find encrypting and decrypting Word documents is a piece of cake after reading this blog!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
