Java Constructors
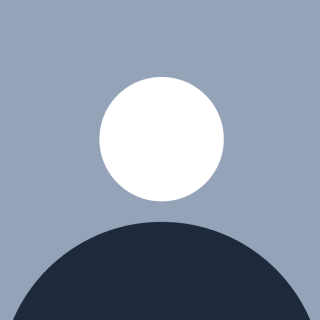
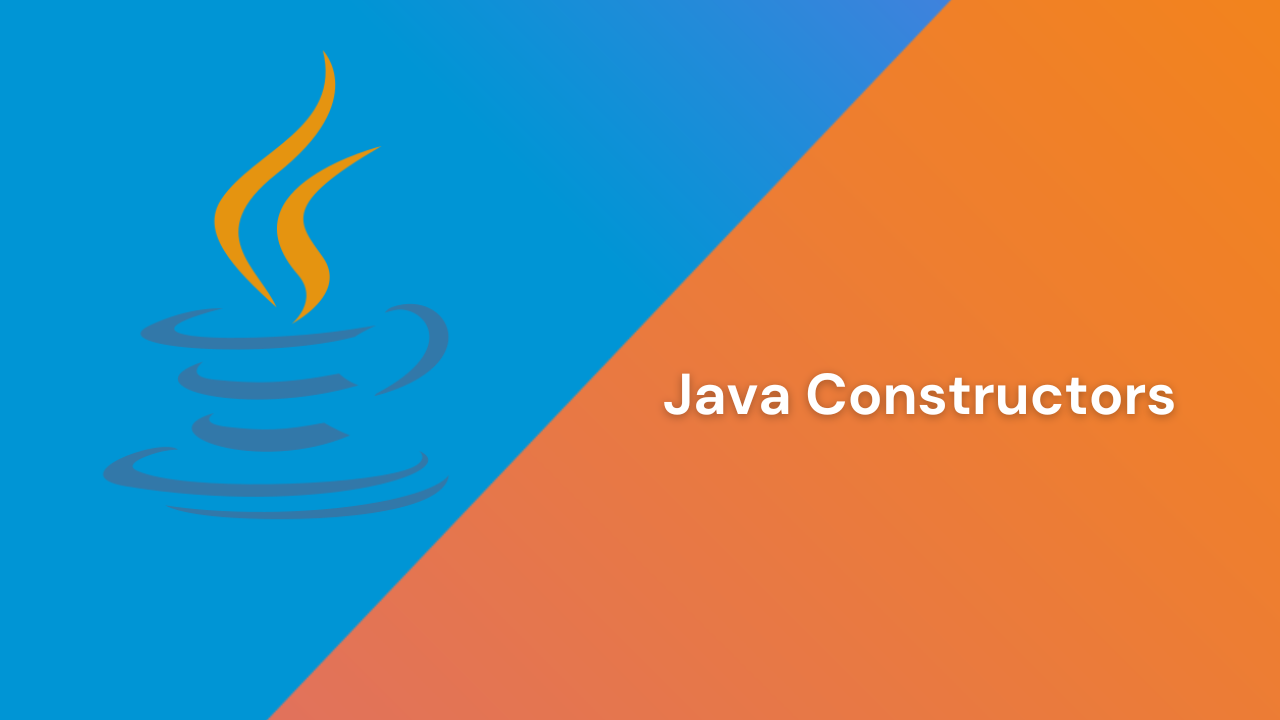
Constructors in Java are special methods used to initialize objects. They are called when an instance of a class is created. Constructors have the same name as the class and do not have a return type.
Rules to Create Constructors
The constructor name must be the same as the class name.
Constructors do not have a return type.
A constructor is called when an object of a class is created.
If no constructor is defined, Java provides a default constructor.
A No-Argument Constructor
A no-argument constructor, also known as a default constructor, is a constructor that does not take any parameters. It is used to create an object with default values. Here is a detailed explanation:
Purpose of No-Argument Constructor
The primary purpose of a no-argument constructor is to initialize an object. When an object is created, the no-argument constructor is called to set up the initial state of the object.
If no specific values are provided during object creation, the no-argument constructor initializes the object with default values. This is useful when you want to create an object without needing to specify any initial values.
Example
A class Example
with a no-argument constructor:
public class Example {
public Example() {
// No-argument constructor
}
}
In this example, the Example
class has a no-argument constructor. When an instance of the Example
class is created using the new
keyword, this constructor is called:
Example example = new Example();
The constructor must have the same name as the class. In this case, the constructor is named
Example
, which matches the class name.The constructor does not take any parameters, hence the term "no-argument".
Constructors do not have a return type, not even
void
.If no constructors are explicitly defined in a class, Java automatically provides a default no-argument constructor. However, if any constructor is defined, the default constructor is not provided automatically.
No-argument constructors are often used in scenarios where objects need to be created with default settings or when the specific values for the object's attributes are not known at the time of creation. They are also essential for frameworks and libraries that rely on reflection to create objects, such as JavaBeans, Hibernate, and Spring.
Example with Default Values
An example where a no-argument constructor initializes an object with default values:
public class Person {
private String name;
private int age;
// No-argument constructor
public Person() {
this.name = "Unknown";
this.age = 0;
}
// Getters and setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person();
System.out.println("Name: " + person.getName()); // Output: Name: Unknown
System.out.println("Age: " + person.getAge()); // Output: Age: 0
}
}
In this example, the Person
class has a no-argument constructor that initializes the name
attribute to "Unknown" and the age
attribute to 0. When a Person
object is created using the no-argument constructor, these default values are assigned.
Default and Parameterized Constructors
Default Constructor
A default constructor is provided by Java if no constructors are explicitly defined in the class. It initializes the object with default values.
public class Example {
// Default constructor provided by Java
}
Example:
public class Example {
private int value;
// Default constructor provided by Java
}
public class Main {
public static void main(String[] args) {
Example example = new Example(); // Calls the default constructor
System.out.println(example.value); // Outputs 0 (default value for int)
}
}
Parameterized Constructor
A parameterized constructor is a constructor that takes one or more parameters. It is used to initialize an object with specific values.
public class Example {
private int value;
public Example(int value) {
this.value = value;
}
}
Example:
public class Example {
private int value;
public Example(int value) {
this.value = value;
}
}
public class Main {
public static void main(String[] args) {
Example example = new Example(10); // Calls the parameterized constructor
System.out.println(example.value); // Outputs 10
}
}
Constructor Chaining with this() and super()
Constructor chaining is the process of calling one constructor from another constructor within the same class or from the parent class.
Calling Same Class’s Constructors with this()
The this()
keyword is used to call another constructor from the same class. This is useful when you have multiple constructors and want to avoid duplicating code. By chaining constructors, you can centralize common initialization logic in one place.
public class Example {
private int value;
public Example() {
this(0); // Calls the parameterized constructor
}
public Example(int value) {
this.value = value;
}
}
Calling Parent Class’s Constructors with super()
The super()
keyword in Java is used to call a constructor from the parent class. This is particularly useful in inheritance when you want to ensure that the parent class is properly initialized before the child class adds its own initialization logic.
Parent Class
public class Parent {
public Parent() {
// Parent class constructor
System.out.println("Parent class constructor called");
}
}
In this example, the Parent
class has a no-argument constructor. When an instance of the Parent
class is created, this constructor is called, and it prints a message indicating that the parent class constructor has been called.
Child Class
public class Child extends Parent {
public Child() {
super(); // Calls the parent class constructor
System.out.println("Child class constructor called");
}
}
The Child
class extends the Parent
class. In the Child
class constructor, the super()
keyword is used to call the constructor of the Parent
class. This ensures that the parent class is initialized before the child class. After calling super()
, the child class constructor continues its own initialization and prints a message indicating that the child class constructor has been called.
Example Output
When you create an instance of the Child
class:
public class Main {
public static void main(String[] args) {
Child child = new Child();
}
}
The output will be:
Parent class constructor called
Child class constructor called
Private Constructors
Private constructors in Java are used to restrict the instantiation of a class from outside the class itself. This is particularly useful in design patterns like the Singleton pattern, where you want to ensure that only one instance of the class is created and used throughout the application.
Singleton Design Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. Here’s a detailed explanation of how the Singleton pattern works using the provided code:
public class Singleton {
private static Singleton instance;
private Singleton() {
// Private constructor
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Explanation
Private Static Instance Variable
private static Singleton instance;
This variable holds the single instance of the
Singleton
class.It is declared as
static
so that it belongs to the class rather than any particular object.It is declared as
private
to prevent direct access from outside the class.
Private Constructor
private Singleton() { // Private constructor }
The constructor is private to prevent instantiation from outside the class.
This ensures that the only way to create an instance of the class is through the
getInstance
method.
Public Static Method (getInstance)
public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; }
This method provides a global point of access to the single instance of the class.
It checks if the
instance
isnull
. If it is, it creates a new instance of theSingleton
class.If the
instance
is notnull
, it simply returns the existing instance.This ensures that only one instance of the
Singleton
class is created, even if multiple threads try to access it simultaneously.
Example: Bicycle Gear
An example of a Bicycle
class with a gear attribute.
public class Bicycle {
private int gear;
// No-argument constructor
public Bicycle() {
this.gear = 1; // Default gear
}
// Parameterized constructor
public Bicycle(int gear) {
this.gear = gear;
}
// Method to display gear
public void displayGear() {
System.out.println("Gear: " + gear);
}
public static void main(String[] args) {
// Creating Bicycle objects using different constructors
Bicycle bike1 = new Bicycle();
Bicycle bike2 = new Bicycle(5);
bike1.displayGear(); // Output: Gear: 1
bike2.displayGear(); // Output: Gear: 5
}
}
Conclusion
Java constructors are special methods used to initialize objects. They have the same name as the class and no return type. There are no-argument constructors (default) and parameterized constructors. Constructor chaining can be done using this()
for the same class and super()
for the parent class. Private constructors are used in design patterns like Singleton to restrict instantiation.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer