The Essentials of Namespaces in C++ Explained
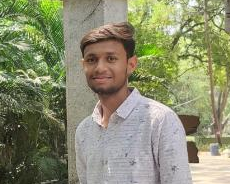
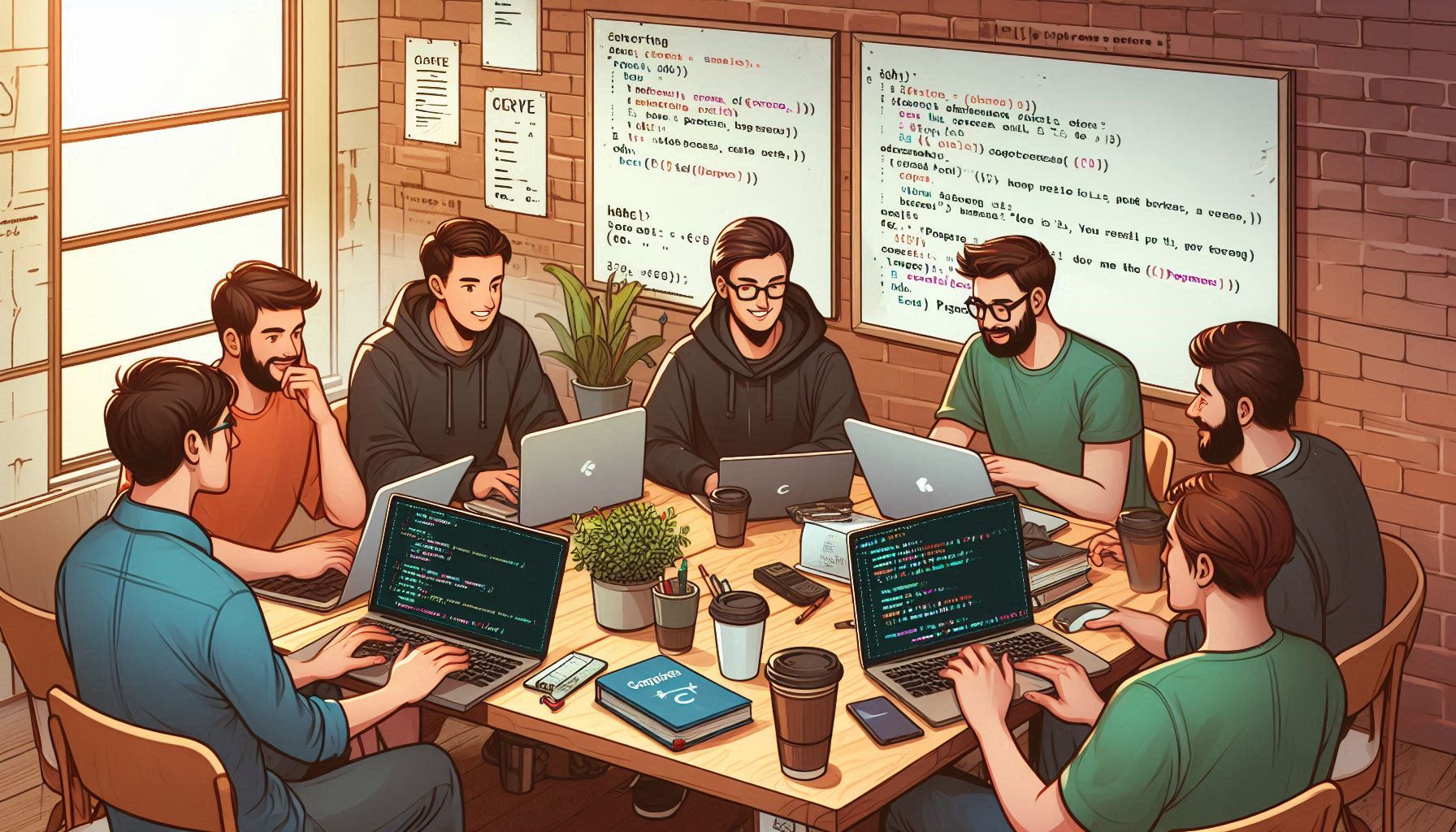
Introduction
In modern programming, codebases have grown in size and complexity, often involving thousands of lines of code and multiple modules. As a result, naming conflicts can become a serious issue. When multiple developers work on the same project or when integrating third-party libraries, it’s common to encounter functions, variables, or classes with the same names. This can lead to errors or ambiguous code behavior. To address this issue, C++ introduces a powerful feature known as namespaces.
In this blog, we’ll take a deep dive into what namespaces are, why they’re essential, how to use them effectively, and some best practices.
What is a Namespace?
A namespace in C++ is a declarative region that allows you to group identifiers (like variables, functions, and classes) under a unique name. The primary goal of namespaces is to avoid naming conflicts by organizing code into logical groups.
For example, if two different libraries provide a function named sort()
, you can use namespaces to differentiate between them, like std::sort()
for the Standard Library’s sort function and myLib::sort()
for your custom sorting implementation.
Syntax of Namespaces
The syntax for defining a namespace is quite simple:
namespace namespace_name {
// Code declarations go here
}
Example:
#include <iostream>
namespace first_space {
void func() {
std::cout << "Inside first_space" << std::endl;
}
}
namespace second_space {
void func() {
std::cout << "Inside second_space" << std::endl;
}
}
int main() {
first_space::func(); // Calls the function in first_space
second_space::func(); // Calls the function in second_space
return 0;
}
Why Use Namespaces?
Namespaces are essential for the following reasons:
Avoiding Naming Conflicts: In large projects or when integrating multiple libraries, two or more functions, variables, or classes may have the same name. Without namespaces, this would cause compiler errors. With namespaces, these conflicts can be easily avoided.
Organizing Code: Namespaces allow you to logically group related classes, functions, and variables. This improves code readability and maintainability by clearly showing which part of the code belongs to which functionality.
Reusability: You can define several namespaces across different projects without worrying about name collisions. This enhances code reusability.
Modularity: In software engineering, modularity is a key principle. Namespaces help divide code into smaller, logical units, facilitating easier debugging and development.
Using using
Keyword with Namespaces
Sometimes, repeatedly writing the namespace name can be tedious, especially if a namespace is frequently used. C++ provides the using
keyword to bring a namespace or specific identifiers from a namespace into the current scope.
Example:
#include <iostream>
namespace first_space {
void func() {
std::cout << "Inside first_space" << std::endl;
}
}
int main() {
using namespace first_space;
func(); // No need to prefix with namespace
return 0;
}
In this example, the using namespace first_space;
directive eliminates the need to prefix func()
with first_space::
.
However, be cautious when using the using
directive because it can sometimes lead to name collisions. If two namespaces contain the same function name, ambiguity will arise, and the compiler will throw an error.
Selective Use of using
Instead of importing the entire namespace, you can bring in just specific members:
using first_space::func;
This is a safer approach because it avoids polluting the global namespace with unnecessary identifiers.
Nested Namespaces
In large projects, it’s common to see nested namespaces, where one namespace is defined within another. This helps to create even finer logical groupings.
Example:
namespace outer_space {
namespace inner_space {
void display() {
std::cout << "Inside inner_space" << std::endl;
}
}
}
int main() {
outer_space::inner_space::display();
return 0;
}
From C++17 onwards, you can define nested namespaces more concisely:
namespace outer_space::inner_space {
void display() {
std::cout << "Inside inner_space" << std::endl;
}
}
Anonymous Namespaces
C++ also supports anonymous namespaces where the namespace has no name. This effectively means that any identifiers defined within that namespace are unique to the file where they are declared. Anonymous namespaces are used when you want to ensure that certain functions or variables are only accessible within a particular translation unit (source file).
Example:
namespace {
int hidden_variable = 10;
void hidden_function() {
std::cout << "This is hidden within the file" << std::endl;
}
}
int main() {
hidden_function(); // This works fine within the same file
std::cout << hidden_variable << std::endl;
return 0;
}
The anonymous namespace makes hidden_variable
and hidden_function
inaccessible from other translation units, helping to avoid external name conflicts.
Common Pitfalls and Best Practices
Overusing
using namespace
: Using the entire namespace can lead to ambiguity and reduce the clarity of your code. It is generally better to use specific names or limitusing
directives to the smallest scope possible.Avoid Namespace Pollution: Avoid bringing too many names into the global scope. Excessive use of
using
directives can lead to namespace pollution, making it harder to track where specific identifiers are coming from.Use Meaningful Namespaces: Namespaces should be descriptive and meaningful. For example, instead of using generic names like
ns1
, use names likenetworking
orgraphics
that reflect the purpose of the contained functions and classes.Consistent Grouping: Try to keep related functionality in the same namespace. For example, all database-related classes can be grouped under a
database
namespace, while networking functionality can be grouped under anetworking
namespace.
Conclusion
Namespaces are a critical feature in C++ that help to organize code, prevent naming conflicts, and improve code maintainability. As your projects grow in size and complexity, leveraging namespaces effectively becomes even more important. By understanding how to define, use, and manage namespaces, you can write cleaner, more scalable, and conflict-free code.
Happy coding!
Subscribe to my newsletter
Read articles from Darsh Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
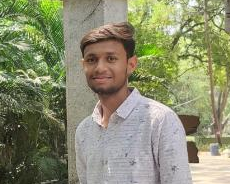
Darsh Patel
Darsh Patel
I'm a final-year student at PES University, specializing in Full-Stack Development and AI/ML. With hands-on experience in building web applications and working on machine learning models, I'm passionate to use it in real world. My academic journey has been a mixture of technical learning and practical application, where I've honed my skills in development, data analysis and artificial intelligence. When I’m not coding, you can find me exploring new trends, tinkering with projects, or sharing some insights here or maybe reading a newspaper.