Java Access Modifiers
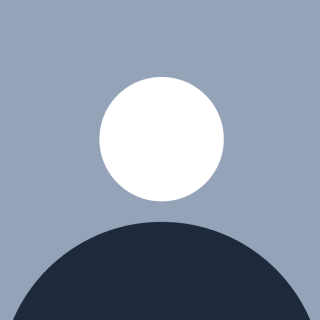
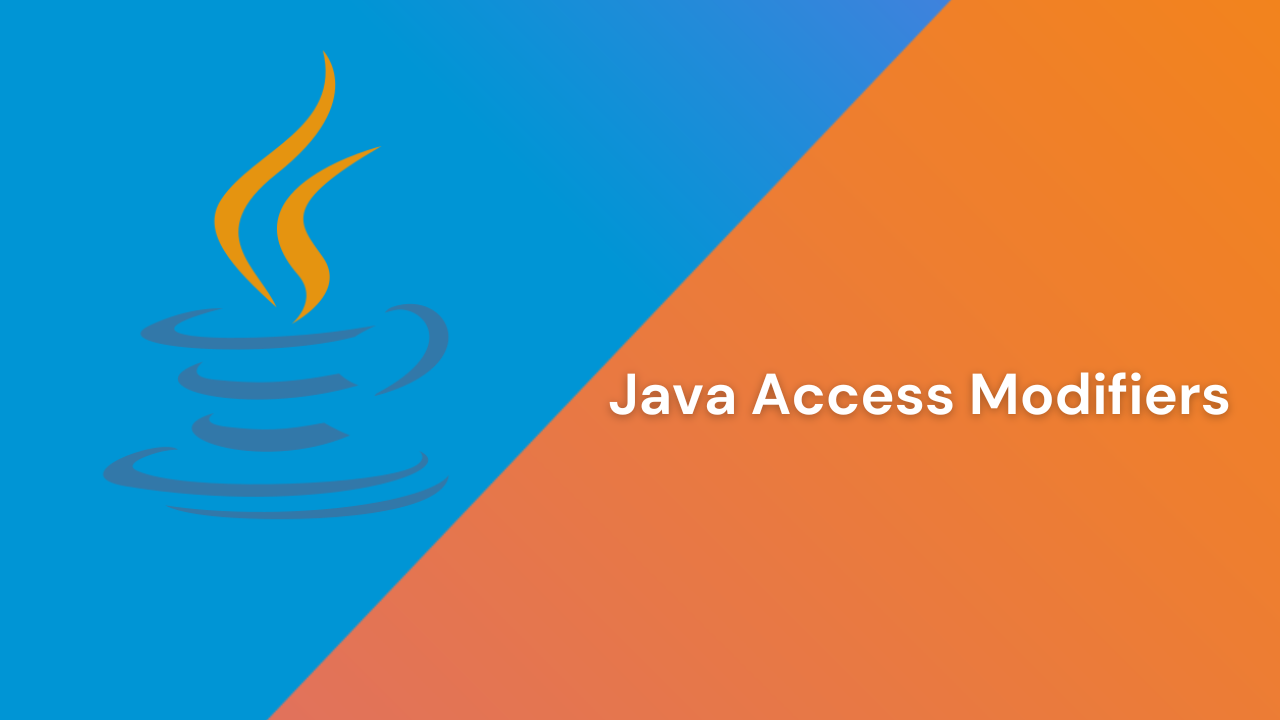
Access modifiers in Java are keywords that set the accessibility or scope of classes, methods, and other members. They are crucial for encapsulation, one of the core principles of object-oriented programming. Java provides four main access modifiers: public
, protected
, default
(no modifier), and private
. Each modifier determines the visibility of the member to other classes.
Public Access Modifier
The public
access modifier in Java is the most permissive access level. When a member (such as a class, method, or field) is declared as public
, it can be accessed from any other class, regardless of the package it belongs to. This means that there are no restrictions on the visibility of the member; it is accessible everywhere in the application.
public class PublicClass {
public int publicField;
public void publicMethod() {
// Method logic
}
}
public class PublicClass
: This declares a class namedPublicClass
with thepublic
access modifier. Because the class is public, it can be accessed from any other class in any package. This is useful when you want to create a class that should be universally accessible.public int publicField
: This declares an integer field namedpublicField
with thepublic
access modifier. Since the field is public, it can be accessed directly from any other class. For example:PublicClass obj = new PublicClass(); obj.publicField = 10; // Accessing the public field
public void publicMethod()
: This declares a method namedpublicMethod
with thepublic
access modifier. Since the method is public, it can be called from any other class.PublicClass obj = new PublicClass(); obj.publicMethod(); // Calling the public meth
Private Access Modifier
The private
access modifier in Java is the most restrictive level of access control. When a member (such as a field or method) is declared as private
, it can only be accessed within the class it is declared in. This means that no other class, not even subclasses or classes in the same package, can access these private members directly. This encapsulation is crucial for hiding the internal implementation details of a class and protecting the integrity of its data.
public class PrivateClass {
private int privateField;
private void privateMethod() {
// Method logic
}
}
The primary purpose of the
private
access modifier is to achieve encapsulation. By restricting access to the internal state and behavior of a class, you can control how the data is modified and accessed. This helps in maintaining the integrity of the data and prevents unintended interference from external classes.private int privateField
: This line declares an integer field namedprivateField
with theprivate
access modifier. Since it is private, it can only be accessed and modified within thePrivateClass
class. No other class can directly access this field.private void privateMethod() { // Method logic }
: This line declares a method namedprivateMethod
with theprivate
access modifier. This method can only be called from within thePrivateClass
class. No other class can invoke this method directly.
Example of Controlled Access
To provide controlled access to the private field privateField
, you can use public getter and setter methods:
public class PrivateClass {
private int privateField;
// Public getter method
public int getPrivateField() {
return privateField;
}
// Public setter method
public void setPrivateField(int privateField) {
this.privateField = privateField;
}
private void privateMethod() {
// Method logic
}
}
In this example, the getPrivateField
and setPrivateField
methods allow controlled access to the privateField
. You can add validation or other logic in these methods to ensure the integrity of the data.
Protected Access Modifier
The protected
access modifier in Java provides a level of access control that is more permissive than private
but more restrictive than public
. It allows access to the member by classes in the same package and by subclasses, even if they are in different packages. This is particularly useful for inheritance, as it allows subclasses to access and override the protected members of their superclass.
Any class within the same package as the class containing the protected member can access the protected member. This is similar to the default (package-private) access level.
Subclasses, regardless of whether they are in the same package or a different package, can access protected members of their superclass. This is a key feature for inheritance, as it allows subclasses to utilize and extend the functionality of their superclass.
public class ProtectedClass {
protected int protectedField;
protected void protectedMethod() {
// Method logic
}
}
Example
public class ProtectedClass {
protected int protectedField;
protected void protectedMethod() {
// Method logic
}
}
class SamePackageClass {
public void accessProtectedMember() {
ProtectedClass obj = new ProtectedClass();
obj.protectedField = 10; // Allowed because SamePackageClass is in the same package
obj.protectedMethod(); // Allowed because SamePackageClass is in the same package
}
}
package differentPackage;
import ProtectedClass;
public class SubClass extends ProtectedClass {
public void accessProtectedMember() {
this.protectedField = 20; // Allowed because SubClass is a subclass of ProtectedClass
this.protectedMethod(); // Allowed because SubClass is a subclass of ProtectedClass
}
}
Encapsulation: While
protected
allows more access thanprivate
, it still helps in encapsulating the internal state of a class to some extent by restricting access to subclasses and classes within the same package.Inheritance: The primary use of
protected
is to facilitate inheritance. Subclasses can access and override protected members, allowing for more flexible and reusable code.Package-Level Access: Classes within the same package can access protected members, which can be useful for organizing related classes that need to interact closely
Default Access Modifier
If no access modifier is specified, the member is given default access, also known as package-private access. This means the member is accessible only within its own package. It is the access level given to a class, method, or field when no explicit access modifier is specified. This means the member is accessible only within its own package. Members with default access are not accessible from classes in different packages, even if they are subclasses.
Members with default access are accessible only within the same package. This is useful for grouping related classes together and allowing them to interact closely while restricting access from unrelated classes in different packages.
nlike other access modifiers (
public
,protected
,private
), default access is specified by the absence of an access modifier keyword.
class DefaultClass {
int defaultField;
void defaultMethod() {
// Method logic
}
}
Class
DefaultClass
: The class itself has default access because no access modifier is specified. This means thatDefaultClass
can only be accessed by other classes within the same package.Field
defaultField
: This integer field has default access. It can be accessed and modified by any class within the same package asDefaultClass
.Method
defaultMethod
: This method also has default access. It can be called by any class within the same package asDefaultClass
.
Comparison
The table below summarizes the available access modifiers. We can see that a class, regardless of the access modifiers used, always has access to its members:
Modifier | Class | Package | Subclass | World |
public | Y | Y | Y | Y |
protected | Y | Y | Y | N |
default | Y | Y | N | N |
private | Y | N | N | N |
Canonical Order of Modifiers
In Java, the order in which modifiers are applied to a class, method, or field is important for readability and consistency. This order is known as the canonical order of modifiers.
Annotations
Access Modifiers
Other Modifiers
Type
Annotations
Annotations are a form of metadata that provide additional information about the code. They are placed before the access modifiers. Examples of annotations include @Override
, @Deprecated
, and @SuppressWarnings
.
@Override
public void someMethod() {
// Method logic
}
Access Modifiers
Access modifiers control the visibility of the class, method, or field. The main access modifiers in Java are public
, protected
, private
, and the default (no modifier). These modifiers should come immediately after any annotations.
public class MyClass {
// Class logic
}
Other Modifiers
Other modifiers include static
, final
, abstract
, synchronized
, native
, strictfp
, and transient
. These modifiers specify additional properties of the class, method, or field. They should be placed after the access modifiers.
public static final int MY_CONSTANT = 10;
Type
The type of the member (class, method, or field) comes last. This includes the return type for methods, the data type for fields, and the class type for class declarations.
public static final int myField;
public void myMethod() {
// Method logic
}
Examples
Class Declaration
@Deprecated
public abstract class MyClass {
// Class logic
}
Field Declaration
private static final int MY_CONSTANT = 10;
Method Declaration
@Override
public synchronized void myMethod() {
// Method logic
}
Conclusion
Access modifiers in Java control the visibility of classes, methods, and fields. The four main access modifiers are public
, private
, protected
, and default (no modifier). Public
allows access from any class, private
restricts access to within the same class, protected
permits access within the same package and subclasses, and default access is limited to the same package. These modifiers are essential for encapsulation and managing the scope of class members.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer