How understanding browser rendering engines can make you a better React developer (and why it matters!) 🚀
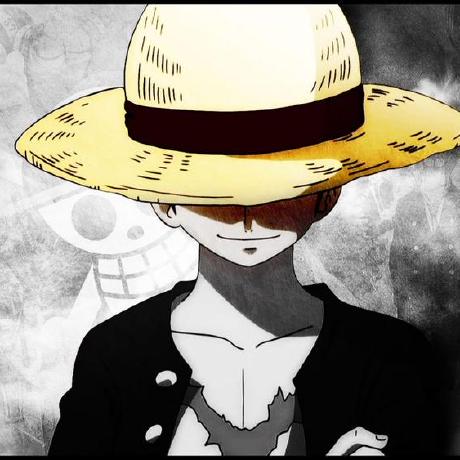
As React developers, we often focus on components, state management, and performance optimisations. But there’s one critical aspect of web development that tends to get overlooked: how browsers actually render the pages we create.
Understanding how browser rendering engines work is a game-changer. It helps you write better, more efficient code, optimize your applications, and deliver smoother user experiences. Let’s dive into why it’s worth paying attention and how it can improve your skills as a React developer.
What Happens When a Browser Renders a Page?
When a browser receives your HTML, CSS, and JavaScript, it follows a series of steps known as the critical rendering path. This process includes:
Parsing HTML to create the DOM (Document Object Model)
Parsing CSS to create the CSSOM (CSS Object Model)
Combining the DOM and CSSOM to create the Render Tree
Calculating the layout (also known as reflow)
Painting the pixels to the screen (also known as repaint)
Each of these steps is essential for displaying your web page. Understanding these stages allows you to identify potential bottlenecks and avoid actions that slow down the rendering process.
Let’s take a detailed look at some common issues that can arise if you don’t take browser rendering into account.
Reflows and Repaints:
Reflows and repaints are crucial aspects of the browser rendering process that can significantly impact the performance of your web application. As a React developer, understanding these concepts can help you avoid common performance pitfalls.
A reflow occurs whenever the layout of the page changes. This can be triggered by altering the size, position, or visibility of an element. For instance, adjusting the width, height, or margin properties of a component can cause the browser to recalculate the layout, affecting the entire page or part of it. Reflows are expensive in terms of performance because they require the browser to reprocess and rebuild the layout for the affected parts of the page, which can slow down the user interface if done frequently.
A repaint happens when changes to an element’s appearance don’t affect the layout, such as changing the background-color or visibility of an element. While repaints are less costly than reflows, they still impact performance because the browser has to redraw parts of the screen.
In React, reflows and repaints can be triggered if you’re not careful with how often your components update or how deeply they manipulate the DOM. For example, triggering multiple reflows in quick succession can cause what’s known as layout thrashing, where the browser is forced to recalculate the layout repeatedly. This can make your application feel sluggish, particularly on mobile devices or slower browsers.
The key to avoiding excessive reflows and repaints is to minimize unnecessary DOM manipulations. You can use techniques like batching updates in React or using CSS transforms instead of positioning properties, which bypass reflows altogether.
JavaScript Execution:
JavaScript is an essential part of any modern React application, but it can also be a source of significant performance bottlenecks if not optimized properly. Understanding how the browser executes JavaScript is critical for writing efficient code and ensuring that your app runs smoothly.
When a browser encounters JavaScript, it must stop rendering and processing the page until the JavaScript is executed. This is known as render-blocking behavior. If your JavaScript files are large or poorly optimized, they can significantly delay the rendering of your page, causing slow load times and a poor user experience.
React apps are typically composed of numerous components, each potentially executing JavaScript that modifies the DOM or updates state. While React’s Virtual DOM helps optimize some of this work by batching updates, heavy or inefficient JavaScript logic can still lead to performance issues. For example, unoptimized loops, event listeners, or recursive functions can cause the browser to become unresponsive, especially on older devices.
One solution is to use lazy loading for components that are not needed immediately. This allows you to split your JavaScript into smaller chunks and only load what’s necessary when the user interacts with certain parts of the app. Memoization is another helpful technique, where you cache the results of expensive function calls to avoid recalculating them unnecessarily.
By optimising how and when your JavaScript code runs, you can ensure that the browser spends less time executing scripts and more time rendering your app. This will lead to faster, more responsive interfaces, which is something both users and recruiters will appreciate.
CSS Optimisations:
CSS plays a critical role in determining how fast a web page renders, and it’s something that every React developer should pay attention to. While CSS seems straightforward, it can significantly impact performance if not optimized properly, particularly because it is render-blocking by default.
When the browser encounters a CSS file, it pauses rendering until all the CSS has been downloaded, parsed, and applied. This can delay the display of your page, leading to what’s known as the “flash of unstyled content” or slow initial renders. For large-scale React applications, poorly managed CSS can compound this issue.
One way to optimise CSS is by prioritising the critical CSS—the styles that are necessary for the initial render. You can defer non-essential CSS to load after the page has rendered, improving load times. Another technique is minifying CSS files to reduce their size, which speeds up downloading and parsing. Additionally, CSS-in-JS libraries like styled-components allow you to scope your styles locally to components, reducing the amount of CSS the browser needs to process at any given time.
React developers should also be mindful of unused CSS. Over time, stylesheets can become cluttered with styles that are no longer in use. Tools like PurgeCSS can help clean up unused styles, resulting in leaner, faster-loading stylesheets.
Lastly, media queries and responsive design should be optimized for performance. Rather than loading a universal set of CSS rules, serve optimized CSS based on the user’s device to ensure quicker load times on mobile and slower connections.
By fine-tuning your CSS, you can reduce the render-blocking behavior and improve the overall performance of your React applications, leading to faster, smoother user experiences.
Critical Rendering Path:
The critical rendering path refers to the sequence of steps the browser takes to convert HTML, CSS, and JavaScript into a fully rendered page. As a React developer, understanding this process is key to optimizing your application’s load times and ensuring a smooth user experience.
The critical rendering path involves several stages: parsing the HTML to build the DOM (Document Object Model), parsing the CSS to create the CSSOM (CSS Object Model), and then combining the two to form the Render Tree. This tree is used to calculate the layout (also known as reflow), which is finally painted on the screen.
React applications often consist of large JavaScript bundles that can block the critical rendering path, slowing down the time it takes for the browser to render the page. Techniques like code splitting and lazy loading can help optimize this. By breaking up your JavaScript into smaller, more manageable chunks, you reduce the amount of blocking resources that the browser needs to handle before it can render content.
Another way to optimize the critical rendering path is by prioritizing above-the-fold content. This means ensuring that the content visible to the user upon page load is rendered first, while other non-essential content can load asynchronously in the background.
React developers should also focus on minimizing render-blocking resources like CSS and JavaScript. By deferring non-essential scripts and styles or using asynchronous loading methods, you can shorten the critical rendering path and speed up your app’s initial render.
Understanding and optimizing the critical rendering path will not only improve the performance of your React app but also reduce load times, leading to better user engagement and higher search engine rankings.
Final Thoughts
Understanding browser rendering engines isn’t just a bonus skill—it’s crucial for building high-performance React apps. By diving into how browsers process HTML, CSS, and JavaScript, you can make smarter decisions when writing code, avoid common pitfalls, and create web experiences that are faster and more efficient. As React developers, it’s time to go beyond components and start thinking about how our code interacts with the browser.
Subscribe to my newsletter
Read articles from Sourabh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
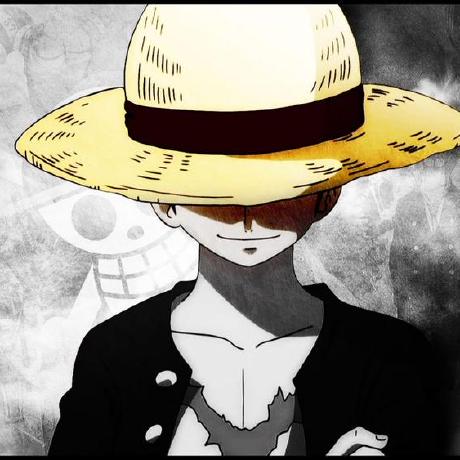