Understanding Thread Safety and Locks in C# with a Practical Example

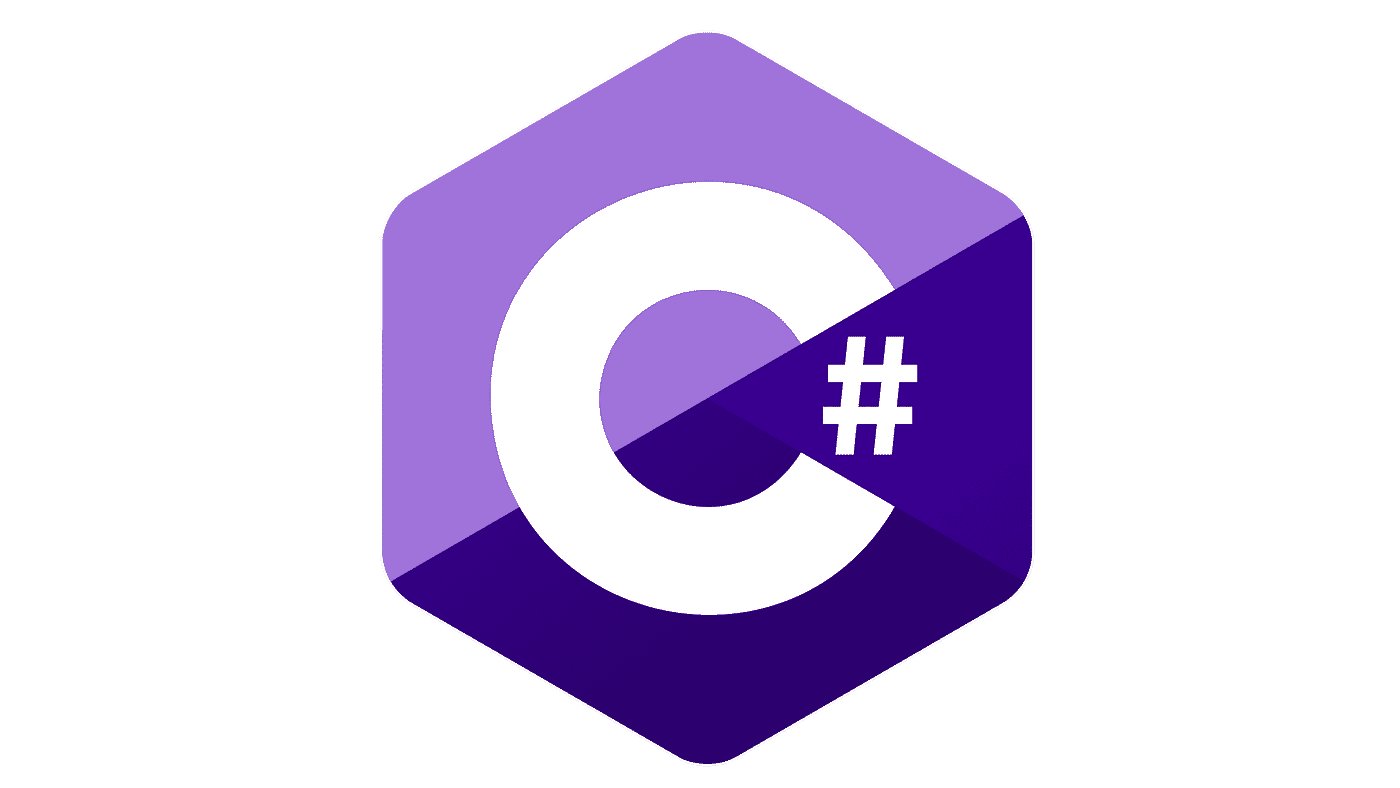
In the world of multi-threaded applications, ensuring that your data stays consistent across multiple threads can be a challenging task. If not handled correctly, multiple threads trying to access and modify the same piece of data can lead to unexpected behavior, race conditions, or data corruption. This is where the concepts of thread safety and locks come into play.
In this post, we'll walk through what thread safety is, why locks are essential in multi-threaded applications, and how to implement them correctly. We'll use a practical example: generating unique IDs in a C# application, where multiple threads are involved.
What is Thread Safety?
Thread safety is a key concept in concurrent programming that ensures multiple threads can safely interact with shared data or resources without causing errors or inconsistencies. If a piece of code is "thread-safe," it can be executed by multiple threads simultaneously without leading to unexpected behavior or corrupting shared resources.
In C#, when two or more threads attempt to read or modify a shared variable at the same time, it can lead to issues. For example, if two threads are incrementing the same variable, one might overwrite the other's work, leading to incorrect results. This is known as a race condition.
Thread Safety Issue: A Practical Example
Let’s consider a scenario where we're generating unique IDs in a format like:
APPS24/09/27/01
The unique ID includes the current date (24/09/27
), followed by a two-digit number that ranges from 00
to 99
. To ensure that the IDs remain unique across multiple threads, we need to increment a number and wrap around after 99
.
Without proper synchronization, race conditions could occur, leading to duplicate IDs. Here's an example of how race conditions can occur if multiple threads try to generate IDs at the same time.
private static int _lastUniqueNumber = 0;
public string GenerateUniqueId()
{
var now = DateTime.Now;
var day = now.ToString("dd");
var month = now.ToString("MM");
var year = now.ToString("yy");
// Incrementing the number
_lastUniqueNumber = (_lastUniqueNumber + 1) % 100;
return $"APPS{year}/{month}/{day}/{_lastUniqueNumber:D2}";
}
In this code, multiple threads can access _lastUniqueNumber
at the same time. Without protection, both threads might read the same value, increment it, and assign the same new value. This results in duplicate IDs, which is a critical issue in systems where uniqueness is mandatory.
Introducing Locks for Thread Safety
To avoid such issues, we can use a lock in C#. A lock allows us to restrict access to a specific section of code, ensuring that only one thread can execute it at a time. This section of code is known as a critical section. While one thread is inside this critical section, other threads must wait until the lock is released.
Here’s the solution to our problem using a lock to ensure that the unique number is safely incremented, even in multi-threaded environments.
Using Locks in C#: A Safe Approach
Let’s implement thread safety using a lock:
private static int _lastUniqueNumber = 0; // Shared resource
private static readonly object _lock = new object(); // Lock object
public string GenerateUniqueId()
{
var now = DateTime.Now;
var day = now.ToString("dd");
var month = now.ToString("MM");
var year = now.ToString("yy");
int uniqueNumber;
// Lock to ensure only one thread can modify the unique number at a time
lock (_lock)
{
// Increment and wrap around after 99
_lastUniqueNumber = (_lastUniqueNumber + 1) % 100;
uniqueNumber = _lastUniqueNumber;
// Simulate saving to a database
SaveLastUniqueNumberToDb(uniqueNumber);
}
return $"APPS{year}/{month}/{day}/{uniqueNumber:D2}";
}
How This Works:
Lock Object: The
lock
statement in C# requires an object (_lock
in this case) to manage the locking mechanism. This object is used to ensure that only one thread can hold the lock at a time. When one thread enters the critical section, others must wait until it exits and releases the lock.Thread Synchronization: The block of code inside the
lock (_lock)
ensures that only one thread at a time can increment_lastUniqueNumber
. This prevents race conditions where two threads might try to increment the same number simultaneously.Wrap-Around Logic: After incrementing, we use modulo 100 (
% 100
) to ensure the unique number wraps around after reaching99
, giving us numbers from00
to99
.Save to Database: In real applications, saving the current unique number to a database ensures the number is persisted, even if the application restarts. This prevents gaps or duplicate IDs from being generated.
Why Locks Are Important
Without a lock, if two threads run GenerateUniqueId()
at the same time, both may read the same value of _lastUniqueNumber
before either increments it. As a result, both threads might generate the same ID, which breaks the uniqueness requirement.
By using a lock:
Only one thread can modify
_lastUniqueNumber
at a time.Other threads that attempt to access the critical section must wait until the lock is released, ensuring that the ID generation logic remains consistent.
Things to Keep in Mind About Locks
While locks provide a straightforward way to ensure thread safety, they must be used carefully to avoid performance issues or deadlocks.
Performance: Locking introduces a small performance cost since threads need to wait their turn. In high-traffic scenarios, this could potentially slow down your application if used excessively or in large critical sections.
Deadlocks: If you have multiple locks and threads, deadlocks can occur. A deadlock happens when two threads are each waiting for the other to release a lock, causing them to get stuck indefinitely. Always ensure that locks are well-ordered and released promptly to avoid this.
Conclusion
In this post, we explored the concepts of thread safety and locks in C#. We saw how race conditions can occur when multiple threads try to modify shared data simultaneously and how using a lock can prevent these issues.
When working with shared resources, it’s crucial to implement proper thread synchronization to maintain data consistency. By locking critical sections of code, we can ensure that only one thread accesses or modifies shared data at a time.
This is especially important in scenarios like unique ID generation, where data integrity is paramount. With locks, we can safely handle multi-threaded environments and ensure that each generated ID remains unique.
What are your thoughts?
If you've faced similar challenges in your applications, feel free to share how you solved them! Let’s discuss in the comments.
#CSharp #Concurrency #ThreadSafety #Locks #DotNet #Programming #Hashnode
Subscribe to my newsletter
Read articles from Ujjwal Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ujjwal Singh
Ujjwal Singh
👋 Hi, I'm Ujjwal Singh! I'm a software engineer and team lead with 10 years of expertise in .NET technologies. Over the years, I've built a solid foundation in crafting robust solutions and leading teams. While my core strength lies in .NET, I'm also deeply interested in DevOps and eager to explore how it can enhance software delivery. I’m passionate about continuous learning, sharing knowledge, and connecting with others who love technology. Let’s build and innovate together!