Best Practices for Automating Security Testing in DevSecOps (2024)
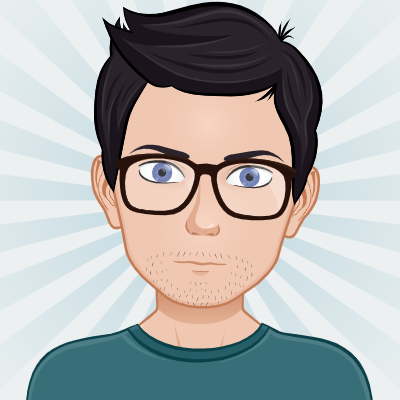
A Comprehensive Guide
In today's fast-paced software development landscape, integrating security into the DevOps pipeline—commonly referred to as DevSecOps—is crucial. As organizations strive for rapid delivery while maintaining robust security, automating security testing becomes a key practice. This guide will explore best practices for automating security testing within a DevSecOps framework, providing actionable insights, code snippets, and comparisons of various tools to help you implement effective security measures.
Understanding the Importance of Automated Security Testing
Automated security testing helps identify vulnerabilities early in the development lifecycle, reducing the risk of security breaches and ensuring compliance with industry standards. By integrating security testing into CI/CD pipelines, organizations can achieve:
Faster Feedback Loops: Immediate identification of vulnerabilities allows for quicker remediation.
Reduced Costs: Early detection minimizes the cost associated with fixing security issues later in the development process.
Enhanced Security Posture: Continuous monitoring and testing ensure that applications remain secure throughout their lifecycle.
Key Components of Automated Security Testing
Before diving into best practices, it's essential to understand the key components involved in automated security testing:
Static Application Security Testing (SAST): Analyzes source code for vulnerabilities without executing it.
Dynamic Application Security Testing (DAST): Tests running applications to identify vulnerabilities that may not be visible in source code.
Interactive Application Security Testing (IAST): Combines SAST and DAST techniques to provide real-time insights during application execution.
Software Composition Analysis (SCA): Identifies vulnerabilities in third-party libraries and dependencies.
Comparison of Testing Techniques
Technique | Description | Pros | Cons |
SAST | Analyzes source code without execution | Early detection of vulnerabilities | May produce false positives |
DAST | Tests running applications | Identifies runtime vulnerabilities | Requires a deployed environment |
IAST | Monitors applications during execution | Comprehensive insights with fewer false positives | More complex setup |
SCA | Scans dependencies for known vulnerabilities | Identifies risks from third-party libraries | Limited to known vulnerabilities |
Best Practices for Automating Security Testing
1. Integrate Security Testing Early in the CI/CD Pipeline
How to Implement: Incorporate SAST tools into your version control system (e.g., Git) to analyze code on every commit. This ensures that vulnerabilities are identified before they reach production.
Example Code Snippet:
# Example GitHub Actions workflow for SAST using SonarQube
name: CI
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Run SonarQube Scanner
run: |
sonar-scanner \
-Dsonar.projectKey=my_project \
-Dsonar.sources=. \
-Dsonar.host.url=http://your-sonarqube-server \
-Dsonar.login=$SONAR_TOKEN
2. Utilize Multiple Testing Techniques
Employing a combination of SAST, DAST, and IAST provides a more comprehensive security assessment. Each technique covers different aspects of application security.
Implementation Strategy:
Use SAST during the coding phase.
Implement DAST during staging or pre-production.
Leverage IAST for real-time monitoring in production environments.
3. Automate Dependency Scanning with SCA Tools
Integrate SCA tools into your CI/CD pipeline to automatically scan for vulnerabilities in third-party libraries.
Example Tool Integration: Using Snyk, you can automate dependency checks as follows:
# Install Snyk CLI
npm install -g snyk
# Test your project for vulnerabilities
snyk test
4. Implement Continuous Monitoring and Feedback Loops
Set up continuous monitoring to automatically run security tests at defined intervals or upon specific triggers (e.g., pull requests).
Example Configuration:
# Example Jenkins pipeline configuration for continuous monitoring
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('SCA') {
steps {
sh 'snyk test'
}
}
stage('DAST') {
steps {
sh 'zap.sh -quickurl http://your-app-url'
}
}
}
}
5. Prioritize Vulnerabilities Based on Risk Assessment
Not all vulnerabilities are created equal. Implement a risk-based approach to prioritize remediation efforts based on factors such as exploitability and impact.
Risk Assessment Frameworks:
CVSS (Common Vulnerability Scoring System): Assigns scores based on severity.
OWASP Top Ten: Focus on the most critical web application security risks.
6. Foster Collaboration Between Development and Security Teams
Encourage communication between developers and security teams to create a shared responsibility model for security.
Collaboration Tools:
Use platforms like Slack or Microsoft Teams for real-time communication.
Implement ticketing systems (e.g., Jira) to track vulnerability remediation efforts.
7. Leverage Automation Tools for Reporting and Compliance
Automate reporting processes to ensure compliance with industry regulations (e.g., GDPR, PCI-DSS). Use tools that generate detailed reports on vulnerabilities and remediation efforts.
Example Reporting Tool Integration: Using Burp Suite, you can automate reporting as follows:
# Generate a report after running scans
burpsuite --project-file=my_project.burp --report-file=my_report.html --report-type=html
8. Regularly Update and Maintain Security Tools
Ensure that all automated security tools are regularly updated to leverage new features and vulnerability databases.
Update Strategy:
Schedule regular maintenance windows for tool updates.
Monitor vendor announcements for critical updates.
9. Conduct Regular Training and Awareness Programs
Provide ongoing training for development teams on secure coding practices and the importance of automated security testing.
Training Resources:
OWASP training materials.
Online courses on platforms like Coursera or Udemy focused on secure coding and DevSecOps practices.
10. Evaluate Tool Effectiveness Regularly
Periodically assess the effectiveness of your automated security testing tools by measuring metrics such as false positive rates, time taken to remediate vulnerabilities, and overall security posture improvement.
Common Challenges when automating Security Testing
1. Tool Sprawl and Integration Issues
One of the primary challenges in automating security testing is the proliferation of tools within the DevOps ecosystem. Organizations often adopt multiple security tools to address different aspects of security, leading to tool sprawl. This can result in integration issues where tools do not communicate effectively with each other or with existing CI/CD pipelines.
Key Points
Diverse Toolsets: The use of various tools (SAST, DAST, IAST, SCA) can create complexities in managing and integrating them.
Compatibility Challenges: Tools may not seamlessly integrate with existing development workflows, leading to inefficiencies.
Solution: To mitigate this issue, organizations should select tools that offer robust integration capabilities and prioritize those that are designed to work within their existing CI/CD frameworks. For example, using tools like SonarQube for SAST and OWASP ZAP for DAST can streamline the process if they are configured to work together.
2. Alert Fatigue
Automated security tools can generate a high volume of alerts, many of which may be false positives. This can lead to alert fatigue among development and security teams, causing them to overlook critical vulnerabilities due to the overwhelming number of notifications.
Key Points:
False Positives: Many automated tools report issues that are not actual vulnerabilities, leading to wasted time and resources.
Overwhelmed Teams: The sheer volume of alerts can desensitize teams to warnings, increasing the risk of overlooking genuine threats.
Solution: Implementing a risk-based approach to prioritize alerts based on severity can help teams focus on the most critical vulnerabilities first. Additionally, leveraging machine learning algorithms that learn from past incidents can help reduce false positives over time.
3. Lack of Security Skills
Many development teams may lack the necessary security expertise required to effectively implement and manage automated security testing tools. This skills gap can hinder the successful adoption of DevSecOps practices.
Key Points:
Training Needs: Developers may need training on secure coding practices and how to use security tools effectively.
Cultural Shift: A shift in mindset is required for developers to take ownership of security as part of their responsibilities.
Solution: Organizations should invest in training programs that educate developers about security best practices and how to utilize automated tools effectively. Pairing developers with security experts during initial implementations can also facilitate knowledge transfer.
4. Resistance to Change
Integrating automated security testing into existing workflows often meets resistance from development teams who may perceive it as an impediment to their speed and efficiency. This resistance can stem from a lack of understanding of the benefits or fear of additional workload.
Key Points:
Cultural Barriers: Development teams may view security as an obstacle rather than an integral part of the development process.
Fear of Slowing Down Delivery: Concerns about added time for testing can lead to pushback against automation initiatives.
Solution: To overcome resistance, organizations should communicate the benefits of automated security testing clearly—emphasizing how it enhances overall software quality and reduces long-term costs associated with breaches. Demonstrating quick wins through successful implementations can also help gain buy-in from skeptical team members.
5. Insufficient Automation Coverage
Many organizations struggle with achieving comprehensive automation coverage across all stages of the software development lifecycle (SDLC). Security testing may be limited to specific phases or types of testing, leaving gaps that could be exploited by attackers.
Key Points:
Limited Testing Phases: Security tests might only be conducted at certain points in the SDLC rather than continuously.
Incomplete Coverage: Critical areas such as third-party dependencies or infrastructure as code (IaC) may be overlooked.
Solution: Organizations should aim for a holistic approach by integrating automated security testing throughout the entire SDLC—from code commit through deployment and into production monitoring. Utilizing tools like Snyk for SCA ensures that third-party libraries are continuously monitored for vulnerabilities.
Example Integration
Here’s how you might set up a GitHub Actions workflow that includes both SAST and SCA:
name: CI Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Run SAST with SonarQube
run: |
sonar-scanner \
-Dsonar.projectKey=my_project \
-Dsonar.sources=. \
-Dsonar.host.url=http://your-sonarqube-server \
-Dsonar.login=$SONAR_TOKEN
- name: Run SCA with Snyk
run: snyk test
6. Complexity in Managing Security Policies
As organizations scale their DevSecOps efforts, managing security policies across diverse teams and projects becomes increasingly complex. Inconsistent application of policies can lead to vulnerabilities being introduced into production environments.
Key Points:
Policy Management Challenges: Ensuring all teams adhere to established security policies can be difficult.
Lack of Standardization: Variability in how policies are implemented across teams increases risk.
Solution: Establish clear, standardized policies for security practices that all teams must follow. Regular audits and reviews can help ensure compliance and identify areas for improvement.
7. Continuous Monitoring Difficulties
Automated security testing is not a one-time effort; it requires continuous monitoring to adapt to new threats and vulnerabilities that emerge over time. However, maintaining this level of vigilance can be challenging without proper tools and processes in place.
Key Points
Dynamic Threat Landscape: New vulnerabilities are constantly being discovered.
Resource Constraints: Continuous monitoring requires dedicated resources which may not always be available.
Solution: Implementing solutions like Security Information and Event Management (SIEM) systems can help automate monitoring efforts and provide real-time alerts on potential threats. Tools such as Splunk or Elastic Stack (ELK) are popular choices for aggregating logs and monitoring application behavior continuously.
Conclusion
Automating security testing within a DevSecOps framework is essential for maintaining robust application security while enabling rapid delivery cycles. By following these best practices—integrating multiple testing techniques early in the CI/CD pipeline, leveraging automation tools, fostering collaboration, and continuously evaluating your processes—you can significantly enhance your organization's security posture.
By implementing these best practices for automating security testing in DevSecOps, organizations can not only improve their overall security posture but also foster a culture of collaboration between development and security teams. This proactive approach will ultimately lead to more secure applications and reduced risk of breaches in an increasingly complex digital landscape.
Automating security testing in a DevSecOps environment is essential for maintaining robust security while enabling rapid software delivery. However, organizations face several challenges when implementing automated security testing. This article explores the most common challenges associated with automating security testing in DevSecOps, providing insights and strategies to overcome them.
Citations
[1] https://www.opsera.io/blog/devsecops-automation [2] https://www.hackerone.com/knowledge-center/devops-security-challenges-and-6-critical-best-practices [3] https://www.dynatrace.com/news/blog/what-is-devsecops/ [4] https://www.techtarget.com/searchsoftwarequality/tip/SAST-vs-DAST-vs-IAST-Security-testing-tool-comparison [5] https://insights.sei.cmu.edu/blog/5-challenges-to-implementing-devsecops-and-how-to-overcome-them/ [6] https://www.armorcode.com/learning-center/what-is-devsecops [7] https://www.microtica.com/blog/common-devsecops-challenges-and-how-to-overcome-them [8] https://www.lambdatest.com/blog/cicd-pipeline-challenges/
[1] https://qawerk.com/blog/top-10-open-source-software-security-testing-tools/ [2] https://www.geeksforgeeks.org/software-testing-security-testing-tools/ [3] https://www.getastra.com/blog/security-audit/best-penetration-testing-tools/ [4] https://thectoclub.com/tools/best-security-testing-tools/ [5] https://www.contrastsecurity.com/glossary/iast-vs-sast [6] https://www.softwaresecured.com/post/top-testing-options-comparison [7] https://www.techtarget.com/searchsoftwarequality/tip/SAST-vs-DAST-vs-IAST-Security-testing-tool-comparison [8] https://www.lambdatest.com/blog/cicd-pipeline-challenges/
Subscribe to my newsletter
Read articles from Piyush T Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
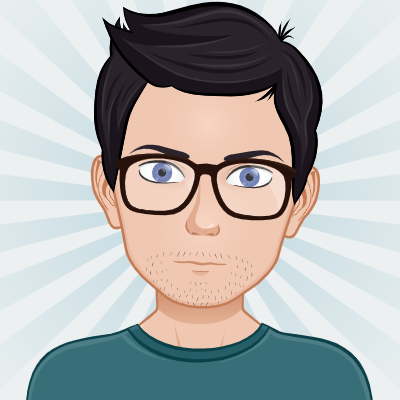