Understanding Python Literals, Operators, and a Simple Program

Table of contents
- What Are Python Literals?
- Numeric and Non-Numeric Literals
- String Literals in Python
- Python Assignment Operators
- Python Comparison Operators
- Python Logical Operators
- Identity Operator: is
- Python Arithmetic Operators
- Logical Comparisons in Python
- Python Ternary Operator
- How to Calculate the Area of a Circle in Python
- Conclusion
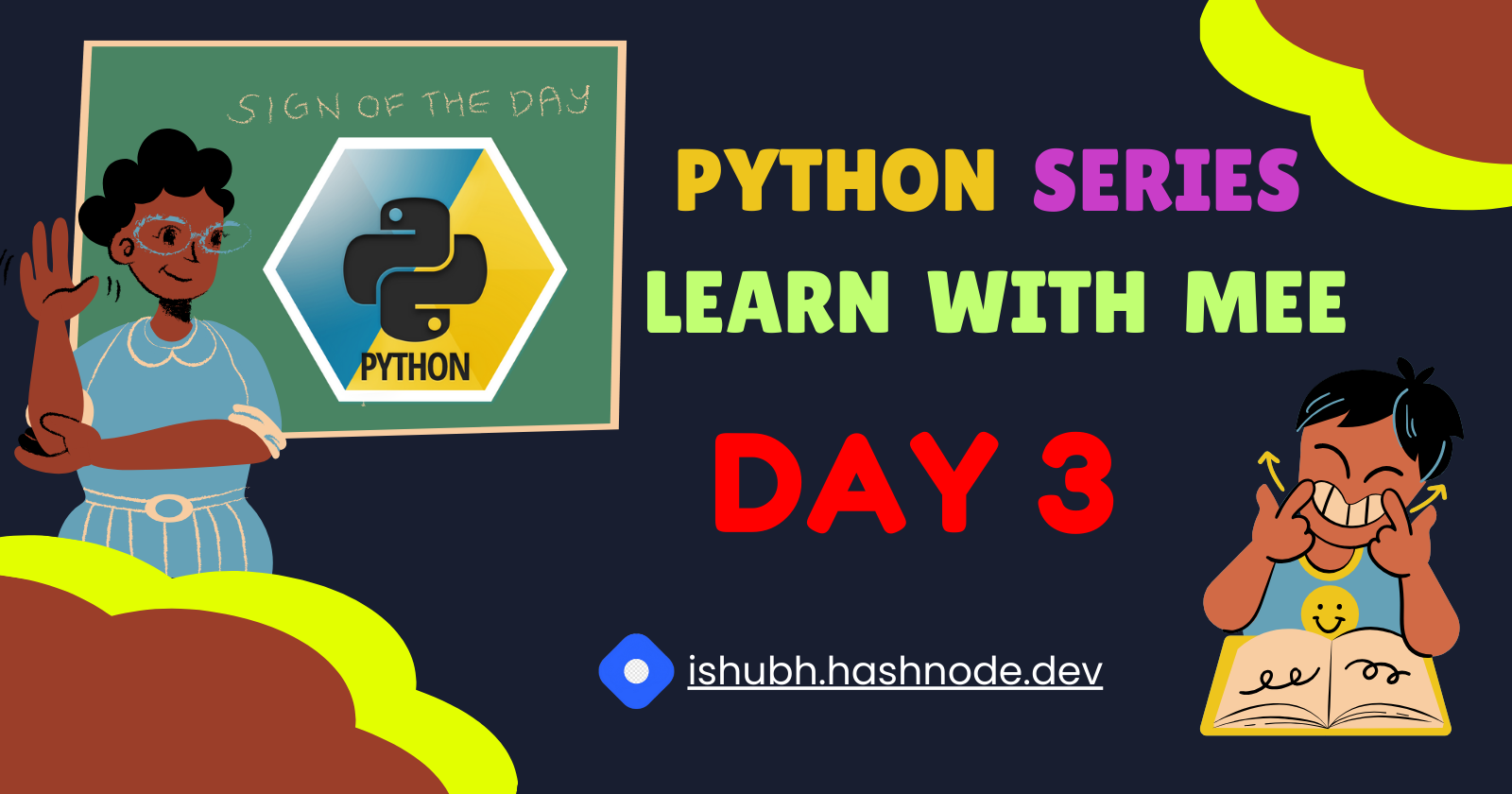
If you are new to Python programming and want to understand key concepts like literals, operators, and how to write basic Python programs, you’ve come to the right place! This guide will walk you through the fundamentals of Python literals, arithmetic and logical operators, and how to calculate the area of a circle using Python.
What Are Python Literals?
In Python, literals refer to the raw values assigned to variables. These literals can be of various types, including numeric, string, and Boolean values. #2Articles1Week, #Hashnode.
Here’s an example of different types of Python literals:
name = "Ganesha" # A string literal
age = 12 # An integer literal
pi = 3.14 # A floating-point literal
String Literals: Represent sequences of characters.
Numeric Literals: Include integers and floating-point numbers.
Boolean Literals: Represent
True
orFalse
values.
Numeric and Non-Numeric Literals
Literals are divided into numeric and non-numeric categories. Numeric literals include integers like 12
and floating-point numbers like 3.14
. Non-numeric literals can be strings or Boolean values like True
and False
.
Here’s a simple example:
is_married = True # Boolean Literal
name = "Ganesha" # String Literal
age = 12 # Integer Literal
String Literals in Python
Python allows the use of both single and double quotes for string literals. You can also use triple quotes ('''
or """
) for multi-line strings.
Example of string literals:
name1 = 'John'
name2 = "Ariana"
# Multi-line string literal
description = """
This is a multi-line
string in Python.
"""
Python Assignment Operators
Assignment operators in Python are used to assign values to variables. The simplest form is the =
operator, which assigns the value on the right-hand side to the variable on the left-hand side.
For example:
Number = 45
Employee_name = "Kriti"
Python Comparison Operators
Comparison operators compare two values and return a Boolean value (True
or False
). Common comparison operators in Python include:
==
(equal to)!=
(not equal to)>
(greater than)<
(less than)
Example:
cond_1 = (1 == True) # True, because 1 is considered True in Python
cond_2 = (0 == False) # True, because 0 is considered False in Python
To display the results:
print(cond_1) # Output: True
print(cond_2) # Output: True
Python Logical Operators
Logical operators such as and
, or
, and not
are used to combine conditional statements. These are often employed in decision-making processes within the program.
Here’s how the not
operator works:
is_rain = True
print(is_rain) # Output: True
print(not is_rain) # Output: False
Identity Operator: is
The is
operator checks whether two variables refer to the same object in memory. This is especially useful for comparing object identities rather than just values.
Example:
num1 = 1
num2 = 2
print(num1 is num2) # Output: False
Python Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations like addition, subtraction, multiplication, division, and modulus.
Here’s a breakdown:
num1 = 12
num2 = 13
print(f"Addition: {num1 + num2}")
print(f"Subtraction: {num1 - num2}")
print(f"Multiplication: {num1 * num2}")
print(f"Division: {num1 / num2}")
print(f"Modulus: {num1 % num2}") # Remainder
Python also supports power calculations using **
or the pow()
function:
print(3 ** 2) # Output: 9
print(pow(3, 2)) # Output: 9
Logical Comparisons in Python
Python supports logical comparisons using the operators >
, <
, >=
, <=
, ==
, and !=
. These are frequently used in decision-making within programs.
Example:
num1 = 20
num2 = 5
print(f"Is num1 greater than num2? {num1 > num2}") # Output: True
print(f"Is num1 less than num2? {num1 < num2}") # Output: False
print(f"Is num1 equal to num2? {num1 == num2}") # Output: False
Python Ternary Operator
Python supports the ternary operator, which is used to write conditional statements in a single line. This is a concise way of assigning values based on conditions.
Example:
num1 = 10
num2 = 20
print("num1 is big" if num2 < num1 else "num2 is big") # Output: num2 is big
How to Calculate the Area of a Circle in Python
Now that we’ve covered the basics, let’s create a simple Python program to calculate the area of a circle using the formula π * r²
.
Here’s the program:
radius = float(input("Enter the radius: "))
pi = 3.14
area_of_circle = pi * (radius ** 2)
print(f"Area of the circle: {area_of_circle}")
This program takes the radius of the circle as input from the user and calculates the area using the formula π * r²
. The result is then displayed to the user.
Conclusion
This guide has covered essential concepts like Python literals, assignment operators, comparison operators, and a real-world example of calculating the area of a circle. Mastering these fundamentals is crucial for developing efficient and error-free Python programs. Whether you are a beginner or an experienced coder, understanding how to work with Python literals and operators will help you write cleaner, more concise code.
By following this guide, you now have a better understanding of how to use Python operators and work with literals effectively. Ready to take your Python programming skills to the next level? Start practicing with these basic concepts and apply them in real-world scenarios.
Subscribe to my newsletter
Read articles from Shubham Sutar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Sutar
Shubham Sutar
"Tech enthusiast and blogger exploring the latest in gadgets, software, and innovation. Passionate about simplifying tech for everyday users and sharing insights on trends that shape the future."