Flexbox Fundamentals: Creating Flexible Layouts
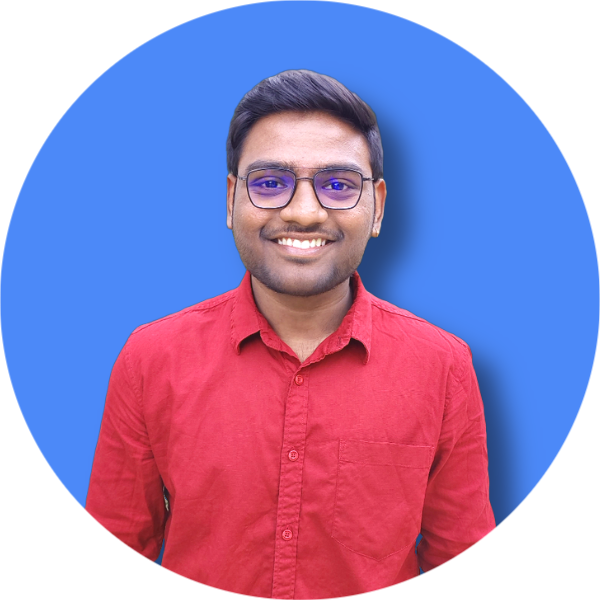
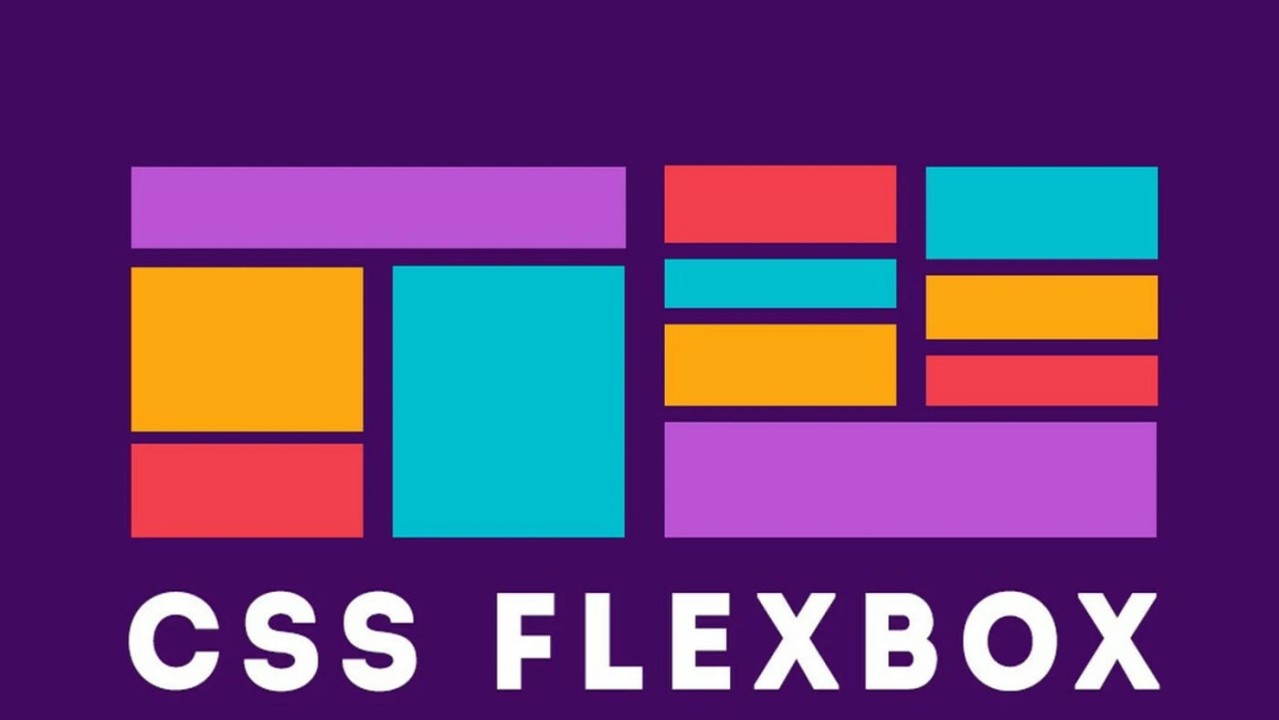
Flexbox, short for Flexible Box Layout, is a powerful CSS layout module that allows you to create complex, responsive layouts with ease. Unlike traditional layouts (like floats or grid systems), Flexbox is designed to distribute space dynamically and align content, making it especially useful for modern web design.
Introduction to Flexbox and Its Advantages
The Flexbox model provides an efficient way to align, distribute, and resize items within a container, even when their size is unknown or dynamic. It’s a one-dimensional layout system, meaning it works along a single axis: either horizontal (row) or vertical (column).
Key Advantages:
Flexible and Responsive: Flexbox automatically adjusts item sizes based on the available space.
Align and Center Easily: You can vertically and horizontally center items with minimal code.
Control Over Spacing: Flexbox allows for dynamic spacing, whether it’s between or around items.
Reorder Items: Easily reorder items in the layout without changing the HTML structure.
Flexbox Containers and Items
Flexbox consists of two main components:
Flex Container: The parent element that holds flex items.
Flex Items: The child elements inside the flex container that are laid out using Flexbox rules.
Setting Up a Flex Container
To make an element a Flexbox container, you need to apply the display: flex;
property to the parent element.
Example:
.container {
display: flex;
}
This turns .container
into a flex container, and all its child elements become flex items.
Aligning and Distributing Content
Flexbox provides powerful tools for aligning and distributing content within the container. Here are some important properties:
1. Flex Direction:
The flex-direction
property defines the direction in which the flex items are placed inside the container.
Row: Default direction, places items in a horizontal row.
Column: Places items in a vertical column.
Example:
.container {
display: flex;
flex-direction: column;
}
2. Justify Content:
The justify-content
property aligns items along the main axis (horizontal for row
, vertical for column
).
Values:
flex-start
: Align items to the start.flex-end
: Align items to the end.center
: Center items.space-between
: Distribute items evenly with space between them.space-around
: Distribute items with space around them.
Example:
.container {
display: flex;
justify-content: space-between;
}
3. Align Items:
The align-items
property aligns items along the cross axis (vertical for row
, horizontal for column
).
Values:
stretch
: Default, items stretch to fill the container.flex-start
: Align items to the start of the cross axis.flex-end
: Align items to the end.center
: Center items along the cross axis.baseline
: Align items based on the baseline of their text.
Example:
.container {
display: flex;
align-items: center;
}
4. Align Self:
You can override the alignment of a specific flex item using align-self
.
Example:
.item {
align-self: flex-end;
}
Building Responsive Layouts with Flexbox
One of the best features of Flexbox is its ability to create responsive layouts with minimal effort. Flex items automatically adjust based on available space, making it ideal for building fluid and adaptable designs.
1. Flex Grow, Shrink, and Basis:
flex-grow
: Defines how much a flex item should grow relative to others.flex-shrink
: Defines how much a flex item should shrink relative to others.flex-basis
: Defines the initial size of a flex item before growing or shrinking.
Example:
.item {
flex-grow: 1; /* All items will take up equal space */
}
2. Creating a Responsive Navigation Bar:
Here’s an example of how Flexbox can be used to create a responsive navigation bar:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexbox Navigation Bar</title>
<style>
.navbar {
display: flex;
justify-content: space-between;
background-color: #333;
padding: 10px;
}
.navbar a {
color: white;
padding: 14px 20px;
text-decoration: none;
text-align: center;
}
</style>
</head>
<body>
<div class="navbar">
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Services</a>
<a href="#">Contact</a>
</div>
</body>
</html>
In this example:
The navigation bar uses
display: flex
andjustify-content: space-between
to space the links evenly across the container.The links remain centered and responsive as the screen size changes.
Common Flexbox Issues and Solutions
While Flexbox simplifies layout, you may encounter some common issues. Here’s how to troubleshoot them:
1. Items Not Centering:
If items aren’t centering, ensure the following:
Use
justify-content: center;
for horizontal centering.Use
align-items: center;
for vertical centering.
Example:
.container {
display: flex;
justify-content: center;
align-items: center;
}
2. Flex Items Overflowing:
If flex items overflow their container, check:
The
flex-shrink
property. By default, items can shrink, but you may need to adjust this.Ensure
box-sizing: border-box;
is applied to include padding and borders in the element’s total size.
3. Uneven Spacing Between Items:
If items have uneven spacing, use justify-content: space-between;
or space-around;
to distribute them evenly.
Example:
.container {
display: flex;
justify-content: space-around;
}
Practical Examples and Exercises
Example 1: Simple Flexbox Layout
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexbox Layout</title>
<style>
.container {
display: flex;
justify-content: space-around;
align-items: center;
height: 100vh;
}
.box {
width: 100px;
height: 100px;
background-color: lightblue;
display: flex;
justify-content: center;
align-items: center;
font-size: 18px;
}
</style>
</head>
<body>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
</div>
</body>
</html>
In this example:
- The three boxes are evenly spaced and centered both vertically and horizontally using
justify-content
andalign-items
.
Conclusion
CSS Flexbox is a game-changer for creating flexible and responsive layouts with minimal effort. By mastering the use of Flex containers, items, and alignment properties, you can build layouts that adapt smoothly to different screen sizes and content requirements.
Start experimenting with Flexbox in your projects and experience how it simplifies complex layouts. In the next blog, we’ll explore CSS Grid, another powerful layout tool that works perfectly alongside Flexbox. Keep practicing, and enjoy your Flexbox journey!
With these exercises and examples, you’ll be well on your way to mastering Flexbox for modern web design. Happy coding!
Subscribe to my newsletter
Read articles from Surya Durgesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
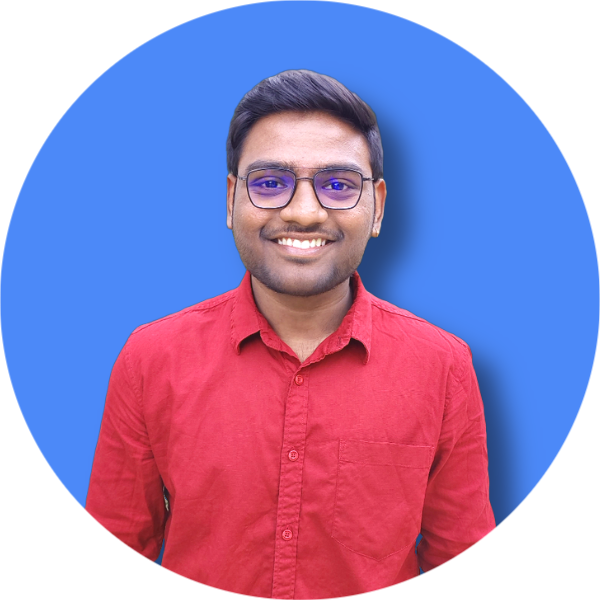
Surya Durgesh
Surya Durgesh
I'm always excited about the possibilities of learning and always open to new ideas