01.Variables and Datatypes
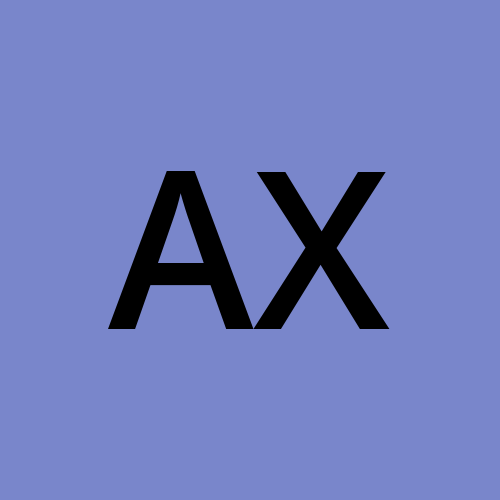
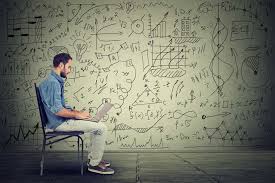
Data Types in JavaScript
JavaScript supports several types of data that variables can store. Here are the main ones, along with examples:
1. Numbers
Numbers in JavaScript can be either integers or floating-point values. JavaScript treats both as the same type, so there is no separate int
or float
.let count = 10; // Integer let temperature = 98.6; // Floating-point number
2. String
Strings represent textual data, enclosed either in single ('
) or double ("
) quotes.
Example:let greeting = "Hello, World!"; // String variable
3. Boolean
Boolean values can only be either true
or false
, used primarily for conditional statements.
Example:let isJavaScriptFun = true; // Boolean variable
4. Undefined
A variable is undefined
if it has been declared but not assigned a value.
Example:let result; // Undefined variable console.log(result); // Output: undefined
5. Null
The null
type represents the intentional absence of any object value. It is often used when we want to explicitly clear a variable.
Example:let user = null; // Null variable
6. Object Literals
Objects in JavaScript are a collection of properties, where each property is defined by a key-value pair. An object can hold various data types.
Example:const person = {
firstName: "John", lastName: "Doe", age: 30, isMarried: true }; // Object literal
Note:
"null" simply means the value of nothing, while "undefined" refers to the absence of value
If you want to build something(Website), the first thing that you do is reserve memory and we have various ways to do this in JavaScript
var, let, and const
1. var
Declaration
The var
keyword is one of the oldest ways to declare variables in JavaScript. Variables declared using var
are function-scoped and can be redeclared or updated.var name = "John"; // Declaring and initializing a string variable name = "Doe"; // Reassigning a new value
2. let
Declaration
Introduced in ES6, let
is block-scoped, meaning the variable only exists within the surrounding block or curly braces {}
. It cannot be redeclared in the same scope, though it can be updated.let age = 25; // Declaring a number variable age = 30; // Reassigning a new value
3. const
Declaration
The const
keyword is used to declare a constant variable, which cannot be reassigned after its initial value. Like let
, it is block-scoped.const birthYear = 1995; // Declaring a constant // birthYear = 2000; // This will throw an error, as constants can't be reassigned
The Difference Between var
, let
, and const
Scope:
var
is function-scoped.let
andconst
are block-scoped.
Redeclaration:
var
can be redeclared and updated.let
can be updated but not redeclared in the same scope.const
can neither be redeclared nor updated.
Hoisting:
var
is hoisted, meaning it is accessible before its declaration, albeit with anundefined
value.let
andconst
are also hoisted but are not initialized, so accessing them before declaration results in aReferenceError
.
Subscribe to my newsletter
Read articles from Alex Xela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
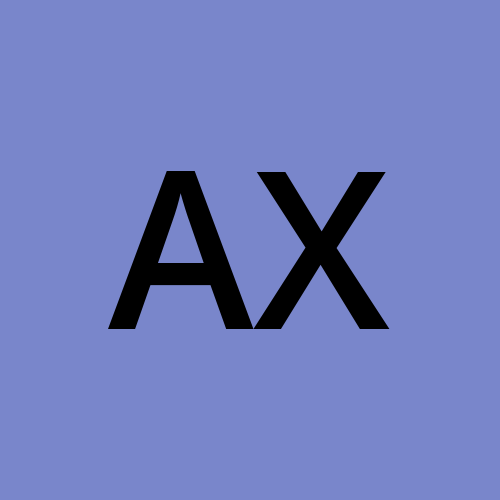