Profiling WebAssembly with pprof and wzprof
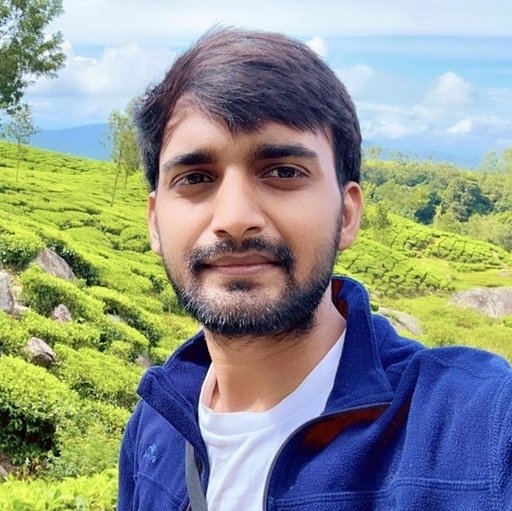
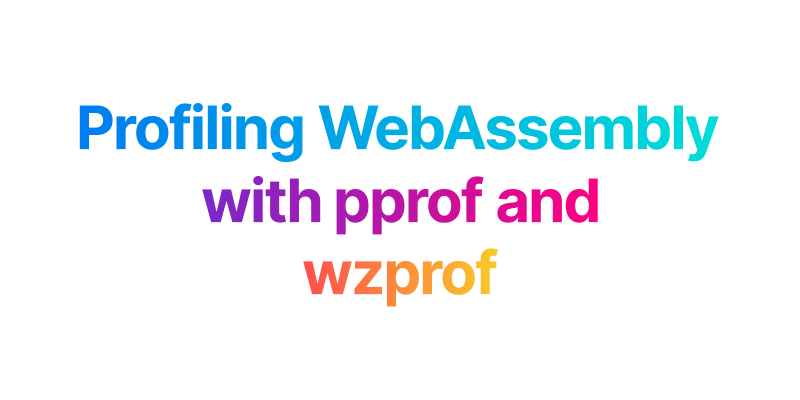
In modern software engineering, profiling is a critical practice for understanding and optimizing the performance of applications. While languages like Go have powerful built-in tools like pprof, WebAssembly introduces a new realm of cross-platform execution that benefits from tools like wzprof.
What is Go?
Go, also known as Golang, is a programming language designed by Google in 7 and open-sourced in 2009. It has gained popularity due to its simplicity, efficiency, and strong concurrency model, making it a go-to language for building scalable, high-performance applications.
Multi-paradigm: Go supports procedural, functional, and concurrent programming styles.
Memory safety: It features garbage collection and automatic memory management.
Portability: Go compiles to multiple platforms, including WebAssembly, making it versatile for both server and web environments.
Tooling: Go provides tools for building, testing, profiling, and managing dependencies through the Go toolchain. For WebAssembly, this toolchain can still be utilized, meaning developers can use Go’s profiling tools even when their applications run in a WebAssembly environment.
Profiling with pprof
pprof is one of the most widely used tools for profiling applications written in Go. It allows developers to measure CPU usage, memory allocations, blocking operations, and goroutines in real time.
CPU Profiling: Measures the time spent in different parts of the application.
Memory Profiling: Tracks heap allocations and helps identify memory usage hotspots.
Block Profiling: Identifies goroutines blocked on synchronization primitives like mutexes.
Goroutine Profiling: Examines the state of goroutines to diagnose performance issues.
To use pprof, the Go runtime exposes profiling data via HTTP endpoints. This can be analyzed through the command-line interface or visualized in the browser.
WebAssembly, although a different compilation target, benefits from pprof as it can expose similar profiling data.
What is WebAssembly?
WebAssembly (Wasm) is a compact, binary instruction format that runs in a stack-based virtual machine. Originally designed for web browsers, Wasm has evolved to support a wide range of platforms, including servers, embedded systems, and more.
Portable bytecode: WebAssembly modules are compiled to a low-level, efficient bytecode that can be executed on any host supporting a Wasm runtime.
Sandboxed execution: WebAssembly provides strong isolation, making it secure for running untrusted code in various environments.
Host functions: Wasm modules can communicate with the host environment through imported and exported functions, enabling interaction with native systems.
In the Go ecosystem, Wasm serves as a compilation target via GOOS=wasip1 GOARCH=wasm
. This allows Go applications to run in the Wasm environment while retaining access to powerful profiling tools like pprof and wzprof.
Profiling WebAssembly with wzprof
wzprof is a specialized profiling tool for WebAssembly, bringing the power of pprof to Wasm modules, regardless of the language they were originally written in. It is based on Wazero, a lightweight WebAssembly runtime written in Go, and outputs profiles in a format compatible with existing pprof tools.
Cross-language profiling: While pprof is traditionally used in Go applications, wzprof extends its functionality to WebAssembly modules written in other languages, such as Rust, C, or Kotlin.
Function instrumentation: wzprof instruments all WebAssembly function calls, capturing stack traces and profiling their execution, similar to how pprof works for Go applications.
pprof endpoints: By exposing HTTP endpoints compatible with pprof, wzprof enables integration with continuous profiling systems like Grafana’s Pyroscope or Polar Signals’ Parca.
CPU and Memory Profiling with wzprof
When profiling a WebAssembly module with wzprof, two key types of profiling are supported:
- CPU Profiling: Tracks how much time the CPU spends on different WebAssembly functions.
- Memory Profiling: Monitors memory allocations and helps detect memory usage patterns.
wzprof uses the function listener in Wazero to instrument the WebAssembly module execution. It doesn’t matter which language the Wasm module was compiled from—wzprof treats it as bytecode and instruments all function calls uniformly.
Generating Profiles Using wzprof
Let's walk through generating CPU and memory profiles for a simple Go program compiled to WebAssembly.
Write the Go code:
package main
import "fmt"
func main() {
fmt.Println("hello, wzprof")
}
Build the Go code into WebAssembly:
GOOS=wasip1 GOARCH=wasm go build -o main.wasm main.go
Run wzprof to create CPU and memory profiles:
wzprof -sample 1 -memprofile ./memprofile.out ./main.wasm
wzprof -sample 1 -cpuprofile ./cpuprofile.out ./main.wasm
In these commands:
The -sample 1 flag indicates the sampling rate (1 sample per second).
The -memprofile and -cpuprofile flags specify the file paths for the memory and CPU profiles, respectively.
Visualizing the Memory Profile
Once you have generated the memory profile, you can visualize it using Go’s built-in tools.
go tool pprof -http :4001 memprofile.out
Above command launches a local HTTP server to display the memory profile in an interactive web interface. You can explore the memory allocations and identify potential memory leaks or inefficiencies.
The Future of Profiling WebAssembly with wzprof
wzprof represents a significant leap in WebAssembly profiling. It opens new possibilities for cross-language profiling in server environments, browser-based applications, and embedded systems. As we move forward, several exciting developments lie ahead:
Deeper integration: Profiling from the guest module, through host functions, down to the kernel level, could be a game-changer.
eBPF and WebAssembly: As profiling with eBPF gains traction, it could eventually intersect with WebAssembly profiling, providing even more granular insights.
Support for interpreted languages: Future versions of wzprof may extend to profiling interpreted languages running in WebAssembly, such as Python or JavaScript.
Conclusion
Profiling WebAssembly applications is crucial for building efficient, performant systems. Tools like pprof and wzprof offer powerful ways to monitor CPU and memory usage in Wasm modules. Whether you’re optimizing a Go application or profiling modules from other languages, these tools provide the insights necessary to improve your application’s performance.
Thank you 😊 for taking the time ⏰ to read this blog post 📖. I hope you found the information 📚 helpful and informative 🧠. If you have any questions ❓ or comments 💬, please feel free to leave them below ⬇️. Your feedback 📝 is always appreciated.
Subscribe to my newsletter
Read articles from Rajiv Ranjan Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
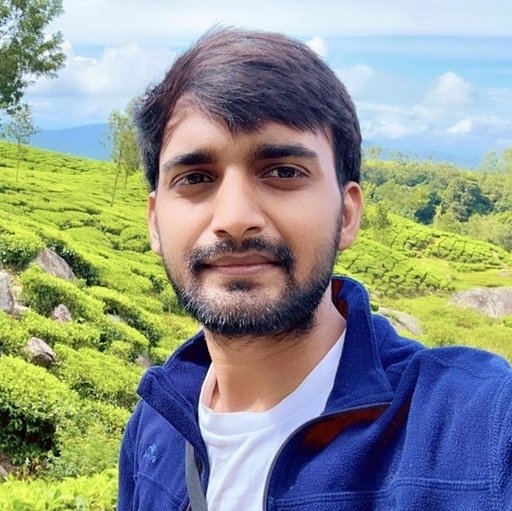
Rajiv Ranjan Singh
Rajiv Ranjan Singh
SDE JL2 @A.P. Moller - Maersk Ex @Lummo | GSoC '23 Mentor @Jenkins | GSoC '22 @Keptn | Ex SWE Intern @redBus, @LocalStack & @Economize | LFX '21 @moja global | GSoD '21 @Wechaty | GSoD'20 @gRPC-Gateway | ICPC Regionalist 2020 I write software for a living, and I've primarily worked on "backend" systems & infrastructure. I currently work at A.P. Moller - Maersk. Interested in Algorithms, Distributed Systems, & Databases.