elixir 101
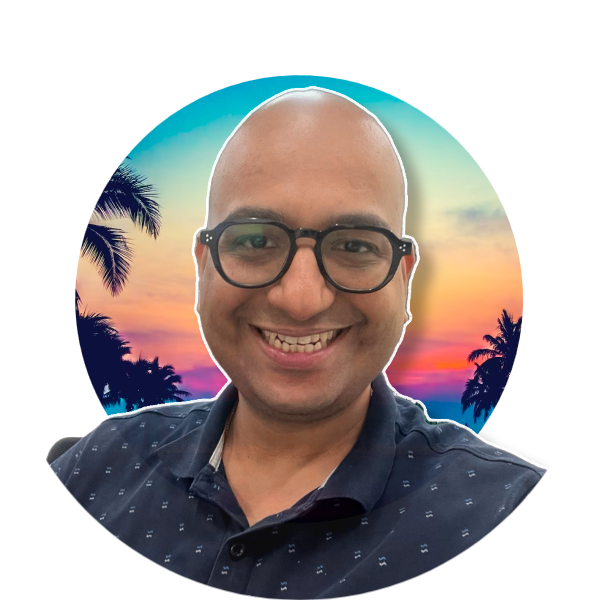
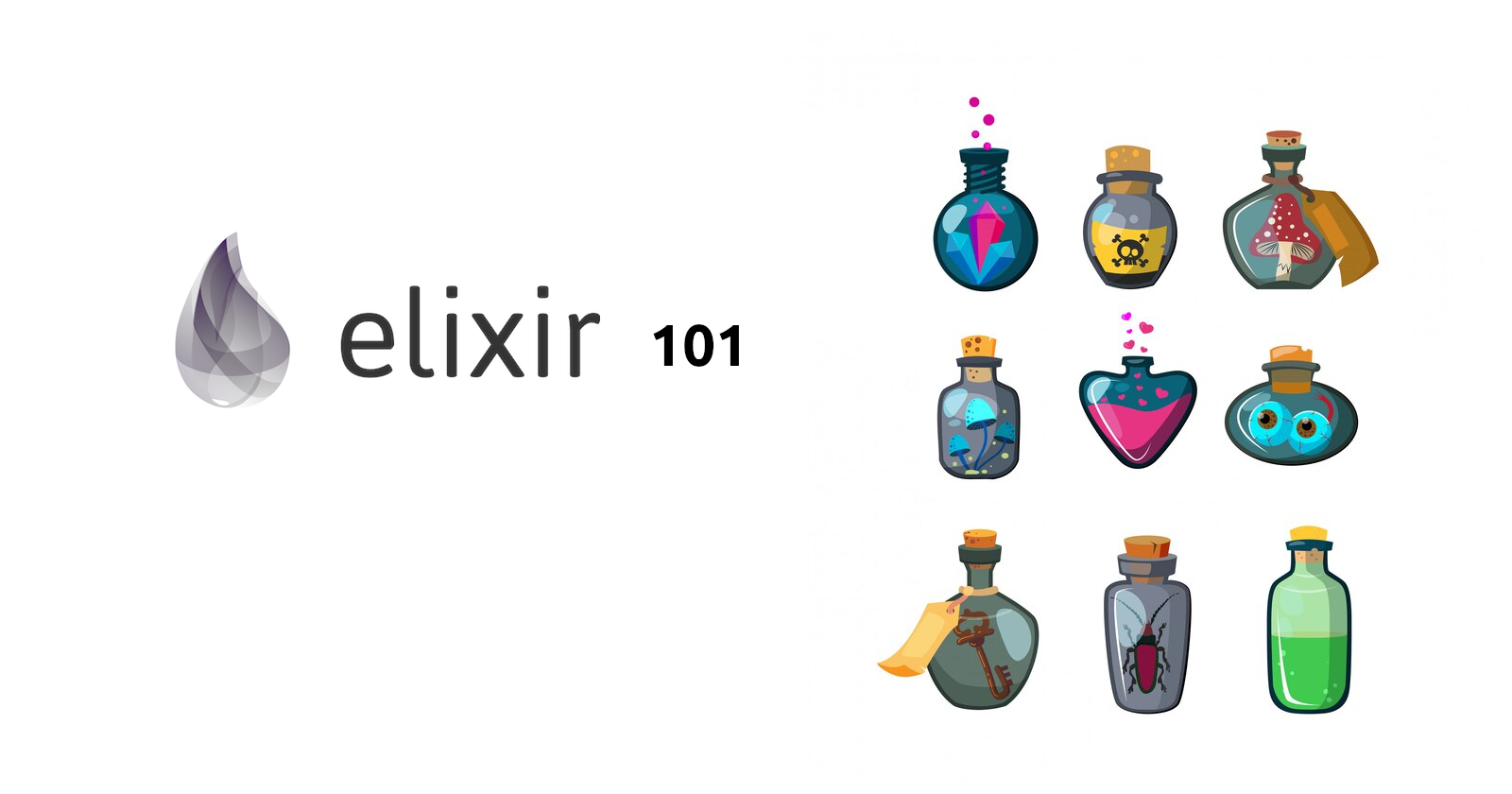
Elixir is a functional, concurrent language built on the Erlang VM (BEAM), known for its fault tolerance and scalability. To get started you'll need to understand basic syntax, concurrency, pattern matching, and working with data structures.
1. Basic Syntax
- Variables and Immutability: Variables are immutable. Once you bind a value to a variable, it cannot be changed.
x = 5
# x = 6 (will not work)
- Atoms: Constants whose name is their value, often used as labels or keys.
:ok
:error
- Tuples: Fixed-size collections of values.
{:ok, "Success"}
{:error, "Something went wrong"}
- Lists: Linked lists, frequently used for collections of data.
[1, 2, 3, 4]
- Maps: Key-value stores for more complex data.
%{name: "John", age: 30}
2. Pattern Matching
- Fundamental to Elixir, it’s used to de-structure data and bind variables.
{:ok, result} = {:ok, 42}
3. Control Flow
case
: Used for branching logic based on pattern matching.
case x do
1 -> "one"
2 -> "two"
_ -> "something else"
end
cond
: Similar toelse if
in other languages.
cond do
x == 1 -> "one"
x == 2 -> "two"
true -> "something else"
end
with
: Chains pattern matching expressions, simplifying nestedcase
statements.
with {:ok, result} <- some_function(),
{:ok, processed} <- another_function(result) do
{:ok, processed}
else
_ -> {:error, "Something went wrong"}
end
4. Functions
- Functions are first-class citizens and can be defined with multiple clauses using pattern matching.
defmodule Math do
def sum(a, b), do: a + b
def factorial(0), do: 1
def factorial(n), do: n * factorial(n - 1)
end
5. Concurrency (Processes)
- Processes: Lightweight and isolated. Use
spawn
to create a new process.
spawn(fn -> IO.puts("Hello from another process") end)
- Messaging: Processes communicate via message passing.
send(self(), {:hello, "world"})
receive do
{:hello, msg} -> IO.puts(msg)
end
6. Collections
- Enum Module: Provides a set of algorithms for working with collections.
Enum.map([1, 2, 3], fn x -> x * 2 end)
Enum.filter([1, 2, 3, 4], fn x -> rem(x, 2) == 0 end)
7. Mix (Build Tool)
- Elixir projects are managed with
mix
.
mix new my_project
cd my_project
mix compile
8. Testing
- Elixir has built-in support for testing with
ExUnit
.
defmodule MathTest do
use ExUnit.Case
test "sum/2 adds two numbers" do
assert Math.sum(1, 2) == 3
end
end
Advance topics
Advanced OTP (Open Telecom Platform)
- Supervisors, GenServer, GenStage, and more complex process management are key to building robust, fault-tolerant applications. These tools manage how processes are spawned, monitored, and restarted in case of failure.
Metaprogramming
- Elixir allows you to write code that generates code, primarily through macros. This is useful for DSLs (Domain-Specific Languages) and compile-time optimizations but is often complex.
Distributed Systems
- Elixir excels at building distributed systems. Understanding clustering, distributed databases (like Mnesia), and how to build scalable systems across nodes would fall into this category.
NIFs (Native Implemented Functions)
- Integrating with C/C++ code for performance-critical tasks. This requires careful handling due to potential side effects.
Complex Multi-Process Architectures
- Designing and implementing systems with complex inter-process communication, where you need to manage state and concurrency across many processes.
Conclusion
The above concepts should help you build and maintain most Elixir applications, especially in web development, where tools like Phoenix leverage these concepts.
I recommend reading the official docs.
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
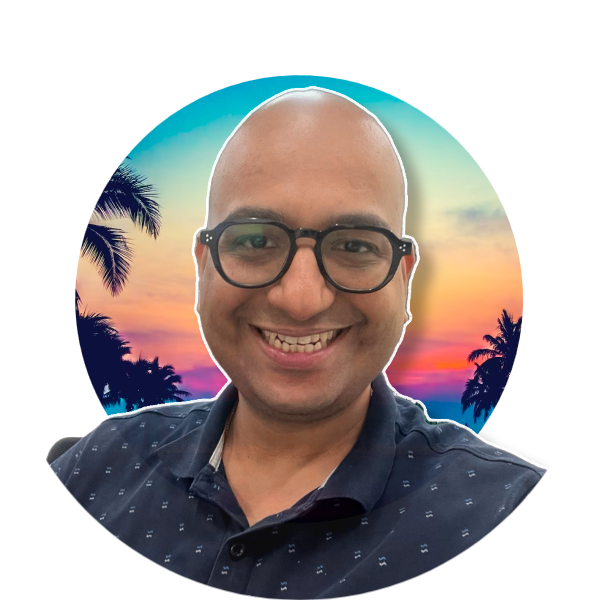
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.