Understanding TypeScript: Applications in Modern Web Development

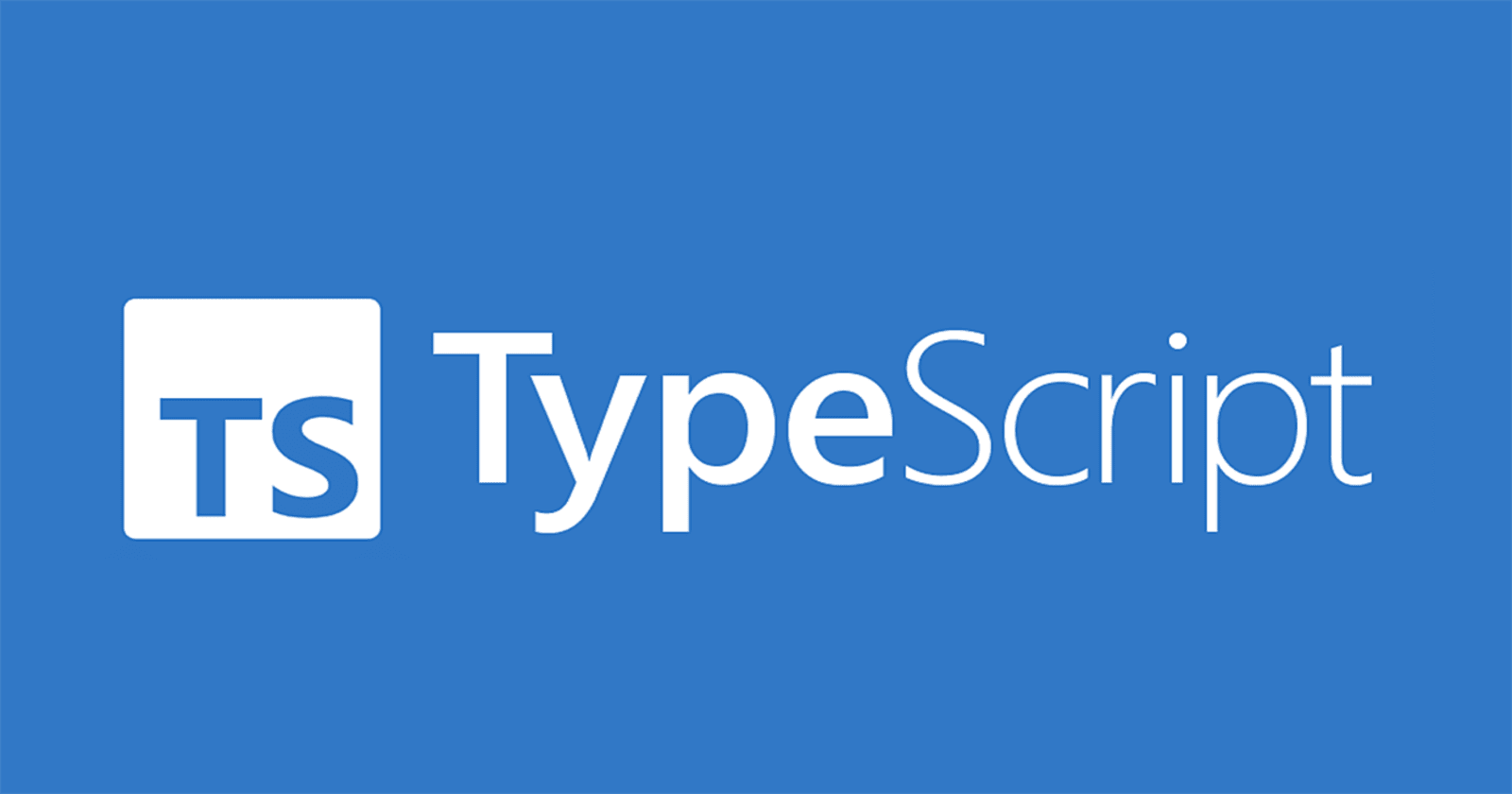
What is TypeScript?
TypeScript, a statically typed superset of JavaScript, was created by Microsoft. It introduces optional type definitions into JavaScript, allowing developers to specify the types of variables, function parameters, and return values. This type checking helps to detect mistakes during development rather than at runtime, making TypeScript an effective tool for developing large and complicated applications.
Pros and Cons of TypeScript
Pros
Static Typing: TypeScript allows developers to specify the types of variables and function parameters, which reduces the possibility of runtime mistakes. This is especially useful in huge codebases where understanding variable types can be tricky.
Improved Tooling and Autocompletion: TypeScript type definitions improve IDE support, allowing for greater autocompletion, navigation, and refactoring capabilities. Developers can develop code more quickly and with fewer errors.
Better Readability and Maintainability: The specified types make the code more readable and understandable. This is very handy when introducing new developers to a project or reviewing old code.
Early Error Detection: TypeScript performs type checks at compile time, allowing developers to catch errors early in the development process, which can significantly reduce debugging time.
Rich Ecosystem: TypeScript is widely used in the JavaScript ecosystem, supported by prominent frameworks and libraries such as React, Angular, and Vue. Many libraries include type definitions, allowing for simple integration
Cons
Learning Curve: For developers used to dynamic typing in JavaScript, the switch to TypeScript can be difficult. Understanding types, interfaces, and generics may take more time and effort.
Initial Setup and Configuration: TypeScript requires an initial setup, including configuration files and build tools, which can be cumbersome for smaller projects or for developers new to the ecosystem.
Compilation Step: Unlike JavaScript, which runs directly in the browser, TypeScript needs to be compiled into JavaScript. This adds an extra step in the development process.
Building a Login Page Using React and TypeScript
Let’s explore how to create a simple login page using React with TypeScript. This example will highlight the benefits of TypeScript while also demonstrating how it integrates seamlessly with React.
Setting Up Your Project
First, ensure you have Node.js installed. You can create a new React project with TypeScript using Create React App by running the following command:
npx create-react-app my-login-app --template typescript
Creating the Login Component
Navigate to your project directory and create a new component called Login.tsx in the “src” folder.
// src/Login.tsx import React, { useState } from 'react'; const Login: React.FC = () => { const [email, setEmail] = useState<string>(''); const [password, setPassword] = useState<string>(''); const [error, setError] = useState<string | null>(null); const handleSubmit = (event: React.FormEvent<HTMLFormElement>) => { event.preventDefault(); if (!email || !password) { setError('Please fill in all fields'); return; } // Handle login logic here console.log('Logging in with:', email, password); setError(null); // Clear error on successful submission }; return ( <form onSubmit={handleSubmit}> <h1>Login</h1> {error && <p style={{ color: 'red' }}>{error}</p>} <div> <label htmlFor="email">Email:</label> <input type="email" id="email" value={email} onChange={(e) => setEmail(e.target.value)} required /> </div> <div> <label htmlFor="password">Password:</label> <input type="password" id="password" value={password} onChange={(e) => setPassword(e.target.value)} required /> </div> <button type="submit">Login</button> </form> ); }; export default Login;
Integrating the Login Component
Now, integrate the
Login
component into your main application file,App.tsx
.//src/App.tsx import React from 'react'; import Login from './Login'; const App: React.FC = () => { return ( <div> <Login /> </div> ); }; export default App;
Running Your Application
Finally, run your application using:
bashCopy codenpm start
You should see a simple login form with email and password fields. TypeScript ensures that you cannot submit the form without filling in both fields, providing immediate feedback.
Conclusion
TypeScript is an invaluable element in modern web development, particularly for projects that use frameworks like as React. Its static typing, enhanced tooling, and error detection features improve the developer experience and result in more maintainable code.
Developers may construct robust apps with confidence while using TypeScript, knowing that possible flaws will be identified early in the development process. Whether you're developing small components or large-scale apps, TypeScript may drastically enhance your workflow and code quality.
Subscribe to my newsletter
Read articles from Omkar Mante directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
