"Understanding Unit Testing in Python: Writing Test Cases with unittest for Caesar Cipher"
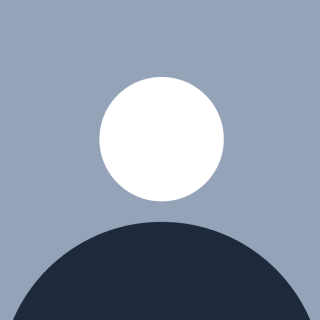
“unittest” is a Python's built-in testing framework. It helps write and organize test cases to validate that the code behaves as expected.
We're importing the encryption and decryption functions from the “ceaser.py” module.
import unittest from ceaser import encryption,decryption
“TestCaesarCipher” class defines the test cases and It inherits from unittest.Testcase*, which gives it access to various testing methods. And also provides methods to compare actual and expected outputs.*
class TestCaesarCipher(unittest.TestCase):
“self.assertEqual” compares two values. If the values are equal, the test passes; if not, the test fails. For example:
The string ”hello world” with a shift of 3 should be encrypted to “khoor zruog”.
Special characters like commas and spaces remain unchanged (e.g., ”hello, world!” becomes “mjqqt, btwqi!” with a shift of 5)
def test_encryption(self):
self.assertEqual(encryption("hello world", 3), "khoor zruog")
self.assertEqual(encryption("hello, world!", 5), "mjqqt, btwqi!")
def test_decryption(self):
self.assertEqual(decryption("khoor zruog", 3), "hello world")
self.assertEqual(decryption("mjqqt, btwqi!", 5), "hello, world!")
if __name__==”__main__” ensures that allows the code to run tests when we run the script but not when the script is imported as a module.
unittest.main() method runs all the test cases within the TestCaeserCipher class.
if __name__ == '__main__': unittest.main()
In short, testing ensures that your code behaves as expected, reduces bugs, and helps maintain high code quality over time.
Subscribe to my newsletter
Read articles from Arunmathavan K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by