OOP Basics in Java for Beginners Introduction to Object-Oriented Programming (OOP)

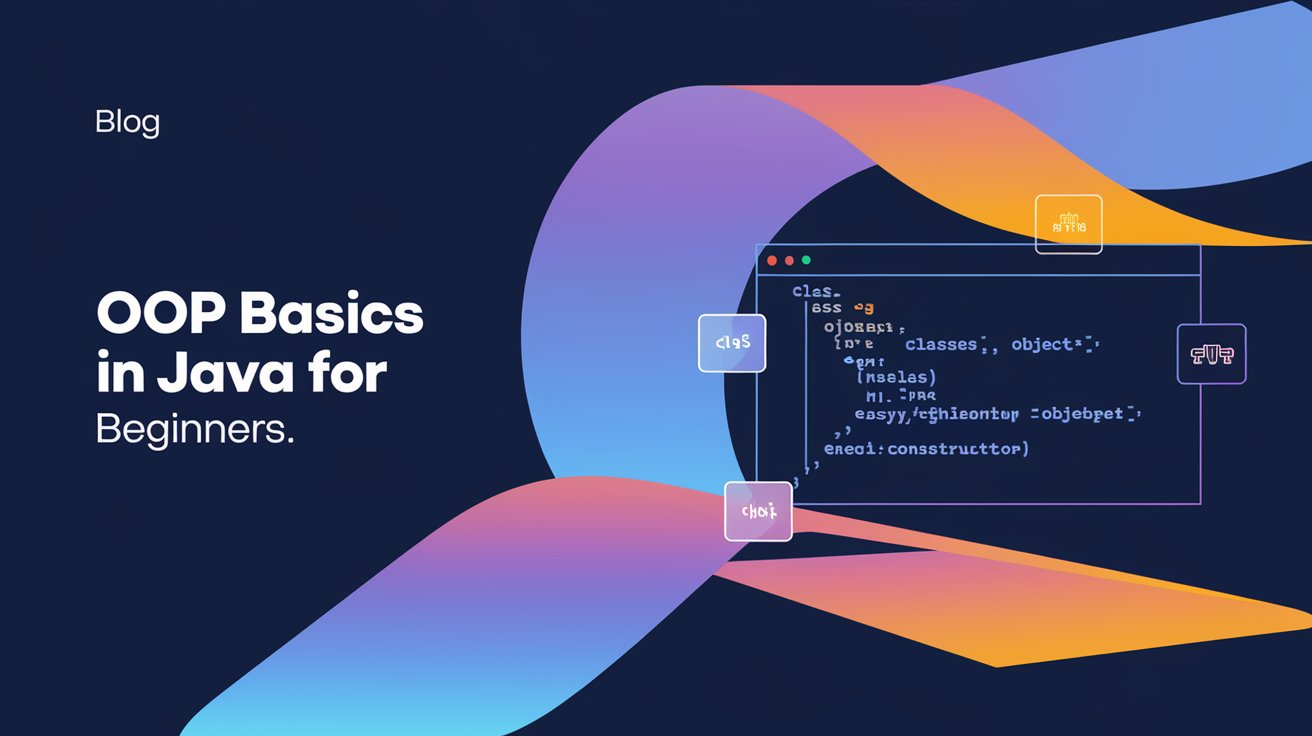
Object-Oriented Programming (OOP) is a programming paradigm that uses "objects" to represent data and methods. It enables better organization of code, promotes code reuse, and makes complex systems easier to manage. Java is one of the most popular languages that support OOP principles, which include encapsulation, inheritance, and polymorphism.
Key Concepts of OOP
Classes: A class is a blueprint for creating objects. It defines a data type by bundling data (attributes) and methods (functions) that operate on the data.
Objects: An object is an instance of a class. It is created using the class blueprint and can hold data and perform methods defined in the class.
Methods: Methods are functions defined within a class that describe the behaviors of an object. They can manipulate the object's data and can return values.
Constructors: A constructor is a special method that is called when an object is created. It initializes the object's properties and allocates memory.
The
this
Keyword: Thethis
keyword is a reference to the current object. It is used to differentiate between instance variables and parameters or to invoke other constructors.
Understanding Classes
Here’s a simple example of a class definition in Java:
public class Car {
// Attributes
String color;
String model;
// Constructor
public Car(String color, String model) {
this.color = color; // Using 'this' keyword to refer to the current object's color
this.model = model; // Using 'this' keyword to refer to the current object's model
}
// Method
public void displayInfo() {
System.out.println("Car Model: " + model + ", Color: " + color);
}
}
Breakdown of the Car Class
Attributes:
color
andmodel
are instance variables that hold the state of the Car object.Constructor:
public Car(String color, String model)
is a constructor that initializes the attributes of the Car object.Method:
displayInfo()
is a method that prints out the car's details.
Creating Objects
You can create an object of the Car
class like this:
public class Main {
public static void main(String[] args) {
// Creating an object of the Car class
Car myCar = new Car("Red", "Toyota");
myCar.displayInfo(); // Output: Car Model: Toyota, Color: Red
}
}
Explanation
The
new
keyword is used to create an instance of theCar
class.The constructor initializes the
color
andmodel
attributes formyCar
.The
displayInfo()
method is called to print the car's information.
Methods in Java
Methods allow you to define actions that can be performed on an object. Here’s an example of a class with multiple methods:
public class Calculator {
// Method to add two numbers
public int add(int a, int b) {
return a + b;
}
// Method to subtract two numbers
public int subtract(int a, int b) {
return a - b;
}
}
Using the Calculator Class
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
System.out.println("Addition: " + calc.add(5, 3)); // Output: Addition: 8
System.out.println("Subtraction: " + calc.subtract(5, 3)); // Output: Subtraction: 2
}
}
Constructors in Java
Constructors are special methods used to initialize objects. If you do not define a constructor, Java provides a default constructor. Here’s how you can define a parameterized constructor:
public class Person {
String name;
// Constructor
public Person(String name) {
this.name = name;
}
}
The this
Keyword
The this
keyword is essential when the parameter name is the same as the instance variable name. It helps to distinguish between the two. Here’s an example:
public class Employee {
String name;
// Constructor
public Employee(String name) {
this.name = name; // 'this.name' refers to the instance variable
}
}
Conclusion
Understanding the basics of OOP in Java is crucial for building structured and efficient applications. By mastering classes, objects, methods, constructors, and the this
keyword, you lay a solid foundation for more advanced topics in Java programming.
Whether you're creating simple applications or complex systems, OOP principles will help you write cleaner and more maintainable code.
Subscribe to my newsletter
Read articles from Sabat Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
