02. Operators
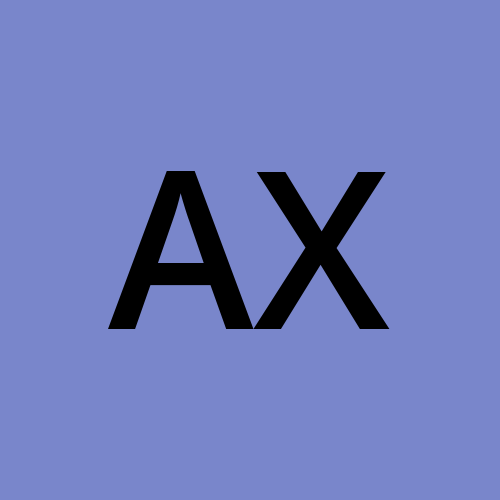
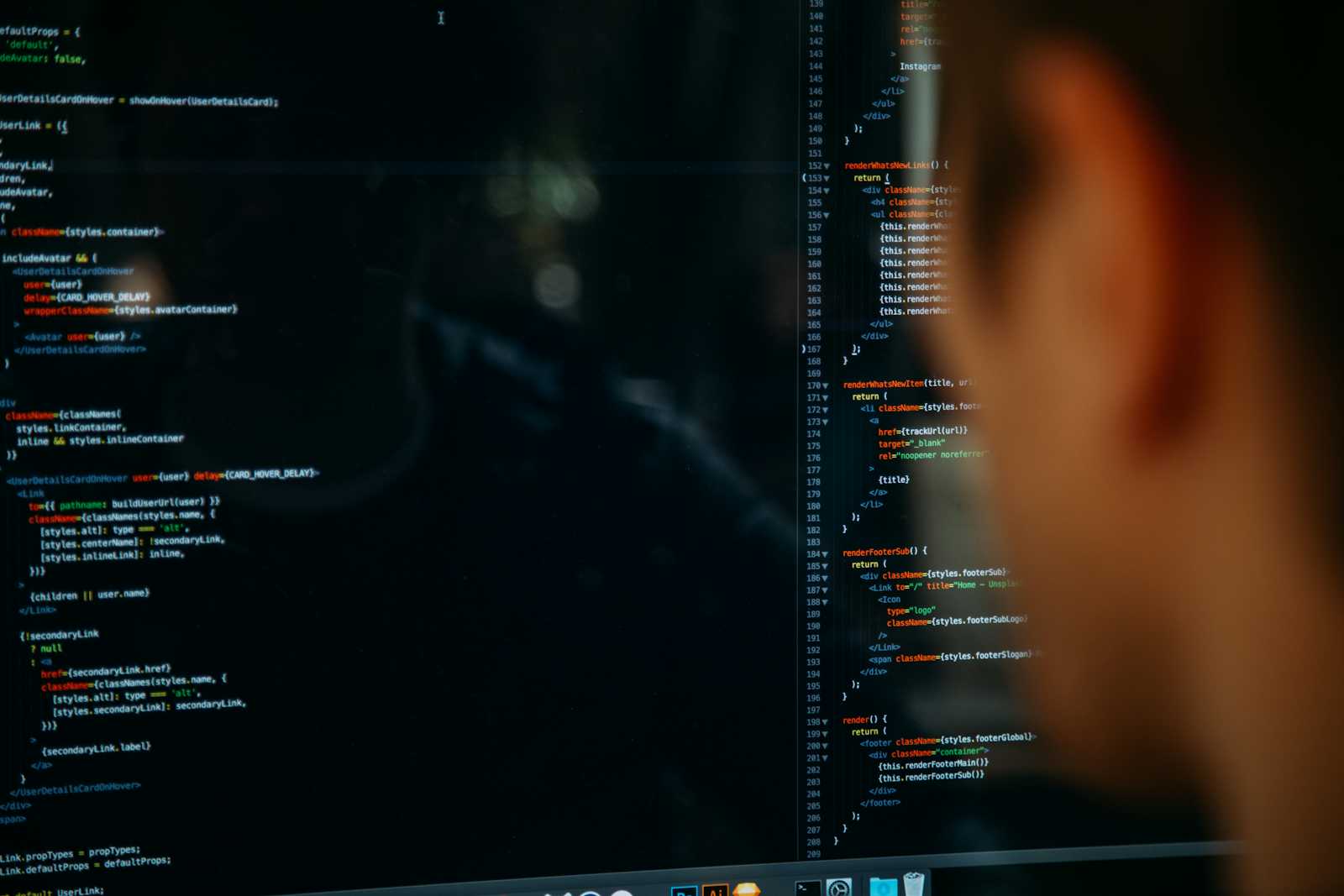
In JavaScript, operators are used to perform various operations on variables and values. In this article, we will explore the different types of operators in JavaScript, including unary, arithmetic, relational, equality, binary logical, and conditional operators.
Unary Operators
Unary operators are used with a single operand. Here are some examples of unary operators in JavaScript:
1. delete
The delete
operator deletes a property from an object.let person = { name: 'John', age: 30 };
delete person.age;
console.log(person); // Output: { name: 'John' }
2. void
The void
operator evaluates the given expression and returns undefined
.let x = void (10);
console.log(x); // Output: undefined
3. typeof
The typeof
operator determines the type of a given object.let x = 10; console.log(typeof x); // Output: "number"
4. +
(Unary Plus)
The unary plus operator converts its operand to Number type.let x = '10'; console.log(+x); // Output: 10
5. -
(Unary Negation)
The unary negation operator converts its operand to Number type and then negates it.let x = 10;
console.log(-x); // Output: -10
6. ~
(Bitwise NOT)
The bitwise NOT operator performs a bitwise NOT operation on its operand.let x = 10; console.log(~x); // Output: -11
7. !
(Logical NOT)
The logical NOT operator returns the opposite boolean value of its operand.let x = true; console.log(!x); // Output: false
Arithmetic Operators
Arithmetic operators take numerical values (either literals or variables) as their operands and return a single numerical value. Here are some examples of arithmetic operators in JavaScript:
1. **
(Exponentiation)
The exponentiation operator returns the result of raising the first operand to the power of the second operand.let x = 2; let y = 3; console.log(x ** y); // Output: 8
2. *
(Multiplication)
The multiplication operator returns the product of its operands.let x = 2; let y = 3; console.log(x * y); // Output: 6
3. /
(Division)
The division operator returns the quotient of its operands.let x = 6; let y = 2; console.log(x / y); // Output: 3
4. %
(Remainder)
The remainder operator returns the remainder of dividing the first operand by the second operand.let x = 7; let y = 2; console.log(x % y); // Output: 1
5. +
(Addition)
The addition operator returns the sum of its operands.let x = 2; let y = 3; console.log(x + y); // Output: 5
6. -
(Subtraction)
The subtraction operator returns the difference of its operands.let x = 5; let y = 2; console.log(x - y); // Output: 3
Relational Operators
Relational operators compare their operands and return a boolean value based on whether the comparison is true. Here are some examples of relational operators in JavaScript:
1. <
(Less than)
The less-than operator returns true
if the first operand is less than the second operand.let x = 2; let y = 3; console.log(x < y); // Output: true
2. >
(Greater than)
The greater than operator returns true
if the first operand is greater than the second operand.let x = 3; let y = 2; console.log(x > y); // Output: true
3. <=
(Less than or equal)
The less than or equal operator returns true
if the first operand is less than or equal to the second operand.let x = 2; let y = 2; console.log(x <= y); // Output: true
4. >=
(Greater than or equal)
The greater than or equal operator returns true
if the first operand is greater than or equal to the second operand.let x = 3; let y = 3; console.log(x >= y); // Output: true
Equality Operators
The result of evaluating an equality operator is always of type boolean based on whether the comparison is true. Here are some examples of equality operators in JavaScript:
1. ==
(Equality)
The equality operator returns true
if both operands are equal.let x = 2; let y = 2; console.log(x == y); // Output: true
2. !=
(Inequality)
The inequality operator returns true
if both operands are not equal.let x = 2; let y = 3; console.log(x != y); // Output: true
3. ===
(Strict Equality)
The strict equality operator returns true
if both operands are equal and of the same type.let x = 2; let y = 2; console.log(x === y); // Output: true
4. !==
(Strict Inequality)
The strict inequality operator returns true
if both operands are not equal or of different types.let x = 2; let y = '2'; console.log(x !== y); // Output: true
Binary Logical Operators
Binary logical operators implement boolean (logical) values and have short-circuiting behavior. Here are some examples of binary logical operators in JavaScript:
1. &&
(Logical AND)
The logical AND operator returns true
if both operands are true.let x = true; let y = true; console.log(x && y); // Output: true
2. ||
(Logical OR)
The logical OR operator returns true
if at least one operand is true.let x = true; let y = false; console.log(x || y); // Output: true
3. ??
(Nullish Coalescing)
The nullish coalescing operator returns the first operand if it is not null or undefined, and the second operand otherwise.let x = null; let y = 10; console.log(x ?? y); // Output: 10
Conditional (Ternary) Operator
The conditional operator returns one of two values based on the logical value of the condition.let x = 2; let y = 3; console.log(x > y ? 'x is greater' : 'y is greater'); // Output: "y is greater"
I hope this comprehensive guide to JavaScript operators helps you understand the different types of operators and how to use them effectively in your code.
Subscribe to my newsletter
Read articles from Alex Xela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
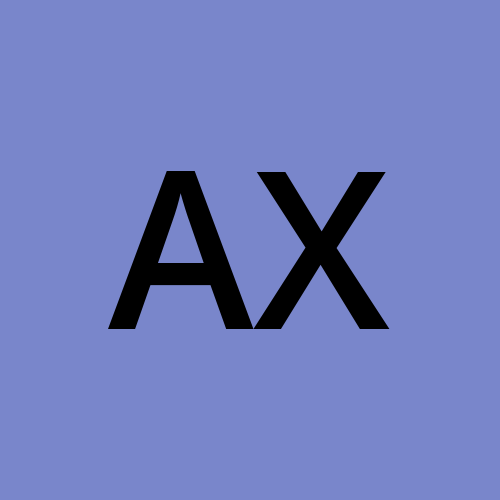