03.Functions
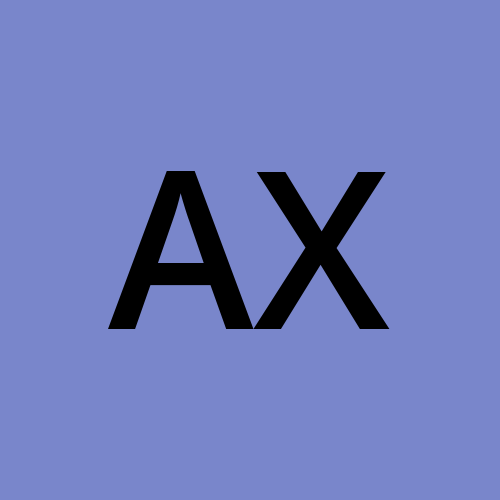
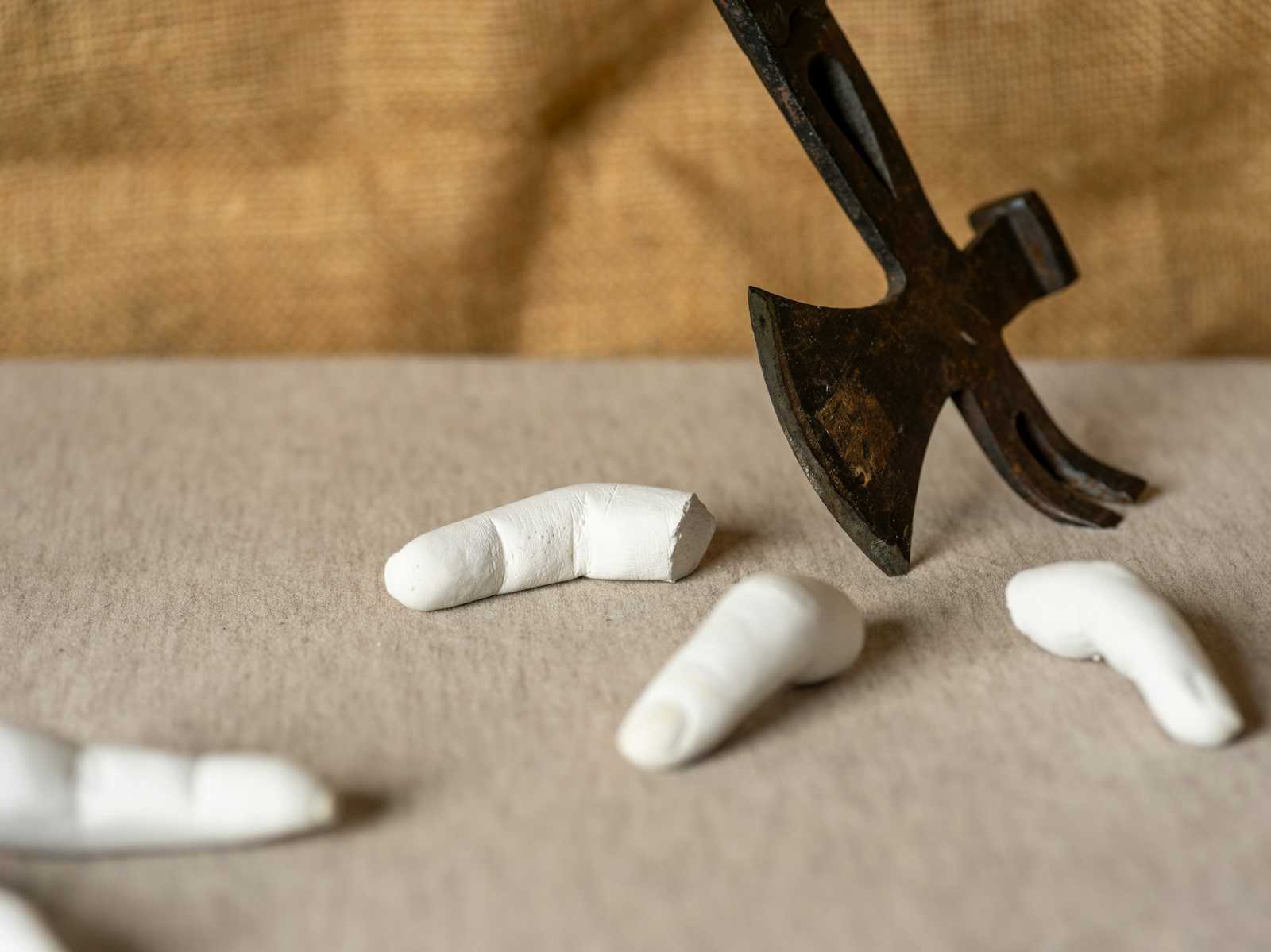
Functions in JavaScript: A Comprehensive Guide
In JavaScript, functions are a fundamental concept that allows you to group a set of statements together to perform a specific task. In this article, we will explore the world of functions in JavaScript, including their syntax, types, and uses.
What is a Function?
A function is a block of code that can be executed multiple times from different parts of your program. It takes in arguments, performs some operations, and returns a value.
Function Syntax
The basic syntax of a function in JavaScript is as follows:function functionName(parameters) { // code to be executed }
Here, functionName
is the name of the function, parameters
are the inputs to the function, and the code inside the curly braces is the function body.
Example: A Simple Function
Let's create a simple function that takes in a name and a boolean value indicating whether the person can solve math problems or not.function educationSystem(name, canSolveMath) { if (cannotSolveMath) { console.log('Dr.' + name); } else { console.log('Er.' + name); } }
educationSystem(Alan, 1)
In this example, the function educationSystem
takes in two parameters: name
and canSolveMath
. It then checks the value of canSolveMath
and logs a message to the console accordingly.
Function Types
There are several types of functions in JavaScript, including:
Named Functions: These are functions that have a name and can be called by that name.function add(x, y) { return x + y; }
Anonymous Functions: These are functions that do not have a name and are often used as event handlers or callbacks.let add = function(x, y) { return x + y; };
Arrow Functions: These are a shorthand way of creating functions that are introduced in ECMAScript 2015.let add = (x, y) => x + y;
Immediately Invoked Function Expressions (IIFE): These are functions that are executed immediately after they are defined.(function() { console.log('Hello, World!'); })();
Subscribe to my newsletter
Read articles from Alex Xela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
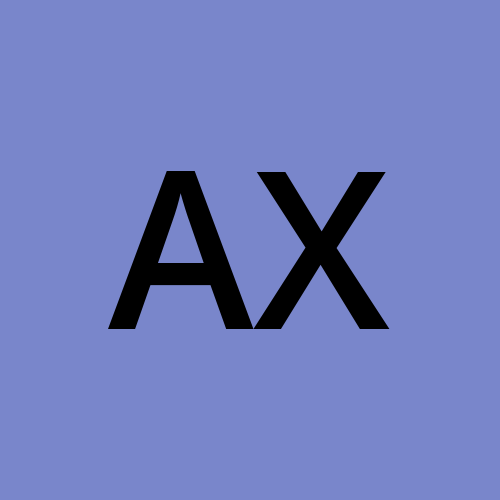