Mastering Order Processing Systems: A Comprehensive Guide to Building Efficient Business Solutions with Python
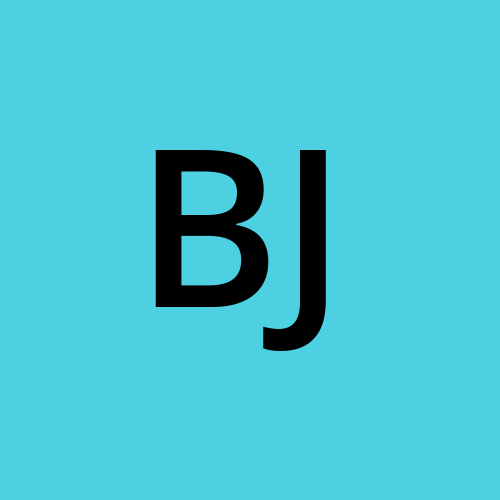
Part 4: Database Design and Initialization
Introduction
In any order processing system, the database plays a vital role as it serves as the foundation for storing and managing critical information such as user details, product inventory, and order data. A well-structured database ensures that the system is efficient, reliable, and scalable. In this part of the blog series, we’ll dive into the database schema used in the project, explain the role of each table, and demonstrate how to initialize the database using Python and SQLite.
Overview of the Database Schema
The database for our order processing system is designed to store information about users, products, orders, and the details of each order. Here's a high-level view of the key tables involved:
users
table: Stores user credentials and role information, ensuring that only authorized personnel can access the system.products
table: Contains information about products such as product ID, name, price, and stock levels.orders
table: Holds data related to customer orders, including order ID, customer name, order date, and status.order_details
table: Records the specific items and quantities for each order.user_registrations
table: Stores information about user registration requests, which are approved or rejected by an admin.
Each of these tables has been designed with specific fields to ensure the integrity of the data and support efficient order processing. Let's now take a deeper look at each of these tables.
Explanation of the Tables
1. Users Table
The users
table is responsible for storing user credentials and roles. The role-based access control (admin, manager, employee) helps secure the system by ensuring that only authorized users can perform certain actions.
Here’s the structure of the users
table:
Column Name | Data Type | Description |
user_id | INTEGER | Primary key, auto-incremented user identifier. |
username | TEXT | Unique username for each user. |
password | TEXT | Encrypted password for authentication. |
role | TEXT | Role of the user (admin, manager, employee). |
2. Products Table
The products
table stores essential information about the products available for purchase, including product identification, pricing, and inventory levels.
Column Name | Data Type | Description |
product_id | TEXT | Primary key, unique identifier for each product. |
name | TEXT | Name of the product. |
price | REAL | Price of the product. |
stock | INTEGER | Current stock level for the product. |
This table helps the system manage inventory levels and ensure that products are available for sale.
3. Orders Table
The orders
table tracks high-level information about customer orders. This includes the order ID, customer details, and order status.
Column Name | Data Type | Description |
order_id | TEXT | Primary key, unique identifier for each order. |
customer_name | TEXT | Name of the customer who placed the order. |
date | TEXT | Date when the order was placed (formatted as a string). |
status | TEXT | Current status of the order (e.g., "Processing", "Shipped"). |
order_status | TEXT | Tracks the progress of the order, default is "Processing". |
4. Order Details Table
The order_details
table is used to track the specific items and their quantities within each order. This table links products to the orders.
Column Name | Data Type | Description |
order_id | TEXT | Foreign key referencing the orders table. |
product_id | TEXT | Foreign key referencing the products table. |
quantity | INTEGER | Quantity of the product ordered. |
The order_details
table allows the system to manage orders containing multiple products.
5. User Registrations Table
The user_registrations
table keeps track of users who have requested access to the system. Admins can approve or reject these registration requests.
Column Name | Data Type | Description |
registration_id | INTEGER | Primary key, auto-incremented ID for registration requests. |
username | TEXT | Username requested by the new user. |
password | TEXT | Password chosen by the new user (stored as a hash). |
email | TEXT | Email address of the new user. |
role | TEXT | Role requested by the new user (admin, manager, employee). |
status | TEXT | Registration status ("Pending", "Approved", "Rejected"). |
This table supports the admin workflow for managing new user registrations.
Code Snippet: init_db()
Function
The init_db()
function is responsible for initializing the database and creating all the necessary tables. Let’s take a look at the code that sets up this schema in SQLite:
def init_db():
conn = sqlite3.connect('order_system.db')
c = conn.cursor()
# Create Users Table
c.execute('''
CREATE TABLE IF NOT EXISTS users (
user_id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT,
role TEXT
)
''')
# Create User Registrations Table
c.execute('''
CREATE TABLE IF NOT EXISTS user_registrations (
registration_id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT,
email TEXT,
role TEXT,
status TEXT
)
''')
# Create Products Table
c.execute('''
CREATE TABLE IF NOT EXISTS products (
product_id TEXT PRIMARY KEY,
name TEXT,
price REAL,
stock INTEGER
)
''')
# Create Orders Table
c.execute('''
CREATE TABLE IF NOT EXISTS orders (
order_id TEXT PRIMARY KEY,
customer_name TEXT,
date TEXT,
status TEXT,
order_status TEXT DEFAULT 'Processing'
)
''')
# Create Order Details Table
c.execute('''
CREATE TABLE IF NOT EXISTS order_details (
order_id TEXT,
product_id TEXT,
quantity INTEGER,
FOREIGN KEY(order_id) REFERENCES orders(order_id),
FOREIGN KEY(product_id) REFERENCES products(product_id)
)
''')
conn.commit()
conn.close()
logging.info("Database initialized")
Running the Script to Create and Initialize the Database
To create and initialize the database, you simply need to run the init_db()
function. This will create all the necessary tables and ensure that your database is ready for storing data.
Here’s how you can run the initialization script:
Create a Python script (e.g.,
db_
setup.py
) that contains theinit_db()
function.Run the script in your terminal:
python db_setup.py
Check the database: After running the script, you should find an
order_system.db
file in your project folder. You can use an SQLite database browser (such as DB Browser for SQLite) to view the tables and their structure.
Conclusion
In this part, we explored the database design of the order processing system, covering the schema and its key components. The init_db()
function plays a crucial role in setting up the system’s foundation, ensuring that the database tables are created properly. Now that our database is initialized, we can begin building the functionality that interacts with these tables, starting with the user interface in the next part.
Stay tuned for the next part, where we’ll start learning how to Populate the Database with Initial Data.
Link to My Source code : https://github.com/BryanSJamesDev/-Order-Processing-System-OPS-/tree/main
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
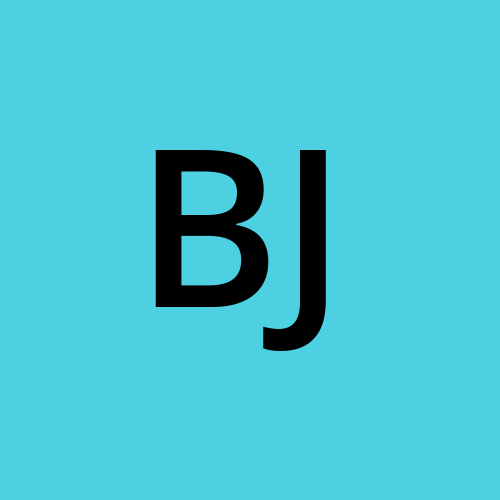